Why Java Security Manager Is Failing Your Application's Safety
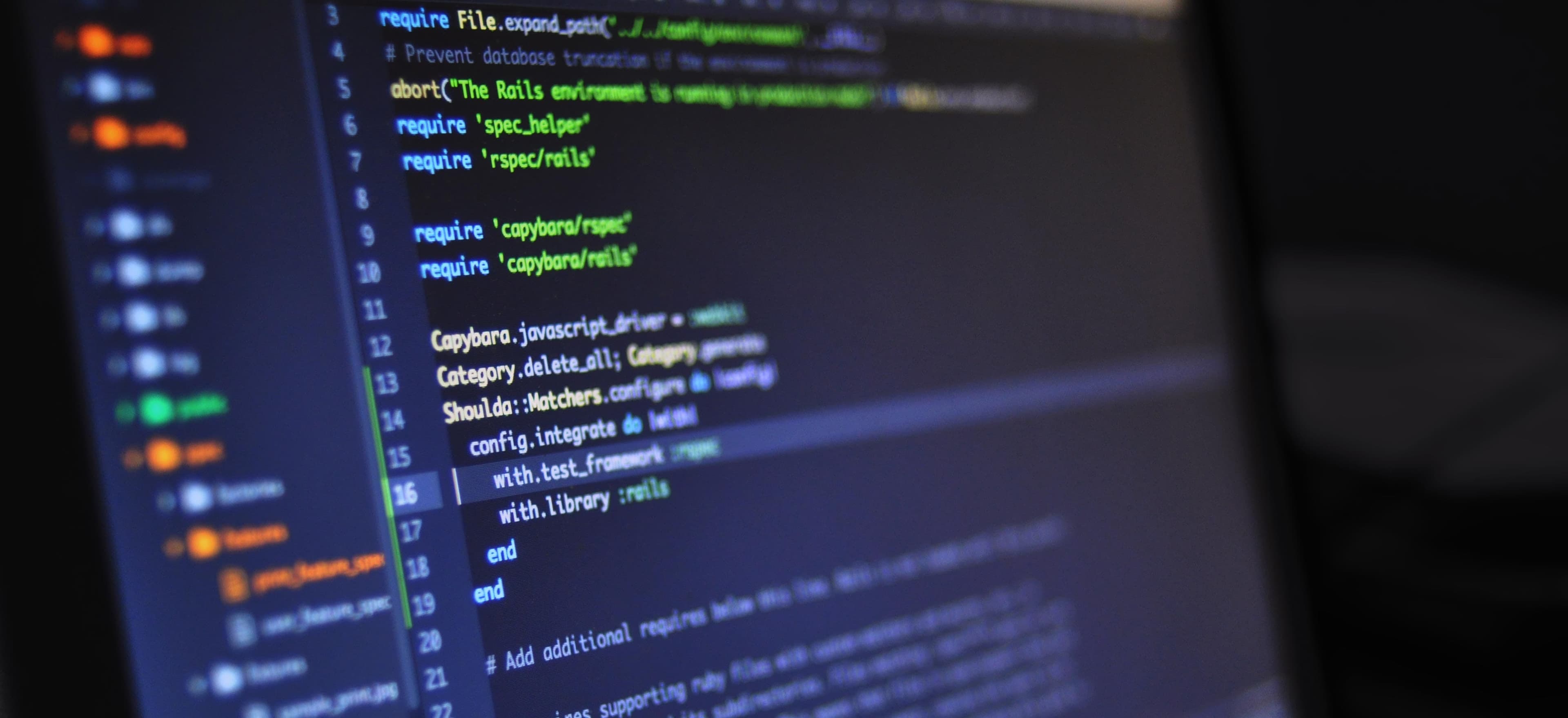
- Published on
Why Java Security Manager Is Failing Your Application's Safety
In an era where application security is of paramount importance, Java developers often turn to the Java Security Manager as a first line of defense. Introduced in Java 1.0, the Security Manager was designed to enforce security policies in Java applications, particularly when running untrusted code. However, despite its long-standing presence, many developers find that relying solely on the Java Security Manager can undermine their application's safety.
In this blog post, we will explore the limitations of the Java Security Manager, why it may fail to provide the expected safety, and offer potential alternatives and best practices.
What Is the Java Security Manager?
The Java Security Manager is a class that allows developers to set up security policies in their applications. It enables you to control the permissions of various operations, such as file access, network connections, and process execution. By defining a security policy, you can specify what code can and cannot do.
