Common Pitfalls When Joining Strings in JDK 8
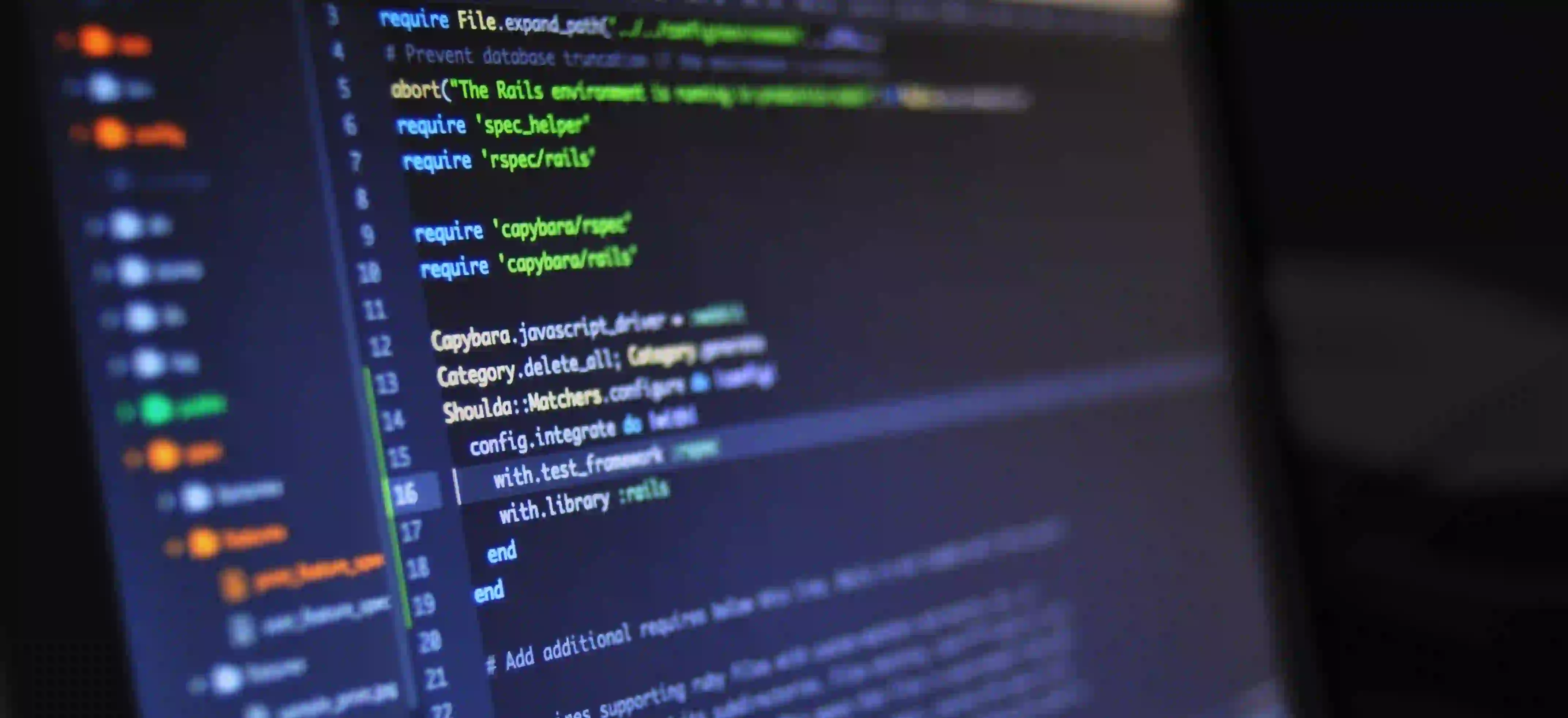
Common Pitfalls When Joining Strings in JDK 8
String manipulation is a fundamental aspect of programming in Java, prevalent in applications ranging from simple scripts to large enterprise systems. In JDK 8, the introduction of new methods and utilities for string manipulation has enhanced the way we can join strings. This post will explore some common pitfalls when joining strings in JDK 8, highlighting best practices, code examples, and insightful commentary.
Understanding String Joining in JDK 8
Before we dive into potential pitfalls, let's review how string joining works in JDK 8. The String.join()
method is the most straightforward way to concatenate strings in Java. Here's a simple example:
String[] words = {"Hello", "World", "from", "Java"};
String result = String.join(" ", words);
System.out.println(result); // Output: Hello World from Java
Explanation of the Code
In the above code snippet, we have an array of words and use String.join()
to concatenate them into a single string, separated by a space.
