Bridging the Gap: Java and Kotlin Interoperability Issues
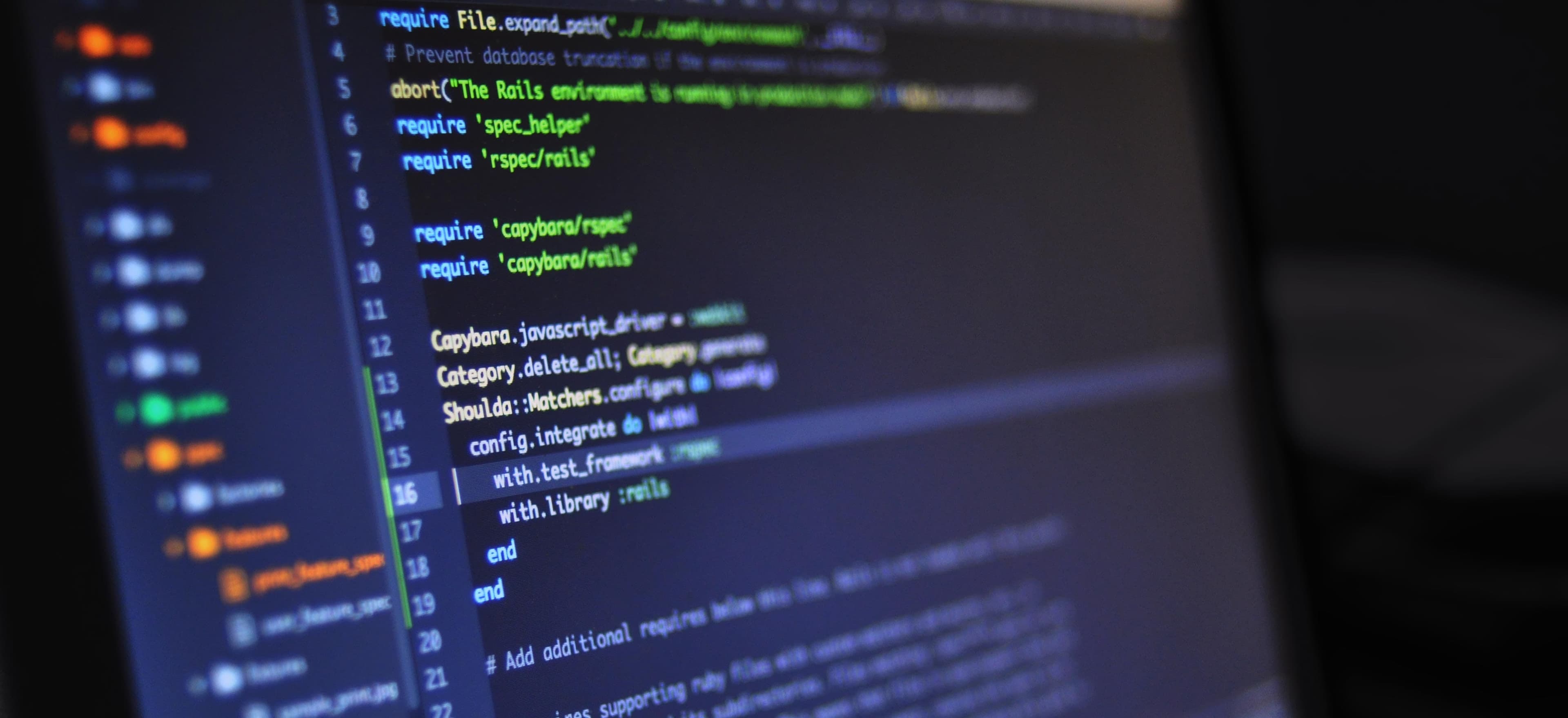
- Published on
Bridging the Gap: Java and Kotlin Interoperability Issues
In the modern landscape of Android app development, the choice of programming languages plays a critical role in the efficiency and maintainability of codebases. Java has long been the staple language for Android developers, but Kotlin has emerged as a strong competitor, offering more concise syntax and modern programming features. As Kotlin was designed to interoperate seamlessly with Java, it naturally raises questions about the potential issues that may arise during the integration of these two languages. In this blog post, we will explore these interoperability challenges, provide code snippets, and discuss solutions to bridge the gap between Java and Kotlin.
