Avoiding Common Pitfalls in Java REST APIs: Best Practices
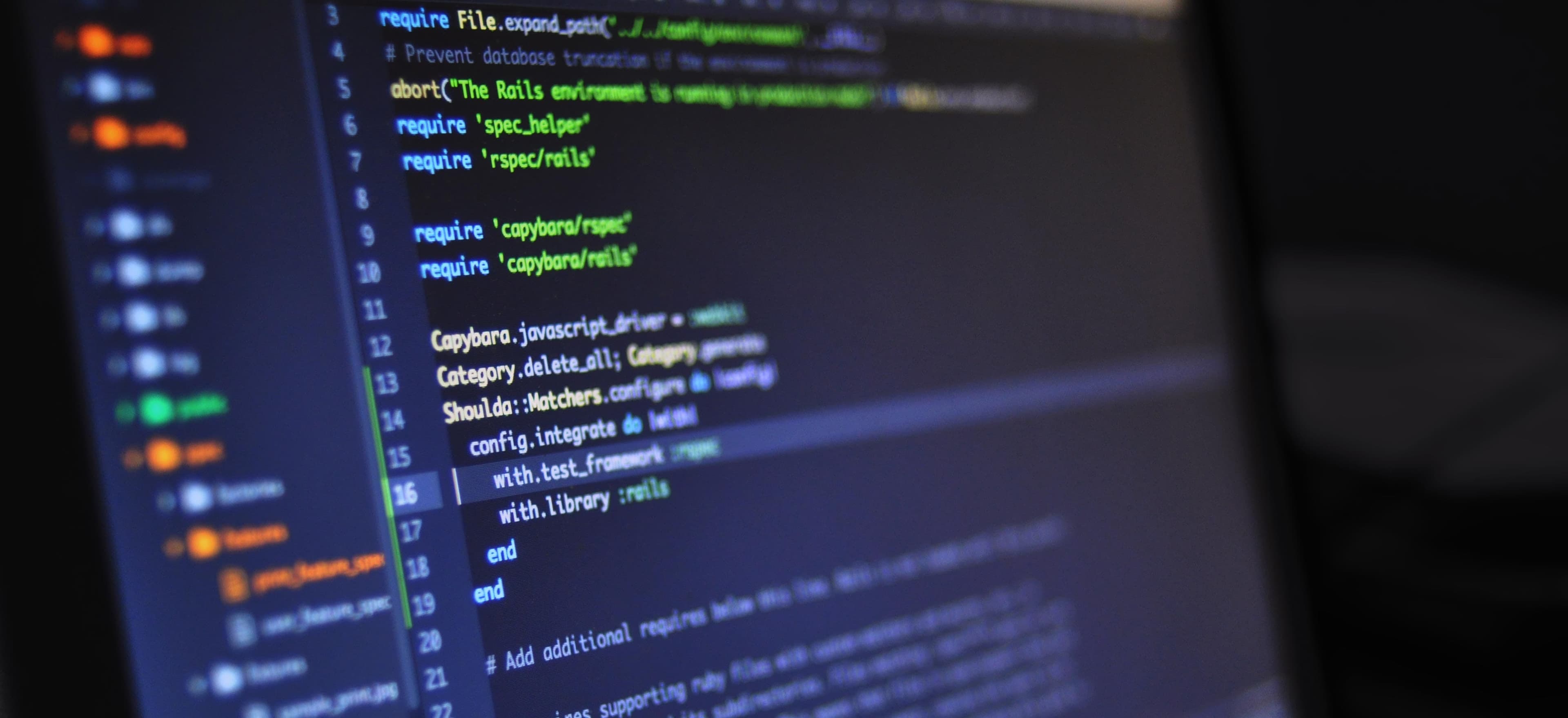
- Published on
Avoiding Common Pitfalls in Java REST APIs: Best Practices
In the rapidly evolving world of software development, crafting robust REST APIs using Java requires a well-thought-out approach. REST (Representational State Transfer) APIs are essential for communication between clients and servers, particularly for web and mobile applications. However, there are common pitfalls that developers encounter when designing these APIs. In this blog post, we will explore best practices for avoiding these pitfalls while ensuring the scalability, maintainability, and efficiency of your Java REST APIs.
Understanding REST Principles
Before diving into best practices, let's briefly review the fundamental principles of REST. REST APIs leverage standard HTTP methods to interact with resources represented in a structured format, typically JSON or XML. It follows several principles, including:
- Statelessness: Each API call must contain all the information the server needs to fulfill the request.
- Resource-based: REST APIs are centered around resources identified by URIs.
- Uniform Interface: The API should have a consistent structure.
These principles form the basis for best practices in API design. Now, let's look at how to avoid common pitfalls.
