Unlocking Sealed Classes: Why They're a Game Changer in Java 15
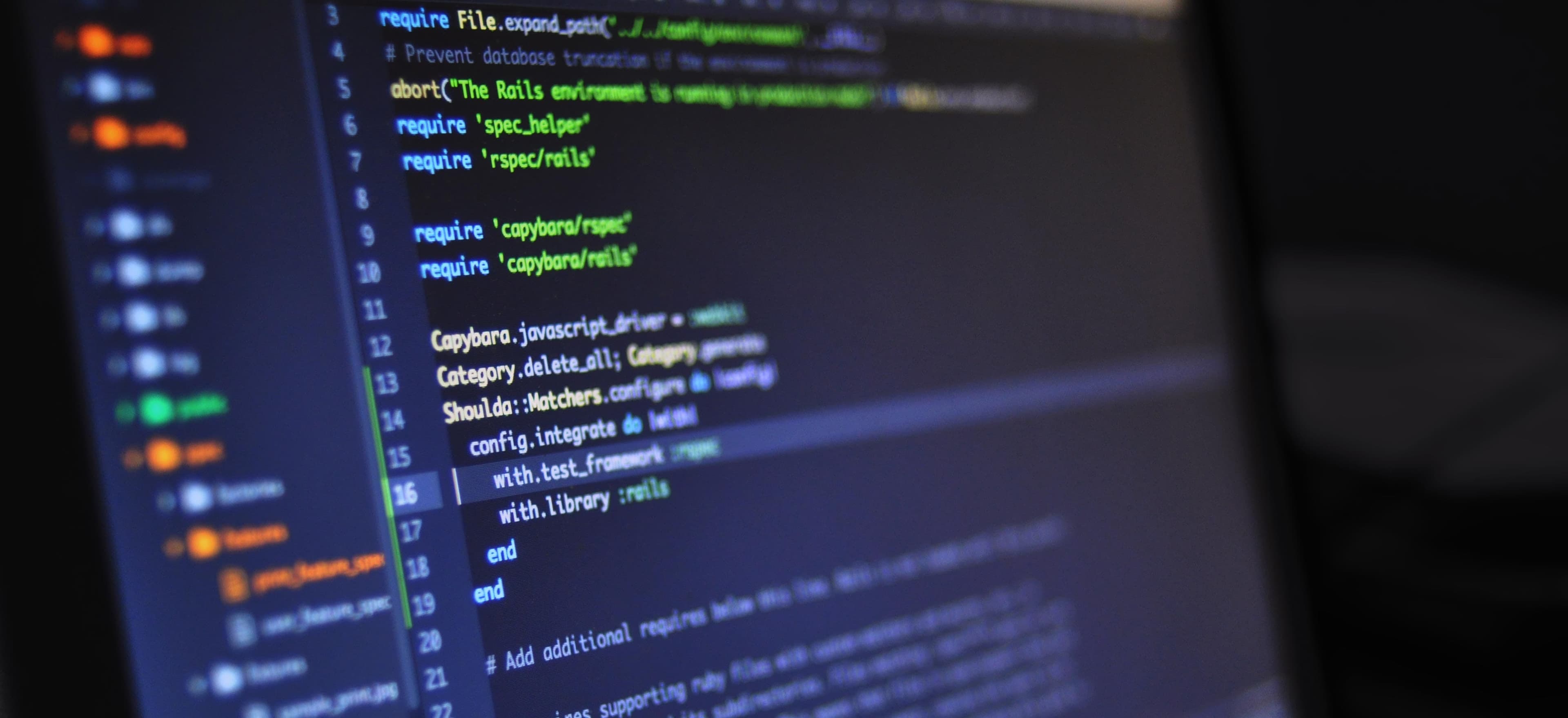
- Published on
Unlocking Sealed Classes: Why They're a Game Changer in Java 15
Java 15 marked a significant milestone in the Java ecosystem by introducing sealed classes. As developers, we strive for code that is not only functional but also secure, maintainable, and easy to understand. Sealed classes offer a powerful mechanism to enforce stricter controls over class hierarchies, promising to enhance readability and security. This blog post will delve into what sealed classes are, their benefits, and practical usage examples.
What are Sealed Classes?
