Mastering Java: Common Data Structure Pitfalls
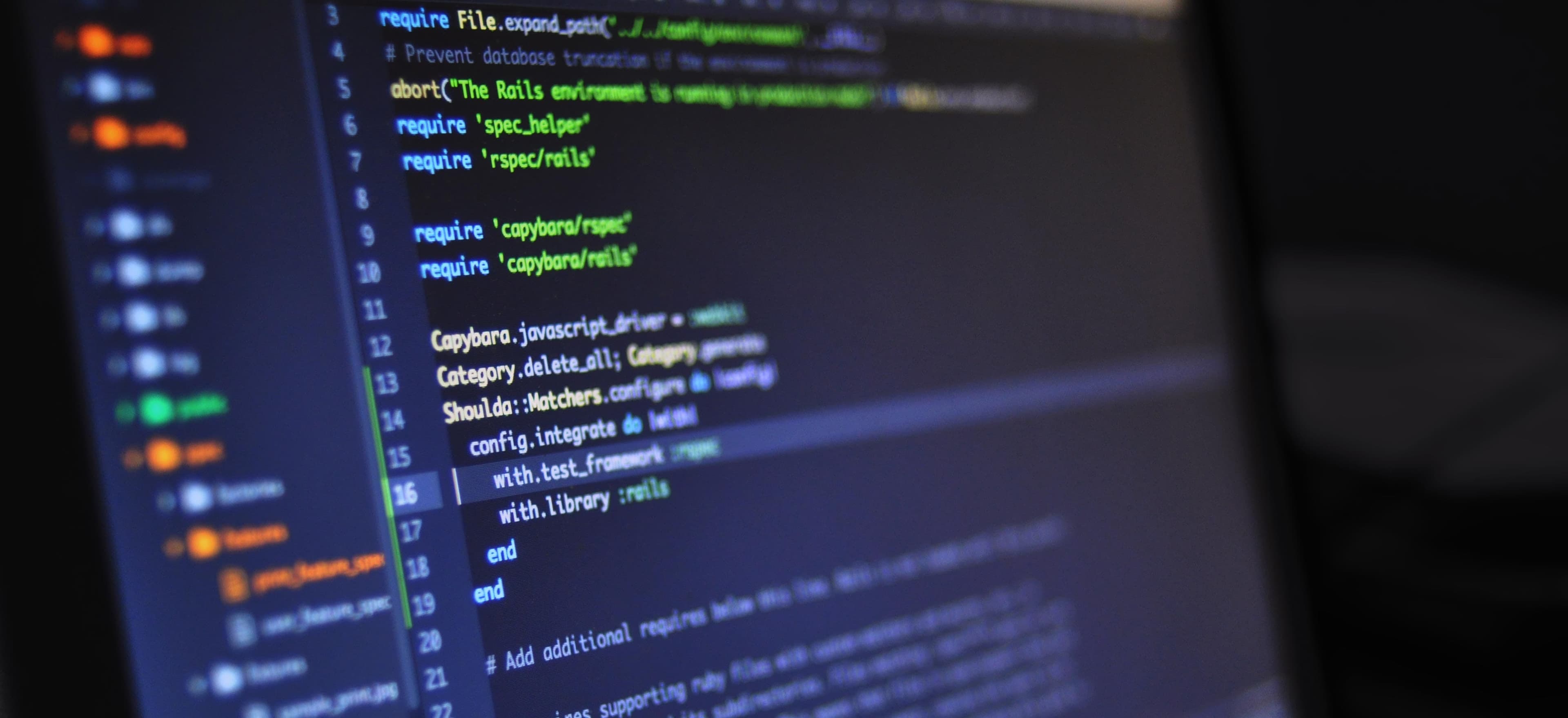
- Published on
Mastering Java: Common Data Structure Pitfalls
Java is a versatile and powerful programming language, widely used for building robust applications. Among its many features, the manipulation of data structures stands out as a crucial skill for any Java developer. However, with this power comes responsibility. Misunderstanding or poorly utilizing these structures can lead to significant pitfalls. In this blog post, we will explore some of the most common data structure pitfalls in Java, detailing how to avoid them and improve your coding practices.
1. Understanding Data Structures in Java
Before diving into common pitfalls, it's essential to grasp what data structures are. In essence, data structures are specialized formats for organizing and storing data in a way that enables efficient access and modification. Java provides several built-in data structures through the Java Collections Framework, including:
- List: An ordered collection that can contain duplicate elements (e.g.,
ArrayList
,LinkedList
). - Set: A collection that does not allow duplicate elements (e.g.,
HashSet
,TreeSet
). - Map: A collection of key-value pairs (e.g.,
HashMap
,TreeMap
).
