Handling Module Conflicts in Java's Module System
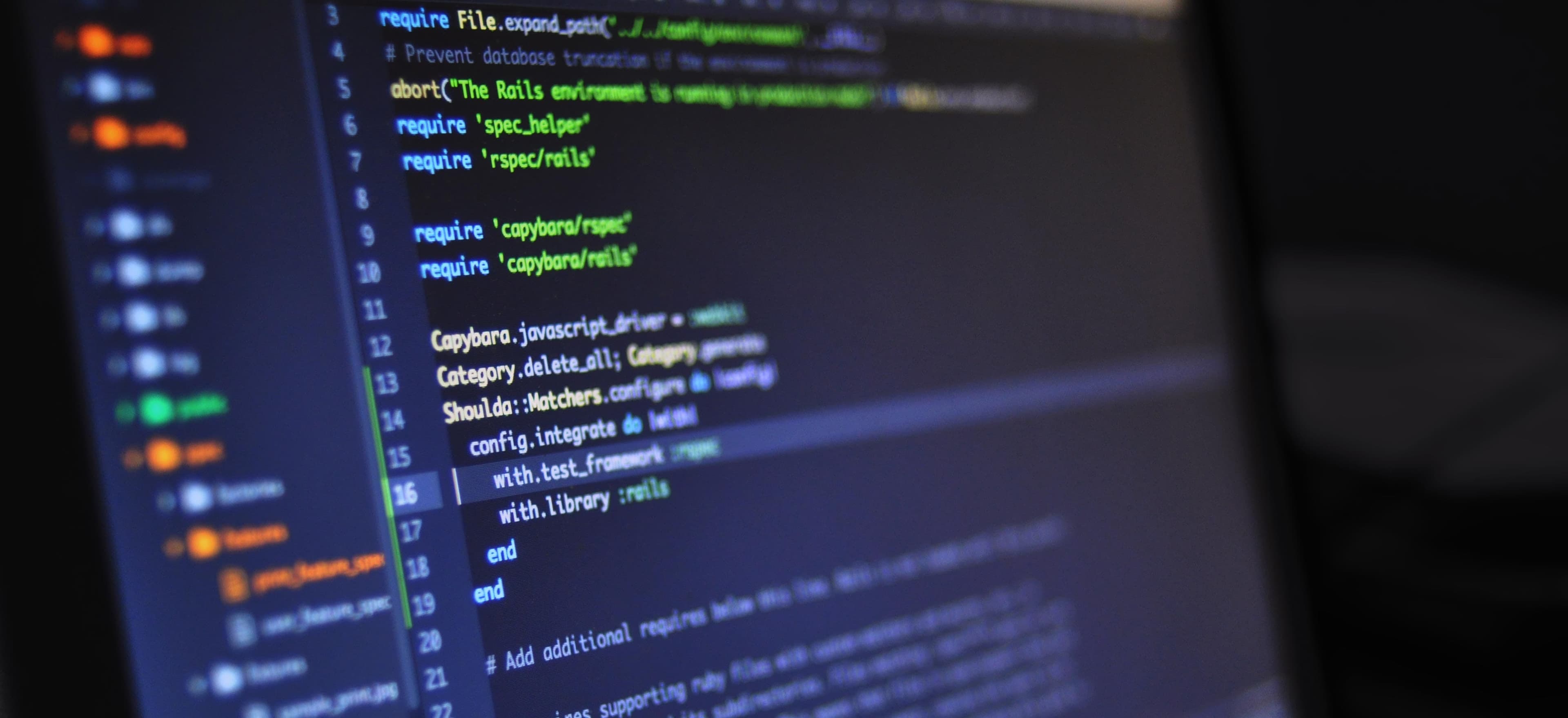
- Published on
Handling Module Conflicts in Java's Module System
Java's module system, introduced in Java 9, offers a powerful way to organize code and manage dependencies. However, it also introduces challenges, especially when it comes to handling module conflicts. In this post, we’ll explore the Java Module System, understand how to manage module conflicts, and provide code examples to highlight solutions.
Understanding the Java Module System
The Java Module System allows developers to break their applications into smaller, more manageable parts known as modules. Each module is defined in a module-info.java
file, which describes its dependencies and the packages it exports to other modules.
Key Benefits of the Module System
- Encapsulation: Modules can restrict access to their internals, exposing only what is necessary.
- Reduced Namespace Clutter: By grouping packages into modules, the overall namespace of Java applications is cleaner.
