Mastering Synchronized Blocks to Prevent Deadlocks
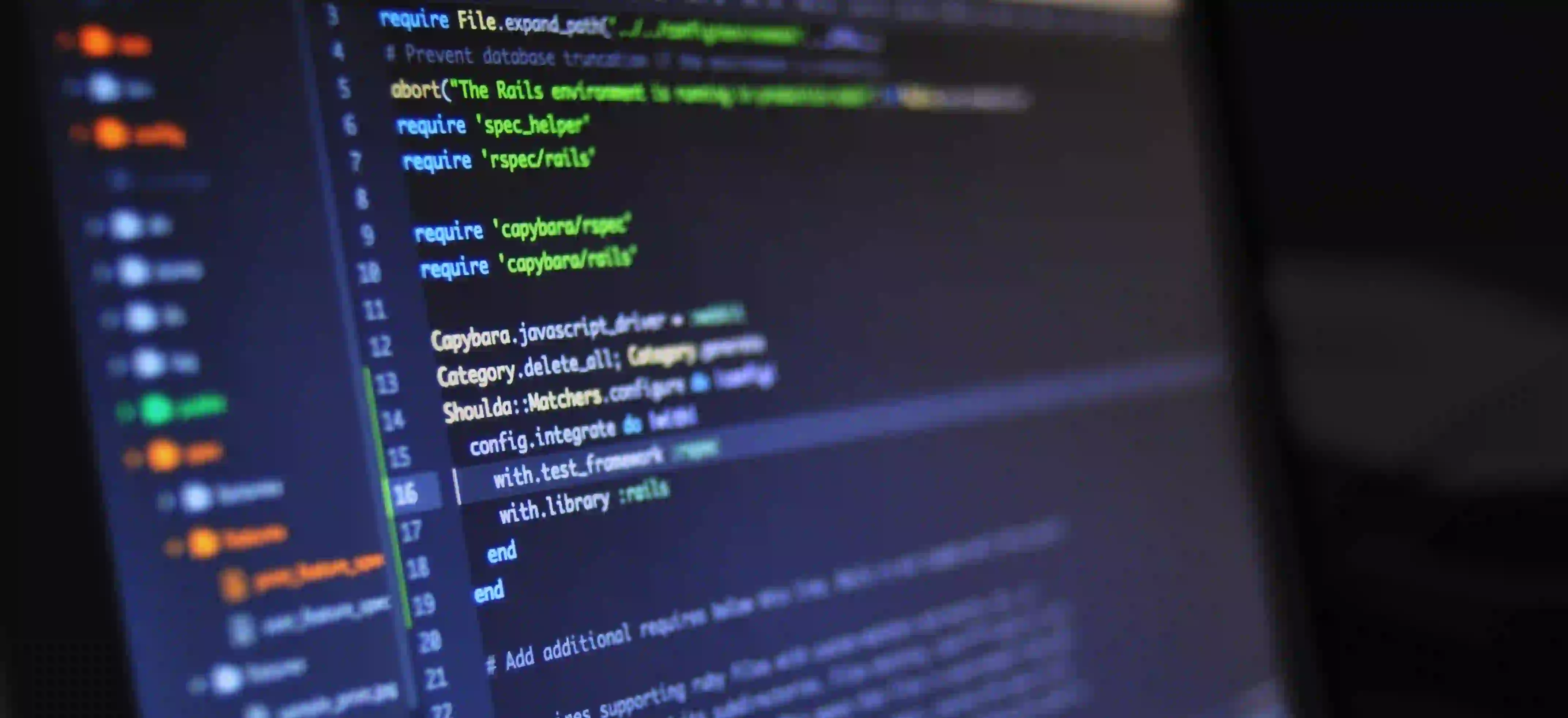
Mastering Synchronized Blocks to Prevent Deadlocks in Java
Java is a programming language that has a rich set of functionalities, especially when it comes to concurrent programming. With the power of multi-threading, developers can create efficient applications that perform multiple operations simultaneously. However, with greater power come greater risks. One of the most notorious issues in multi-threaded environments is the deadlock. In this blog post, we will dive deep into synchronized blocks, explore how they can be used effectively to prevent deadlocks, and demonstrate code practices through examples.
What is a Deadlock?
A deadlock occurs when two or more threads are blocked forever, each waiting for the other to release a lock. In simpler terms, a deadlock arises when two or more threads are paused indefinitely due to their mutual holding of resources. Understanding this is crucial to mastering synchronized blocks in Java.
