Common Pitfalls in Java for Raspberry Pi IoT Projects
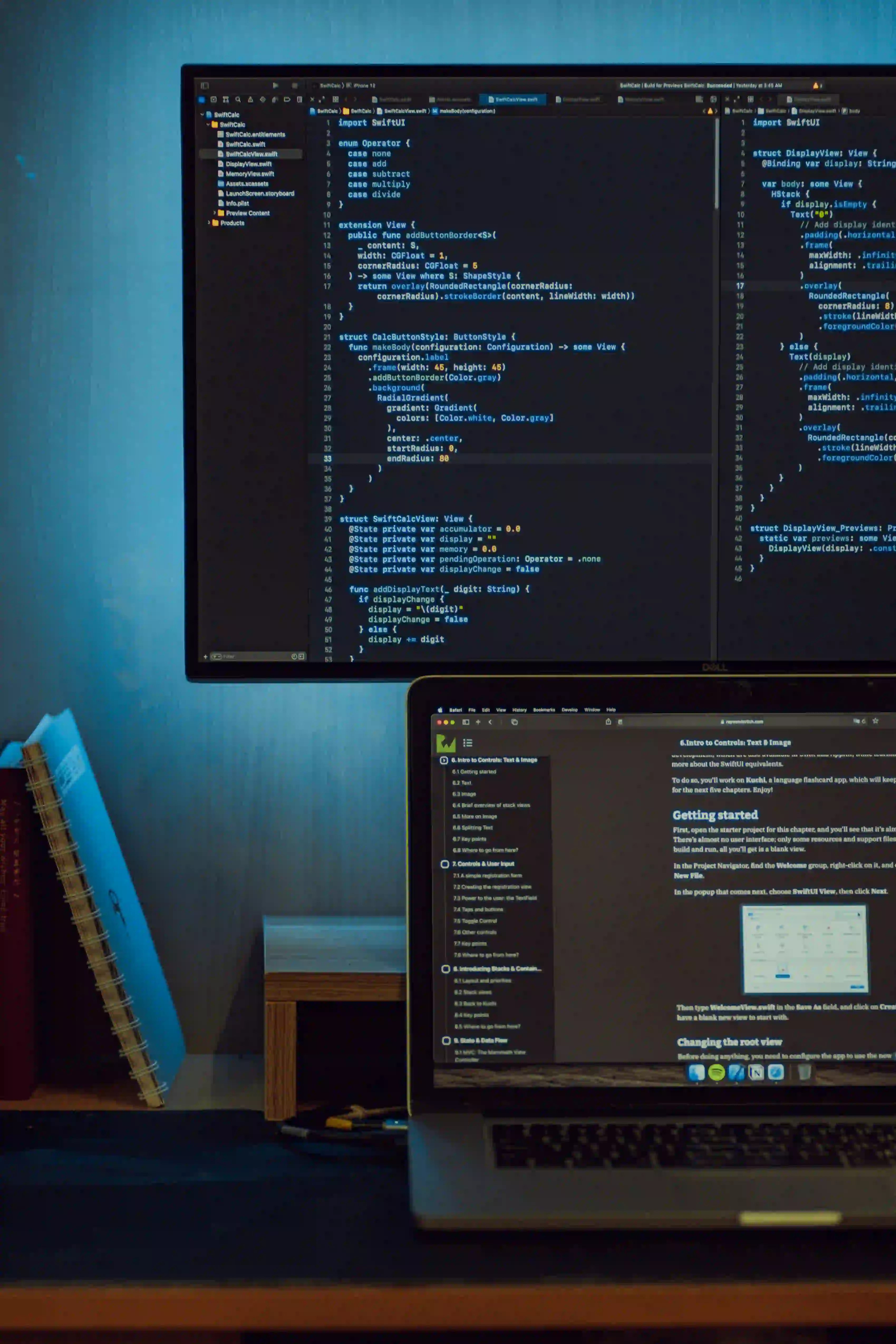
Common Pitfalls in Java for Raspberry Pi IoT Projects
The advent of the Internet of Things (IoT) has turned Raspberry Pi devices into a popular platform for developing innovative applications. Using Java for these projects can bring numerous benefits due to its versatility and extensive libraries. However, like any development environment, there are common pitfalls that developers might encounter. This blog post aims to identify these issues and provide practical solutions.
Why Choose Java for IoT Projects on Raspberry Pi?
Java offers a robust, platform-independent solution that is well-suited for IoT applications. Its extensive libraries, such as the Java Communication API (javax.comm) and libraries for networking and threading, make it an excellent choice for building scalable IoT applications.
Before diving into the common pitfalls, let's explore a few key features of Java that make it well-suited for Raspberry Pi:
- Portability: Write once, run anywhere. Java applications can be executed on any device with a Java Virtual Machine (JVM).
- Rich Ecosystem: Java has a massive library ecosystem that provides pre-built functionalities for various tasks.
- Large Community Support: A vibrant community means more resources, tutorials, and libraries available to help solve problems.
