Mastering Memory Management to Prevent Java Leaks
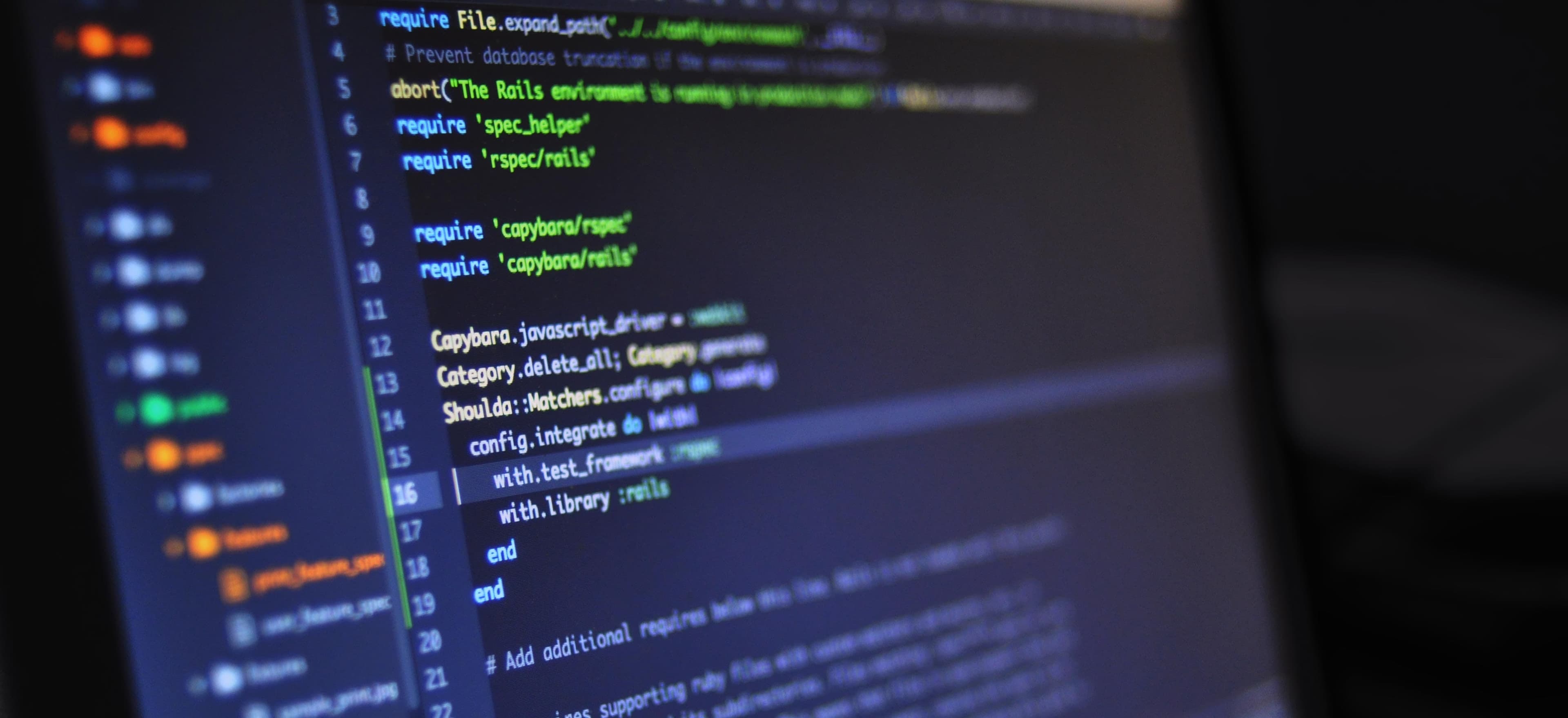
- Published on
Mastering Memory Management to Prevent Java Leaks
Java, a cornerstone of modern programming, excels at memory management through its robust garbage collection mechanisms. However, even the most sophisticated systems can falter. Memory leaks in Java can lead to performance degradation and application crashes. In this blog post, we will delve into memory management, examine techniques for preventing leaks, and explore the tools available to help us maintain efficient usage of memory.
Understanding Memory Management in Java
Java manages memory through a combination of heap and stack memory allocation. The heap is where Java objects are stored, while the stack holds method references and local variables. The garbage collector steps in to automatically reclaim memory that is no longer in use.
Why Garbage Collection Matters
Garbage collection is crucial because it:
- Reduces developer workload: Automatic memory management means fewer memory-related bugs.
