Top Caching Pitfalls in Java Applications and How to Avoid Them
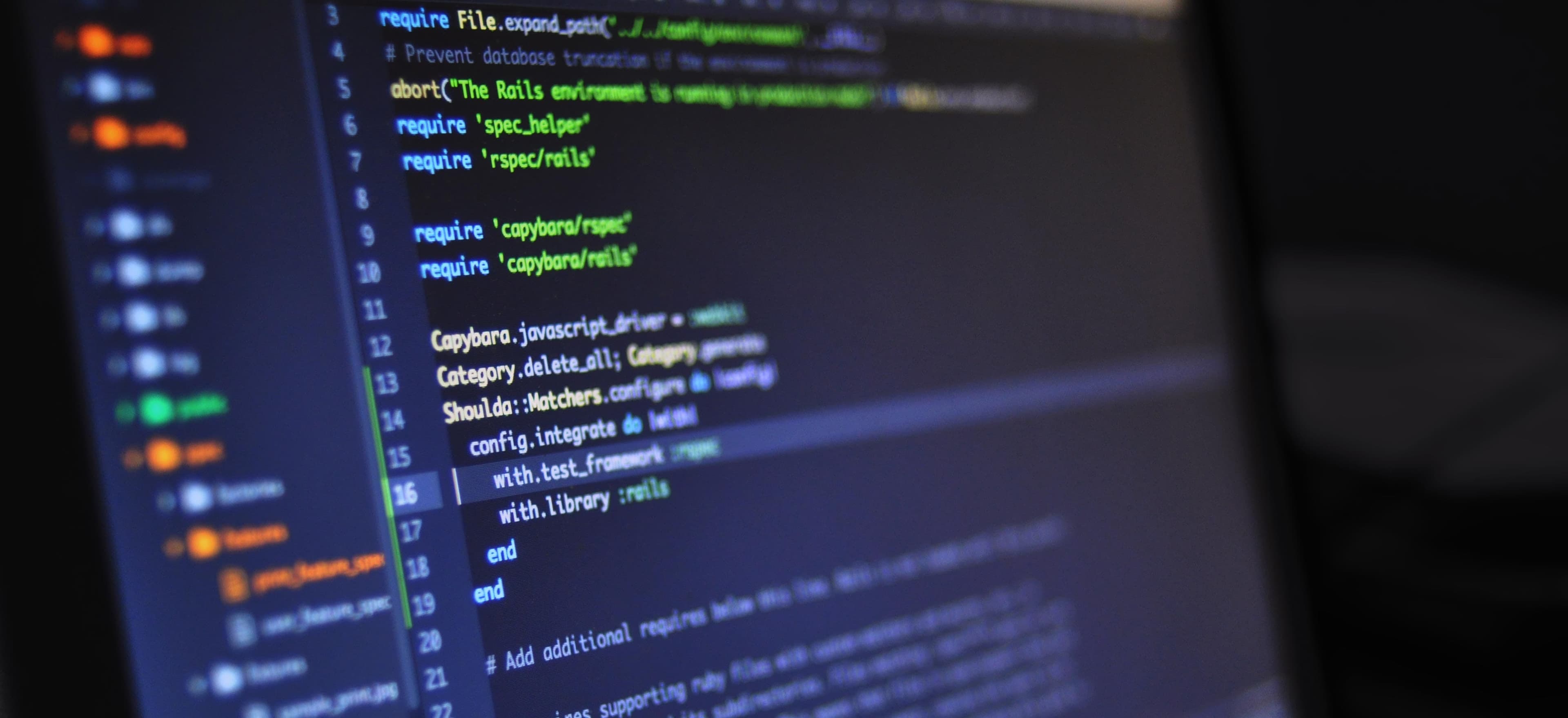
- Published on
Top Caching Pitfalls in Java Applications and How to Avoid Them
Caching is a crucial performance optimization technique in Java applications. It significantly improves application speed by storing data in a temporary storage area, making it faster to retrieve frequently accessed information. However, improper caching can lead to various issues that may degrade your application’s performance rather than enhance it. In this blog post, we will discuss the top caching pitfalls in Java applications and how to avoid them effectively.
1. Caching Too Much Data
The Issue
While it may seem beneficial to cache as much data as possible, doing so can lead to memory bloat. Caching too much can overwhelm your application's memory, causing it to run out and result in poor performance or even crashes.
The Solution
Instead, you should focus on caching only the data that is expensive to retrieve or calculate. Implement a caching strategy based on data access patterns. Here’s a small example using Guava Cache
:
import com.google.common.cache.CacheBuilder;
import com.google.common.cache.CacheLoader;
import com.google.common.cache.LoadingCache;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.TimeUnit;
public class CacheExample {
private LoadingCache<String, String> cache;
public CacheExample() {
cache = CacheBuilder.newBuilder()
.maximumSize(100) // Limit the cache size
.expireAfterWrite(10, TimeUnit.MINUTES) // Data expiration policy
.build(new CacheLoader<String, String>() {
public String load(String key) {
return fetchDataFromDatabase(key); // Fetch data if not in cache
}
});
}
public String getData(String key) throws ExecutionException {
return cache.get(key); // Retrieving from cache or loading
}
private String fetchDataFromDatabase(String key) {
// Simulated database access
return "Fetched data for key: " + key;
}
}
In this example, we set a maximum size for the cache and implemented a data expiration policy. This helps keep the cache in check and avoids memory overload.
