How to Upgrade to Lettuce 5 Without Breaking Your App
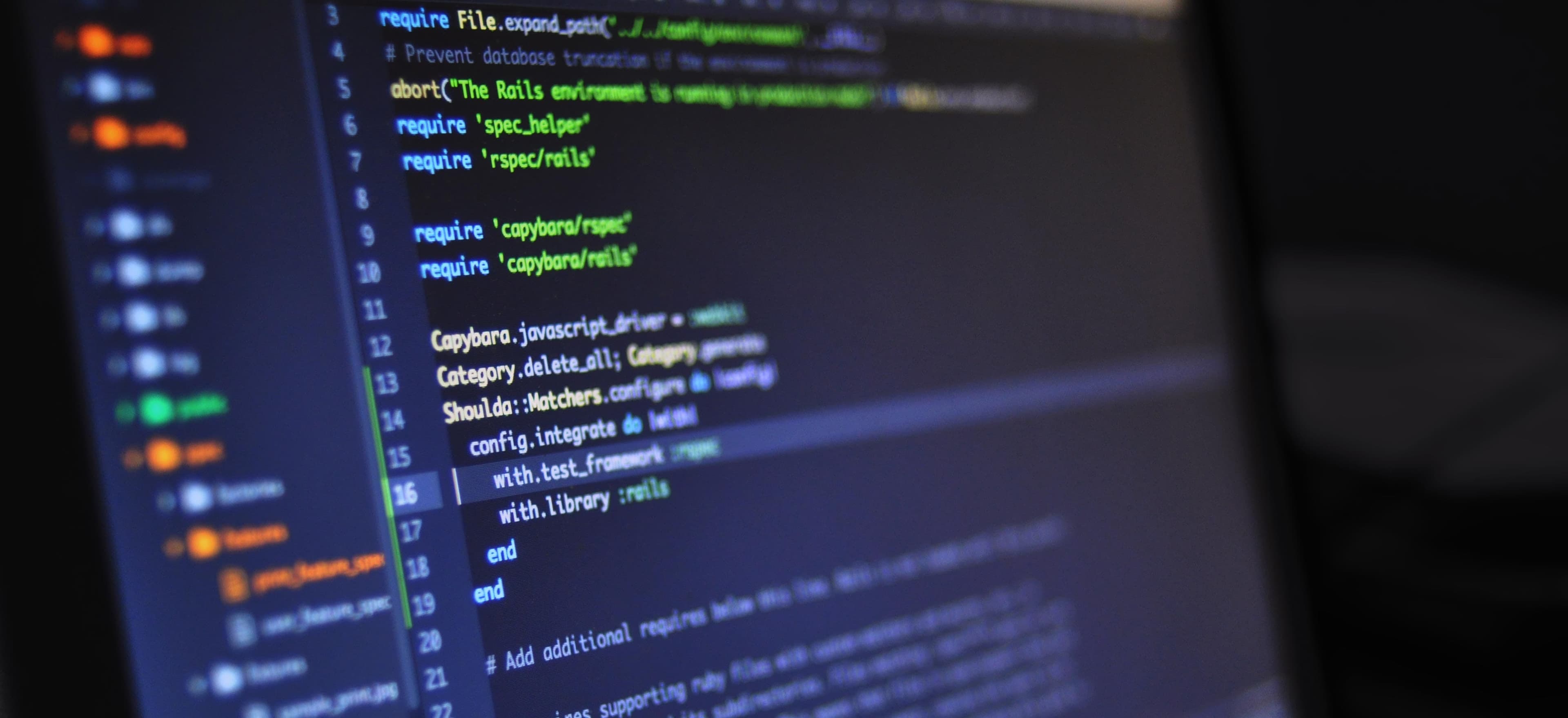
- Published on
How to Upgrade to Lettuce 5 Without Breaking Your App
If your application relies on Redis as its caching layer or data store, chances are that you’re using the Lettuce library. As of October 2023, Lettuce released version 5, introducing several enhancements and optimizations that can significantly improve performance and usability. However, upgrading from previous versions might feel daunting, especially if you have a large codebase that depends on prior Lettuce API functionalities. In this blog post, we will walk through how to upgrade to Lettuce 5 without breaking your application, ensuring a smooth transition.
Why Upgrade to Lettuce 5?
- Performance Improvements: Version 5 introduces an optimized connection handling mechanism that reduces latency in communication with Redis server.
- Reactive Support: It enhances reactive programming, making it easier to build responsive applications.
