Mastering Wildcards in Java Generics: Common Pitfalls Explained
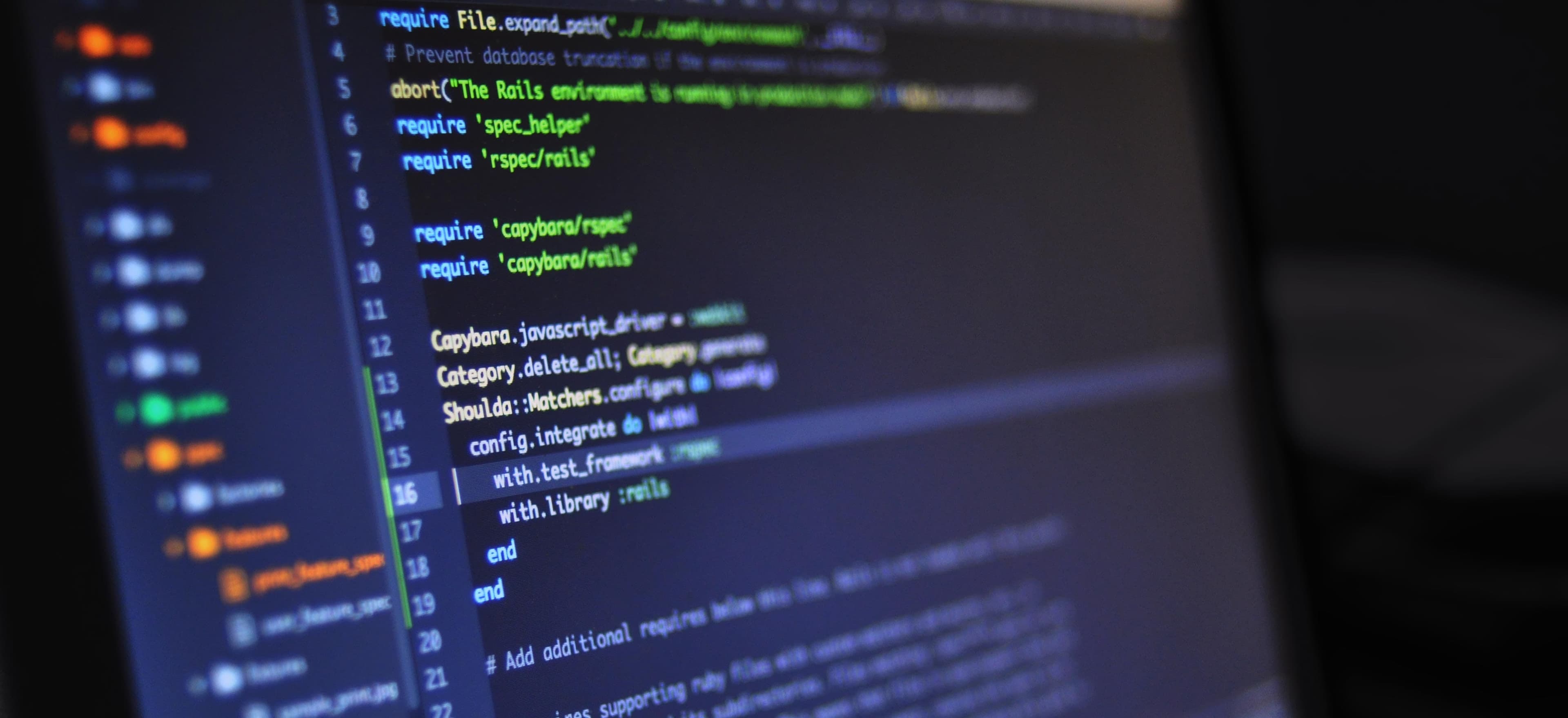
- Published on
Mastering Wildcards in Java Generics: Common Pitfalls Explained
Java Generics is a powerful feature that allows developers to write code with more flexibility and security, reducing the risk of runtime errors related to type casting. However, the introduction of generics also brought a level of complexity, particularly with the use of wildcards. In this blog post, we will explore wildcards in Java Generics, common pitfalls, and best practices to help you harness their full potential.
Understanding Wildcards in Java Generics
A wildcard in Java Generics is represented by the question mark (?
). It signifies an unknown type. Wildcards are particularly useful in the following situations:
- Unknown Type: When you want to relax the restrictions on a type parameter.
- Functionality: To allow a method to operate on different types of collections.
There are three main types of wildcards in Java:
- Unbounded Wildcards: Denoted as
<?>
, they allow any type. - Upper Bounded Wildcards: Denoted as
<? extends Type>
, they restrict the unknown type to be a subtype ofType
. - Lower Bounded Wildcards: Denoted as
<? super Type>
, they restrict the unknown type to be a supertype ofType
.
