Mastering Recursion: Preventing StackOverflowErrors
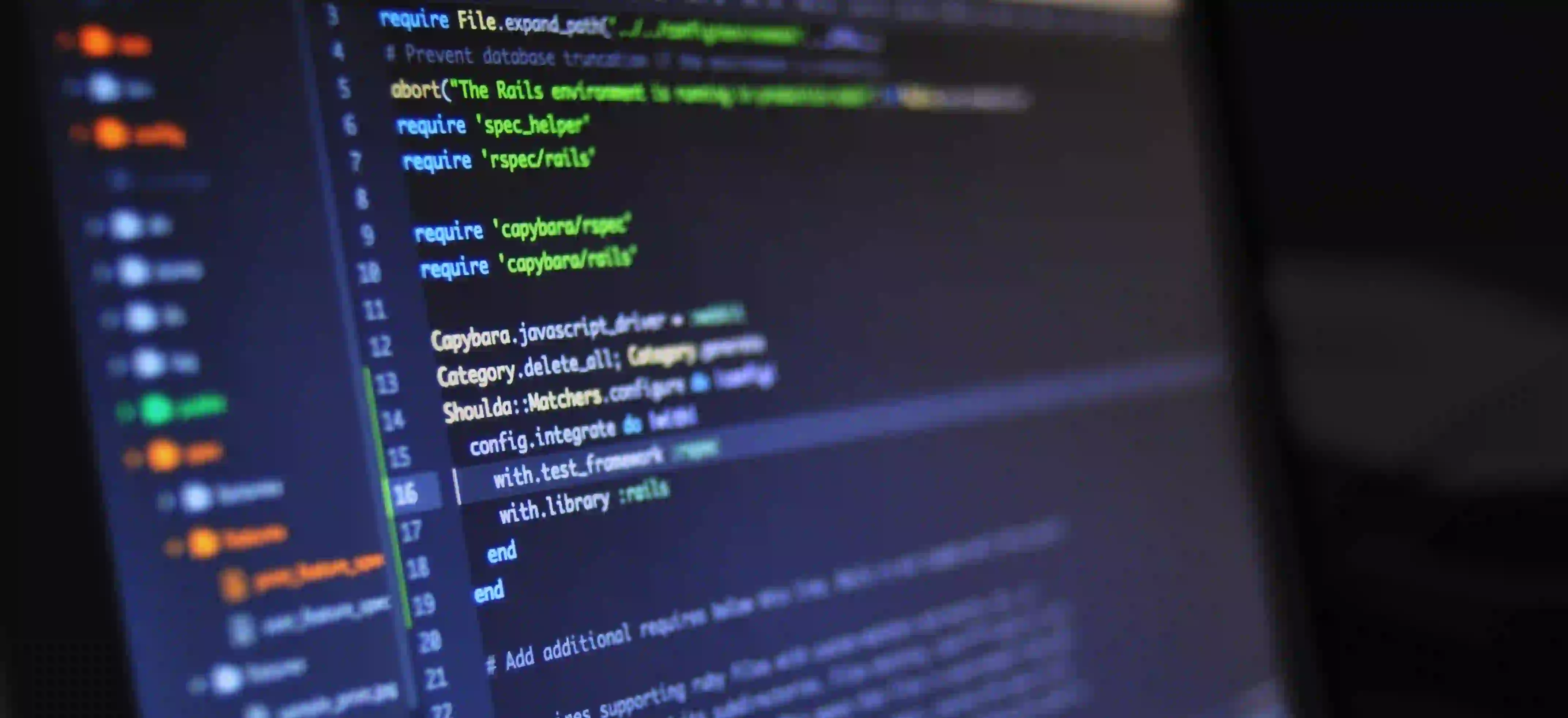
Mastering Recursion: Preventing StackOverflowErrors
Recursion is an elegant programming technique that simplifies complex problems. By having a function call itself with a modified set of parameters, we can often express our logic in a much clearer way than with iterative solutions. However, recursion comes with its own challenges. One of the most common issues developers face is the dreaded StackOverflowError
. In this post, we’ll explore what recursion is, why it can lead to a stack overflow, and how to prevent it.
