Common Pitfalls in Java Development with VS Code
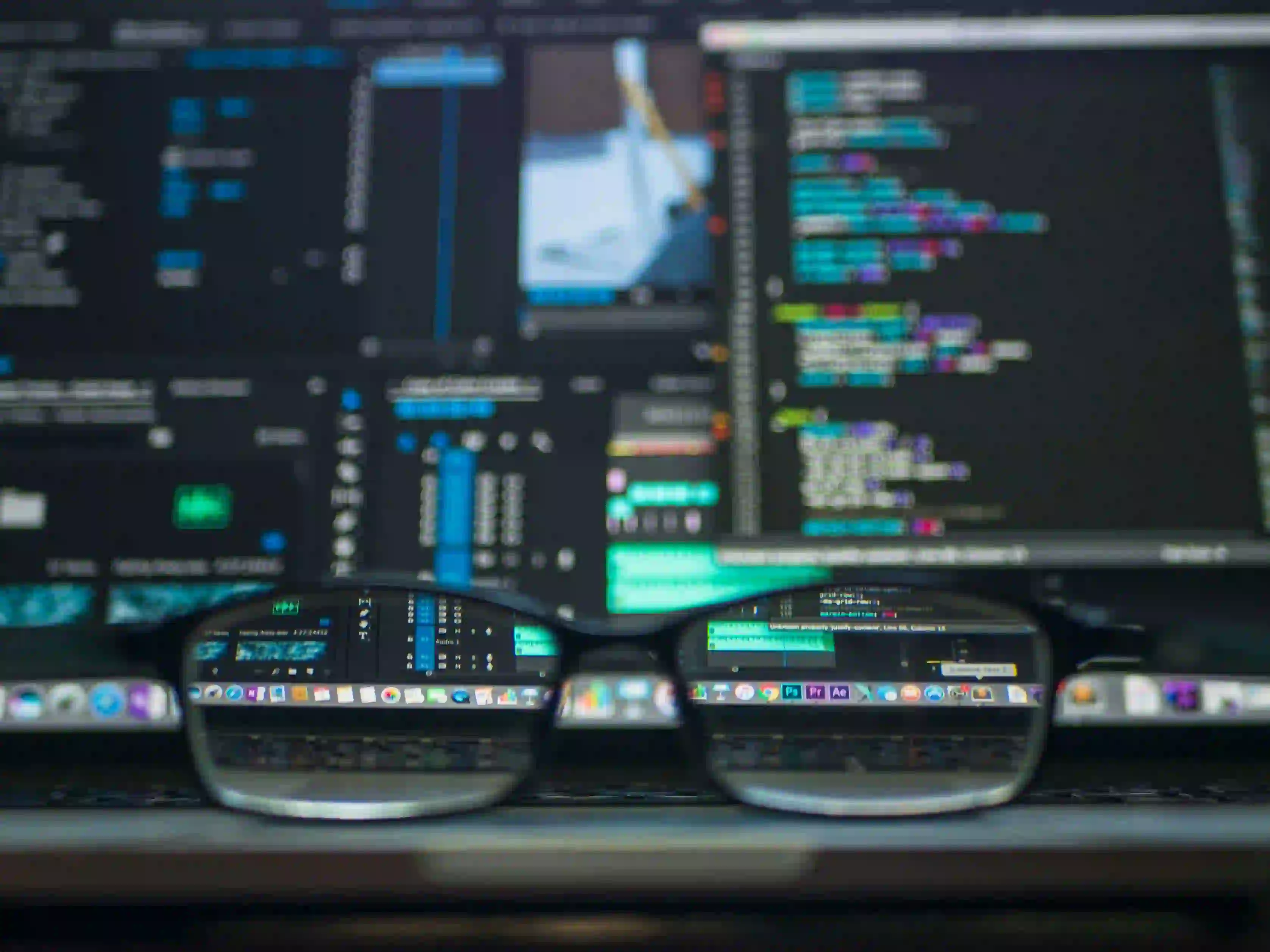
Common Pitfalls in Java Development with VS Code
Java is one of the most widely used programming languages, powering everything from web applications to Android apps. When paired with Visual Studio Code (VS Code), a powerful and versatile text editor, developers have a robust environment for Java development. However, despite its capabilities, there are common pitfalls that developers can encounter while using VS Code for Java. In this blog post, we will explore these pitfalls and how to avoid them, providing practical solutions and best practices along the way.
1. Incomplete Java Setup
One of the most common issues developers face when starting with Java in VS Code is an incomplete or incorrect setup of the Java Development Kit (JDK). If VS Code is unable to locate the JDK, you may encounter multiple errors when trying to compile or run your Java applications.
Solution
To ensure your Java environment is properly configured, follow these steps:
-
Download the JDK: Make sure to download the correct version of the JDK from the official Oracle website or consider using AdoptOpenJDK.
-
Set the JAVA_HOME Environment Variable: This allows the terminal to find the JDK when needed. Typically, you set this variable to the installation path of your JDK.