Why Use the Java Security Manager?
- Granular Permissions: It allows developers to enforce fine-grained control over what code can perform sensitive operations.
- Enhanced Protection: The Security Manager is useful in environments where untrusted code needs to be executed, such as applets and plugin architectures.
- Legacy Support: Some applications still rely on the Security Manager for backward compatibility reasons.
Given these points, it might seem that the Security Manager is a robust solution for application safety. However, let’s delve into some of its key shortcomings.
Limitations of the Java Security Manager
1. Overhead and Complexity
Setting up and maintaining security policies can become increasingly complex, especially as applications grow. Security policies are typically defined in external policy files, which can create a performance overhead. This leads to longer loading times and may result in a more complicated deployment process.
// Sample policy file
grant {
permission java.io.FilePermission "/tmp/*", "read,write";
};
In the example above, you need to properly declare permissions, and any oversight or misconfiguration can expose the application to security risks.
2. Not Foolproof
The Security Manager isn’t foolproof. Attackers have discovered numerous vulnerabilities in Java's security architecture that can bypass the Security Manager entirely. For instance, certain classes, like java.lang.reflect
, can execute operations that might not be permitted on the surface level.
import java.lang.reflect.Field;
public class UnsafeAccess {
public static void main(String[] args) throws Exception {
Field field = String.class.getDeclaredField("value");
field.setAccessible(true);
char[] value = (char[]) field.get("secret");
// Now the application can manipulate 'value' directly.
}
}
In this code, by using reflection, an attacker could potentially modify application internals, bypassing safeguards set by the Security Manager.
3. Deprecation and Future Trends
Given the challenges and limitations, it's worth noting that Oracle has deprecated the Java Security Manager as of JDK 17. This decision indicates a trend moving away from the Security Manager for managing security, pushing developers towards more modern security practices.
Alternatives to Java Security Manager
As developers seek more viable solutions for application safety, consider the following alternatives:
1. Java Platform Module System (JPMS)
With the introduction of JPMS in Java 9, modularity is now a core feature of Java. The module system allows you to better control what each part of your application can access. By clearly defining module boundaries, you can reduce opportunities for malicious code to interact in undesired ways.
// module-info.java
module my.module {
exports com.mydomain.safe;
requires java.base;
}
2. Security Frameworks and Libraries
Consider leveraging established security frameworks such as Spring Security or Apache Shiro. These libraries provide robust authentication, authorization, and security mechanisms that are well-maintained, regularly updated, and widely adopted.
Spring Security Example:
@EnableWebSecurity
public class SecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(HttpSecurity http) throws Exception {
http.csrf().disable() // Disable CSRF for this example only
.authorizeRequests()
.antMatchers("/public/**").permitAll()
.anyRequest().authenticated();
}
}
This example demonstrates how easy it can be to secure individual routes in a web application using Spring Security.
3. Containerization and Isolation
With the rise of container technologies (like Docker), developers can isolate their applications in secure environments. This means if an application were to be compromised, the impact would be limited to that container, rather than the entire server.
4. Static Code Analysis
Analyzing your codebase for potential security vulnerabilities using tools like FindBugs or SonarQube can help identify risky patterns before they become an issue in production. Ensuring proper coding practices and secure coding patterns helps reduce vulnerabilities from the outset.
Best Practices for Java Application Security
- Keep Your Dependencies Updated: Software libraries frequently offer updates to fix security vulnerabilities. Always ensure that you are using the latest versions.
- Employ Vulnerability Scanners: Use tools like OWASP Dependency-Check to identify vulnerable dependencies within your project.
- Educate Your Team: Regular training on secure coding techniques will help reduce the risk of human error leading to security flaws.
- Implement Continuous Security: Incorporate security checks into your CI/CD pipelines. This helps ensure that security is considered at every stage of development.
Closing Remarks
The Java Security Manager, despite its historical significance and utility, is no longer a reliable cornerstone for securing Java applications. Its limitations, coupled with the shift towards modern development practices, suggest it might be time to migrate to alternative security measures that offer greater robustness and reliability.
For further reading on Java application security, you might find the following resources useful:
- Oracle: Security in Java
- OWASP: Top Ten Java Security Issues
Embracing modern security frameworks, modularization, and continuous security practices will help elevate the safety of your Java applications far beyond the capabilities of the traditional Security Manager. Secure your applications today for a safer tomorrow.