Common Pitfalls in Java Application Internationalization
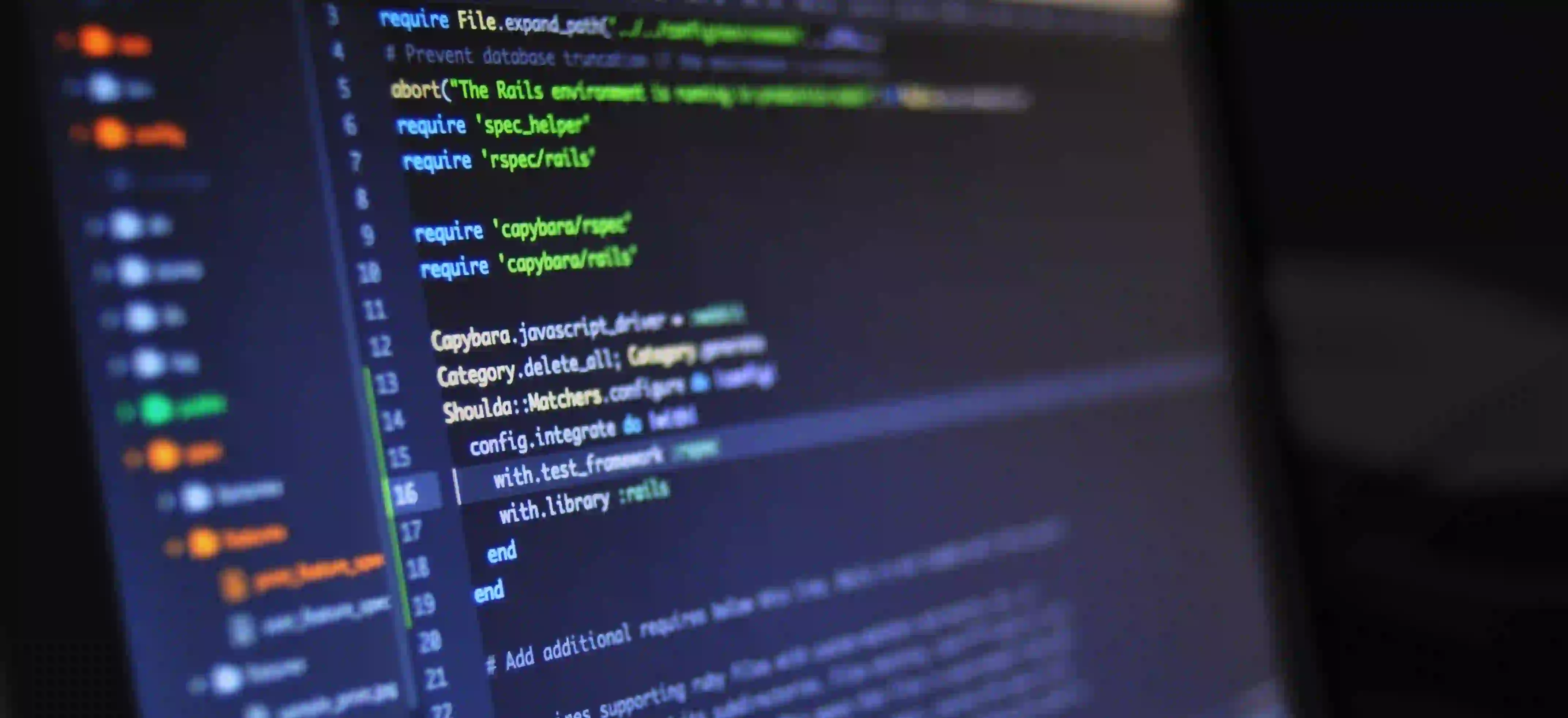
Common Pitfalls in Java Application Internationalization
Internationalization (i18n) is the process of designing your application in such a way that it can be adapted to various languages and regions without engineering changes. It is a crucial aspect of software development that often gets overlooked, especially when the application initially targets a single locale. In this blog post, we will discuss common pitfalls you may encounter when internationalizing Java applications and how to avoid them.
What is Internationalization?
Before diving into the pitfalls, let's clarify what internationalization means in the context of Java applications. It involves preparing your application to handle multiple languages, date formats, currencies, and other locale-specific elements. Java provides rich support for internationalization with its built-in libraries.
Why is Internationalization Important?
- User Satisfaction: Users prefer applications in their native language. If your application is easy to understand, users are likely to have a better experience.
- Market Expansion: Internationalized applications can be easily localized for different markets, enabling your business to reach a global audience.
- Compliance: Certain markets may have specific regulations regarding language use in software.
