Overcoming Java's Performance Hurdles in Embedded Systems
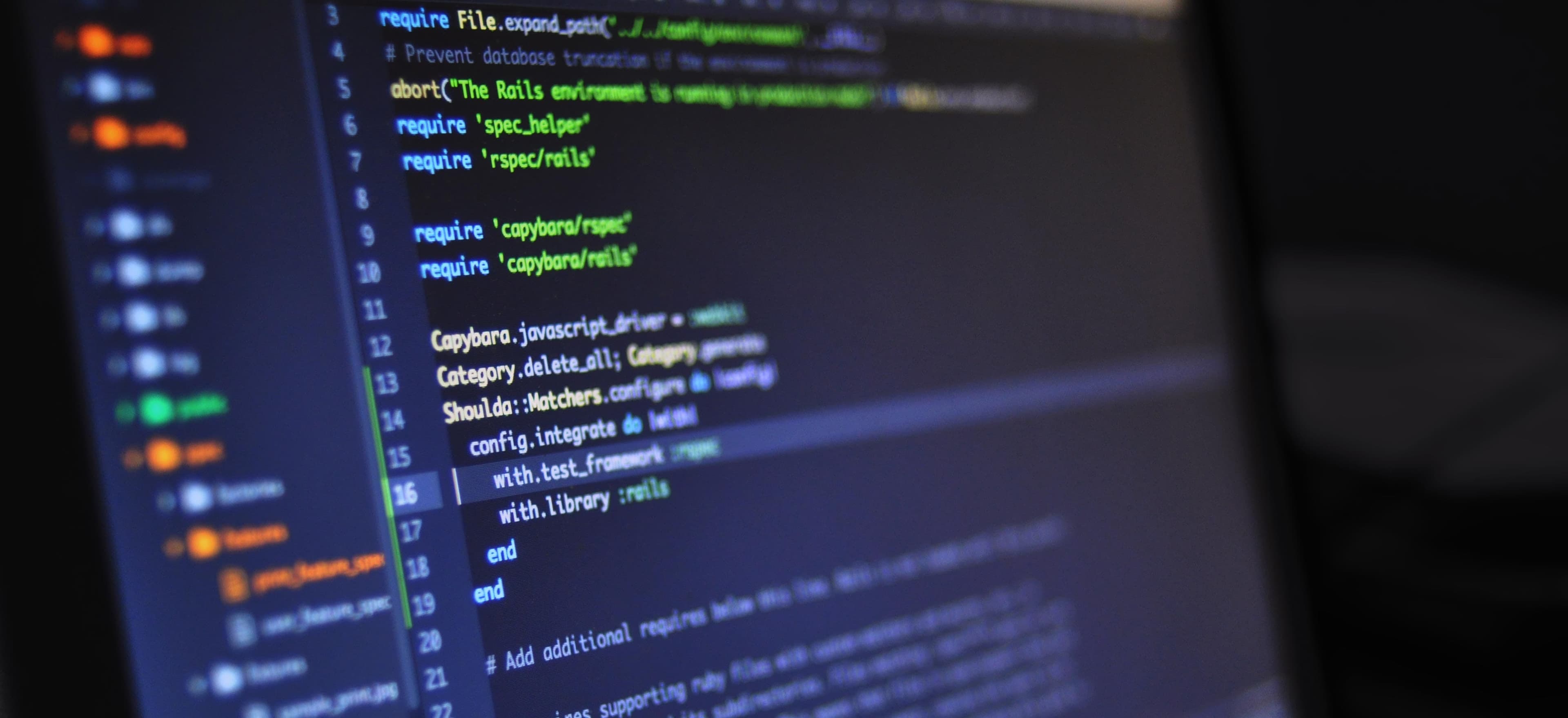
- Published on
Overcoming Java's Performance Hurdles in Embedded Systems
In the evolving landscape of technology, embedded systems have become ubiquitous, integrating with everything from household appliances to industrial machinery. While C and C++ have long dominated this domain due to their performance and efficiency, Java is steadily gaining traction. Its rich ecosystem and cross-platform capabilities make it an appealing option. However, Java’s performance in embedded systems raises some concerns. This blog will explore these concerns and discuss strategies for overcoming potential performance hurdles.
Understanding Embedded Systems
Embedded systems are specialized computing systems that perform dedicated functions within larger systems. They are characterized by:
- Resource Constraints: Limited memory and processing power.
- Real-Time Requirements: Many embedded systems require timely and deterministic responses.
