Common Pitfalls in Java API Testing with Rest-Assured
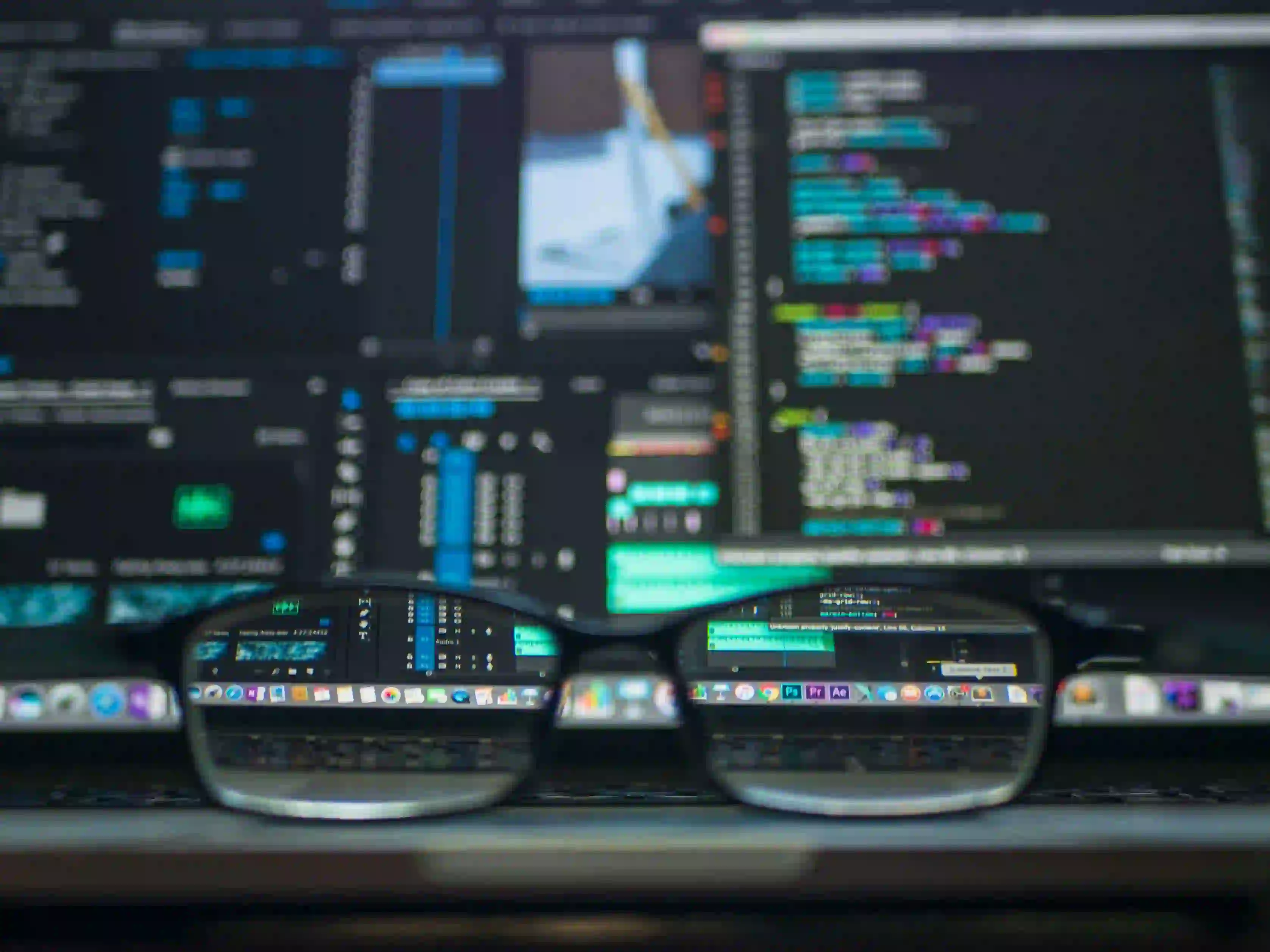
Common Pitfalls in Java API Testing with Rest-Assured
API testing is an essential practice in modern software development, helping to ensure the reliability and performance of services your applications depend on. Rest-Assured is a powerful Java library that simplifies testing REST APIs, making it a popular choice for developers. However, like any tool, it comes with its own set of challenges. In this blog post, we will explore some common pitfalls in Java API testing with Rest-Assured, along with best practices to avoid them.
Table of Contents
- Common Pitfalls
- Lack of Proper Setup
- Ignoring Response Validations
- Inadequate Error Handling
- Hardcoding URLs and Endpoints
- Not Using Environment Variables
- Overlooking JWT Tokens and Authentication
- Failing to Clean Up Data
- Best Practices
- Use Builders for HTTP Requests
- Parameterize Your Tests
- Grouping and Organizing Tests
- Utilize Assertions Effectively
- Conclusion
Common Pitfalls
1. Lack of Proper Setup
One of the most common pitfalls developers face is not properly setting up Rest-Assured before running tests. Without the correct base URI and port configurations, your tests may fail to connect to the right API endpoint.
import io.restassured.RestAssured;
public class ApiTest {
public void setup() {
RestAssured.baseURI = "http://localhost";
RestAssured.port = 8080; // The port the server is running on
}
}
Why? Proper setup ensures that all subsequent calls use the correct server context, which is critical for executing the tests smoothly.
