Overcoming Java Type Erasure: Tips for Effective Generics
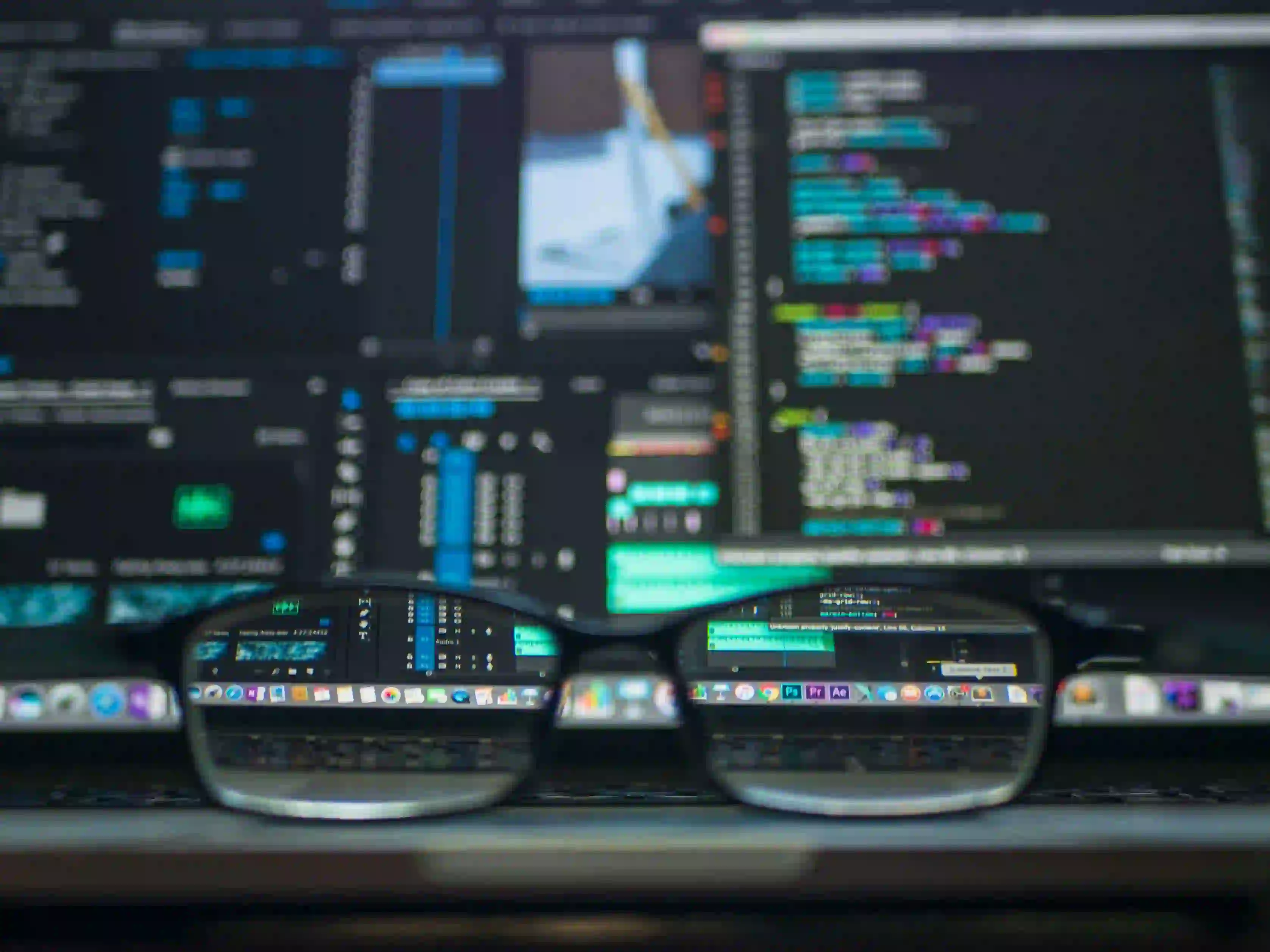
Overcoming Java Type Erasure: Tips for Effective Generics
Java generics provide a powerful way to create classes, interfaces, and methods with a placeholder for the type of data they operate on. This concept allows for type safety at compile time, which leads to fewer runtime errors. However, one of the most intricate aspects of Java generics is type erasure. In this post, we will dive deep into what type erasure is and provide effective techniques to manage and overcome its limitations while still leveraging the benefits of generics in Java.
