Choosing Between JavaFX and Swing: The Performance Debate
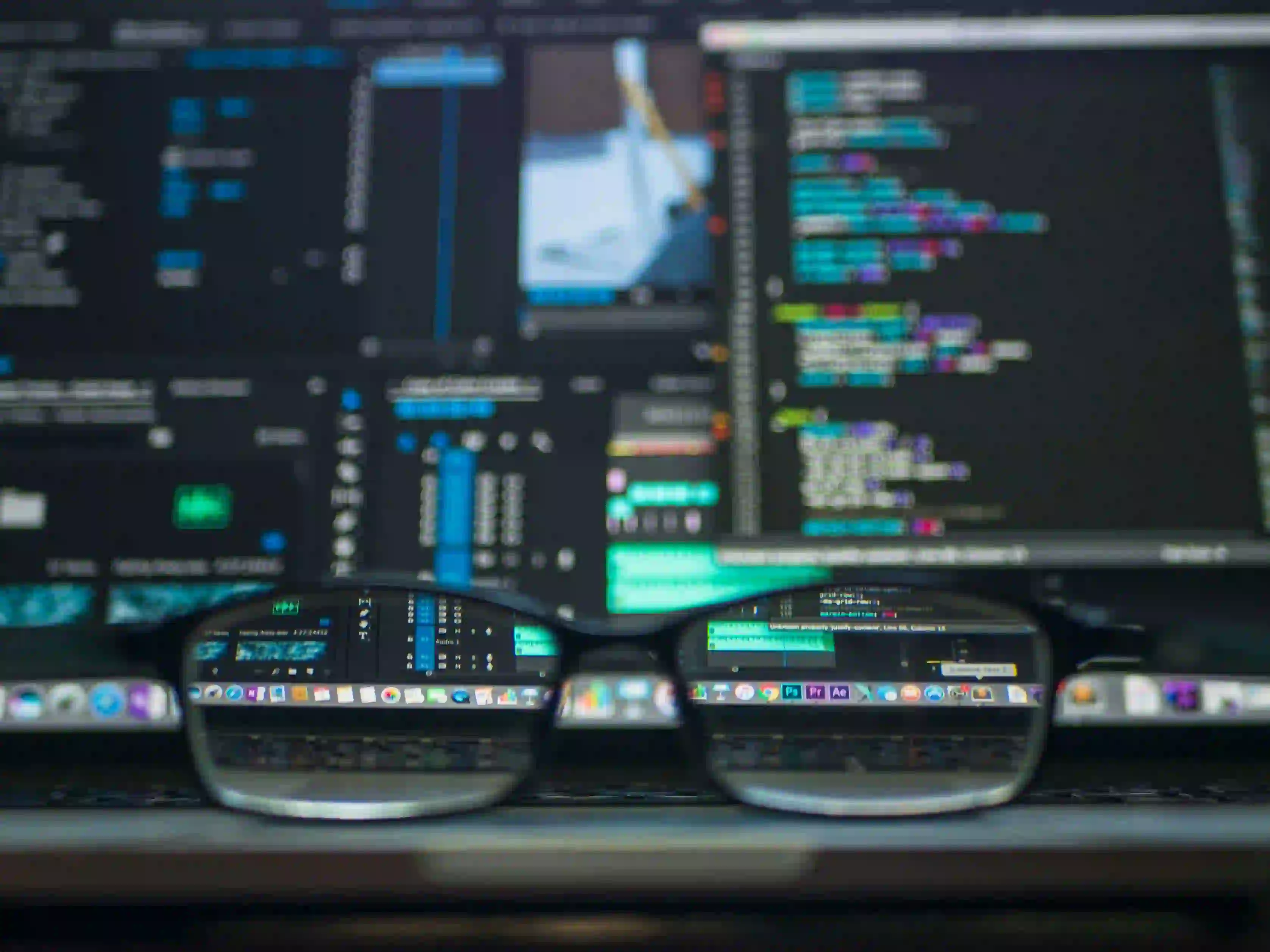
Choosing Between JavaFX and Swing: The Performance Debate
Java has a rich toolkit for developing graphical user interfaces (GUIs), and among the most prominent libraries are JavaFX and Swing. Both have their distinct strengths and weaknesses, but when it comes to performance, a thorough understanding is crucial. In this post, we will explore JavaFX and Swing, comparing their performance, usability, and functionalities, so you can make an informed decision for your next project.