2. Ignoring Response Validations
Validating the responses from your API calls is crucial. A common mistake is to neglect this step and instead assume a successful response equates to a working service.
import static io.restassured.RestAssured.*;
import static org.hamcrest.Matchers.*;
public void testGetUser() {
given()
.pathParam("userId", 1)
.when()
.get("/users/{userId}")
.then()
.statusCode(200)
.body("name", equalTo("Leanne Graham")); // Validate specific fields
}
Why? Checking not just the status code but also the content of the response gives you assurance about the integrity of the API.
3. Inadequate Error Handling
When dealing with APIs, it's not uncommon to encounter various types of errors. Ignoring how your API handles errors can lead to false positives in tests.
import static io.restassured.RestAssured.*;
public void testCreateUser() {
given()
.contentType("application/json")
.body("{ \"username\": \"newuser\" }")
.when()
.post("/users")
.then()
.statusCode(201)
.body("message", equalTo("User created successfully")); // Expecting a specific message
}
Why? A well-defined error response can guide API consumers and improve user experience. Test how your API reacts to malformed requests or missing fields.
4. Hardcoding URLs and Endpoints
Hardcoding URLs directly in your test cases can make the code less maintainable. If the API changes or is replaced by a different one, you have to edit multiple test cases.
public void testGetUser() {
String baseUri = System.getenv("API_BASE_URI"); // Use environment variable
given()
.baseUri(baseUri)
.pathParam("userId", 1)
.when()
.get("/users/{userId}")
.then()
.statusCode(200);
}
Why? Utilizing environment variables allows for easy changes across different environments (development, staging, production), leading to cleaner and more maintainable code.
5. Not Using Environment Variables
Another common oversight is not utilizing environment variables for configurations such as API keys, base URIs, and other sensitive data.
public void setup() {
RestAssured.baseURI = System.getenv("API_BASE_URI");
RestAssured.basePath = "/api/v1"; // Modifying the base path for versioning
}
Why? This practice enhances security and flexibility, as sensitive information can be hidden from your codebase.
6. Overlooking JWT Tokens and Authentication
Many APIs require authentication, particularly when modifying resources. Forgetting to include tokens or credentials can lead to unauthorized access errors, complicating testing.
public void testCreateUser() {
String token = getJwtToken(); // Assume this method retrieves a valid JWT
given()
.auth()
.oauth2(token)
.contentType("application/json")
.body("{ \"username\": \"newuser\" }")
.when()
.post("/users")
.then()
.statusCode(201);
}
Why? Including authentication ensures that your tests reflect real-world scenarios and interactions with the API.
7. Failing to Clean Up Data
Testing often involves creating resources. If those resources are not cleaned up post-tests, they can pollute subsequent test runs.
public void testCreateAndDeleteUser() {
int userId = given()
.contentType("application/json")
.body("{ \"username\": \"newuser\" }")
.when()
.post("/users")
.then()
.extract()
.path("id"); // Extract new user ID for cleanup
// Perform cleanup
given().pathParam("id", userId)
.when()
.delete("/users/{id}")
.then().statusCode(204);
}
Why? Ensuring a clean environment allows for accurate test results and prevents flaky tests.
Best Practices
Use Builders for HTTP Requests
Instead of using static string methods, consider using builders for your HTTP requests. This improves readability and maintainability.
import static io.restassured.RestAssured.*;
import static io.restassured.specification.FilterableRequestSpecification;
public void testGetUser() {
FilterableRequestSpecification requestSpec = new FilterableRequestSpecification();
requestSpec.baseUri("http://localhost:8080")
.header("Authorization", "Bearer " + getJwtToken())
.pathParam("userId", 1);
given()
.spec(requestSpec)
.when()
.get("/users/{userId}")
.then()
.statusCode(200);
}
Why? This method provides a central way to configure requests, making it easier to manage headers, parameters, and authentication.
Parameterize Your Tests
Utilizing parameterization allows you to run the same test with different inputs, expanding your test coverage with minimal code duplication.
@ParameterizedTest
@ValueSource(strings = { "user1", "user2", "user3" })
void testGetUser(String username) {
given()
.pathParam("username", username)
.when()
.get("/users/{username}")
.then()
.statusCode(200);
}
Why? Parameterization helps to verify multiple scenarios, enhancing your API testing without bloating your codebase.
Grouping and Organizing Tests
Consider structuring your tests into groups or suites based on functionality. This aids in better test management and execution times.
@RunWith(Suite.class)
@Suite.SuiteClasses({
ApiUserTests.class,
ApiAuthTests.class
})
public class ApiTestSuite {}
Why? Grouping tests allows for targeted execution and clear organization of different functionalities.
Utilize Assertions Effectively
Instead of checking a single condition, use multiple assertions to get maximum validation from your tests.
import static org.hamcrest.Matchers.*;
public void testGetUser() {
given()
.pathParam("userId", 1)
.when()
.get("/users/{userId}")
.then()
.statusCode(200)
.body("name", equalTo("Leanne Graham"))
.body("email", containsString("@")); // More robust validation
}
Why? Effective assertions ensure that your tests adequately verify the functionality of your API, leading to higher confidence in the system.
The Last Word
Java API testing with Rest-Assured provides developers with a powerful toolkit for verifying API functionality and performance. However, the common pitfalls highlighted in this post can easily derail your testing efforts. By adhering to best practices and avoiding these traps, you can create robust, reliable tests that contribute significantly to the quality of your applications.
To learn more about Rest-Assured and its capabilities, visit the official documentation. By continuously refining your testing strategies, you can ensure your applications not only meet specifications but also delight end-users with reliability and performance.