For Windows:
🔧snippet.shsetx JAVA_HOME "C:\Program Files\Java\jdk-11.0.10"
For macOS/Linux:
🔧snippet.shexport JAVA_HOME="/Library/Java/JavaVirtualMachines/jdk-11.0.10.jdk/Contents/Home"
-
Verify Installation: After installation, you can check whether Java is correctly set up by running:
🔧snippet.shjava -version
By ensuring that you've completed these steps, you can focus on writing code rather than troubleshooting environment variables.
2. Ignoring Extensions
VS Code offers various extensions that can significantly enhance your Java development experience. However, many developers overlook these tools. Ignoring the necessary extensions can lead to a lack of features like IntelliSense, debugging tools, or even error highlighting.
Solution
Make sure to install the following key extensions for Java development in VS Code:
- Java Extension Pack: This includes essential tools such as the Language Support for Java(TM) by Red Hat, Debugger for Java, Maven for Java, and others.
- Spring Boot Tools: For those working with the Spring framework, this extension simplifies the process of developing Spring applications.
To install these extensions, simply navigate to the Extensions view (Ctrl+Shift+X
), search for the extensions mentioned, and click on install.
3. Mismanaging Dependencies
Java projects often rely on external libraries that can be managed using build systems like Maven or Gradle. New developers to Java in VS Code might try to manually download JAR files and troubleshoot conflicts without realizing that this can lead to significant management issues down the line.
Solution
Here's how to efficiently manage dependencies:
-
Use Maven: Create a
pom.xml
file to manage your project. Here’s an example of a simplepom.xml
:📄snippet.txt<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.example</groupId> <artifactId>my-app</artifactId> <version>1.0-SNAPSHOT</version> <dependencies> <dependency> <groupId>org.apache.commons</groupId> <artifactId>commons-lang3</artifactId> <version>3.12.0</version> </dependency> </dependencies> </project>
In this example, we included the Apache Commons Lang library, which simplifies string manipulations and other tasks.
-
Use Gradle: Alternatively, you could use a
build.gradle
file:📄snippet.txtplugins { id 'java' } group 'com.example' version '1.0-SNAPSHOT' repositories { mavenCentral() } dependencies { implementation 'org.apache.commons:commons-lang3:3.12.0' }
Using a build tool not only simplifies dependency management but also ensures consistency across different development environments.
4. Neglecting Code Quality Tools
Code quality is essential for maintainability, especially in larger projects. On VS Code, many developers neglect tools that could assist in maintaining code quality, such as linters, formatters, or testing frameworks.
Solution
Make use of tools such as:
-
Checkstyle: This tool helps ensure that your code adheres to a coding standard. You can configure it by creating a configuration file (
checkstyle.xml
) and integrating it into your project. -
SonarLint: This VS Code extension can identify and fix code issues in real-time by providing feedback as you code.
Integrating these tools can create a significant impact on your code quality. Here’s a simple configuration snippet for Checkstyle:
<module name="Checker">
<module name="TreeWalker">
<module name="LineLength">
<property name="max" value="80"/>
</module>
<module name="JavadocType"/>
</module>
</module>
5. Overlooking Version Control Integration
The lack of proper version control can lead to many headaches, particularly for team projects. Many developers while using VS Code take version control for granted, which can result in lost or conflicted code, especially during collaborative projects.
Solution
Make use of the built-in Git features in VS Code.
-
Initialize Git: Start by initializing a Git repository in your project folder:
🔧snippet.shgit init
-
Track changes: Use the Source Control panel in VS Code to keep track of your changes, stage files, and make commits.
-
Branching and Merging: Regularly use branches to manage new features or fixes. This allows you to work in isolation without affecting the main codebase.
By actively managing your code with version control, you can mitigate the risks that come with collaborative development.
6. Ignoring Debugging Features
VS Code is rich with debugging features that are often underutilized by developers. Debuggers allow developers to step through code, inspect variables, and evaluate expressions, making identifying issues much faster.
Solution
-
Set breakpoints: Click on the left margin of the editor to set a breakpoint on the line you’re interested in.
-
Launch the debugger: Press
F5
to start debugging. You’ll be able to step in and out of methods, and examine variables in the "Variables" section.
Here’s an example of debugging code:
public class Main {
public static void main(String[] args) {
int x = 1;
int y = 3;
int sum = add(x, y);
System.out.println("Sum: " + sum);
}
public static int add(int a, int b) {
return a + b;
}
}
With a breakpoint set at the sum
calculation, you can inspect the values of x
and y
during execution.
The Bottom Line
Java development with VS Code can be both efficient and enjoyable, provided you take care to avoid common pitfalls. Properly setting up your JDK, effectively managing dependencies, utilizing extensions, and making the most of debugging features can enhance your experience.
For further reading on these topics, consider checking out the official Java Development documentation or the VS Code Java documentation.
By addressing these issues early and developing good habits, you will significantly improve your productivity and the quality of your Java applications. Enjoy coding in Java with VS Code, and happy developing!