The Legacy of Swing
Swing has been part of Java since version 1.2. It is a mature library, rich with components and known for its capability to create complex GUI applications. The following code snippet demonstrates a simple Swing application:
import javax.swing.JButton;
import javax.swing.JFrame;
import javax.swing.JPanel;
public class SimpleSwingApp {
public static void main(String[] args) {
JFrame frame = new JFrame("Simple Swing Example");
JPanel panel = new JPanel();
JButton button = new JButton("Click Me!");
button.addActionListener(e -> System.out.println("Button clicked!"));
panel.add(button);
frame.add(panel);
frame.setSize(300, 200);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setVisible(true);
}
}
Why Use Swing?
- Mature and Stable: Swing has been around for decades, making it a tried-and-true choice for enterprise applications.
- Excellent Documentation: The extensive documentation and numerous community resources make Swing accessible even to newbies.
- Wide Component Variety: Swing provides a wide array of customizable components.
However, Swing does come with its limitations. Being reliant on the older AWT (Abstract Window Toolkit), its performance can lag behind newer technologies.
Enter JavaFX
Introduced in Java 8, JavaFX was designed as a modern replacement for Swing, with a focus on rich internet applications. Its capabilities go beyond desktop applications, allowing developers to build applications that can run on a multitude of platforms.
Below is a simple JavaFX application:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class SimpleJavaFXApp extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
Button button = new Button("Click Me!");
button.setOnAction(e -> System.out.println("Button clicked!"));
StackPane layout = new StackPane(button);
Scene scene = new Scene(layout, 300, 200);
primaryStage.setScene(scene);
primaryStage.setTitle("Simple JavaFX Example");
primaryStage.show();
}
}
Why Opt for JavaFX?
- Modern UI Design: With FXML and CSS styling, JavaFX applications can look visually appealing.
- Web Integration: JavaFX can render web content and allows for the integration of web libraries.
- Better Performance: JavaFX uses hardware-accelerated graphics, which can greatly improve performance, especially for animation-heavy applications.
Performance Comparison
Now that we have a fundamental understanding of both frameworks, let’s focus on performance. Below are key areas for comparison:
1. Rendering Performance
JavaFX employs a scene graph that is highly optimized for rendering, which means it can handle complex graphics more efficiently than Swing. Given that JavaFX utilizes hardware acceleration, animations and graphics render smoothly, providing a better user experience.
2. Memory Consumption
Memory consumption is another crucial factor, especially for applications that run on constrained environments. JavaFX typically requires a larger footprint due to more advanced features. However, this can be mitigated by effective resource management and mindful coding practices.
3. Threading Model
Both Swing and JavaFX have different approaches to threading. Swing uses a single-threaded model, which can lead to performance issues if the UI thread is blocked. JavaFX, on the other hand, has a better-managed threading model, allowing background tasks to run without hanging the UI.
import javafx.concurrent.Task;
// Background task example in JavaFX
Task<Void> task = new Task<Void>() {
@Override
protected Void call() throws Exception {
// Long-running background operation
Thread.sleep(2000);
return null;
}
};
new Thread(task).start();
4. Component Performance
When it comes to responsiveness and component interaction, JavaFX often outperforms Swing. Swing’s components may feel sluggish, particularly when rendering complex interfaces. In contrast, JavaFX components have a more refined mechanism for responding to user interactions and rendering updates.
Use Cases: When to Choose Which?
While both JavaFX and Swing offer their unique advantages, your project requirements should guide your decision. Here are some considerations:
-
Legacy Systems: If you're working with an existing Swing application or require a stable environment, Stick with Swing.
-
New Applications: For modern applications with rich graphical interfaces, JavaFX is the preferred choice due to its enhanced performance and aesthetics.
-
Platform Dependence: Consider JavaFX for applications that aim to run on multiple platforms, including browsers.
Bringing It All Together
In conclusion, both JavaFX and Swing serve important roles in Java GUI development. Swing remains a solid choice for legacy or enterprise applications, thanks to its stability and extensive feature set. Conversely, JavaFX is suited for modern, visually appealing applications with a need for superior performance.
Ultimately, your project's requirements and your programming environment should dictate your choice between JavaFX and Swing. If you're interested in delving deeper into JavaFX or Swing, consider reading through the Java documentation here for JavaFX and here for Swing.
Happy coding!