How to Fix Java OutOfMemoryError: Metaspace Issues
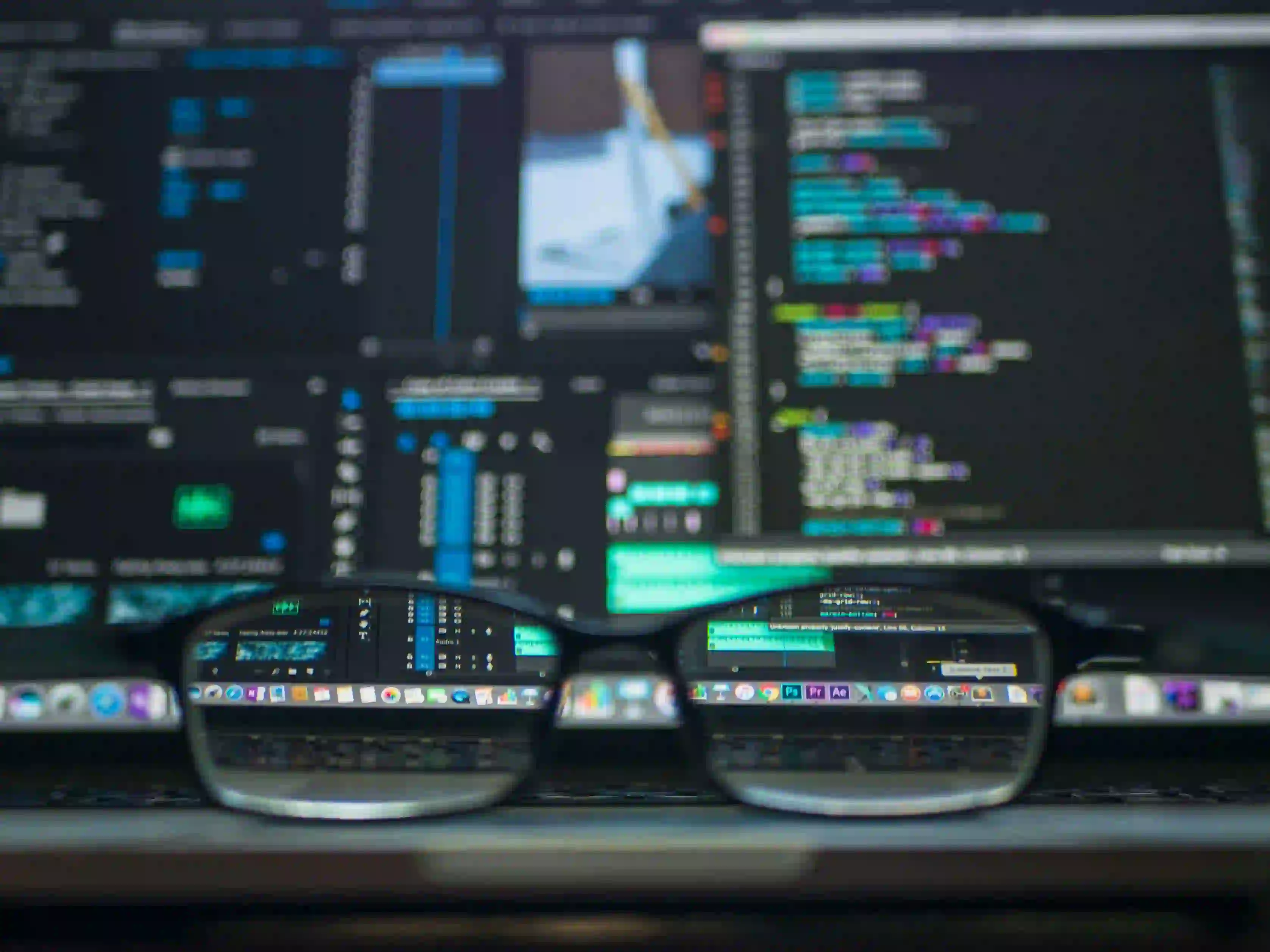
How to Fix Java OutOfMemoryError: Metaspace Issues
Java developers often find themselves facing a variety of challenges, one of which is the dreaded OutOfMemoryError
. A specific variant of this error known as Metaspace
can be particularly troublesome, especially in applications that dynamically load a large number of classes at runtime. In this blog post, we will explore what Metaspace
is, why it might lead to OutOfMemoryError
, and how you can effectively troubleshoot and resolve these issues.
