Common Pitfalls When Migrating from Java 8 to Java 11
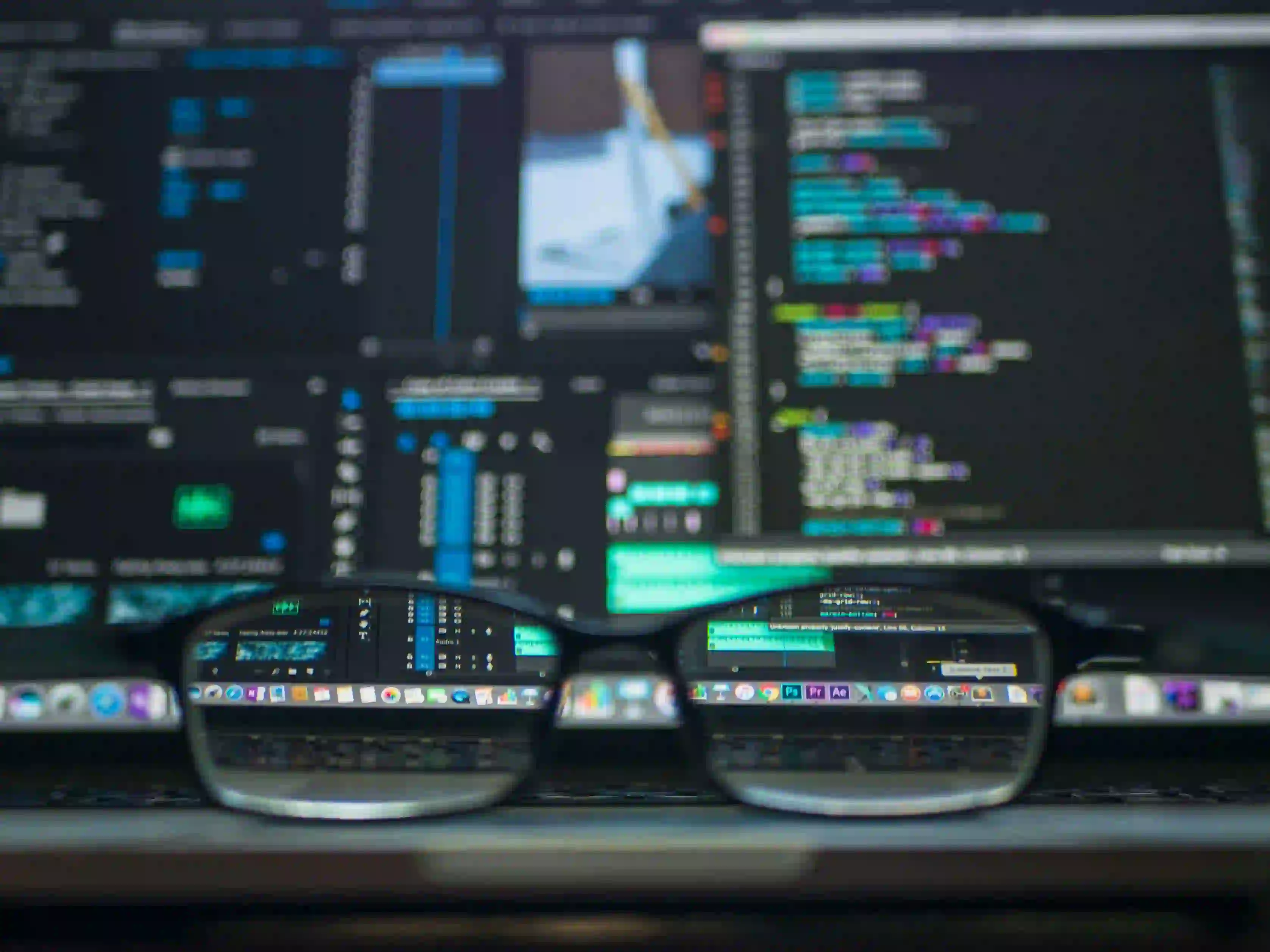
Common Pitfalls When Migrating from Java 8 to Java 11
The evolution from Java 8 to Java 11 introduces several new features and improvements, but it also presents unique challenges that developers must navigate. This blog post explores common pitfalls that can occur during this migration and offers insights into how to avoid them. With a focus on practical examples and clear explanations, you'll be guided through this transition process.
