Common Pitfalls in Java Cryptography: Avoiding Security Flaws
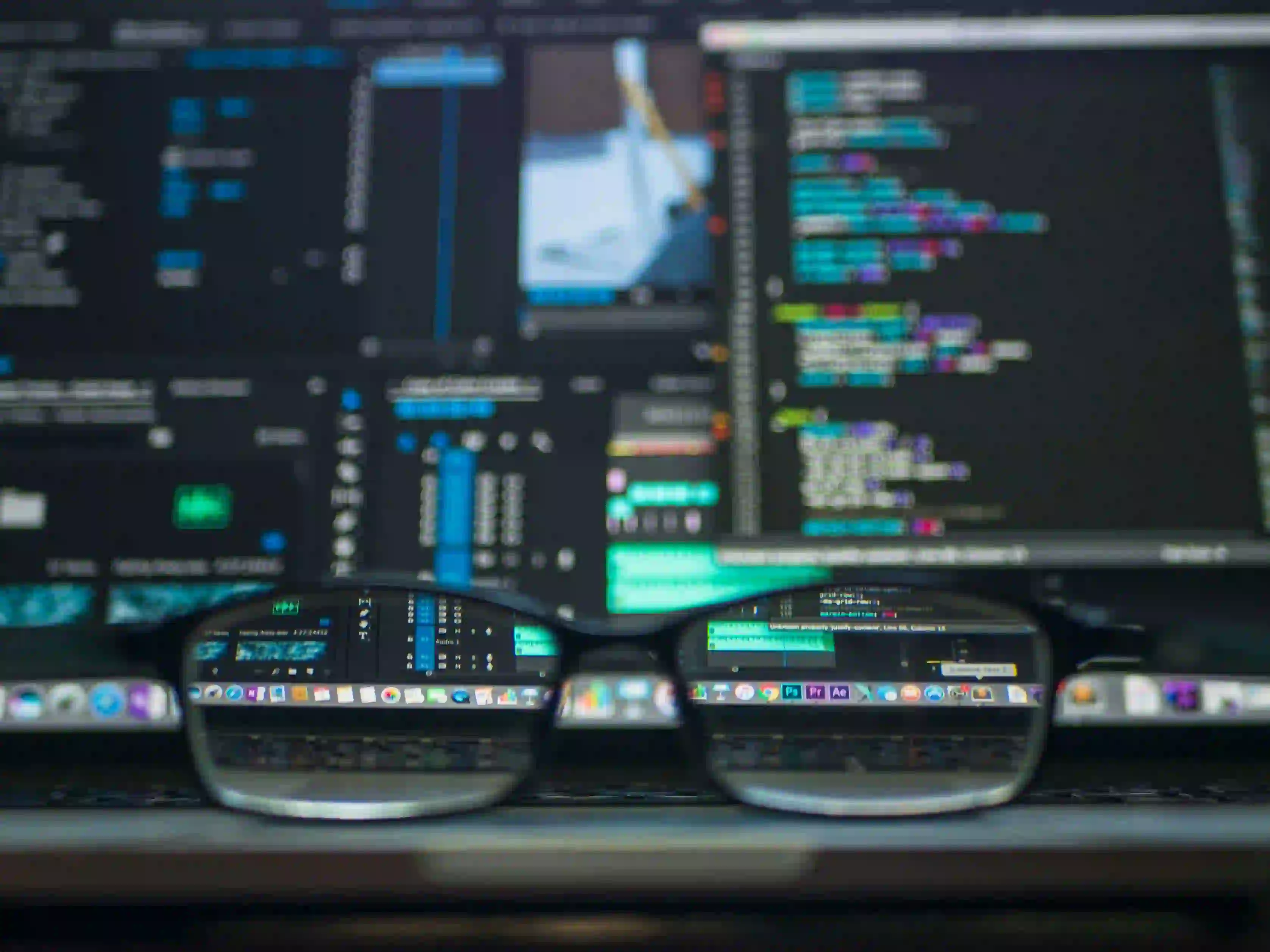
Common Pitfalls in Java Cryptography: Avoiding Security Flaws
Cryptography plays an integral role in maintaining the confidentiality, integrity, and authenticity of data in software systems. In Java, developers have access to a rich set of cryptographic libraries that enable secure communication and data protection. However, even seasoned developers can fall prey to common pitfalls that lead to security vulnerabilities. This blog post aims to highlight these pitfalls, provide solutions, and point you towards best practices for implementing Java cryptography securely.
Understanding Java Cryptography
Java’s cryptography support is primarily provided by the Java Cryptography Architecture (JCA) and Java Cryptography Extension (JCE). Both frameworks allow developers to implement various cryptographic functions such as encryption/decryption, secure hash functions, and digital signatures.
While these libraries are robust, improper usage can result in security flaws. Here are some common pitfalls and how to avoid them.
1. Using Weak or Deprecated Algorithms
The Trap
Using outdated or weak algorithms can significantly compromise the security of your application. For instance, the use of MD5 or SHA-1 hashing algorithms is strongly discouraged due to vulnerabilities that can lead to collision attacks.
