Mastering Time Zones in Java: Common Pitfalls to Avoid
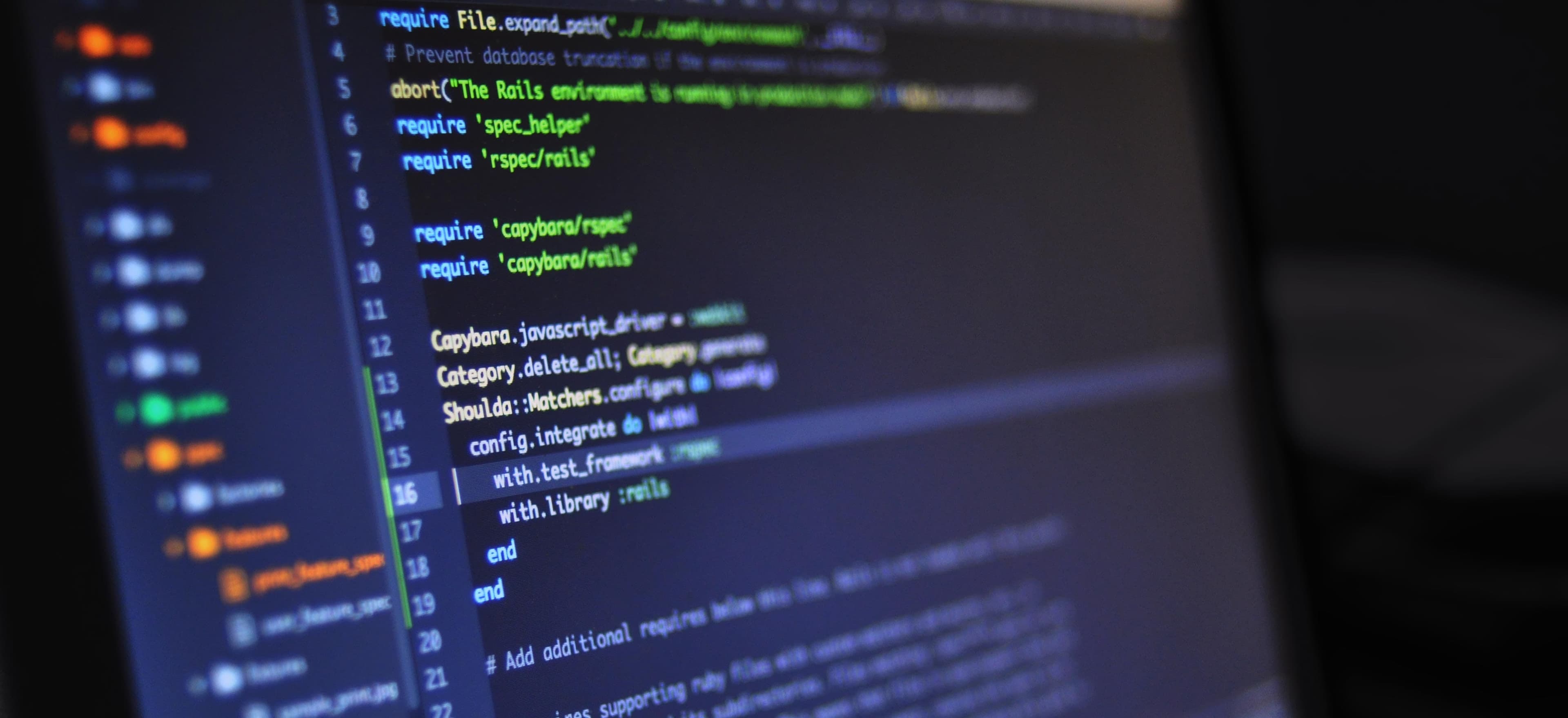
- Published on
Mastering Time Zones in Java: Common Pitfalls to Avoid
When it comes to dealing with time zones in Java, many developers find themselves navigating through a web of complexity. Improper handling not only leads to incorrect application behavior but can also negatively impact user experience or application logic. This blog post aims to cover common pitfalls encountered with time zones in Java, providing you with the insights necessary to master this crucial aspect of programming.
The Complexity of Time Zones
Time zones are affected by myriad factors such as daylight saving time (DST), geopolitical changes, and cultural conventions. As a result, dealing with time zones is not as straightforward as it might seem. Below, we explore various scenarios where programmers commonly falter, and how to handle these cases effectively.