Understanding the Interoperability
Interoperability refers to the ability of two different programming languages to work together within the same context. When Kotlin was introduced, it was designed to be fully interoperable with Java, which means that you can call Kotlin code from Java and vice versa. However, this interoperability is not without its issues.
The primary goal of interoperability between Java and Kotlin is to enhance productivity without sacrificing performance. While this sounds promising, Java's and Kotlin's differing paradigms — such as null safety and extension functions — can lead to confusion and bugs if not handled carefully.
Common Interoperability Issues
1. Null Safety
Kotlin has built-in null safety, preventing null pointer exceptions, which are one of the most common runtime errors in Java. However, since Java allows null references, this can cause issues when calling Java code from Kotlin.
Example Scenario
Let’s consider a simple Java class:
public class User {
private String name;
public User(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
In the code above, the name
property can be null. If we attempt to call this code from Kotlin, the compiler will raise warnings.
Kotlin Usage
fun main() {
val user = User(null)
val name = user.name // Warning: Requires '!!' or '?.'
println(name?.length) // Safe call operator
}
In the Kotlin code snippet above, the safe call operator (?.
) ensures that we do not attempt to access properties or methods on a null reference. This is critical in avoiding potential runtime crashes.
2. Extension Functions
Kotlin's extension functions allow developers to add new functionality to existing classes without modifying their source code. However, this feature does not translate to Java.
Example Scenario
fun String.addExclamation(): String {
return this + "!"
}
You can call this function from Kotlin easily:
fun main() {
val greeting = "Hello"
println(greeting.addExclamation()) // Output: Hello!
}
However, trying to access the addExclamation
function in Java will result in a compilation error because Java does not recognize Kotlin's extension functions as part of the class's API.
3. Varargs and Default Parameters
Kotlin allows varargs and default parameters, allowing for cleaner function calls. Java, on the other hand, does not have these capabilities. This may result in some awkward translations.
Example Scenario
Kotlin Function with Default Parameters:
fun greet(name: String = "World") {
println("Hello, $name!")
}
When this Kotlin function is called from Java, the default parameters will need to be manually specified unless you overload the function.
Bridging the Gap: Solutions
Leveraging Annotations
To address the null safety issue, you can use annotations. In your Kotlin code, you can denote types as nullable or non-nullable using annotations like @Nullable
and @NonNull
. Here is how you can implement that:
Example
// Kotlin function
fun greetUser(@Nullable name: String) {
if (name == null) {
println("Hello, Guest!")
} else {
println("Hello, $name!")
}
}
Calling this from Java will require checking for null, but it clarifies intention and usage — a significant step toward solving interoperability problems.
Using Kotlin's @JvmName and @JvmField
Kotlin provides annotations like @JvmName
and @JvmField
which can assist in interoperability by explicitly naming generated methods and ensuring that properties are accessible in Java.
Example of @JvmField
class User(@JvmField var name: String)
In this instance, the name
property can be accessed directly in Java without the usual getters and setters.
Adopting Best Practices
As with any technology, adhering to best practices in both Kotlin and Java can significantly minimize interoperability issues. Here are a few tips:
-
Document Your API: Clear documentation helps prevent misunderstandings about how to work with the code.
-
Type Safety: Be consistent in how you represent nullability in both languages to prevent accidental nulls from slipping through.
-
Use Wrapper Classes: When dealing with varargs or default parameters, consider wrapping them in a class to enhance readability and manageability.
References for Further Reading
To Wrap Things Up
The interoperability between Java and Kotlin opens up exciting possibilities for developers, allowing them to leverage the strengths of both languages. However, it’s essential to be aware of and address tenacious issues that arise during integration. By understanding null safety, extension functions, and default parameters, and by following best practices, developers can minimize these issues and create robust applications.
The gap between Java and Kotlin is not insurmountable; it simply requires a keen understanding and thoughtful approach. Whether you are migrating from Java to Kotlin or integrating both languages within the same project, approaching with awareness and preparedness can lead to success in any development endeavor.
By understanding these interoperability issues, developers can confidently bridge the gap between Java and Kotlin, ultimately leading to better applications and an enhanced programming experience.
Checkout our other articles