Troubleshooting Java Process Interaction in OS Environments
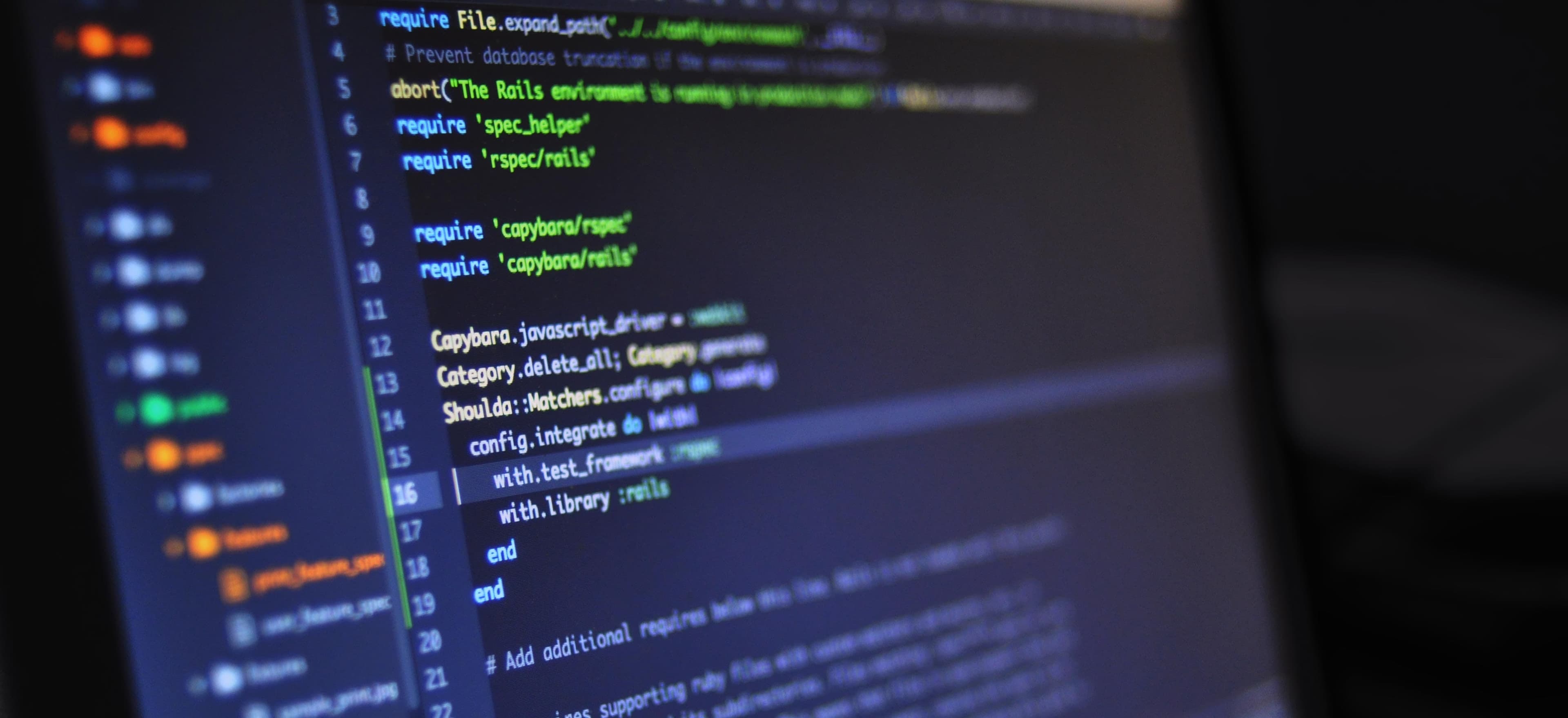
- Published on
Troubleshooting Java Process Interaction in OS Environments
Java is a powerful programming language with a platform-independent philosophy. One of its standout features is the ability to interact with processes in an operating system (OS) environment. However, this interaction can sometimes lead to unexpected behaviors. In this blog post, we will explore how Java interacts with OS processes, common issues that might arise during this interaction, and effective troubleshooting strategies.