- New Features: Features like better support for Redis Streams, transactions, and Lua scripting were added.
- Bug Fixes and Optimizations: Continuous improvements for existing functionalities lead to a more stable development experience.
Prerequisites
Make sure you have the current version of Lettuce and later releases in your build configuration. If you're using Maven, your pom.xml
file should include the following dependency for Lettuce 5:
<dependency>
<groupId>io.lettuce.core</groupId>
<artifactId>lettuce-core</artifactId>
<version>5.x.x</version>
</dependency>
Replace "5.x.x" with the latest release version number.
Step 1: Backup Your Current Code
Before initiating the upgrade, back up your current codebase. This practice is crucial, as it allows you to revert to a stable version if anything goes awry during the upgrade process. Use version control systems like Git, and create a new branch dedicated to the upgrade process.
Step 2: Review Breaking Changes
Understanding breaking changes is key to a smooth transition. Notably, Lettuce 5 introduces several API changes. Here are a few notable modifications:
- Connection Handling: The connection API may have slight syntax changes.
- Reactive API Changes: If you are utilizing reactive programming, some method names and signatures have been updated.
- Removed Deprecated Methods: Ensure that you are not using any methods that were marked as deprecated in earlier versions.
For an exhaustive list, refer to the Lettuce documentation for version 5 changes.
Step 3: Update Your Code
Connection Changes
One of the most notable changes is how connections are established. Here’s an example of how to create a connection in Lettuce 5:
Old Syntax (Lettuce 4.x):
RedisClient redisClient = RedisClient.create("redis://localhost:6379");
RedisConnection<String, String> connection = redisClient.connect();
New Syntax (Lettuce 5.x):
RedisClient redisClient = RedisClient.create(RedisURI.create("redis://localhost:6379"));
RedisConnection<String, String> connection = redisClient.connect();
Why the Change?
The upgrade enhances uniformity and expands the configurability of your Redis connections. The new RedisURI
class allows you to set more advanced options, such as authentication or timeouts.
Step 4: Test Features In Use
Utilize the Streaming API
Lettuce 5 offers improved support for Redis Streams, making it easier to work with data in a streaming context. If you are migrating from earlier versions, here’s a simple example of using streams in Lettuce 5:
// Creating Stream
RedisCommands<String, String> commands = connection.sync();
commands.xadd("mystream", "key1", "value1");
// Reading from Stream
List<MapRecord<String, String, String>> records = commands.xread(StreamOffset.latest("mystream"));
records.forEach(record -> {
System.out.println(record.getId() + ": " + record.getValue());
});
Why This Matters?
Utilizing streams allows for processing data in real-time, a key asset for modern applications. This feature is increasingly becoming a standard for data management, and adopting it now prepares your app for future scalability.
Step 5: Utilize Reactive Programming
Lettuce 5 enhances support for Java's reactive programming model. If you are moving towards a more reactive architecture, consider using this improved functionality:
ReactiveRedisClient client = ReactiveRedisClient.create(RedisURI.create("redis://localhost:6379"));
Mono<String> value = client.connect().flatMap(connection -> connection.sync().get("myKey"));
Why Consider This?
Reactive programming improves resource efficiency, especially for applications requiring numerous I/O operations. It also enables more intuitive code handling regarding asynchronous operations.
Step 6: Conduct Thorough Testing
Testing is pivotal throughout this upgrade. After making code changes, it is essential to:
- Run Unit Tests: Ensure that your unit tests pass. Address any failing tests that may arise due to the new changes.
- Performance Testing: Measure the performance of your application post-upgrade to quantify the improvements and identify any regressions.
- End-to-End Testing: Validate the entire application flow, ensuring all features that interact with Redis are functioning correctly.
Step 7: Monitor After Deployment
After deploying the upgraded version, monitor your application for any runtime issues or performance discrepancies. Use tools like Spring Cloud Sleuth or integrating with APM solutions such as New Relic or AppDynamics to monitor performance metrics and logs.
The Closing Argument
Upgrading to Lettuce 5 can greatly enhance your application's performance and maintainability. By following the steps outlined—from backing up your code and reviewing breaking changes to updating and thoroughly testing your application—you can transition smoothly.
Don't forget to leverage the new features, especially in reactive programming and stream handling. With these improvements, your application can become not just functional but significantly more efficient.
For more insights into Redis and Lettuce, consider visiting the official Lettuce documentation and checking out the Redis documentation.
Now, go ahead and make your application better by embracing the changes that Lettuce 5 offers! Happy coding!