- Improved Dependency Management: Modules make it easier to manage dependencies explicitly, reducing potential conflicts.
What Are Module Conflicts?
Module conflicts occur when two modules define the same package or when there are version mismatches among modules that are being used together. This can lead to runtime errors, making the application unstable or unusable.
Example Scenario
Imagine you have two modules, moduleA
and moduleB
, both exporting the package com.example.utils
. If both modules are included in your application, the Java runtime cannot resolve which com.example.utils
package to use, leading to conflicts.
Managing Module Conflicts
1. Avoiding Conflicts by Refactoring
The best way to avoid module conflicts is to ensure that your application's design does not lead to them. Refactoring your modules can significantly reduce the chances of conflicts occurring.
Code Example 1: Refactoring Modules
Suppose you have two modules that have overlapping package names. You can refactor them to create a unique package for each.
// In moduleA
package com.example.moduleA.utils;
public class Utility {
public static void doSomething() {
System.out.println("Doing something in moduleA");
}
}
// In moduleB
package com.example.moduleB.utils;
public class Utility {
public static void doSomething() {
System.out.println("Doing something in moduleB");
}
}
In this refactored example, the packages are distinct, which avoids conflicts entirely.
2. Using the requires transitive
Directive
The requires transitive
directive allows a module to inherit the dependencies of another module. This can help if multiple modules depend on a common module, ensuring that the correct version is used.
Code Example 2: Using requires transitive
module moduleA {
requires transitive moduleCommon;
exports com.example.moduleA;
}
module moduleB {
requires moduleCommon;
exports com.example.moduleB;
}
// moduleCommon
module moduleCommon {
exports com.example.common;
}
In this setup, moduleB
can use the exported members of moduleCommon
, inheriting all necessary functionality without redefining it.
3. Utilizing the --patch-module
Option
When working with modules, sometimes you encounter a situation where a specific conflict arises due to multiple versions of a module being compiled. The Java Virtual Machine (JVM) provides the --patch-module
option during execution to resolve this.
Example of --patch-module
java --patch-module moduleA=path/to/your/modified/moduleA.jar -m moduleMain/com.example.Main
This command allows the JVM to use a tailored version of moduleA
, effectively overriding the default and mitigating conflict risks.
4. Leveraging the Java Module System’s Services
The service loader mechanism in Java can also help manage module conflicts. By leveraging services, you can provide different implementations for certain functionalities, thus maintaining modular integrity.
Code Example 3: Using Service Loader
// Define a service interface
public interface ProcessorService {
void process();
}
// moduleA provides an implementation
module moduleA {
provides ProcessorService with com.example.moduleA.ProcessorA;
}
// moduleB provides an implementation that can be loaded
module moduleB {
provides ProcessorService with com.example.moduleB.ProcessorB;
}
In your application, you can dynamically load implementations of ProcessorService
without worrying about direct dependencies that could lead to conflicts.
Loading the Service
ServiceLoader<ProcessorService> serviceLoader = ServiceLoader.load(ProcessorService.class);
for (ProcessorService processor : serviceLoader) {
processor.process(); // Uses the appropriate implementation
}
5. Version Management with Dependency Management Tools
When feasible, employ dependency management tools like Maven or Gradle. These tools offer plugins that resolve conflicts automatically by selecting the latest compatible version or by excluding specific transitive dependencies that may cause conflict.
Using Maven as an Example
With Maven, conflicts can be minimized by managing versions in the pom.xml
.
<dependencyManagement>
<dependencies>
<dependency>
<groupId>com.example</groupId>
<artifactId>moduleA</artifactId>
<version>1.0</version>
</dependency>
<dependency>
<groupId>com.example</groupId>
<artifactId>moduleB</artifactId>
<version>1.0-SNAPSHOT</version>
<exclusions>
<exclusion>
<groupId>com.example</groupId>
<artifactId>conflicting-module</artifactId>
</exclusion>
</exclusions>
</dependency>
</dependencies>
</dependencyManagement>
Useful Resources
For a deeper dive into the Java Module System and handling of conflicts, consider these resources:
Final Thoughts
Managing module conflicts in Java's Module System is essential for building stable applications. By following best practices such as refactoring code, utilizing the requires transitive
directive, and leveraging services, you can minimize or even avoid conflicts altogether. With various tools at your disposal, handling module dependencies can become a streamlined process. As the module system continues to evolve, staying informed will enable you to better utilize its features for robust application design.
By understanding these key concepts and strategies, you can harness the full power of Java’s module system to create cleaner, more manageable, and conflict-free applications.