Mastering Java Garbage Collection Tuning for Optimal Performance
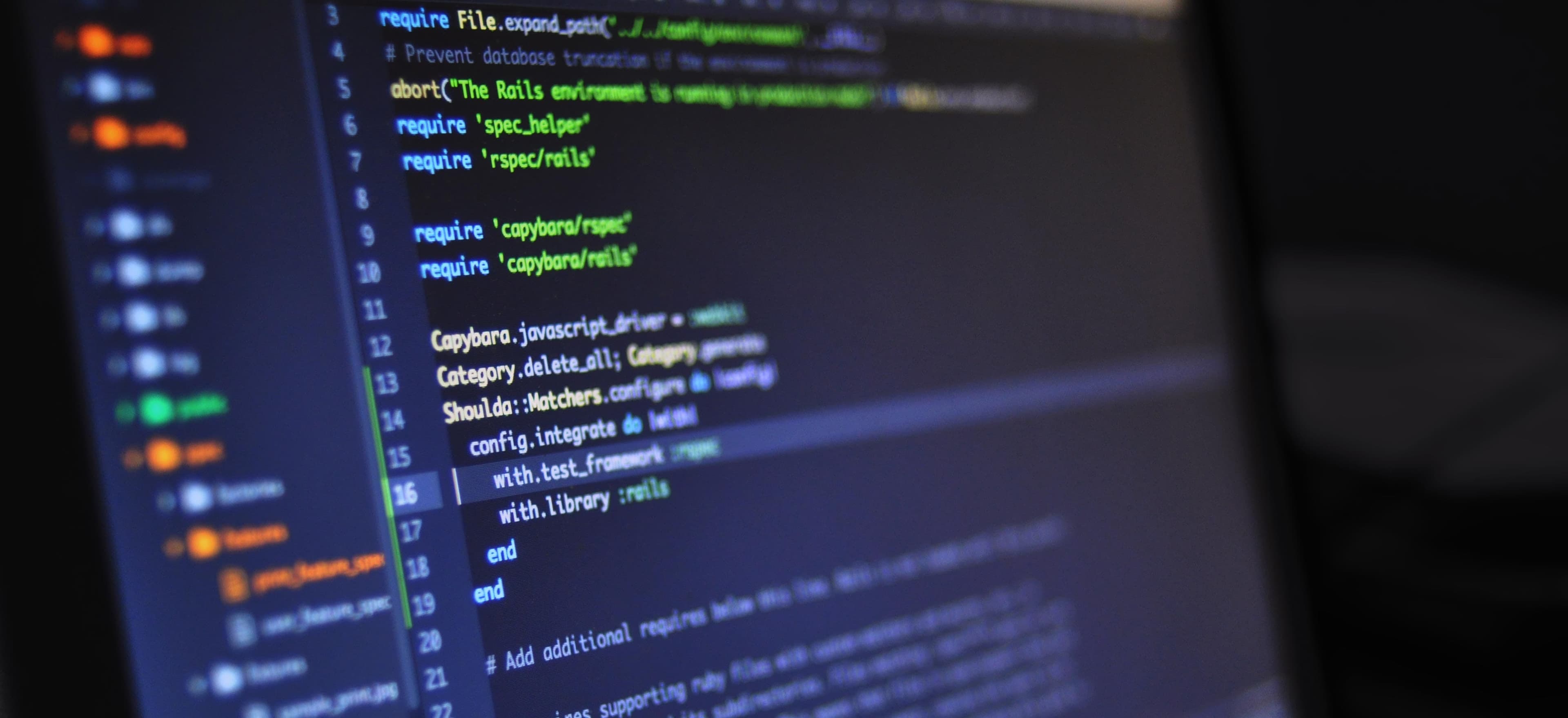
- Published on
Mastering Java Garbage Collection Tuning for Optimal Performance
In the realm of Java programming, one frequently overlooked aspect of performance optimization is Garbage Collection (GC). It often silently does its work in the background, yet its impact can make or break the efficiency of your applications. This post will unravel the intricacies of Java Garbage Collection and provide you with insights on how to tune it for optimal performance.
Understanding Java Garbage Collection
Java's automatic memory management ensures that developers can focus on building applications without worrying excessively about memory leaks and manual memory management. However, this convenience comes with a trade-off—garbage collection might introduce latency or pauses that can affect the user experience.
What is Garbage Collection?
Garbage Collection refers to the process of identifying and disposing of objects in memory that are no longer needed by the program. Java uses different garbage collectors, each employing unique strategies to manage memory. Understanding these strategies can empower you to choose the right one for your application.