What is Type Erasure?
Type Erasure is a process in Java where generic type information is removed (or "erased") during compilation. This means that the generic parameters are replaced with their bounds or Object
if no bounds are specified. The fundamental reason for type erasure is to maintain backward compatibility with older versions of Java, which didn’t support generics.
For example, consider the following generic class declaration:
class Box<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
During compilation, the Box
class effectively transforms into:
class Box {
private Object item;
public void setItem(Object item) {
this.item = item;
}
public Object getItem() {
return item;
}
}
Implications of Type Erasure
While type erasure simplifies the implementation of generics and ensures backward compatibility, it does present some challenges:
-
No Runtime Type Information: After type erasure, the generic type information is not available at runtime, leading to limitations when you want to perform type checks or instantiate generics.
-
Restrictions on Generics: You cannot create instances of a type parameter, which limits some operations such as creating arrays of a generic type.
-
Raw Types: Using generics as raw types (e.g.,
Box
instead ofBox<String>
) can lead to unsafe type operations and warnings.
Tips for Effective Use of Generics
To successfully navigate type erasure and leverage the full power of generics in Java, consider the following strategies:
1. Use Bounded Type Parameters
Bounded type parameters enhance the flexibility of your code. By specifying an upper bound, you ensure that the generic type has certain characteristics or behaviors.
Here is an example:
class NumberBox<T extends Number> {
private T number;
public NumberBox(T number) {
this.number = number;
}
public double doubleValue() {
return number.doubleValue();
}
}
In this example, T
must extend Number
, which gives direct access to the methods defined in the Number
class. This means that you can use T
as if it's a Number
object, providing additional safety and functionality. By explicitly defining the bounds, you maintain type safety and prevent runtime errors.
2. Utilize Wildcards
Wildcards in generics are helpful when you want to work with unknown types. They allow for more flexible code. For example, the syntax ? extends T
allows methods to accept any subclass of T
, while ? super T
permits the acceptance of any superclass.
Here is a code snippet demonstrating wildcards:
import java.util.List;
public class WildcardExample {
public void printNumbers(List<? extends Number> numbers) {
for (Number number : numbers) {
System.out.println(number);
}
}
}
In this case, the method printNumbers
can accept a list of any subclass of Number
, such as Integer
or Double
. This is particularly beneficial when you don’t need to know the exact type, just that it behaves like a Number
.
3. Use Generic Methods
Sometimes, the best way to manage type erasure is to add generics at the method level rather than the class level. This makes your method more versatile without the overhead of applying generics across the entire class.
Consider the following method:
public class GenericMethodExample {
public <T> void printArray(T[] array) {
for (T element : array) {
System.out.println(element);
}
}
}
Here, the method printArray
is generic, allowing you to pass in arrays of any type. This is a clean way to ensure type safety while working with various kinds of data.
4. Create a Factory Method for Generic Types
When creating instances of a generic type during run-time, consider using factory methods. These methods allow controlled instantiation while retaining type safety.
class Factory {
public static <T> Box<T> createBox() {
return new Box<>();
}
}
5. Use Collections Carefully
Due to type erasure, the collection's method of adding or retrieving elements can be tricky. When using collections, make sure to declare the type properly and avoid raw types.
For instance:
List<String> list = new ArrayList<>();
list.add("Hello");
String str = list.get(0); // No cast needed
By explicitly declaring the type in List<String>
, you ensure compile-time safety and avoid unnecessary casts.
6. Documentation and Comments
Given the complexity of generics, it's crucial to document your code clearly. Describe the intended use of generic types and provide examples of typical usage cases. This approach will help others (and your future self) understand the purpose and limitations of the code.
The Bottom Line
Java generics can be incredibly powerful, enabling more dynamic and safe code practices. However, the challenges posed by type erasure require developers to adapt their approach to utilize generics effectively. By following these tips and understanding how generics work under the hood, you can create more robust Java applications.
For further reading on Java generics and a deeper understanding of type erasure, refer to the official Java documentation on Generics. Additionally, the Java Language Specification provides a comprehensive look at the intricacies of the language features.
Embrace the power of generics—not only for cleaner code but also for avoiding errors down the line. Understanding type erasure is just the first step. Implementing these recommended strategies will help you write better Java applications that are both efficient and maintainable. Happy coding!