Avoiding Common Pitfalls in Java Serialization
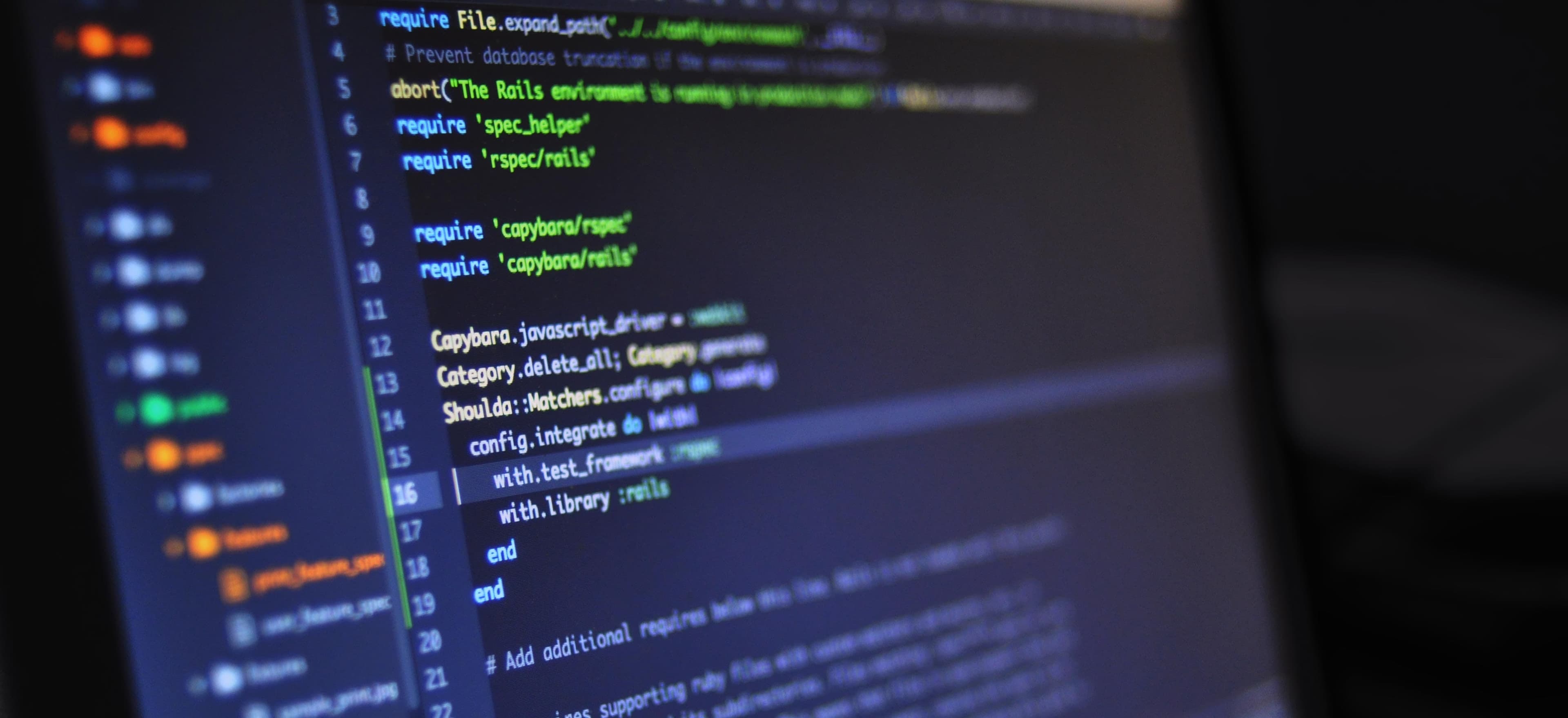
- Published on
Avoiding Common Pitfalls in Java Serialization
Java serialization is a crucial concept in the Java programming language, allowing developers to convert objects into a byte stream for storage or transmission. This might sound straightforward, but many pitfalls can lead to unexpected behavior, performance issues, or security vulnerabilities. In this blog post, we will delve into some of these common pitfalls and discuss how to avoid them effectively.
What is Serialization?
Serialization in Java is the process of converting an object into a sequence of bytes. This is particularly useful when you want to store the object in a file, send it over a network, or save it to a database.
Java offers built-in serialization mechanisms via the java.io.Serializable
interface. Here is a simple example:
import java.io.Serializable;
public class User implements Serializable {
private String username;
private String password; // Sensitive information
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Getters and other methods
}
In this example, the User
class implements Serializable
, marking it as eligible for serialization. However, we need to consider how serialization handles sensitive data, versioning, and object relationships.
