Conquering Java's OutOfMemoryError: PermGen Space Explained
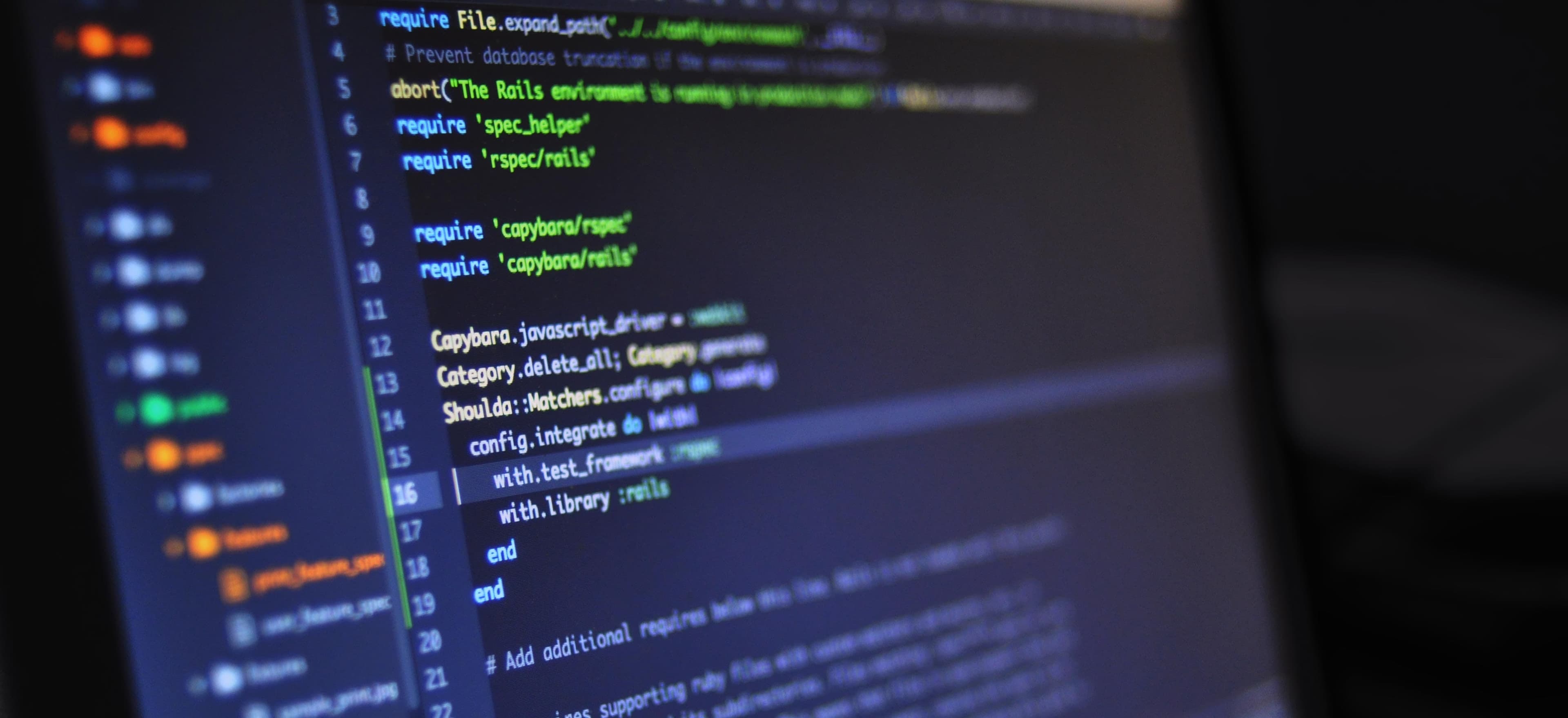
- Published on
Conquering Java's OutOfMemoryError: PermGen Space Explained
Java is renowned for its robustness, portability, and ease of use. However, developers often encounter issues, one of the more notorious being the OutOfMemoryError
, specifically related to Permanent Generation (PermGen) space. In this blog post, we will delve deep into PermGen space, the causes of OutOfMemoryError
related to it, and how to mitigate these issues effectively.
What is PermGen Space?
In Java, memory management is critical for application performance and resource utilization. Java heap memory is divided into several regions, one of which is the Permanent Generation (PermGen). This area is distinct as it holds metadata describing the classes and methods used by the Java application.
