Common Pitfalls in Java and Elasticsearch Integration
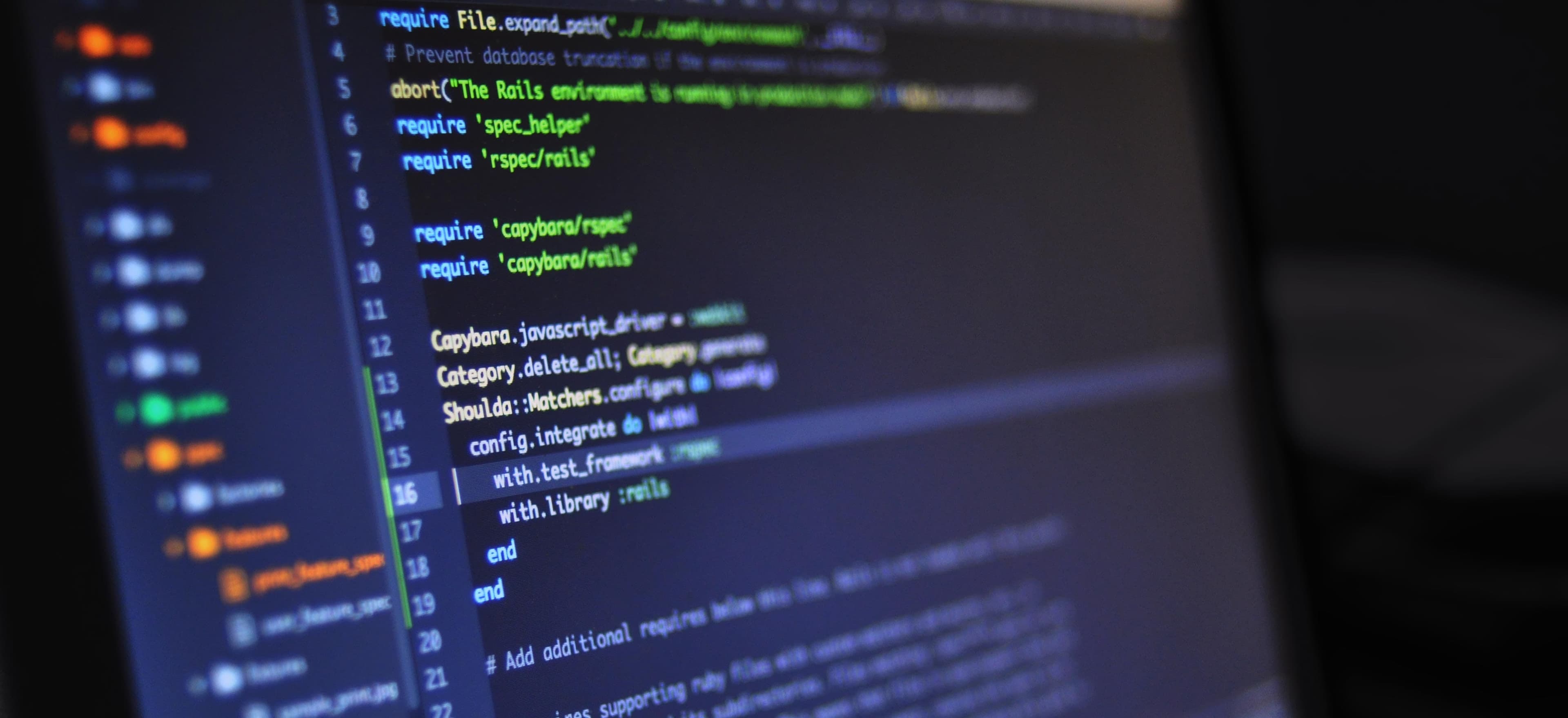
- Published on
Common Pitfalls in Java and Elasticsearch Integration
Integrating Java applications with Elasticsearch can result in powerful and efficient search capabilities. However, this integration is not without its challenges. In this blog post, we will explore some common pitfalls developers encounter during Java and Elasticsearch integration, along with best practices to avoid them. This guide is aimed at Java developers who are looking to leverage Elasticsearch for their real-time search needs.
The Starting Line to Elasticsearch
Elasticsearch is a distributed, RESTful search and analytics engine capable of handling large volumes of data in real time. It is widely used due to its powerful querying capabilities and scalability. When combined with Java, it allows developers to build applications that can process and analyze data quickly.
Common Pitfalls and How to Avoid Them
1. Inefficient Mapping
One of the most critical steps in integrating Java with Elasticsearch is defining the index mapping. Poorly defined mappings can lead to unexpected results and performance issues.
Problem:
Automatically inferring mappings may not suit your data needs. For instance, Elasticsearch might interpret numeric fields as text if not defined correctly.
Solution:
Always define your mappings explicitly. This way, you ensure that each field in your document is indexed correctly:
Map<String, Object> properties = new HashMap<>();
properties.put("user", Map.of("type", "text"));
properties.put("timestamp", Map.of("type", "date"));
properties.put("message", Map.of("type", "text"));
Map<String, Object> mapping = Map.of("properties", properties);
IndexRequest request = new IndexRequest("logs")
.source(mapping);
By defining your mappings explicitly, you can manage data types effectively, ensuring consistency and better performance.