Common Pitfalls and How to Avoid Them
1. Ignoring the serialVersionUID
Every time a class is modified, its default serialVersionUID may change. If you have serialized objects and later change the class structure—like adding a new field or modifying a method—you risk running into InvalidClassException
.
To avoid this, declare an explicit serialVersionUID
in your class:
public class User implements Serializable {
private static final long serialVersionUID = 1L;
private String username;
private String password;
// Constructor and other methods
}
Why? This ensures that your versioning remains consistent, even with modifications, thus safeguarding the serialized data's integrity.
2. Storing Sensitive Information
As seen in our User
class example, sensitive data like passwords should never be serialized directly. When an object is serialized, anyone with access to the byte stream can read sensitive information.
To secure sensitive data, you can mark fields as transient
:
public class User implements Serializable {
private String username;
private transient String password; // Will not be serialized
public User(String username, String password) {
this.username = username;
this.password = password;
}
// Getters and other methods that handle password securely
}
Why? Marking the password as transient
ensures that it is not included during serialization, reducing the risk of exposure.
3. Using Non-Serializable Fields
If your class contains fields of non-serializable types, attempting to serialize the class will throw a NotSerializableException
. Always ensure that all fields in a serializable class are either primitive types or types that implement Serializable
.
import java.io.Serializable;
public class User implements Serializable {
private String username;
private transient NonSerializableObject nonSerializableField; // Causes issues
// Constructor and other methods
}
Solution: Either make sure to mark these fields as transient or replace them with a serializable counterpart.
4. Circular References
Java serialization does manage circular references well, but debugging issues arising from them can still be complex. When objects reference each other, you can end up with an infinite cycle. Be cautious and consider breaking cycles in your object relationships.
How to Avoid: Consider using a custom serialization method:
private void writeObject(java.io.ObjectOutputStream out) throws IOException {
out.defaultWriteObject(); // Serialize default fields
out.writeObject(someOtherObject); // Serialize only required fields
}
Why? This ensures control over what you are serializing and can help to mitigate issues.
5. Serialization Performance
While serialization is necessary, it can introduce performance overhead if not managed properly—especially for large objects or complex object graphs. Always test the performance implications of serialization in your application.
Tip: Use efficient serialization frameworks, such as Kryo or Google's Protocol Buffers, which are designed for speed and compactness. Here is a basic example using Kryo:
Kryo kryo = new Kryo();
Output output = new Output(new FileOutputStream("user.dat"));
kryo.writeObject(output, user);
output.close();
Why? These frameworks can significantly improve serialization speed while also reducing the size of the serialized data.
6. Version Compatibility
Over time, classes may evolve. If an object's structure changes, it's essential to provide compatibility with old and new versions of objects.
Implement readObject
to maintain version control:
private void readObject(java.io.ObjectInputStream in) throws IOException, ClassNotFoundException {
in.defaultReadObject();
// Handle legacy structures
}
Why? Customizing how the object is read back allows for seamless transitions and less headache when dealing with multiple versions of your classes.
7. Testing Serialization
When implementing serialization, rigorous testing is crucial. Create unit tests to verify that the serialized objects can be accurately serialized and deserialized.
Here is an example of how to test serialization:
import java.io.*;
public class UserSerializationTest {
@Test
public void testSerialization() throws IOException, ClassNotFoundException {
User user = new User("john_doe", "secret_password");
// Serialize
ByteArrayOutputStream baos = new ByteArrayOutputStream();
ObjectOutputStream oos = new ObjectOutputStream(baos);
oos.writeObject(user);
oos.close();
// Deserialize
ByteArrayInputStream bais = new ByteArrayInputStream(baos.toByteArray());
ObjectInputStream ois = new ObjectInputStream(bais);
User deserializedUser = (User) ois.readObject();
ois.close();
// Assert equality
assertEquals(user.getUsername(), deserializedUser.getUsername());
// Exclude password comparison as it's transient now.
}
}
Why? Validating serialization during development ensures that future changes to the class won’t break serialization, thereby safeguarding against regression.
A Final Look
Serialization is a powerful feature in Java, but its misuse can lead to serious pitfalls. By avoiding common mistakes such as neglecting serialVersionUID
, serializing sensitive information, or failing to test your implementations, you can ensure your Java applications are robust and secure.
By paying attention to these pitfalls and utilizing the best practices discussed in this article, you will improve your handling of serialization in Java. For further reading, consult the Java Serialization Specification, which covers these topics in greater depth.
Happy coding!
Checkout our other articles