Mastering Pagination and Sorting in Java: Common Pitfalls
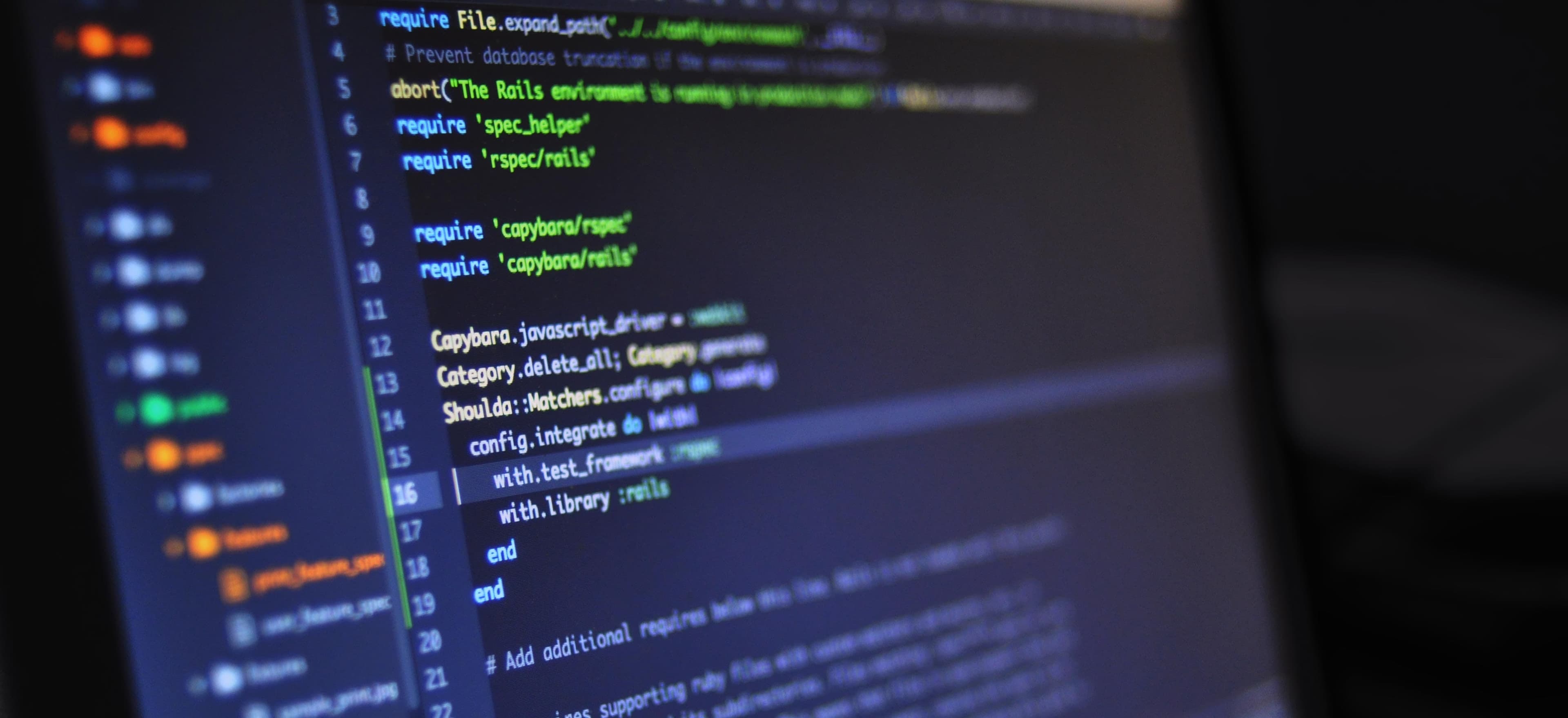
- Published on
Mastering Pagination and Sorting in Java: Common Pitfalls
When developing applications, especially those that deal with vast datasets, pagination and sorting become crucial features. They not only enhance user experience by displaying a manageable subset of data but also significantly improve application performance. However, implementing these features in Java can present multiple pitfalls. This guide dives deep into the common challenges developers face with pagination and sorting and sheds light on effective solutions.
Understanding Pagination
Pagination is the process of dividing a large dataset into smaller, manageable chunks. Imagine reading a book; it wouldn’t be efficient to read all the pages at once. A well-designed pagination strategy allows users to navigate through pages seamlessly.
Key Benefits of Pagination
- Improved UI Navigation: Users can browse data more intuitively.
- Optimized Loading Time: It reduces the amount of data rendered on the screen.
- Enhanced Performance: The application can handle large data sets more effectively.
Implementing Pagination in Java
There are various ways to implement pagination in Java. We'll explore a simple example that demonstrates how to paginate results from a list.