Sealed classes, introduced in Java 15 as a preview feature, are a type of class that restricts which classes can extend or implement them. This feature is a welcome addition to Java's type system, offering an innovative way to handle inheritance hierarchies. Essentially, a sealed class allows you to define a controlled set of subclasses, thereby ensuring that the class model remains predictable and locked down.
Defining Sealed Classes
To declare a sealed class in Java, you use the sealed
modifier along with the permits
clause that defines the permitted subclasses. Here's a simple example:
public sealed class Shape permits Circle, Rectangle {
// Common properties and methods
}
In this example, Shape
is a sealed class and can only be extended by Circle
and Rectangle
. This explicit definition leads to a clear understanding of the class hierarchy at a glance.
The Benefits of Using Sealed Classes
Sealed classes present several advantages that can greatly improve your Java applications:
1. Improved Maintainability
By clearly defining which classes can extend your sealed classes, you reduce the risk of unexpected behavior due to rogue subclasses. A developer who reads your code will immediately know the allowable class hierarchy, making it easier to maintain and modify.
2. Enhanced Security
Sealed classes limit the exposure of your internal implementations. When you restrict subclassing, you minimize the attack surface, thus enhancing the security of your application.
3. More Intentional Design
Sealed classes encourage developers to make intentional design choices. Rather than allowing arbitrary subclassing, you can enforce a defined contract. This not only makes your codebase clearer but also fosters better design practices by promoting clear boundaries.
4. Simplified Pattern Matching
In conjunction with the enhancements of Java 16, such as pattern matching for instanceof
, sealed classes enable more straightforward and safer pattern matching in switch statements. This can lead to more concise and readable code.
Example: Sealed Classes in Action
Let's consider a practical example that illustrates the benefits of sealed classes in a voting system. We want to define different types of voter eligibility based on age and citizenship:
Step 1: Define the Sealed Class
We'll start by defining our sealed class VoterEligibility
:
public sealed class VoterEligibility permits Citizen, NonCitizen {
public abstract boolean canVote();
}
Step 2: Create Subclasses
Now, we will implement the subclasses based on the permitted types:
public final class Citizen extends VoterEligibility {
private final int age;
public Citizen(int age) {
this.age = age;
}
@Override
public boolean canVote() {
return age >= 18;
}
}
public final class NonCitizen extends VoterEligibility {
@Override
public boolean canVote() {
// Non-citizens cannot vote.
return false;
}
}
Step 3: Using the Sealed Class
Here's how you might use this sealed class in your application:
public class VotingSystem {
public static void checkVotingEligibility(VoterEligibility voter) {
if (voter.canVote()) {
System.out.println("Eligible to vote!");
} else {
System.out.println("Not eligible to vote.");
}
}
public static void main(String[] args) {
Citizen citizen = new Citizen(20);
NonCitizen nonCitizen = new NonCitizen();
checkVotingEligibility(citizen); // Outputs: Eligible to vote!
checkVotingEligibility(nonCitizen); // Outputs: Not eligible to vote.
}
}
Practical Considerations
While implementing sealed classes can seem daunting, here are some practical considerations:
-
Backward Compatibility: If you are maintaining an existing codebase, consider how sealing classes might affect existing interfaces and class hierarchies. Introduce sealed classes gradually.
-
Testing and Refactoring: As with any new feature, it’s essential to update your testing frameworks and documentation accordingly. Make sure your team is familiar with sealed classes to avoid confusion.
-
Maintain Clear Documentation: Always document the purpose of sealed classes in your code. It’s crucial for future maintainers to grasp why certain design choices have been made.
Final Thoughts
The introduction of sealed classes in Java 15 is a game changer that allows for a more controlled and manageable inheritance model. By restricting which classes can extend or implement your types, you pave the way for better design, greater security, and improved maintainability.
As Java evolves, features like sealed classes reflect the community's desire for clearer code organization and reduced complexity. To experience the full benefits of sealed classes, start implementing them in your projects and witness the transformation in your design practices.
For additional information on sealed classes, check out the official Java documentation here.
By understanding and utilizing sealed classes, you equip yourself with a modern tool that enhances how you model your Java applications. Sealed classes might just be the answer to your quest for cleaner, more maintainable code. Give them a try, and unlock the full potential of your Java projects!
Checkout our other articles