- Power Efficiency: Especially important for battery-operated devices.
Java, despite its strengths, is often viewed as heavyweight for such environments. However, its potential can be harnessed through careful optimization.
Common Performance Issues with Java in Embedded Systems
Before we can mitigate these hurdles, we must first identify them:
- Garbage Collection: Java’s automatic memory management can introduce latency due to sporadic garbage collection cycles.
- JVM Overhead: The Java Virtual Machine (JVM) itself can consume significant resources and take time to start up.
- Interpretation and Compilation: The Just-In-Time (JIT) compilation process introduces delays, especially in small, low-latency embedded systems.
- Libraries and Frameworks: Many Java libraries were originally designed for desktop applications, leading to overhead that is not suitable for embedded systems.
Strategies for Overcoming Performance Hurdles
1. Choosing the Right JVM
The first step in improving Java application performance in embedded systems is choosing an appropriate JVM. There are several lightweight JVM implementations optimized for embedded devices, including:
- OpenJ9: A highly optimized JVM that provides fast launch and low memory footprint.
- GraalVM: Offers ahead-of-time (AOT) compilation capabilities, which can lead to faster startup times and reduced runtime overhead.
Selecting a JVM tailored for embedded systems can significantly reduce performance bottlenecks.
2. Managing Memory Efficiently
Java's garbage collector can introduce latency that is unacceptable in some embedded environments. To mitigate this effect, consider the following strategies:
- Use Smaller and More Controlled Data Structures: Where appropriate, opt for smaller, primitive data types over larger objects. This reduces the memory footprint and consequently the amount of garbage produced.
// Instead of using Integer, use int
int[] values = new int[100]; // Lightweight and quicker allocation
- Preallocate Memory: If the max size of a data structure is known, allocate it upfront to avoid dynamic resizing.
List<String> list = new ArrayList<>(100); // Predefined size
- Use Weak References: In certain scenarios, consider using
WeakReference
to allow the garbage collector to reclaim memory of objects that are not heavily used.
WeakReference<MyClass> weakRef = new WeakReference<>(new MyClass());
3. Optimize Your Code Structure
The way you structure your code plays a vital role in performance. Here are some techniques you can use:
- Reduce Object Creation: Use object pooling strategies to reuse objects rather than creating and destroying them frequently. This helps minimize garbage collection frequency.
class ObjectPool {
private List<MyObject> available = new ArrayList<>();
public MyObject borrowObject() {
if(available.isEmpty()) {
return new MyObject();
}
return available.remove(available.size() - 1);
}
public void returnObject(MyObject obj) {
available.add(obj);
}
}
- Minimize Synchronization: Avoid synchronized methods where possible. Use concurrent data structures like
ConcurrentHashMap
that minimize locking overhead.
4. Real-Time Java
If your application demands real-time performance, consider using Real-Time Java technologies, such as the Real-Time Specification for Java (RTSJ). This specification introduces features that help ensure predictable response times.
- Scoped Memory Areas: Manage memory more predictively to avoid garbage collection pauses.
- No-Heap Real-Time Threads: Utilize threads that do not access the heap, ensuring no non-deterministic behavior from garbage collection.
5. Profiling and Benchmarking
It is essential to profile your application to identify bottlenecks actively. Use tools like:
- VisualVM: A visual tool that helps with profiling and monitoring memory usage.
- Java Mission Control: A tool to analyze the performance of Java applications.
Benchmark your application under real workload conditions and adjust based on the results. Identify which areas can be optimized without significant rewrites.
6. Choose Native Libraries When Necessary
In cases where performance remains an issue, consider utilizing native code through the Java Native Interface (JNI). This allows you to write performance-critical components in C or C++ while still utilizing Java for the majority of your application.
public class MyNativeClass {
static {
System.loadLibrary("my_native_library");
}
// Native method declaration
public native void performCriticalOperation();
}
Final Considerations
Overcoming Java's performance hurdles in embedded systems is undeniably challenging, but with the right tools and techniques, it is achievable. By choosing appropriate JVM, managing memory, optimizing code, leveraging real-time Java, and profiling, developers can harness Java's benefits while minimizing its drawbacks.
Java is increasingly becoming a strong contender in the embedded systems arena. Stay updated with the latest developments in the Java ecosystem, as new technologies and practices continue to emerge.
For further reading, check out these resources:
By implementing these strategies, you can improve your Java applications’ performance in embedded systems, reaping the rewards of Java's capabilities without the typical performance penalties.
Checkout our other articles