The Solution
Always opt for updated, secure algorithms. For hashing, the SHA-256 or SHA-3 families are preferred. For encryption, consider using AES (Advanced Encryption Standard) with a key length of at least 128 bits.
import java.security.MessageDigest;
import java.security.NoSuchAlgorithmException;
public class SecureHash {
public static String hash(String input) throws NoSuchAlgorithmException {
MessageDigest digest = MessageDigest.getInstance("SHA-256");
byte[] hashBytes = digest.digest(input.getBytes());
StringBuilder hexString = new StringBuilder();
for (byte b : hashBytes) {
String hex = Integer.toHexString(0xff & b);
if (hex.length() == 1) hexString.append('0');
hexString.append(hex);
}
return hexString.toString();
}
public static void main(String[] args) {
try {
String hashedPassword = hash("SecurePassword");
System.out.println("Hashed Password: " + hashedPassword);
} catch (NoSuchAlgorithmException e) {
e.printStackTrace();
}
}
}
Commentary: Here, we utilize SHA-256 for hashing instead of MD5 or SHA-1. The hash
method returns a hashed representation of the input. This enhances security, reducing the risk of attacks.
2. Hard-Coding Keys
The Trap
Another common pitfall is hard-coding cryptographic keys directly into your source code. This dramatically increases the risk of key exposure, especially in environments where the code could be accessed by unauthorized parties.
The Solution
Utilize external configuration files or environment variables to manage your keys securely. This approach ensures that keys are not stored within your application and can be replaced or rotated easily.
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import java.nio.file.Files;
import java.nio.file.Paths;
public class KeyManagement {
public static SecretKey generateKey() throws Exception {
KeyGenerator keyGenerator = KeyGenerator.getInstance("AES");
keyGenerator.init(256); // 256-bit key size
return keyGenerator.generateKey();
}
public static void saveKey(SecretKey key) throws Exception {
byte[] keyBytes = key.getEncoded();
Files.write(Paths.get("secret.key"), keyBytes);
}
public static void main(String[] args) {
try {
SecretKey secretKey = generateKey();
saveKey(secretKey);
System.out.println("Secret Key has been generated and saved.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary: The generateKey
method creates a new AES key, which is then saved to an external file. This method of key management prevents direct exposure of keys, aligning with security best practices.
3. Ignoring Java's Security Manager
The Trap
Java provides a Security Manager to enforce access control policies for code running within the Java Virtual Machine (JVM). Ignoring the use of the Security Manager can expose your application to vulnerabilities, especially in a multi-tenant environment.
The Solution
Leverage the Security Manager to define permissions for cryptographic operations. This ensures that only authorized code can perform sensitive actions.
public class SecurityManagerExample {
public static void main(String[] args) {
System.setSecurityManager(new SecurityManager());
try {
// Attempting to access a restricted operation
System.out.println("Attempting access protected resources...");
} catch (SecurityException se) {
System.err.println("Security Exception: " + se.getMessage());
}
}
}
Commentary: Here, we set a Security Manager to enforce permissions. Any unauthorized access attempts will trigger a SecurityException
, highlighting the importance of controlled access.
4. Insecure Random Number Generation
The Trap
Generating keys or initialization vectors (IVs) using insecure random generators can leave your cryptographic implementations vulnerable. Relying on java.util.Random
or other non-secure sources leads to predictability.
The Solution
Always use SecureRandom
for generating cryptographic keys and IVs:
import java.security.SecureRandom;
import javax.crypto.KeyGenerator;
public class SecureRandomExample {
public static void main(String[] args) {
try {
SecureRandom secureRandom = new SecureRandom();
byte[] randomBytes = new byte[16]; // 16 bytes for AES
secureRandom.nextBytes(randomBytes);
KeyGenerator keyGenerator = KeyGenerator.getInstance("AES");
keyGenerator.init(128, secureRandom); // Using SecureRandom for key generation
System.out.println("Secure random bytes and key generated.");
} catch (Exception e) {
e.printStackTrace();
}
}
}
Commentary: In this example, we replaced the default random number generation with SecureRandom
, which provides a stronger guarantee of randomness. This is critical for cryptographic security.
5. Failing to Validate Inputs Properly
The Trap
Input validation is crucial in cryptographic applications, especially since unvalidated inputs can lead to vulnerabilities like injection attacks.
The Solution
Always validate any external inputs used in cryptographic operations. Implement strict requirements regarding allowed formats, lengths, and character sets.
public class InputValidation {
public static boolean isValidInput(String input) {
return input != null && input.matches("^[a-zA-Z0-9]{8,}$");
}
public static void main(String[] args) {
String input = "ValidPass123";
if (isValidInput(input)) {
System.out.println("Valid input!");
} else {
System.out.println("Invalid input!");
}
}
}
Commentary: The isValidInput
method checks that the input adheres to a specific pattern. This validation helps minimize the risk of injection vulnerabilities.
The Closing Argument
Securing your Java application through cryptographic implementations is crucial in today’s threat landscape. By avoiding common pitfalls such as using weak algorithms, hard-coding keys, neglecting security management, relying on insecure random number generation, and failing to validate inputs, you strengthen your application's security posture.
For more in-depth reading, consider checking the following resources:
Remember, security is an ongoing process. Stay updated with new vulnerabilities, best practices, and changes in cryptography standards. Happy coding!