Common Pitfalls
1. Misunderstanding Date
vs. Calendar
In Java, the Date
and Calendar
classes were instrumental in handling date and time until Java 8, when the new java.time
package was introduced. However, many developers continue to use Calendar
, resulting in confusion surrounding time zones.
For example:
import java.util.Calendar;
import java.util.TimeZone;
Calendar calendar = Calendar.getInstance(TimeZone.getTimeZone("America/New_York"));
calendar.set(2023, Calendar.OCTOBER, 30, 12, 0); // October 30, 12:00 PM
System.out.println(calendar.getTime());
Here, you're setting a specific date and time in the Eastern Time Zone, but Calendar
itself is mutable and prone to errors. This can lead to incorrect assumptions about time zone behavior, particularly concerning DST transitions.
The Solution: Use the java.time
API.
Here's how you can achieve the same using the new ZonedDateTime
class:
import java.time.ZonedDateTime;
import java.time.ZoneId;
ZonedDateTime zonedDateTime = ZonedDateTime.of(2023, 10, 30, 12, 0, 0, 0, ZoneId.of("America/New_York"));
System.out.println(zonedDateTime);
This approach is not only cleaner but also less prone to errors. The ZonedDateTime
class automatically handles DST transitions for you.
2. Ignoring Daylight Saving Time
One of the trickiest aspects of working with time zones is daylight saving time. Many developers forget that not all regions observe DST, and those that do can start and end it on different dates.
ZonedDateTime startOfDST = ZonedDateTime.of(2023, 3, 12, 2, 0, 0, 0, ZoneId.of("America/New_York"));
ZonedDateTime endOfDST = ZonedDateTime.of(2023, 11, 5, 2, 0, 0, 0, ZoneId.of("America/New_York"));
System.out.println("Start of DST: " + startOfDST);
System.out.println("End of DST: " + endOfDST);
Output:
Start of DST: 2023-03-12T02:00:00-05:00[America/New_York]
End of DST: 2023-11-05T02:00:00-04:00[America/New_York]
Notice how the time reflects the change after DST starts and ends. Failing to account for these vital changes can lead to misleading information.
Recommendation: When dealing with user input, always convert dates to UTC for storage. Upon retrieval, convert back to the user’s time zone, ensuring accurate representation.
3. Using String Representation for Time Zone Data
It’s tempting to simply use string representations of dates and times when working with time zones. However, string formats are error-prone and can lead to parsing exceptions.
import java.time.ZonedDateTime;
import java.time.format.DateTimeFormatter;
String dateString = "2023-10-30T12:00:00-04:00[America/New_York]";
ZonedDateTime zonedDateTime = ZonedDateTime.parse(dateString);
System.out.println(zonedDateTime);
Verifying the source of your string is paramount. If the string originates from user input or an unreliable source, the format can easily lead to misinterpretations.
Best Practice: Always validate and standardize date strings before processing them. Use the DateTimeFormatter
class for consistent parsing.
4. Converting Between Time Zones
Another common mistake occurs when converting dates between time zones. Many developers mistakenly think that simply changing the time zone of a ZonedDateTime
instance will provide the correct time.
Consider this scenario:
ZonedDateTime originalTime = ZonedDateTime.now(ZoneId.of("America/New_York"));
ZonedDateTime convertedTime = originalTime.withZoneSameInstant(ZoneId.of("Europe/London"));
System.out.println(originalTime);
System.out.println(convertedTime);
This provides precise conversion while accounting for DST. However, using withZoneSameLocal
would lead to incorrect hour representation, as it doesn’t adjust for time offset.
Quick Tip: Always utilize withZoneSameInstant
instead of withZoneSameLocal
.
Additional Resources for Mastering Java Time Zones
To further enhance your knowledge of managing time zones, consider these resources:
- Java Time Zone documentation on the Oracle website.
- The comprehensive guide on Java 8's new date and time API from Baeldung.
The Closing Argument
Mastering time zones in Java is paramount for creating robust applications that function properly across various regions and circumstances. By recognizing the pitfalls discussed in this article, you equip yourself with strategies to avoid common mistakes. Remember to leverage modern Java features, utilize proper APIs, and stay updated on related resources to further hone your skills.
Navigating through time zones can be a daunting task, but with thoughtful approaches, calculating complexities can turn into manageable parameters of your Java applications. Here’s to writing error-free, timezone-sensitive code!
Checkout our other articles