Key Characteristics of PermGen Space:
- Storage of Class Metadata: Java dynamically loads classes, and their metadata is stored in this space.
- Not Resizable: Unlike the heap memory, the size of PermGen is fixed, which can lead to complications if your application requires more classes than it can accommodate.
Starting from Java 8, the PermGen space was removed and replaced by Metaspace, which utilizes native memory and allows for dynamic resizing. Nevertheless, understanding the traditional PermGen space remains critical for legacy applications running on older versions.
What Causes OutOfMemoryError: PermGen Space?
The OutOfMemoryError
related to PermGen space typically arises when the applications attempt to load more classes than the allocated memory can support. Here are a few common causes:
- Excessive Class Loading: Applications that use frameworks such as Spring or Hibernate can lead to numerous classes being loaded into PermGen.
- Classloader Leaks: Common in web applications where classloaders are not released properly, causing memory to be consumed continuously.
- High Number of JSPs: Each JSP is compiled into a class, leading to an explosion in class counts especially on large-scale applications.
Symptoms of PermGen Issues
Developers might see symptoms such as:
- Application crashes with
java.lang.OutOfMemoryError: PermGen space
messages. - Slow application performance as the garbage collection (GC) tries to clean up memory.
Calculating Adequate PermGen Size
Before addressing how to fix PermGen-related issues, it’s important to understand how to set the size accurately. You can configure PermGen space with the following JVM options:
-XX:PermSize=<initial_size> -XX:MaxPermSize=<max_size>
Example:
For example, if you want to allocate an initial size of 128 MB and a maximum of 256 MB, you can do it as follows:
java -XX:PermSize=128m -XX:MaxPermSize=256m -jar your-app.jar
Why Increase PermGen Space?
You might wonder why you should consider increasing PermGen space rather than resolving the underlying issues. Right-sizing the PermGen can serve as a temporary workaround to problems arising from frequent deployments or heavy class-loading scenarios without jumping into a code refactor immediately.
Best Practices to Prevent PermGen Issues
- Identify Unused Classes: Use tools such as VisualVM or Eclipse Memory Analyzer to inspect the PermGen region and see what's being loaded unnecessarily.
- Proper Classloader Management: Ensure that classes loaded by custom classloaders are properly unloaded by managing visibility and scope.
- Limit the Number of JSPs: In web applications, reducing the number of JSP files can minimize class loading.
- Upgrade to Java 8 or Above: If you are still using versions prior to Java 8, consider upgrading. This move not only removes concerns related to PermGen but also brings performance improvements and other enhancements.
Example: Using VisualVM to Monitor PermGen Usage
When troubleshooting PermGen-related issues, utilizing monitoring tools becomes paramount. VisualVM is a free tool that can assist you in analyzing various aspects of your Java application. Start your application with the following command to enable monitoring:
java -Dcom.sun.management.jmxremote -jar your-app.jar
Then, launch VisualVM and connect to your Java application. Look for the "Memory" tab, and you will find detailed information regarding your PermGen space usage.
Code Snippet: Implementing a Custom ClassLoader
If you need to implement your ClassLoader and ensure that classes are cleaned up properly, here’s a simple example:
public class CustomClassLoader extends ClassLoader {
@Override
protected Class<?> findClass(String name) throws ClassNotFoundException {
// Custom logic to load classes
byte[] b = ...; // Load class bytes here
return defineClass(name, b, 0, b.length);
}
// Method to ensure unloading of classes
public void clearCache() {
// Clear references held by your class loader
// Implementing based on your application’s architecture
}
}
Explanation:
- This
CustomClassLoader
overrides thefindClass
method. - Implement a method
clearCache()
to manage the lifecycle of loaded classes and references effectively. This is crucial in applications that need to dynamically load or reload classes, helping avoid memory leaks.
Bringing It All Together
Understanding OutOfMemoryError: PermGen space
is indispensable for Java developers, particularly when managing large applications or dealing with legacy systems. By correctly configuring the PermGen space, implementing best practices, and leveraging monitoring tools, developers can ensure their Java applications run smoothly without memory issues. Finally, transitioning to Java 8 or later is highly encouraged for modern applications, emphasizing performance and memory management enhancements.
For additional reading on Java memory management, you can check out Java Performance: The Definitive Guide and Oracle's Java Documentation.
With this understanding, you are now equipped to address the challenges associated with PermGen space and optimize your Java applications accordingly. Happy coding!
Checkout our other articles