2. Ignoring Bulk Requests
When inserting a large number of documents into Elasticsearch, doing it one by one can severely impact performance. This can lead to slower processing times and higher resource consumption.
Problem:
Inserting documents individually:
for (MyDocument doc : documents) {
IndexRequest request = new IndexRequest("my_index").source(doc);
client.index(request, RequestOptions.DEFAULT);
}
Solution:
Use the Bulk Processor to index documents in batches:
BulkProcessor.Listener listener = new BulkProcessor.Listener() {
@Override
public void beforeBulk(long executionId, BulkRequest request) {
LOG.info("Adding " + request.numberOfActions() + " actions");
}
@Override
public void afterBulk(long executionId, BulkRequest request, BulkResponse response) {
if (response.hasFailures()) {
LOG.error("Bulk indexing has failures: " + response.buildFailureMessage());
}
}
@Override
public void afterBulk(long executionId, BulkRequest request, Throwable failure) {
LOG.error("Bulk indexing failed", failure);
}
};
BulkProcessor bulkProcessor = BulkProcessor.builder(
(request, bulkListener) -> client.bulkAsync(request, RequestOptions.DEFAULT, bulkListener),
listener).setBulkActions(1000).build();
for (MyDocument doc : documents) {
IndexRequest request = new IndexRequest("my_index").source(doc);
bulkProcessor.add(request);
}
By using a bulk processor, you can significantly enhance performance, reduce network overhead, and handle indexing more efficiently.
3. Overlooking Error Handling
In any integration, robust error handling is essential. Failing to manage exceptions can lead to data loss or corrupted indices.
Problem:
Ignoring potential issues while indexing:
IndexRequest request = new IndexRequest("my_index").source(doc);
client.index(request, RequestOptions.DEFAULT); // No error handling
Solution:
Implement comprehensive error handling:
try {
IndexRequest request = new IndexRequest("my_index").source(doc);
client.index(request, RequestOptions.DEFAULT);
} catch (ElasticsearchException e) {
LOG.error("Error indexing document: " + e.getMessage(), e);
} catch (IOException e) {
LOG.error("IO exception: " + e.getMessage(), e);
}
Error handling ensures that your application can respond appropriately to issues, making it more resilient.
4. Underestimating Performance Tuning
Elasticsearch provides several performance tuning options that, if neglected, can lead to subpar application performance.
Problem:
Not tuning Elasticsearch settings can affect query speed and resource utilization.
Solution:
Regularly monitor and adjust settings like shard size, number of replicas, and refresh intervals. Here is an example of how to adjust the refresh interval of an index:
UpdateSettingsRequest updateSettingsRequest = new UpdateSettingsRequest("my_index");
updateSettingsRequest.settings(Settings.builder()
.put("index.refresh_interval", "30s")); // Increase refresh interval
client.indices().putSettings(updateSettingsRequest, RequestOptions.DEFAULT);
Regular performance tuning can lead to major improvements in your application’s overall efficiency.
5. Failing to Utilize Query DSL
Elasticsearch's Query DSL (Domain Specific Language) offers a rich set of features for crafting optimized search queries. Overlooking this can lead to inefficient searches.
Problem:
Using standard queries without leveraging advanced capabilities.
Solution:
Take advantage of Query DSL when constructing queries:
SearchSourceBuilder searchSourceBuilder = new SearchSourceBuilder();
searchSourceBuilder.query(QueryBuilders.boolQuery()
.must(QueryBuilders.matchQuery("user", "kimchy"))
.filter(QueryBuilders.rangeQuery("timestamp")
.gte("2023-01-01")
.lt("2023-02-01")));
SearchRequest searchRequest = new SearchRequest("my_index");
searchRequest.source(searchSourceBuilder);
SearchResponse searchResponse = client.search(searchRequest, RequestOptions.DEFAULT);
Utilizing Query DSL enables more precise searches and optimized resource usage.
6. Not Considering Version Compatibility
As both Java libraries and Elasticsearch evolve, version mismatches can lead to unexpected bugs or broken features.
Problem:
Using an outdated Elasticsearch client with a newer version of the Elasticsearch server:
<dependency>
<groupId>org.elasticsearch.client</groupId>
<artifactId>elasticsearch-rest-high-level-client</artifactId>
<version>7.10.0</version> <!-- Potential version mismatch issue -->
</dependency>
Solution:
Always check compatibility when upgrading versions. Refer to the Elasticsearch Compatibility Matrix to find the correct client version corresponding to your server version.
To Wrap Things Up
Integrating Java with Elasticsearch can significantly enhance your application's performance and search capabilities. However, it is crucial to be aware of the common pitfalls that developers face in this process. By following best practices, such as defining mappings explicitly, utilizing bulk requests, implementing effective error handling, tuning performance, leveraging Query DSL, and maintaining version compatibility, you can avoid many common issues.
By taking the time to address these areas, you will not only improve the robustness of your application but also create a seamless experience for end-users. For further reading on making the most out of your Elasticsearch setup, refer to the official Elasticsearch documentation.
Feel free to reach out in the comments if you have additional questions or experiences to share regarding Java and Elasticsearch integration. Happy coding!
Checkout our other articles