Example Code: Basic Pagination
Here’s a straightforward Java example that shows how to paginate a list of strings:
import java.util.ArrayList;
import java.util.List;
public class PaginationExample {
public static List<String> paginate(List<String> items, int pageNumber, int pageSize) {
if (items == null || items.isEmpty()) {
return new ArrayList<>();
}
int fromIndex = (pageNumber - 1) * pageSize;
if (fromIndex >= items.size()) {
return new ArrayList<>();
}
int toIndex = Math.min(fromIndex + pageSize, items.size());
return items.subList(fromIndex, toIndex);
}
public static void main(String[] args) {
List<String> items = new ArrayList<>();
for (int i = 1; i <= 100; i++) {
items.add("Item " + i);
}
int pageNumber = 2; // Second page
int pageSize = 10; // 10 items per page
List<String> paginatedItems = paginate(items, pageNumber, pageSize);
// Display the paginated results
for (String item : paginatedItems) {
System.out.println(item);
}
}
}
What the Code Does
- Calculate Indices: The code calculates the starting and ending indices for the requested page.
- Extract Sublist: It uses the
subList
method to fetch the relevant items. - Handles Edge Cases: Includes checks for empty lists and invalid page requests.
Common Pitfalls in Pagination
- Negative or Zero Page Numbers: Failure to handle invalid page number requests can lead to index out-of-bound exceptions.
- Off-by-One Errors: Miscalculating the start or end indices can result in data loss or display errors.
- Large Datasets: Exploding memory usage when dealing with very large datasets can be problematic. Always consider implementing server-side pagination.
Sorting Data Efficiently
Sorting complements pagination beautifully. It allows users to view data in a specific order, enhancing the overall usability and aesthetics of the output.
Example Code: Sorting a List
Here’s a simple example demonstrating how to sort a list of strings alphabetically:
import java.util.ArrayList;
import java.util.Collections;
import java.util.Comparator;
import java.util.List;
public class SortingExample {
public static List<String> sortItems(List<String> items) {
Collections.sort(items);
return items;
}
public static void main(String[] args) {
List<String> items = new ArrayList<>();
items.add("Banana");
items.add("Apple");
items.add("Orange");
items.add("Grapefruit");
List<String> sortedItems = sortItems(items);
// Display the sorted results
for (String item : sortedItems) {
System.out.println(item);
}
}
}
Understanding the Code
- Using Collections.sort(): This method provides a simple and efficient way to sort lists without manually implementing sorting algorithms.
- In-place Sorting: The original list is modified, making this approach memory efficient.
Common Pitfalls in Sorting
- Performance of Sorting Algorithms: Be aware that different sorting algorithms have varying time complexities. For example, using Bubble Sort on large datasets is inefficient.
- Not Handling Case Sensitivity: Sorting strings without considering case sensitivity can yield misleading results. Utilize
String.CASE_INSENSITIVE_ORDER
to mitigate this. - Mutating Original Data: If the order of the original list is crucial, consider making a copy of it before sorting.
Combining Pagination and Sorting
To provide a seamless user experience, pagination and sorting should work hand-in-hand. It helps to implement sorting prior to pagination to ensure that users see sorted data displayed page by page.
Example Code: Pagination and Sorting Together
Here's how you could implement both features together:
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
public class PaginatedSortedExample {
public static void main(String[] args) {
List<String> items = new ArrayList<>();
for (int i = 1; i <= 100; i++) {
items.add("Item " + i);
}
// Sort items
Collections.sort(items);
// Paginate items
int pageNumber = 2; // Second page
int pageSize = 10; // 10 items per page
List<String> paginatedItems = paginate(items, pageNumber, pageSize);
// Display the sorted paginated results
for (String item : paginatedItems) {
System.out.println(item);
}
}
public static List<String> paginate(List<String> items, int pageNumber, int pageSize) {
if (items == null || items.isEmpty()) {
return new ArrayList<>();
}
int fromIndex = (pageNumber - 1) * pageSize;
if (fromIndex >= items.size()) {
return new ArrayList<>();
}
int toIndex = Math.min(fromIndex + pageSize, items.size());
return items.subList(fromIndex, toIndex);
}
}
Key Aspects to Note
- Efficiency Gains: By combining sorting and pagination, you reduce the number of computations within user requests.
- Interactivity: Users can expect consistent output based on their sorting preferences while navigating through paginated results.
Bringing It All Together
Mastering pagination and sorting in Java is vital for developing user-friendly applications. Understanding the common pitfalls allows you to mitigate risks and enhance the efficiency of your implementation. Whether you're working with lists or databases, using thoughtful practices ensures that your data rendering is both seamless and efficient.
For a deeper understanding, consider checking out the Java Documentation or resources like GeeksforGeeks that provide extensive discussions on collections and data manipulation in Java.
Happy coding!