2. Ignoring Cache Expiration
The Issue
Data can change, and when cached data remains too long without expiration, it may become stale. This can lead to inconsistencies and incorrect application behavior.
The Solution
Use an appropriate caching strategy that includes expiration. Consider using time-to-live (TTL) and write-through caching patterns. The above example with Guava Cache
also highlights cache expiration, which can be a straightforward way to avoid stale data.
3. Not Considering Thread Safety
The Issue
Java applications are often multithreaded, and caching implementations must be thread-safe. If multiple threads access and modify the cache simultaneously, it can lead to data corruption or inconsistent reads.
The Solution
Choose a caching library that inherently supports concurrency, such as Ehcache
or Caffeine
. Here's a concise example using Caffeine
:
import com.github.benmanes.caffeine.cache.Cache;
import com.github.benmanes.caffeine.cache.Caffeine;
import java.util.concurrent.TimeUnit;
public class CaffeineCacheExample {
private Cache<String, String> cache;
public CaffeineCacheExample() {
cache = Caffeine.newBuilder()
.maximumSize(100)
.expireAfterWrite(5, TimeUnit.MINUTES)
.build();
}
public String getData(String key) {
return cache.get(key, k -> fetchDataFromDatabase(k)); // Thread-safe retrieval
}
private String fetchDataFromDatabase(String key) {
// Simulating data fetch
return "Fetched data for key: " + key;
}
}
In this example, Caffeine
manages concurrency internally, making it an excellent choice for thread-safe caching.
4. Overcomplicating Cache Management
The Issue
Caching can become confusing with overly complex architectures. This complexity can lead to maintenance nightmares and performance overheads.
The Solution
Adopt simplicity in your caching strategy. Stick to a few caching libraries that suit your needs. It often pays to only adopt what you truly require. Identify the most used caching and implement a straightforward cache management layer.
For simple use cases, a local cache may suffice. However, for distributed caching, consider utilizing Memcached or Redis for ease of management.
5. Not Monitoring Cache Performance
The Issue
Without proper monitoring, cache performance can degrade, leading to application bottlenecks. You may end up blindly relying on your caching strategy without understanding its effectiveness.
The Solution
Implement monitoring tools and metrics to constantly evaluate your cache performance. Libraries like Micrometer
can help you track cache hits, misses, and other vital statistics.
Here’s a brief illustration:
import io.micrometer.core.instrument.MeterRegistry;
import io.micrometer.core.instrument.Tag;
public class CacheMetrics {
private final MeterRegistry meterRegistry;
public CacheMetrics(MeterRegistry meterRegistry) {
this.meterRegistry = meterRegistry;
}
public void recordCacheHit() {
meterRegistry.counter("cache.hits").increment();
}
public void recordCacheMiss() {
meterRegistry.counter("cache.misses").increment();
}
}
Integrate this CacheMetrics
class to keep track of your cache’s performance. Analyzing cache hit ratios, alongside application metrics, can inform you whether your cache strategy is working or needs refining.
6. Lack of Cache Invalidation Strategy
The Issue
Cache invalidation strategies dictate when cached data is refreshed or removed. Without an effective invalidation strategy, you may end up serving outdated data, which can have detrimental effects on user experience and data integrity.
The Solution
Choose a suitable invalidation strategy based on your application's requirements: either time-based or event-based invalidation.
For instance, you can implement an event-based strategy which listens to changes in your database and invalidates cached records accordingly:
public void updateDataInDatabase(String key, String newValue) {
updateDatabase(key, newValue);
cache.invalidate(key); // Invalidate related cache on update
}
7. Forgetting to Test Cache Behavior
The Issue
Testing cached logic can often be overlooked, leading to unexpected behaviors in production. It's important to test cache-focused logic thoroughly to identify any issues early.
The Solution
Utilize testing frameworks to emulate cache behavior with JUnit and Mockito, enabling you to test cache interactions effectively. Here is a simplified approach:
import org.junit.jupiter.api.Test;
import static org.mockito.Mockito.*;
import static org.junit.jupiter.api.Assertions.*;
public class CacheTest {
@Test
public void testCacheRetrieval() {
CacheExample cacheExample = new CacheExample();
String key = "testKey";
String expected = cacheExample.getData(key);
assertEquals("Fetched data for key: " + key, expected);
// Ensuring retrieval from cache
assertSame(expected, cacheExample.getData(key)); // Cache hit
}
}
By making cache behavior testable, you can ensure its reliability in both development and production environments.
The Last Word
Caching is a potent tool in Java applications that, when used correctly, can significantly enhance performance. However, it’s crucial to avoid common pitfalls such as over-caching, ignoring expiration, failing to ensure thread safety, and neglecting performance monitoring.
It's essential to continuously educate yourself on best practices and emerging trends. As technology evolves, stay adaptable. For further reading and effective caching solutions, consider checking Spring Caching and Java Caching Standards (JCache). Happy coding!
Checkout our other articles