Troubleshooting Common DataSource Issues in Docker Containers
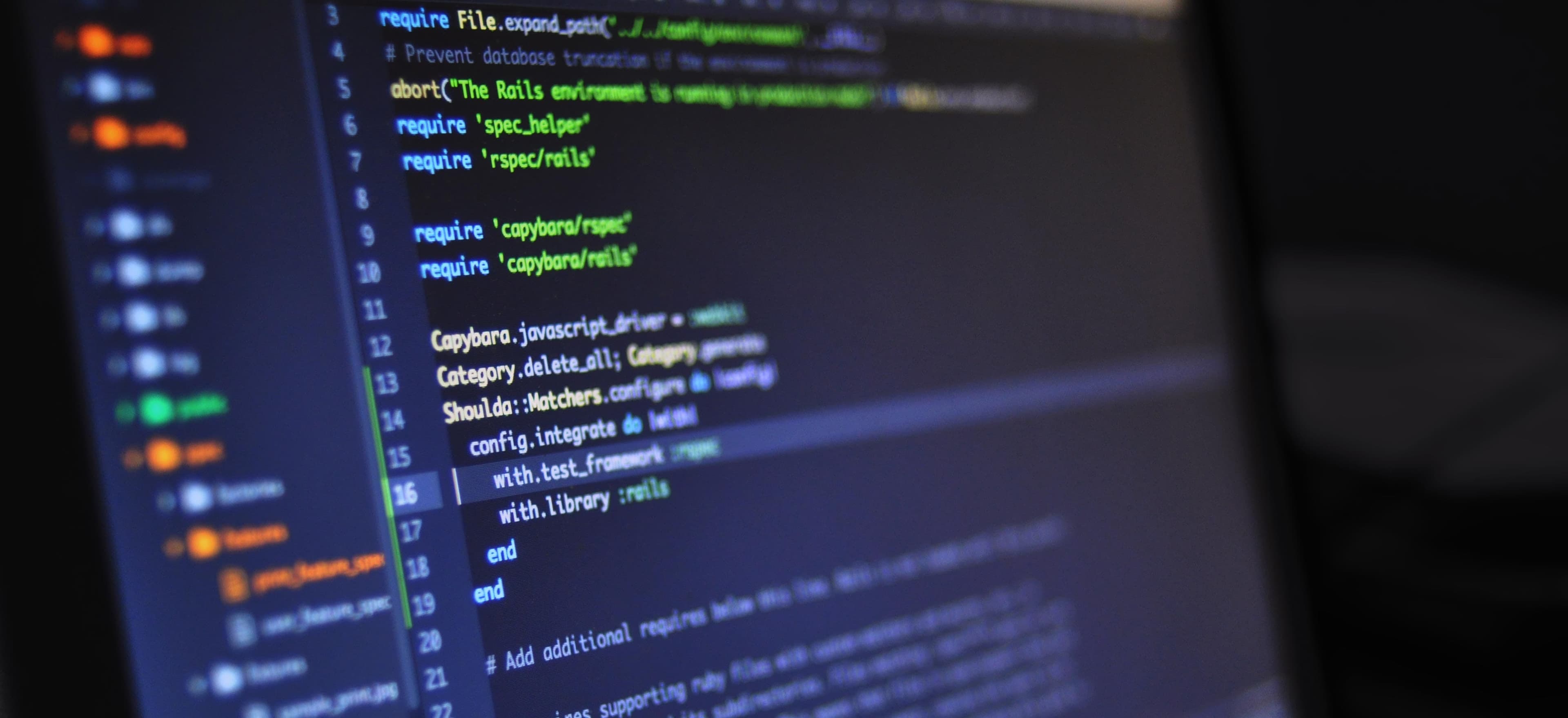
- Published on
Troubleshooting Common DataSource Issues in Docker Containers
When deploying applications using Docker, the isolation and environment configuration that Docker containers provide can sometimes lead to common DataSource issues. DataSource in Java usually refers to the source of data for database connections, and when things go wrong, it can create a significant bottleneck in your application. In this blog post, we will explore common DataSource issues you may encounter while working with Docker containers, alongside tips and troubleshooting methodologies.
Understanding DataSource
Before we dive deep into troubleshooting, let's clarify what we mean by DataSource. A DataSource in Java is like a factory for connections to a database, allowing applications to connect to various data sources easily. It abstracts the complexity of establishing connections and can manage connection pools efficiently.
Here's a conventional setup for a DataSource in a Java application using the HikariCP connection pooling library:
import com.zaxxer.hikari.HikariConfig;
import com.zaxxer.hikari.HikariDataSource;
public class DataSourceConfig {
private static HikariDataSource dataSource;
public static HikariDataSource getDataSource() {
if (dataSource == null) {
HikariConfig config = new HikariConfig();
config.setJdbcUrl("jdbc:mysql://localhost:3306/mydb");
config.setUsername("username");
config.setPassword("password");
config.setMinimumIdle(5);
config.setMaximumPoolSize(10);
config.setConnectionTimeout(30000);
dataSource = new HikariDataSource(config);
}
return dataSource;
}
}
In the above snippet, we configure our DataSource using HikariCP. This is important because it optimizes database connections using pooling, ultimately improving performance.