Understanding Java Process Interaction
In Java, a process refers to a program in execution. Interacting with another process can require starting, reading output, writing input, and handling errors. ProcessBuilder
and Runtime.exec()
methods are commonly used for these purposes. Let's delve into the ProcessBuilder
class.
Using ProcessBuilder
The ProcessBuilder
class provides a way to create operating system processes. It is more flexible compared to Runtime.exec()
, allowing for redirection of input and output, environment variable manipulation, and altering the working directory, which makes it a favorite among developers.
Example Code Snippet
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.io.IOException;
public class ProcessBuilderExample {
public static void main(String[] args) {
ProcessBuilder processBuilder = new ProcessBuilder("ping", "www.google.com");
// Redirecting error stream to standard output
processBuilder.redirectErrorStream(true);
try {
Process process = processBuilder.start();
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
int exitCode = process.waitFor();
System.out.println("Exited with code: " + exitCode);
} catch (IOException | InterruptedException e) {
e.printStackTrace();
}
}
}
Commentary
In this example, we create a ProcessBuilder
instance to execute the ping
command. We redirect the error stream to the standard output stream (for easier debugging). This code efficiently reads output line by line and handles potential exceptions.
Common Issues in Java Process Interaction
-
Blocked Input/Output Streams: Improper handling of output and error streams might cause the process to hang. Always ensure that these streams are read in a separate thread if they are expected to produce a significant amount of data.
-
Permission Denied: Running system commands may fail due to insufficient permissions. Make sure to check user permissions when attempting to execute commands.
-
Command Not Found: The command given in
ProcessBuilder
must exist on the system's path. Double-check the command syntax and the environment. -
Environment Variables: Sometimes, an application might depend on specific environment variables. Make use of the
environment()
method ofProcessBuilder
to manipulate these variables as needed.
Debugging Strategies
When troubleshooting Java process interactions in an OS environment, consider the following strategies:
1. Stream Management
As mentioned, manage the input and output streams correctly. Use separate threads for reading output and error streams to prevent deadlocks.
Example Code Snippet
private static void readStream(final InputStream inputStream) {
new Thread(() -> {
try (BufferedReader reader = new BufferedReader(new InputStreamReader(inputStream))) {
String line;
while ((line = reader.readLine()) != null) {
System.out.println(line);
}
} catch (IOException e) {
e.printStackTrace();
}
}).start();
}
2. Logging
Enhanced logging can provide insights during debugging. Log the command being executed, and capture the error messages accurately to trace issues effectively.
System.out.println("Executing command: " + String.join(" ", processBuilder.command()));
3. Exit Codes
Always check the exit code of the process once it has finished. An exit code of 0
typically means success, while any non-zero code indicates an error.
int exitCode = process.waitFor();
if (exitCode != 0) {
System.err.println("Process terminated with error code: " + exitCode);
}
4. Testing Isolation
If your process interacts with external services, consider creating unit tests that isolate these calls. Utilizing mocking frameworks can help simulate different responses and failure conditions.
5. Consult the Documentation
Sun's Java documentation provides expansive insights into how processes work in Java. Familiarizing yourself with these resources can greatly improve troubleshooting efforts.
In Conclusion, Here is What Matters
Java's ability to interact with processes within an OS opens up a world of possibilities. However, the inherent complexities can lead to challenges that require methodical troubleshooting approaches. By managing streams efficiently, implementing robust logging techniques, checking exit codes, and isolating tests, you can effectively address issues that arise during process interaction.
If you aim to deepen your understanding further, consider exploring additional resources such as the Java Concurrency documentation for threads and process handling in detail.
With these strategies in hand, you'll be well-prepared to tackle Java process interaction challenges head-on. Happy coding!
Checkout our other articles