The Garbage Collection Phases
Java garbage collection typically involves the following phases:
- Marking: The garbage collector scans through the heap to identify which objects are still being referenced.
- Normal Deletion: Unreferenced objects are flagged for deletion.
- Compaction: Memory is compacted to eliminate gaps caused by deleted objects, depending on the collector type.
Standard Java Garbage Collectors
Java offers several garbage collectors designed for various use cases:
- Serial Garbage Collector: Best suited for single-threaded applications.
- Parallel Garbage Collector: Optimized for throughput using multiple threads.
- Concurrent Mark Sweep (CMS) Collector: Aims for low pause times, making it suitable for applications requiring high responsiveness.
- G1 Garbage Collector: Designed for applications with large heaps that require predictable pause times.
Choosing the right garbage collector hinges on the application’s requirements. Each collector has a unique mechanism and trade-offs.
Tuning Garbage Collection
Understanding how to tune garbage collection can significantly improve application performance. Let's look at key parameters and settings that you can adjust.
1. Heap Size Configuration
The default heap settings may not be optimal for your application. You can configure the heap size using:
java -Xms512m -Xmx2048m -jar your_application.jar
- -Xms: Sets the initial heap size.
- -Xmx: Sets the maximum heap size.
Choosing the right size prevents frequent GC cycles and improves performance by allowing more objects to stay in memory.
2. Choosing the Right Collector
Depending on your use case, the garbage collector can be explicitly set as follows:
java -XX:+UseG1GC -jar your_application.jar
- -XX:+UseG1GC: Activates the G1 garbage collector which aims for both throughput and low pause times.
If your application is I/O intensive and requires maximum responsiveness, using CMS might be more appropriate:
java -XX:+UseConcMarkSweepGC -jar your_application.jar
3. Tuning GC Parameters
Various flags allow you to tweak the behavior of the garbage collectors. Here are some fundamental options:
- -XX:NewRatio=n: Sets the ratio between the young generation and the old generation. A higher ratio can reduce the frequency of full GCs but increase the time they take.
- -XX:SurvivorRatio=n: Adjusts the size of the Eden space and survivor spaces in the young generation.
Here’s how you might configure these parameters:
java -XX:NewRatio=3 -XX:SurvivorRatio=8 -jar your_application.jar
4. Monitoring Garbage Collection
Monitoring GC performance is crucial for making informed tuning decisions. Java provides various tools for monitoring, including:
- Java VisualVM: Offers a graphical interface to visualize heap usage and monitor GC activity.
- JConsole: A simple monitoring tool that connects to a Java application via JMX.
Using these tools, you can gain insight into GC behavior, identifying trends in pause times, frequency of collections, and memory usage.
Example Code with Garbage Collection Considerations
The choice of data structures can also influence garbage collection. For instance, using ArrayList
efficiently by preallocating its capacity can reduce the need for resizing, which in turn minimizes GC pressure.
import java.util.ArrayList;
public class EfficientList {
public static void main(String[] args) {
// Preallocate capacity to minimize resizing and reduce GC overhead
ArrayList<String> names = new ArrayList<>(1000);
for (int i = 0; i < 1000; i++) {
names.add("Name " + i);
}
// Use names for further processing
}
}
In this snippet, preallocating the capacity of ArrayList
prevents frequent resizing, hence reducing the number of temporary objects created, which leads to less frequent garbage collections.
Advanced Tuning Techniques
For high-performance applications, consider implementing some advanced techniques:
1. GC Logging
Enabling GC logging helps you gain insights into the garbage collection process:
java -Xlog:gc* -jar your_application.jar
Logging details provides the ability to analyze and visualize performance metrics, allowing for better-informed decisions during tuning.
2. Avoiding Full GCs
Ensure your application avoids frequent full GC pauses by maintaining a healthy working set of objects in memory. This can often involve analyzing your code for possible memory leaks and ensuring effective lifecycle management of objects.
3. Region-based Memory Allocation
For large datasets, consider segmenting objects into regions or using object pooling techniques, which minimizes the number of objects created and garbage collected.
The Last Word
Mastering Java Garbage Collection tuning is pivotal for optimizing application performance. With careful adjustments to heap sizes, collector preferences, and monitoring tools, you can significantly enhance the efficiency of your applications.
Garbage collection is a crucial aspect of Java performance, affecting not only speed but also resource usage and responsiveness. By applying these strategies, you empower yourself to develop high-performance Java applications that provide an excellent user experience.
For further reading, delve into resources like the Java Garbage Collection Tuning Guide and the Java Performance Tuning Book.
Happy coding!
Checkout our other articles