Common DataSource Issues in Docker
1. Networking Issues
When running your Java application in a Docker container, it's critical to configure the network correctly. By default, Docker containers run in an isolated network, meaning they cannot communicate with services outside of that network unless explicitly permitted.
Solution:
Ensure your database is accessible from the container. Use docker exec
to get a shell in your running container and use ping
or telnet
to diagnose connectivity issues. Make sure you're using the correct hostname or IP address.
docker exec -it <container_name> /bin/sh
# Inside the container
ping <database_host>
To create a bridge network that allows for better communication:
docker network create my-network
docker run --network my-network ...
2. Environment Variables Misconfiguration
Docker containers usually rely on environment variables for configuration. A simple typo in these environment variables can cause your application to fail to connect to the DataSource.
Solution:
Check your Dockerfile or docker-compose.yml
to ensure that the variables are set correctly. In a docker-compose.yml
file, you can configure environment variables like this:
version: '3'
services:
myapp:
image: myapp-image
environment:
- DB_HOST=mysql
- DB_PORT=3306
- DB_NAME=mydb
- DB_USER=username
- DB_PASS=password
depends_on:
- mysql
mysql:
image: mysql:5.7
environment:
MYSQL_ROOT_PASSWORD: root
MYSQL_DATABASE: mydb
MYSQL_USER: username
MYSQL_PASSWORD: password
Make sure that these variables are being read correctly in your Java application. You can use System.getenv("VARIABLE_NAME")
to fetch them.
3. Database Initialization Time
One of the most common problems during development occurs when the Java application tries to connect to the database before it is fully up and running. This can be especially troublesome in a microservices architecture where services may need to wait for databases or other services.
Solution:
You can add a simple delay or retry mechanism before your application tries to connect to the database. For example, in a Spring Boot application configuration:
import java.util.concurrent.TimeUnit;
@Bean
public DataSource dataSource() {
// Retry mechanism to wait for the database connection
int retries = 5;
while (retries-- > 0) {
try {
// Attempt to connect
hikariDataSource = getDataSource();
hikariDataSource.getConnection().close();
break;
} catch (SQLException e) {
System.out.println("Database not available yet, retrying...");
try {
TimeUnit.SECONDS.sleep(10);
} catch (InterruptedException ignored) {}
}
}
return hikariDataSource;
}
By implementing this method, your application can effectively wait for the database to be ready before trying to establish a connection.
4. Container Resource Limits
If your container is running out of memory or CPU resources, it can fail to create new database connections or even crash. Monitoring resource usage is critical for ensuring that your application remains operational.
Solution:
Use Docker's configuration options to allocate enough memory and CPU. You can specify limits in your docker-compose.yml
file:
version: '3'
services:
myapp:
image: myapp-image
deploy:
resources:
limits:
memory: 512M
cpus: '0.5'
5. Database Driver Issues
A misconfigured or missing database driver can lead to errors, making it impossible to connect to your DataSource. Java applications need the correct JDBC driver to communicate with a particular type of database.
Solution:
Make sure you include the proper JDBC driver in your Docker image. If you are using Maven, include the dependency in your pom.xml
:
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.26</version>
</dependency>
If you are using a custom Dockerfile, ensure that the JDBC driver JAR is included in the Docker image:
FROM openjdk:11-jre-slim
COPY target/myapp.jar /app/myapp.jar
COPY path_to_driver/mysql-connector-java-8.0.26.jar /app/mysql-connector-java.jar
# Set entry point command
ENTRYPOINT ["java", "-cp", "/app/myapp.jar:/app/mysql-connector-java.jar", "com.example.Main"]
6. Logging for Debugging
Sometimes, the issues may not be overtly apparent. Effective logging is essential for debugging. Ensure your application provides clear and concise logs when trying to establish a DataSource connection.
Solution:
Use logging frameworks like SLF4J or Log4j in your application. By logging connection attempts, errors, and exceptions, you get a clearer picture of where the failure originates.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class ConnectionManager {
private static final Logger logger = LoggerFactory.getLogger(ConnectionManager.class);
public void connect() {
try {
DataSource dataSource = DataSourceConfig.getDataSource();
Connection connection = dataSource.getConnection();
logger.info("Connection established successfully!");
} catch (SQLException e) {
logger.error("Failed to establish connection: {}", e.getMessage());
}
}
}
Final Thoughts
Troubleshooting DataSource issues in Docker containers can be challenging, but by understanding common pitfalls, it becomes easier to diagnose and rectify problems. Always ensure your network configurations are correct, environment variables are set, and appropriate resources are allocated for smooth operations. With these strategies, you can minimize downtime and improve the reliability of your applications.
By implementing robust logging strategies, you can gather essential insights which will further assist in your troubleshooting processes. If you wish to deepen your knowledge in this area, the Docker documentation and HikariCP GitHub page can serve as excellent resources.
For more intricate details, please feel free to reach out in the comments section below or share your unique troubleshooting experiences!