Let's break this down further.
1. Unbounded Wildcards
Unbounded wildcards are useful when you want to work with a method without knowing the specific type of the parameter. For example:
import java.util.List;
public class UnboundedWildcardExample {
public static void printList(List<?> list) {
for (Object element : list) {
System.out.println(element);
}
}
}
Why Use Unbounded Wildcards?
Using List<?>
allows you to accept lists of any type without specifying it. This makes the method flexible and reusable.
2. Upper Bounded Wildcards
Upper bounded wildcards are utilized when you wish to accept a type or its subtypes. This is useful when you want to perform operations on a base class type and its subclasses.
Consider this implementation:
import java.util.ArrayList;
import java.util.List;
class Animal {
public void speak() {
System.out.println("Animal speaks");
}
}
class Dog extends Animal {
@Override
public void speak() {
System.out.println("Dog barks");
}
}
class Cat extends Animal {
@Override
public void speak() {
System.out.println("Cat meows");
}
}
public class UpperBoundedWildcardExample {
public static void makeSound(List<? extends Animal> animals) {
for (Animal animal : animals) {
animal.speak();
}
}
public static void main(String[] args) {
List<Dog> dogs = new ArrayList<>();
dogs.add(new Dog());
makeSound(dogs); // Outputs: Dog barks
List<Cat> cats = new ArrayList<>();
cats.add(new Cat());
makeSound(cats); // Outputs: Cat meows
}
}
Why Use Upper Bounded Wildcards?
The <? extends Animal>
signature allows you to pass lists of Dog
, Cat
, or any other subclass of Animal
. It provides type safety while promoting code reuse.
3. Lower Bounded Wildcards
Lower bounded wildcards enable you to work with a type and its supertypes. This is often used in methods that need to add elements to generic collections.
Here’s an example:
import java.util.ArrayList;
import java.util.List;
public class LowerBoundedWildcardExample {
public static void addAnimals(List<? super Dog> list) {
list.add(new Dog());
}
public static void main(String[] args) {
List<Animal> animals = new ArrayList<>();
addAnimals(animals);
List<Object> objects = new ArrayList<>();
addAnimals(objects);
}
}
Why Use Lower Bounded Wildcards?
In this case, <? super Dog>
allows adding Dog
instances to the list, which could be a list of Animal
or even Object
. This facilitates the addition of specific types into collections meant for broader types.
Common Pitfalls with Wildcards in Java Generics
While wildcards are incredibly useful, they can also lead to confusion and errors if not understood correctly. Here are some common pitfalls:
Pitfall 1: Confusing Upper and Lower Bounds
Developers often confuse upper and lower bounds when using wildcards. Remember:
- Upper bounds (
<? extends Type>
) are for reading from a structure. You cannot add to it. - Lower bounds (
<? super Type>
) are for writing to a structure. You cannot read from it directly.
Pitfall 2: Type Inference Limitations
Java’s type inference can sometimes lead to unexpected outcomes, especially when multiple generics are involved. For instance:
public class TypeInferenceExample {
public static <T> void process(List<T> list) {
// This will work fine
}
public static void main(String[] args) {
List<?> wildcardList = new ArrayList<String>();
// process(wildcardList); // Compile-time error
}
}
Java’s type system doesn’t know what specific type it is dealing with in wildcardList
, leading to compile-time errors.
Pitfall 3: Collecting Data with Wildcards
You cannot add elements to a collection obtained with an upper bounded wildcard. For example:
public static void addToList(List<? extends Number> list) {
// list.add(5); // Compile-time error
}
The reason is that list
might be a List<Integer>
, and adding an Integer
might break type safety.
Best Practices for Using Wildcards
1. Use Wildcards to Make APIs More General
When designing methods or classes, prefer using wildcards over specific types when the type is not critical to the implementation. This improves the flexibility of your APIs.
2. Be Explicit with Upper and Lower Bounds
Always clarify your intent when using wildcards. Specify whether you are using upper or lower bounds based on whether you intend to read or write.
3. Avoid Using Wildcards in Object-Oriented Hierarchies
Stick to the principle of "use generics to model the relationships between types." Avoid overly complex hierarchy designs that require wildcards at every level.
To Wrap Things Up
The integration of wildcards in Java Generics allows for greater flexibility and reusability of code. Understanding and mastering wildcards is essential for building robust applications. By recognizing common pitfalls and employing best practices, you can effectively leverage the power of wildcards without falling into their complexities.
For further reading, you may check the Java Tutorials on Generics, which provides deeper insights into this powerful feature.
Whether you are a seasoned developer or just starting, incorporating wildcards into your Java toolkit can significantly enhance your coding efficiency. Happy coding!
Checkout our other articles