Each of these data structures has its own use case, performance implications, and operational intricacies. However, many developers fall into traps when incorrectly using these structures. Here are some common pitfalls to avoid.
2. Pitfall: Using the Wrong Data Structure
Choosing the appropriate data structure for your needs is fundamental. Using an unsuitable structure can lead to inefficient code that is hard to maintain.
Example
Suppose you need to store a unique collection of elements and check for existence frequently. Using an ArrayList
would be a poor choice here, as it requires O(n) time complexity to check for existence, whereas a HashSet
provides O(1) average time complexity.
Code Snippet
// Poor choice, O(n) time complexity
List<String> namesList = new ArrayList<>();
namesList.add("Alice");
namesList.add("Bob");
if (!namesList.contains("Charlie")) {
namesList.add("Charlie");
}
// Better choice, O(1) time complexity
Set<String> namesSet = new HashSet<>();
namesSet.add("Alice");
namesSet.add("Bob");
if (!namesSet.contains("Charlie")) {
namesSet.add("Charlie");
}
In this example, switching from an ArrayList
to a HashSet
dramatically improves performance. The right choice of data structures makes a world of difference.
3. Pitfall: Misusing Mutable and Immutable Data Structures
In Java, some data structures are mutable (can be modified after creation) while others are immutable (cannot be changed). A common mistake is not recognizing this distinction, which leads to bugs and unexpected behavior.
Example
When using Collections.unmodifiableList()
, any attempts to modify the returned list will throw an exception.
Code Snippet
List<String> originalList = new ArrayList<>();
originalList.add("Apple");
originalList.add("Banana");
// This creates an unmodifiable view of the original list
List<String> unmodifiableList = Collections.unmodifiableList(originalList);
// This will cause UnsupportedOperationException
unmodifiableList.add("Cherry");
Why This Matters
Understanding the mutability of your data structures can prevent runtime exceptions and enhance code reliability.
4. Pitfall: Ignoring Type Safety
Java's collections are generics-based, meaning they are type-safe. However, some developers neglect to specify proper generics, leading to ClassCastException
at runtime.
Example
A List
capturing mixed types can cause issues when retrieving elements:
Code Snippet
List mixedList = new ArrayList();
mixedList.add("Hello");
mixedList.add(123);
// Unsafe cast
String s = (String) mixedList.get(0); // OK
Integer i = (Integer) mixedList.get(1); // OK
// This will throw ClassCastException when retrieving mixed types
String badCast = (String) mixedList.get(1); // Runtime exception
How to Avoid
Always use generics with collections:
List<String> safeList = new ArrayList<>();
safeList.add("Hello");
// safeList.add(123); // Compile-time error
Using generics ensures compile-time type checking, which minimizes runtime errors.
5. Pitfall: Not Understanding Performance Implications
Every data structure has specific performance characteristics in terms of time complexity for operations like insertion, deletion, and access. A common pitfall is not accounting for these implications when selecting a data structure.
Example
Consider how adding an element to an ArrayList
and a LinkedList
behaves:
// ArrayList: O(1) amortized time, but O(n) when resizing is required
List<String> arrayList = new ArrayList<>(2);
arrayList.add("Apple");
arrayList.add("Banana");
arrayList.add("Cherry"); // May require resizing
// LinkedList: O(1) for adding at the end
List<String> linkedList = new LinkedList<>();
linkedList.add("Apple");
linkedList.add("Banana");
linkedList.add("Cherry"); // No resizing
Performance Strategy
Knowing these complexities allows you to make better decisions based on anticipated use cases.
6. Pitfall: Neglecting Concurrency Concerns
In multithreaded applications, data structures become a shared resource, leading to potential race conditions. Using a standard ArrayList
or HashMap
concurrently without proper synchronization can lead to unpredictable behavior.
Solution: Use Concurrent Collections
Java provides thread-safe variants of these data structures such as CopyOnWriteArrayList
and ConcurrentHashMap
:
List<String> concurrentList = new CopyOnWriteArrayList<>();
concurrentList.add("Thread-safe Item");
// Can safely iterate or modify from multiple threads
Utilizing these concurrent collections is crucial when dealing with multiple threads accessing the same structure.
7. Conclusion
Java data structures offer immense power in data organization and manipulation. However, the myriad of choices presents pitfalls that can hinder performance, lead to runtime exceptions, and create maintenance challenges. By understanding the common pitfalls discussed in this post, developers can make more informed choices that enhance code quality and performance.
Additional Resources
For more context on Java data structures and their unique characteristics, check out these useful links:
By being mindful of these factors and committing to best practices, you can master Java's data structures and build more efficient, reliable applications. Happy coding!
Checkout our other articles