Why is Deadlock a Concern?
Deadlocks can lead to severe application performance issues and unresponsiveness. In a system where threads are unable to make progress, not only are resources wasted, but the user experience can also be severely compromised.
Understanding Synchronized Blocks
In Java, a synchronized block is a mechanism that allows threads to safely access shared resources without interfering with each other. This is done by acquiring a lock on the object being synchronized.
Here’s the syntax for a synchronized block:
synchronized (object) {
// critical section
}
In this example, object
acts as the monitor lock. Once a thread enters the synchronized block, it locks the associated object, preventing other threads from entering any synchronized block that's locking on the same object.
The Importance of the 'Why'
Using synchronized blocks helps in maintaining data integrity while preventing data corruption in multi-threaded scenarios. However, improper use of synchronized blocks can lead to performance bottlenecks and, as mentioned, deadlocks.
Strategies to Prevent Deadlocks
To prevent deadlocks, developers should be aware of several key strategies:
-
Lock Ordering: Always acquire locks in a consistent order. If every thread agrees to lock resources in a sequential manner, it minimizes the risk of encountering circular waiting.
-
Using Timeouts: Regularly check for deadlocks by implementing lock timeouts. If a thread cannot acquire a lock within a specific timeframe, it should back off and retry later.
-
Minimizing Lock Scope: Keep the synchronized block as short as possible, release locks quickly, and avoid excessive locking.
Let's look deeper into each strategy with code examples.
Lock Ordering Example
Here's a simple illustration of lock ordering using synchronized blocks:
class Resource {
private final Object lock1 = new Object();
private final Object lock2 = new Object();
public void methodA() {
synchronized (lock1) {
System.out.println("Acquired lock1 in methodA.");
synchronized (lock2) {
System.out.println("Acquired lock2 in methodA.");
// perform actions
}
}
}
public void methodB() {
synchronized (lock1) {
System.out.println("Acquired lock1 in methodB.");
synchronized (lock2) {
System.out.println("Acquired lock2 in methodB.");
// perform actions
}
}
}
}
In this code, both methodA
and methodB
acquire lock1
and lock2
, ensuring a fixed order for all threads. This consistent acquisition pattern prevents circular waiting.
Using Timeouts
Here's how you can implement a timeout mechanism in a more robust setup:
import java.util.concurrent.TimeUnit;
import java.util.concurrent.locks.ReentrantLock;
class Resource {
private final ReentrantLock lock1 = new ReentrantLock();
private final ReentrantLock lock2 = new ReentrantLock();
public void methodA() {
try {
if (lock1.tryLock(1, TimeUnit.SECONDS)) {
try {
if (lock2.tryLock(1, TimeUnit.SECONDS)) {
try {
System.out.println("Locks acquired in methodA.");
// perform actions
} finally {
lock2.unlock();
}
} else {
System.out.println("Could not acquire lock2 in methodA, retrying.");
}
} finally {
lock1.unlock();
}
} else {
System.out.println("Could not acquire lock1 in methodA.");
}
} catch (InterruptedException e) {
Thread.currentThread().interrupt();
}
}
public void methodB() {
// similar implementation
}
}
In this example, tryLock
is used with timeouts to avoid indefinite blocking. If a lock cannot be acquired in the specified time, the method can handle this gracefully, potentially retrying or performing alternate logic.
Minimizing Lock Scope
Reduce the locking time span as much as possible. Here's how you can do it:
class Resource {
private final Object lock = new Object();
private int sharedCounter = 0;
public void incrementCounter() {
synchronized (lock) {
// Critical section begins.
sharedCounter++;
// Critical section ends.
}
}
public int getCounter() {
synchronized (lock) {
return sharedCounter;
}
}
}
In the above implementation, the critical section is minimized to just the increment operation and retrieval of the counter. This locks the object only for the duration necessary, reducing the chances of deadlock while allowing higher concurrency.
Wrapping Up
Mastering synchronized blocks is vital for Java developers dealing with multi-threading. By understanding the mechanics behind synchronization, the potential for deadlocks can be mitigated through practical strategies like lock ordering, using timeouts, and minimizing the scope of locks.
Moreover, you can further understand the intricacies of Java concurrency through resources like Java Concurrency in Practice and Oracle's Java Documentation on concurrent programming.
Use this knowledge to not only prevent deadlocks but also to ensure that your applications remain scalable and efficient in a multi-threaded environment. Happy coding!