1. Use Meaningful Resource Naming
Pitfall: Inconsistent or Unclear Resource Naming
Naming resources inaccurately can lead to confusion and hinder the usability of your API.
Best Practice: Follow Naming Conventions
Your resource names should be intuitive and reflect the corresponding data entity. For instance, use plural nouns for collections, and a singular noun for an individual resource.
// Good resource naming
@GetMapping("/users") // Returns a list of users
public List<User> getAllUsers() {
return userService.findAll();
}
@GetMapping("/users/{id}") // Returns a single user based on ID
public User getUserById(@PathVariable Long id) {
return userService.findById(id);
}
In this example, the resource names /users
and /users/{id}
accurately convey their purpose. This clarity benefits both developers and API consumers.
2. Implement Proper HTTP Status Codes
Pitfall: Incorrect Use of HTTP Status Codes
Using incorrect HTTP status codes can confuse clients and diminish the effectiveness of error handling.
Best Practice: Use Status Codes Appropriately
HTTP status codes communicate the outcome of a client's request. Here are some common codes you should use:
- 200 OK: The request was successful.
- 201 Created: A new resource was successfully created.
- 204 No Content: The request was successful, but there's no content to return.
- 400 Bad Request: The client sent an invalid request.
- 404 Not Found: The requested resource does not exist.
- 500 Internal Server Error: An unexpected error occurred on the server.
Here's how to implement proper status codes:
@PostMapping("/users")
public ResponseEntity<User> createUser(@RequestBody User user) {
User createdUser = userService.create(user);
return new ResponseEntity<>(createdUser, HttpStatus.CREATED);
}
In this snippet, we use the HttpStatus.CREATED
to indicate that a new user has been created successfully. This clarity is essential for clients handling responses.
3. Validate Input Data
Pitfall: Neglecting Input Validation
Failing to validate input can lead to errors, security vulnerabilities, and inconsistent states in your application.
Best Practice: Implement Strong Validation Logic
Always validate incoming data before processing it. You can use Java's Bean Validation API for this purpose.
@PostMapping("/users")
public ResponseEntity<User> createUser(@Valid @RequestBody User user) {
User createdUser = userService.create(user);
return new ResponseEntity<>(createdUser, HttpStatus.CREATED);
}
In this code, the @Valid
annotation ensures that the incoming user data adheres to predefined validation rules. This practice not only improves security but also enhances data integrity.
4. Documentation and API Versioning
Pitfall: Lack of Proper Documentation and Versioning
Many developers overlook the significance of clear documentation and version control for their APIs. This oversight can lead to confusion among users and compatibility issues.
Best Practice: Use OpenAPI Specification and Version Your APIs
Document your API using the OpenAPI Specification (formerly known as Swagger). This will provide a clear reference for users and help with onboarding new developers.
Additionally, always version your APIs to maintain backward compatibility. This can be implemented in the URI or through request headers.
Here’s an example:
@GetMapping("/api/v1/users")
public List<User> getAllUsersV1() {
return userService.findAll();
}
@GetMapping("/api/v2/users")
public List<UserDTO> getAllUsersV2() {
return userService.findAllUsersDto();
}
In this example, we have two versions of the getAllUsers
endpoint. This allows clients to continue using their existing integrations while you deploy improvements to newer versions of the API.
For more details about API documentation, visit OpenAPI Specification.
5. Implement Rate Limiting
Pitfall: Not Considering Rate Limit
Ignoring rate limiting can overwhelm your backend services during high traffic, causing degraded performance or service outages.
Best Practice: Use Rate Limiting Techniques
Implement rate limiting mechanisms, such as token buckets or leaky buckets, to control the number of API requests a client can make in a specific timeframe. This can be accomplished using tools like Spring Cloud Gateway.
Here is a snippet implementing rate limiting using Spring:
@RestController
@RequestMapping("/api/users")
public class UserController {
@GetMapping("/profile")
@RateLimiter(value = "userProfile", limitForPeriod = 5, limitForIP = 3)
public User getUserProfile() {
// Method implementation here.
}
}
In this example, the @RateLimiter
annotation restricts the number of times a user can access the getUserProfile
method within a specific time window. Utilizing rate limiting is crucial for providing a reliable API experience.
Wrapping Up
Designing effective Java REST APIs necessitates careful planning and adherence to best practices to avoid common pitfalls. From clear resource naming to appropriate use of HTTP status codes, robust input validation, thorough documentation, or implementing rate limiting, these practices are critical. Following these guidelines ensures your APIs are user-friendly, secure, and reliable.
For further reading on building REST APIs in Java, check out Java Brains for insightful tutorials.
By implementing these best practices, you'll elevate your REST API development—creating an API that's not only functional but also elegant and robust. Happy coding!