Mastering Floating-Point Precision in Java Programming
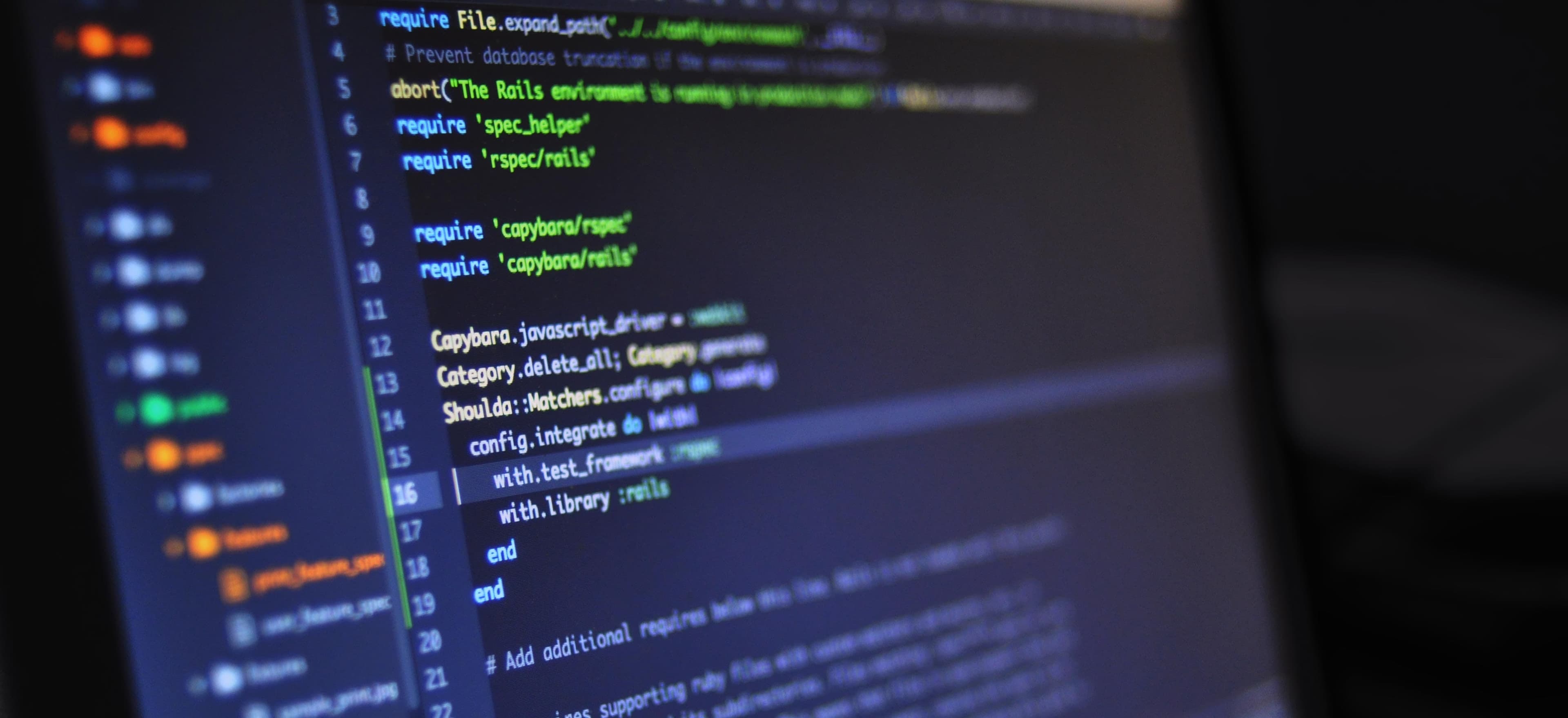
- Published on
Mastering Floating-Point Precision in Java Programming
Floating-point arithmetic is a crucial aspect of programming in Java, especially when dealing with real numbers and calculations that require precision. However, many developers encounter unexpected results when performing arithmetic operations with floating-point numbers. In this blog post, we will explore the intricacies of floating-point precision in Java, examining potential pitfalls and providing effective strategies to ensure accurate and reliable calculations.
Understanding Floating-Point Representation
Floating-point numbers are represented in a binary format that allows computers to handle very large or very small values efficiently. Java uses the IEEE 754 standard for floating-point arithmetic, which defines how numbers should be stored and manipulated. This standard includes two main primitive data types:
float
: A single-precision 32-bit IEEE 754 floating point.double
: A double-precision 64-bit IEEE 754 floating point.