Common Pitfalls in Java Application Internationalization
1. Hardcoding Strings
The Issue
One of the most frequent mistakes developers make is hardcoding strings directly into the source code. For example:
public class Greeting {
public String getGreeting() {
return "Hello, World!";
}
}
The Fix
Instead, you should externalize strings in resource bundles. Java uses ResourceBundle
for this purpose. Here’s how you can declare a resource bundle.
Messages.properties (default)
greeting=Hello, World!
Messages_fr.properties (French)
greeting=Bonjour, le monde!
Then, you can access the localized strings like this:
import java.util.Locale;
import java.util.ResourceBundle;
public class Greeting {
public String getGreeting(Locale locale) {
ResourceBundle messages = ResourceBundle.getBundle("Messages", locale);
return messages.getString("greeting");
}
}
Why This Matters: By externalizing strings, you allow your application to easily switch languages without modifying the source code.
2. Ignoring Locale-Specific Formatting
The Issue
Formatting dates, numbers, and currencies incorrectly can lead to confusion. For instance, "01/04/2022" could mean January 4th in the U.S. but April 1st in many European countries.
The Fix
Use java.text.SimpleDateFormat
and java.text.NumberFormat
for locale-specific formatting:
import java.text.NumberFormat;
import java.util.Locale;
public class Formatter {
public String formatCurrency(double amount, Locale locale) {
NumberFormat currencyFormatter = NumberFormat.getCurrencyInstance(locale);
return currencyFormatter.format(amount);
}
}
Why This Matters: Accurate formatting enhances usability and avoids misinterpretation, fostering trust in your application.
3. Failing to Consider Pluralization
The Issue
Languages have different rules for pluralization. A message like "You have 1 new message" differs from "You have 2 new messages".
The Fix
Java's ResourceBundle
can manage pluralization efficiently. Define messages in Messages.properties
:
newMessages=You have {0} new message.
newMessagesPlural=You have {0} new messages.
Then, implement logic to handle plural cases:
import java.text.MessageFormat;
public class MessageDistributor {
public String getMessage(int count) {
ResourceBundle messages = ResourceBundle.getBundle("Messages");
String message = (count == 1) ?
messages.getString("newMessages") :
messages.getString("newMessagesPlural");
return MessageFormat.format(message, count);
}
}
Why This Matters: Properly handling pluralization ensures that your messages are grammatically correct and culturally appropriate.
4. Ignoring Right-to-Left (RTL) Languages
The Issue
Languages like Arabic and Hebrew are written from right to left. An application designed for left-to-right languages may break when adapted for RTL.
The Fix
Make your layout adaptable to different text directions using ComponentOrientation
. For example:
import javax.swing.*;
import java.awt.*;
public class RtlSupport {
public void setupRtlFrame() {
JFrame frame = new JFrame("Example");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setComponentOrientation(ComponentOrientation.RIGHT_TO_LEFT);
frame.add(new JLabel("مرحبا بالعالم")); // Hello World in Arabic
frame.setSize(300, 200);
frame.setVisible(true);
}
}
Why This Matters: Responsive design for RTL ensures a positive user experience for speakers of such languages.
5. Debugging Localization Issues
The Issue
Localization issues, such as missing translations or incorrect formats, can easily slip through the cracks, especially in larger applications.
The Fix
Implement rigorous testing strategies for your internationalized application. Use automated tests to verify that your application correctly loads resource bundles and formats localized content.
Here's an example of a simple test case to check resource loading:
import java.util.Locale;
import java.util.ResourceBundle;
public class ResourceBundleTest {
public void testFrenchMessages() {
ResourceBundle messages = ResourceBundle.getBundle("Messages", Locale.FRENCH);
assert "Bonjour, le monde!".equals(messages.getString("greeting"));
}
}
Why This Matters: Testing ensures that your internationalization efforts are effective and reliable.
6. Not Updating Translations
The Issue
As your application evolves, new features may be added, creating new strings that need translation. Failing to keep translations up-to-date can lead to a fragmented user experience.
The Fix
Create a systematic process for updating and managing translations. Consider using a tool or service that allows you to manage your translation files easily.
Why This Matters: Regular updates reflect in a quality user experience and prevent confusion due to outdated content.
Wrapping Up
In an increasingly globalized world, internationalizing your Java applications is not just an option—it’s a necessity. By avoiding common pitfalls like hardcoding strings, ignoring locale-specific formatting, and neglecting proper testing, you can develop applications that provide a high-quality experience for users across the globe.
Remember, the key to successful internationalization lies in early and inclusive planning. By implementing these best practices, you can ensure that your application is not only robust and efficient but also user-friendly in diverse cultural contexts.
For a deeper dive into Java internationalization features, check out the Java Internationalization Guide.
Feel free to extend your learning by exploring more about localization techniques and practices. Understanding and applying internationalization correctly will significantly broaden your application's reach and improve user satisfaction.
Happy coding!