- Simplifies code: Developers can focus on application logic rather than memory allocation and deallocation.
However, even with garbage collection, memory leaks can occur if references to objects are maintained longer than necessary. These leaks can result in OutOfMemoryError, hindering application performance.
Common Causes of Memory Leaks
Understanding where and how memory leaks occur is fundamental to effective prevention. Below are some common causes of memory leaks in Java:
-
Static References
Static fields remain in memory for the lifetime of the application. If engaged with large objects or collections, they can prevent them from being garbage collected.public class StaticReferenceExample { private static List<String> staticList = new ArrayList<>(); public static void addToList(String item) { staticList.add(item); } }
Here,
staticList
retains all added items until the application terminates, leading to potential leaks. -
Unbounded Caches
Using caches without eviction policies can lead to memory overload.private Map<Integer, String> cache = new HashMap<>(); public void cacheData(Integer key, String value) { cache.put(key, value); }
As items accumulate, the cache can expand indefinitely, consuming precious memory.
-
Listeners and Callbacks
If Listener or Callback interfaces are not managed properly, they can hold references to their listeners, preventing them from being garbage collected.public class EventSource { private List<EventListener> listeners = new ArrayList<>(); public void addListener(EventListener listener) { listeners.add(listener); } }
If listeners are not removed after they are no longer needed, they will persist, stemming memory leaks.
Techniques to Prevent Memory Leaks
1. Use Weak References
Weak references allow the garbage collector to reclaim memory more aggressively. If only weak references exist to an object, it can be garbage collected even if the program is still running.
import java.lang.ref.WeakReference;
public class WeakReferenceExample {
private WeakReference<Object> weakRef;
public void storeObject(Object obj) {
weakRef = new WeakReference<>(obj);
}
public Object getObject() {
return weakRef.get(); // Return null if the object has been garbage collected
}
}
Use WeakReference
for caches or collections where you can afford to lose data, preventing memory leaks by allowing garbage collection when memory is tight.
2. Clean Up Resources
Always clean up references when an object is no longer needed. This means explicitly setting references to null
or removing event listeners.
public class ResourceCleaner {
private List<EventListener> listeners = new ArrayList<>();
public void removeListener(EventListener listener) {
listeners.remove(listener);
}
public void clearListeners() {
listeners.clear(); // Ensures listeners can be garbage collected
}
}
Make cleanup a habit within your code to avoid inadvertent leaks.
3. Use Soft References for Caching
Soft references are like weak references but are retained longer—until memory runs low. This makes them suitable for caching use cases.
import java.lang.ref.SoftReference;
public class SoftReferenceExample {
private SoftReference<List<String>> softList;
public List<String> getCachedData() {
List<String> list = softList != null ? softList.get() : null;
if (list == null) {
list = new ArrayList<>();
softList = new SoftReference<>(list); // Cache the new list
}
return list;
}
}
By utilizing soft references, we achieve a balance between memory savings and performance.
Tools and Libraries for Memory Leak Detection
Utilizing tools to identify memory-related issues is paramount. Here are some highly effective options:
1. VisualVM
VisualVM is a powerful tool that provides insights into Java applications. You can monitor memory usage, thread activity, and inspect heap dumps to identify memory leaks. Heap dump analysis is particularly critical as it showcases object allocation, reference trees, and more.
2. Eclipse MAT (Memory Analyzer Tool)
Eclipse MAT is another robust tool for analyzing memory leaks. It can process heap dumps to provide insights into memory consumption and retention paths, helping identify which objects are consuming memory in your application.
3. JProfiler
JProfiler offers profiling features that include memory leak detection. It integrates seamlessly with Java applications and provides statistics related to object allocation, allowing you to trace memory usage.
My Closing Thoughts on the Matter
Mastering memory management in Java is essential for developing robust applications. By understanding common pitfalls and employing techniques to prevent memory leaks—like using weak and soft references, cleaning up resources carefully, and leveraging debugging tools—developers can maintain efficient memory usage.
Take charge of your Java applications today, ensuring that they run smoothly without the burden of memory leaks. Want to deep dive further? Explore Java's Garbage Collection for a more comprehensive understanding of how memory management operates within various JVM options.
By mastering memory management, you can significantly improve the performance and reliability of your Java applications. Remember, a proactive approach is always better than a reactive one in the realms of memory and performance management.
Checkout our other articles