Common Pitfalls in Java for Raspberry Pi IoT Projects
1. Memory Management Issues
Problem
Raspberry Pi devices often have limited RAM (most models range from 256MB to 4GB), which can lead to "OutOfMemoryError" if applications are not properly managed.
Solution
Optimize your code to minimize memory consumption. Use memory profiling tools to identify memory leaks and refactor your code accordingly.
Example:
// Inefficient use of memory
List<String> names = new ArrayList<>();
for (int i = 0; i < 1000000; i++) {
names.add("Name " + i);
}
// Optimize by using a fixed-size array or StringBuilder
StringBuilder names = new StringBuilder();
for (int i = 0; i < 1000000; i++) {
names.append("Name ").append(i).append("\n");
}
In the optimized example, we choose StringBuilder
for efficient string concatenation, thereby reducing memory footprint.
2. Poor Thread Management
Problem
Threading can be tricky in any language, and Java is no exception. Improper handling of threads can lead to race conditions and deadlocks, particularly when reading sensor data.
Solution
Use Java's built-in concurrency utilities, such as ExecutorService
, to manage threads effectively.
Example:
ExecutorService executorService = Executors.newFixedThreadPool(4);
for (int i = 0; i < 10; i++) {
executorService.submit(() -> {
// Simulate sensor data collection
System.out.println("Collecting Data: " + Thread.currentThread().getName());
});
}
executorService.shutdown();
In this example, we utilize an ExecutorService
for handling thread management more efficiently, avoiding the overhead of manually managing threads.
3. Networking Issues
Problem
Network connectivity can often be flaky, especially in IoT environments. Poor management of connections can make your application less responsive.
Solution
Implement retries with exponential backoff and consider using non-blocking I/O to enhance responsiveness.
Example:
import java.net.HttpURLConnection;
import java.net.URL;
public void sendData(String data) {
int retries = 0;
while (retries < 5) {
try {
URL url = new URL("http://yourserver.com/data");
HttpURLConnection connection = (HttpURLConnection) url.openConnection();
connection.setRequestMethod("POST");
connection.setDoOutput(true);
connection.getOutputStream().write(data.getBytes());
connection.getResponseCode();
break; // successful send
} catch (Exception e) {
retries++;
try {
Thread.sleep((long) Math.pow(2, retries) * 1000); // Exponential backoff
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
}
}
}
}
In this snippet, we demonstrate retry logic with exponential backoff, which allows the application to handle temporary network issues more gracefully.
4. Platform-Specific Library Usage
Problem
Relying too heavily on platform-specific libraries can make your codebase less portable and cause issues when running on different Raspberry Pi models.
Solution
Always prefer libraries that are explicitly designed for cross-platform compatibility. When possible, use standard Java libraries or abstractions.
For example, rather than using a specific GPIO library, check if a more abstract library like Pi4J can suffice. Pi4J is a Java library that provides a simple way to use the GPIO pins and other hardware features on Raspberry Pi with a clean, consistent API.
5. Lack of Exception Handling
Problem
Not managing exceptions can lead to applications crashing unexpectedly, especially when dealing with network calls or hardware I/O.
Solution
Always implement a comprehensive exception handling strategy around code that interacts with hardware and networks.
Example:
try {
// Code that may throw an exception
readSensorData();
} catch (IOException e) {
System.err.println("Error reading sensor data: " + e.getMessage());
// Additional handling logic here
}
By proactively catching and handling exceptions, the application can log errors and proceed without abrupt termination.
6. Neglecting Software Updates
Problem
IoT applications often need to communicate with external services and APIs that may change over time. Failing to update your code could lead to compatibility issues and security vulnerabilities.
Solution
Implement a regular maintenance schedule to update dependencies and libraries. Use tools like Maven or Gradle for dependency management.
Regularly check for Java updates and patches for libraries you are using.
7. Overlooking Security Practices
Problem
Security should be a top priority in IoT projects, as unsecured devices can become targets for malicious attacks.
Solution
Implement best practices like using HTTPS for data transmission and storing sensitive data securely.
Example:
import javax.crypto.Cipher;
import javax.crypto.spec.SecretKeySpec;
public String encrypt(String data, String key) throws Exception {
SecretKeySpec secretKey = new SecretKeySpec(key.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
return new String(cipher.doFinal(data.getBytes()));
}
Here, we use AES encryption to protect sensitive data, demonstrating an approach to secure communication in IoT applications.
Closing the Chapter
Building IoT applications using Java on Raspberry Pi can be exciting and rewarding. However, being aware of common pitfalls is crucial for developing robust, secure, and efficient applications. By implementing optimized memory management, thoughtful thread handling, sound networking practices, portable libraries, comprehensive exception handling, routine updates, and proper security measures, you can create powerful IoT solutions.
To get started with your Raspberry Pi IoT project using Java, you may find the following resources helpful:
- Java for IoT: Official Oracle Java IoT page.
- Pi4J Library Documentation: A great resource for working with Raspberry Pi hardware.
- Java Concurrency in Practice: Essential reading for understanding threading and concurrency in Java.
Use this guide as a baseline to ensure the success of your Raspberry Pi Java IoT projects. Happy coding!