Why is this useful?
- It simplifies the syntax for joining strings compared to older methods that rely on
StringBuilder
. - It enhances readability, making it easier to understand what the code is doing.
Now that we have a basic understanding of string joining in JDK 8, let's look at some common pitfalls.
1. Using Null Values
When joining strings, one major pitfall is not handling null
values. According to the official Java documentation, String.join()
does not treat null
values well.
Pitfall Example
String[] words = {"Hello", null, "World"};
String result = String.join(" ", words);
// Throws NullPointerException
Solution
To prevent NullPointerException
, ensure that you handle null
values before attempting to join them. One way is to filter out the null values:
String[] words = {"Hello", null, "World"};
String result = Arrays.stream(words)
.filter(Objects::nonNull)
.collect(Collectors.joining(" "));
System.out.println(result); // Output: Hello World
Why this change?
- The use of Java Streams provides a fluent way to manipulate data collections.
- It allows for cleaner code that’s easier to maintain.
2. Performance Implications
Another common issue when joining strings is overlooking performance implications, especially with large datasets. Using String.join()
directly can lead to performance bottlenecks if the dataset is large and you're performing multiple joins.
Pitfall Example
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David", "Eve");
String result = String.join(", ", names);
Performance Concern
Although String.join()
is performant for smaller datasets, it can cause inefficiencies if repeated many times in a loop or when used on large lists.
Solution
For better performance, consider using StringBuilder
:
List<String> names = Arrays.asList("Alice", "Bob", "Charlie", "David", "Eve");
StringBuilder sb = new StringBuilder();
for (int i = 0; i < names.size(); i++) {
sb.append(names.get(i));
if (i < names.size() - 1) sb.append(", ");
}
String result = sb.toString();
System.out.println(result); // Output: Alice, Bob, Charlie, David, Eve
Why is this better?
StringBuilder
is mutable and prevents the creation of multiple intermediate string objects.- This reduces memory overhead and enhances performance.
3. Immutability of Strings
A lesser-known pitfall is forgetting about the immutability of strings in Java. Each time you modify a string, a new string object is created. This behavior can lead to performance issues, especially in large loops.
Pitfall Example
String result = "";
for (String name : names) {
result += name + ", "; // Inefficient due to string immutability
}
Solution
Again, using StringBuilder
mitigates this issue:
StringBuilder sb = new StringBuilder();
for (String name : names) {
sb.append(name).append(", ");
}
String result = sb.toString();
// Remove the last comma and space
if (result.length() > 0) {
result = result.substring(0, result.length() - 2);
}
System.out.println(result); // Output: Alice, Bob, Charlie, David, Eve
Why this approach?
- Reduces object creation overhead and enhances performance in loops.
- Avoids unnecessary memory consumption.
4. Using Incorrect Delimiters
When using String.join()
, another common mistake is using an incorrect delimiter. If you accidentally set the wrong delimiter, the resulting string may not have the desired format.
Pitfall Example
String[] words = {"Hello", "World"};
String result = String.join(",", words);
System.out.println(result); // Output: Hello,World
Solution
Use appropriate delimiters according to your needs. For better readability, you might want a space or another character:
String result = String.join(" ", words);
System.out.println(result); // Output: Hello World
Why this matters?
- Selecting the correct delimiter influences the overall structure and readability of the output string.
5. Failing to Validate Input
Lastly, failure to validate input before joining strings can result in unintended output. This is particularly important when dealing with user input or data from external sources.
Pitfall Example
String userInput = null; // simulating user input
String result = String.join(" ", userInput.split(" "));
// This can lead to NullPointerException
Solution
Validate the input before proceeding:
String userInput = null;
if (userInput != null) {
String result = String.join(" ", userInput.split(" "));
System.out.println(result);
} else {
System.out.println("Input is null");
}
Why is validation critical?
- It helps to ensure your application behaves reliably under various scenarios, increasing robustness.
Final Thoughts
String joining in JDK 8 brings with it powerful functionality, but it also carries potential pitfalls. By understanding common errors such as managing null values, performance implications, immutability, incorrect delimiters, and input validation, you can write cleaner, more efficient Java code.
For more detailed information on string handling in Java, consider reviewing the official Java String documentation. By following best practices and leveraging the tools provided by the Java SDK, you can effectively manipulate strings and avoid these common pitfalls.
Happy coding in Java!