Code Example: Basic Floating-Point Implementation
public class FloatingPointExample {
public static void main(String[] args) {
float floatValue = 0.1f; // Single-precision float
double doubleValue = 0.1; // Double-precision double
System.out.println("Float Value: " + floatValue);
System.out.println("Double Value: " + doubleValue);
}
}
In this code snippet, we define two variables, one as a float
and the other as a double
. Notice the "f" suffix for the float value. This is required to specify that the number should be treated as a float, not a double, which is the default.
The Issue with Precision
When performing arithmetic operations on floating-point numbers, unexpected results can occur due to their inability to represent certain decimal values exactly. For example:
public class PrecisionIssue {
public static void main(String[] args) {
double a = 0.1;
double b = 0.2;
double result = a + b;
System.out.println("Result of 0.1 + 0.2: " + result);
System.out.println("Is result equal to 0.3? " + (result == 0.3));
}
}
You might expect the output to indicate that 0.1 + 0.2 equals 0.3, but due to floating-point precision issues, it doesn't:
Result of 0.1 + 0.2: 0.30000000000000004
Is result equal to 0.3? false
The slight deviation from expected values can lead to problems, especially in financial applications where precision is critical.
Best Practices for Handling Floating-Point Precision
To mitigate issues related to floating-point precision, here are some best practices you can follow:
1. Use BigDecimal for Financial Calculations
For applications where accuracy is paramount, such as financial calculations, consider using BigDecimal
. This class provides operations for arithmetic, scale manipulation, and rounding without the corruption of precision that occurs with primitive data types.
Code Example: BigDecimal in Action
import java.math.BigDecimal;
public class BigDecimalExample {
public static void main(String[] args) {
BigDecimal a = new BigDecimal("0.1");
BigDecimal b = new BigDecimal("0.2");
BigDecimal sum = a.add(b);
System.out.println("Sum of 0.1 + 0.2 with BigDecimal: " + sum);
System.out.println("Is sum equal to 0.3? " + sum.equals(new BigDecimal("0.3")));
}
}
In the above code, BigDecimal
ensures that the addition operation maintains precision. We achieve a precise sum of 0.3 and can reliably check for equality.
2. Rounding and Scale Control
Another helpful technique is controlling rounding and scale in floating-point calculations. The BigDecimal
class allows you to specify rounding modes easily.
Code Example: Rounding with BigDecimal
import java.math.BigDecimal;
import java.math.RoundingMode;
public class RoundingExample {
public static void main(String[] args) {
BigDecimal value = new BigDecimal("2.555");
BigDecimal roundedValue = value.setScale(2, RoundingMode.HALF_UP);
System.out.println("Rounded Value: " + roundedValue);
}
}
In this example, we round 2.555 to two decimal places using the HALF_UP
rounding mode. This is important when dealing with currency or other forms of precision where a specific number of decimal places is required.
3. Avoid ==
for Floating-Point Comparison
When comparing floating-point values, avoid using the equality operator (==
). Instead, consider using a tolerance range to determine equality.
Code Example: Safe Comparison Method
public class SafeComparison {
private static final double EPSILON = 1e-10;
public static void main(String[] args) {
double a = 0.1 + 0.2;
double b = 0.3;
System.out.println("Are a and b approximately equal? " + approximatelyEqual(a, b));
}
public static boolean approximatelyEqual(double x, double y) {
return Math.abs(x - y) < EPSILON;
}
}
In this code snippet, we define a method approximatelyEqual
that compares two floating-point numbers within a defined tolerance (EPSILON
). This method helps to avoid issues arising from rounding errors and precision problems.
4. Stick with Primitive, Except When Needed
For most purposes, especially in scientific computations or applications not sensitive to precision, using float
and double
is entirely adequate. However, be mindful of your application’s needs and switch to BigDecimal
if high precision is required.
Key Takeaways
Mastering floating-point precision in Java programming is essential for delivering reliable applications. With proper understanding and application of these best practices, you can avoid common pitfalls and ensure that your calculations are accurate.
By using the BigDecimal
class for critical applications, rounding modes for control, careful comparisons, and understanding when to choose floats or doubles, you equip yourself with the tools necessary for mastering precision in Java.
For further reading on floating-point arithmetic, consider exploring the following resources:
May your floating-point calculations always be precise!
Checkout our other articles