Table of Contents
- Understanding the Changes
- Pitfall 1: Removal of Deprecated APIs
- Pitfall 2: Module System
- Pitfall 3: Default Garbage Collector Changes
- Pitfall 4:
var
Keyword - Tips for a Smooth Migration
- Conclusion
Understanding the Changes
Java 11 is a Long-Term Support (LTS) release that builds on the features introduced in previous versions, notably Java 9 and Java 10. Understanding what has changed is critical.
- New Features: Includes the
var
keyword, enhancements in the HTTP client API, and more. - Removals: Certain APIs and options have been removed that were part of earlier Java versions.
- Module System: The introduction of the module system can impact the way dependencies are managed.
For a thorough understanding of all the changes, refer to Oracle’s Java SE 11 Documentation.
Pitfall 1: Removal of Deprecated APIs
Understanding the Issue
One of the biggest pitfalls while migrating is encountering deprecated APIs that have been removed in Java 11. For instance, the java.xml.ws package
has been removed, resulting in broken codebases that relied on the Java API for XML Web Services.
Example
import javax.xml.ws.*; // Deprecated in Java 11
public class MyWebService {
public void execute() {
// Logic here
}
}
Why This Matters
If your project uses these APIs, they will no longer compile in Java 11. To resolve this:
- Identify the APIs that your application uses and look them up in the documentation.
- Replace them with alternatives, such as using frameworks like Spring for web services.
Action Steps
- Use the
jdeps
tool to analyze your dependencies. - Update your codebase, making sure to replace deprecated methods or libraries with supported alternatives.
Pitfall 2: Module System
Understanding the Issue
Java introduced the module system in Java 9, and its effects become more apparent in Java 11. This can lead to conflicts in modular dependencies or access issues if your project does not account for module definitions.
Example
In a modular application, the incorrect definition of a module can lead to runtime issues.
module com.myapp {
requires java.base; // base module
exports com.myapp.service; // service package
}
Why This Matters
In the case where a required module is missing, you may encounter errors stating that a class cannot be found when running your application.
Action Steps
- Ensure all transitive dependencies are imported.
- Use the
--module-path
option when running modular code to specify module locations.
Pitfall 3: Default Garbage Collector Changes
Understanding the Issue
Starting from Java 9, the default Garbage Collector changed from the Parallel GC to the G1 GC. Migrating to Java 11 may exacerbate any performance issues if G1 is not tuned properly for your particular application.
Example
You may need to adjust JVM arguments pertaining to garbage collection.
java -XX:+UseG1GC -Xms512m -Xmx4g -jar myapp.jar
Why This Matters
Garbage collection is vital to application performance, especially for large-scale applications. If not handled correctly, you may experience unexpected memory issues.
Action Steps
- Run performance benchmarks before and after migration.
- Monitor application memory usage and tune GC settings as necessary.
Pitfall 4: var
Keyword
Understanding the Issue
Java 11 introduces the var
keyword, allowing for type inference in local variable declarations. While this offers cleaner syntactical patterns, it can also lead to reduced readability if overused.
Example
var list = new ArrayList<String>(); // Cleaner syntax
Why This Matters
Using var
in scenarios where type information is not clear could confuse developers reading the code later.
Action Steps
- Use
var
judiciously and prioritize clarity. - Consider team coding standards on when to use
var
.
Tips for a Smooth Migration
-
Testing: Establish comprehensive test suites and ensure they run correctly after your migration effort. Use testing frameworks such as JUnit 5 for better assertion capabilities.
-
Code Reviews: Foster a culture of peer reviews during the migration. Different perspectives can catch missed issues.
-
Incremental Migration: If possible, migrate a module or component at a time rather than the entire application. This approach makes debugging simpler.
-
Leverage Tools: Utilize tools like Maven or Gradle for managing dependencies and ensuring compatibility with Java 11.
-
Stay Updated: Follow the Java Community for changes and community discussions regarding Java.
To Wrap Things Up
Migrating from Java 8 to Java 11 presents its share of obstacles, but being aware of common pitfalls can significantly ease the transition. By taking proactive steps while acknowledging the modifications and enhancements, you can avoid common traps, make effective use of new features, and ultimately improve the performance and maintainability of your applications.
As the Java ecosystem continues to evolve, staying ahead with a commitment to learning will ensure you make the most of these advancements.
Happy coding!