Understanding Metaspace
In Java 8 and later versions, the Java Virtual Machine (JVM) replaced the PermGen space with Metaspace. This change allowed for increased flexibility regarding class metadata storage. Metaspace is allocated in native memory, unlike PermGen, which was limited to a fixed size in heap memory.
However, even though Metaspace size is theoretically unlimited, it can still run out when your application dynamically loads too many classes, leading to an OutOfMemoryError
.
Key Factors Influencing Metaspace Usage
- Classloader Behavior: Each classloader maintains its own space in Metaspace. Inefficient classloader hierarchies, like those created by a heavily modular architecture, can inflate Metaspace consumption.
- Dynamic Class Loading: Applications that use frameworks for dynamic class loading, like Hibernate or Spring, usually load a large number of classes at runtime.
- Memory Leaks: If classes remain referenced by caches or other static variables, they cannot be garbage-collected, causing memory leaks.
Symptoms of Metaspace Issues
When your application faces Metaspace-related problems, you might notice one or several of the following symptoms:
- Frequent instances of
java.lang.OutOfMemoryError: Metaspace
- Gradual increase in memory usage over time
- Sluggish application performance
Diagnosing the Problem
Before diving into potential solutions, it is essential to diagnose the issue accurately. Here’s how to do that:
1. Enable JVM Logging
You can enable the JVM logging to monitor Metaspace usage. Use the following parameters when starting your Java application:
-javaagent:agent.jar -XX:+PrintGCDetails -XX:+PrintGCTimeStamps -XX:MetaspaceSize=128m -XX:MaxMetaspaceSize=512m
These flags will help you track memory allocation and identify when the OutOfMemoryError
occurs.
2. Analyze Heap Dumps
You can create a heap dump when the error occurs using the command:
jmap -heap <pid>
This dump provides a snapshot of memory usage, allowing you to analyze which classes are consuming the most Metaspace. You can use tools like VisualVM or Eclipse MAT (Memory Analyzer Tool) to analyze the dump.
3. Identify Leaks and Bottlenecks
By analyzing the heap dump, you can determine if there are any memory leaks that are holding onto class metadata. Focus on any instances of classes that should be garbage-collected but are still retained.
Solutions for Metaspace Issues
After gathering diagnostic information, you can proceed with the following solutions:
1. Increase Metaspace Size
The easiest and quickest solution is to increase the Metaspace size through JVM options. You may specify the initial and maximum sizes like so:
-XX:MetaspaceSize=128m -XX:MaxMetaspaceSize=1024m
MetaspaceSize
: This defines the initial size of the Metaspace.MaxMetaspaceSize
: This sets the maximum limit, preventing the system from exhausting native memory.
Increasing the size will help your application run longer without running into memory issues. However, it is crucial to monitor the situation, as this is not a sustainable long-term solution.
2. Optimize Classloading
Ensure that you are not creating unnecessary classloader instances. Use singleton patterns or follow the ServiceLoader pattern effectively to manage shared classes.
Here's a recommended classloader pattern for shared resources:
public class SharedClassLoader extends ClassLoader {
private static final SharedClassLoader instance = new SharedClassLoader();
private SharedClassLoader() {
// Private constructor to restrict instantiation
}
public static SharedClassLoader getInstance() {
return instance;
}
}
3. Clean Up Unused Classes
Implement cleanup mechanisms for unused classes, especially when using frameworks that allow you to load classes dynamically. For instance, ensure you are not retaining references to classes in static caches unnecessarily.
4. Profile Your Application
For long-term performance management, regularly profile your application to identify memory-intensive operations and classloading patterns. Tools like YourKit or JProfiler can assist in real-time monitoring and analysis.
5. Upgrade Libraries and Frameworks
Using outdated libraries may introduce memory leak issues. Check if you are using the latest versions of frameworks like Spring, Hibernate, or others, as updates often include fixes for known memory management issues.
Wrapping Up
Dealing with OutOfMemoryError: Metaspace
can be a frustrating experience, especially in Java applications with dynamic classloading. By understanding and diagnosing the factors contributing to Metaspace usage, you can take proactive steps to mitigate these issues effectively.
To recap:
- Monitor and analyze Metaspace usage.
- Increase Metaspace size as a temporary measure.
- Optimize class loading practices.
- Regularly profile your application for memory leaks.
- Keep your libraries up-to-date.
With a diligent approach and the right tools, you can ensure that your Java applications run smoothly without falling victim to memory-related pitfalls.
For further reading, consider checking out resources such as the Java Performance Tuning Guide and the Java Garbage Collection Documentation. Happy coding!