Understanding Recursion
Recursion occurs when a function calls itself, creating a new frame on the call stack for each invocation. This process will continue until a base case is met, which is the condition that stops the recursion. Here’s a simple example:
public class Factorial {
public static int factorial(int n) {
if (n == 0) { // Base case
return 1;
}
return n * factorial(n - 1); // Recursive call
}
public static void main(String[] args) {
System.out.println(factorial(5)); // Output: 120
}
}
Breakdown of the Code
- Base Case: The
if (n == 0)
condition stops the recursion when it reaches the base case. - Recursive Call: The function calls itself with
n - 1
. This creates a series of calls until the base case is reached.
This code beautifully illustrates how recursion can simplify the computation of a factorial. However, calling factorial(5)
will lead to a series of nested function calls: factorial(5)
, factorial(4)
, down to factorial(0)
. At each level, memory is consumed on the stack.
What is StackOverflowError?
A StackOverflowError
occurs when the call stack limit is exceeded. This typically happens when:
- There's no base case, or the base case is never met.
- The recursion goes too deep, exceeding the stack limit set by the JVM (Java Virtual Machine).
Here’s an example that leads to a StackOverflowError
:
public class InfiniteRecursion {
public static void recurse() {
// This call never ends
recurse();
}
public static void main(String[] args) {
recurse(); // This will throw StackOverflowError
}
}
This simple recursive function doesn't have a terminating condition, resulting in an infinite loop through recursive calls. As the call stack fills up, we eventually run out of memory to store new calls.
Preventing StackOverflowErrors
1. Define a Clear Base Case
The first rule in recursion is to ensure that a base case is defined. Without a clear condition to terminate the recursion, the function will run indefinitely.
public class Fibonacci {
public static int fibonacci(int n) {
if (n <= 1) { // Base cases
return n;
}
return fibonacci(n - 1) + fibonacci(n - 2); // Recursive calls
}
public static void main(String[] args) {
System.out.println(fibonacci(5)); // Output: 5
}
}
2. Optimize with Tail Recursion
Tail recursion occurs when a recursive call is the last operation in a function. While Java does not inherently optimize tail recursion like some other languages (e.g., Scala), restructuring your recursive function into a tail-recursive format can sometimes help clarify logic and avoid deep recursion.
Here’s an example of a tail-recursive version to calculate factorial:
public class TailRecursion {
public static int tailFactorial(int n, int accumulator) {
if (n == 0) return accumulator; // Base case
return tailFactorial(n - 1, n * accumulator); // Tail recursive call
}
public static void main(String[] args) {
System.out.println(tailFactorial(5, 1)); // Output: 120
}
}
3. Using Iterative Solutions
In certain scenarios, especially when working with deeply recursive problems, modifying your approach to use iteration can avoid the pitfalls of stack overflow altogether. For the Fibonacci number calculation, consider the iterative version below:
public class IterativeFibonacci {
public static int fibonacci(int n) {
if (n <= 1) return n;
int a = 0;
int b = 1;
int fib = 0;
for (int i = 2; i <= n; i++) {
fib = a + b;
a = b;
b = fib;
}
return fib;
}
public static void main(String[] args) {
System.out.println(fibonacci(5)); // Output: 5
}
}
4. Analyzing Depth and Stack Size
To prevent StackOverflowErrors
, always be aware of the maximum depth your recursive functions can reach. The JVM has a set stack size (which can be configured). Monitor your recursive algorithms and assess whether the expected depth could exceed this limit.
Utilizing visualizations or performance monitoring tools can assist in identifying potential problem areas in your recursive functions.
To Wrap Things Up
Recursion can significantly simplify problem-solving when used correctly. However, it is crucial to recognize and mitigate StackOverflowErrors. By defining clear base cases, optimizing with tail recursion, considering iterative solutions, and analyzing stack depth, you can write efficient recursive functions in Java.
For further reading on recursion and optimization techniques, check out GeeksforGeeks and Oracle's Documentation on Java.
With practice and a solid understanding of the concepts discussed, you'll be well on your way to mastering recursion while keeping StackOverflowErrors at bay!