Why Your JVM Performance is Slowing Down: Key Issues
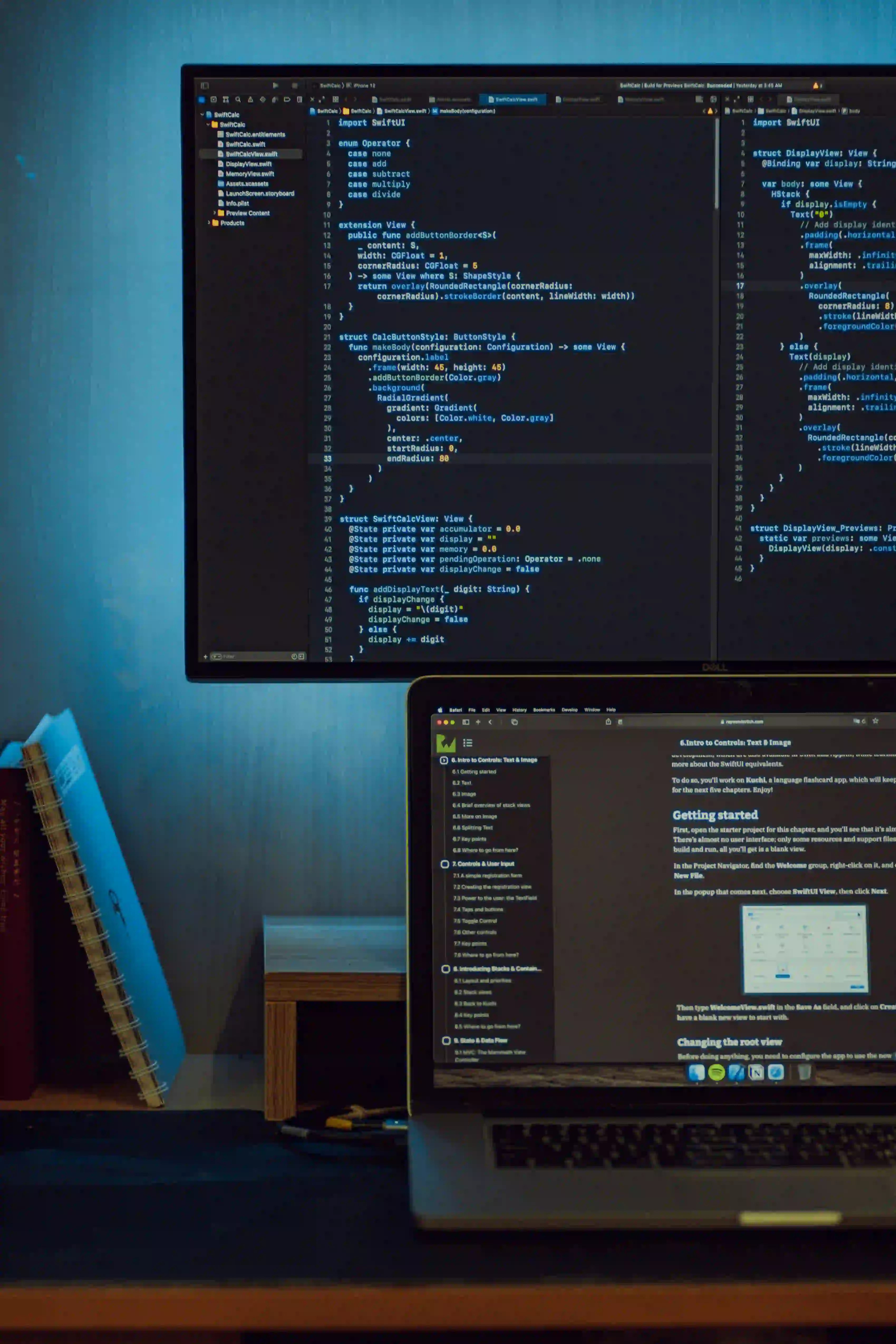
Why Your JVM Performance is Slowing Down: Key Issues
Java Virtual Machine (JVM) is an essential component of Java applications. It facilitates the execution of Java bytecode on any device equipped with a compatible JVM. However, like any other technology, performance issues can arise, leading to slowdowns that can significantly impact your application's efficiency.
In this blog post, we will explore key factors that contribute to JVM performance degradation, how to identify these issues, and strategies for optimizing performance.
Understanding the JVM's Role
Before delving into common performance issues, let's quickly recap the role of the JVM. The JVM is responsible for:
- Loading Code: It loads Java class files.
- Execution: It executes bytecode, which is the compiled version of Java.
- Memory Management: It oversees memory allocation and garbage collection.
- Security: It offers a secure environment for running bytecode.
If any of these aspects encounter issues, the performance of Java applications can be adversely affected.
Key Issues Affecting JVM Performance
1. Poor Garbage Collection
Garbage Collection (GC) is a key feature of the JVM that automatically manages memory by reclaiming space occupied by objects no longer in use. However, poorly configured or inefficient GC can lead to performance bottlenecks.
How to Identify GC Issues
You can identify if garbage collection is slowing down your JVM by:
- Monitoring the GC logs for frequent full GC events.
- Analyzing Heap Dump Files to inspect memory usage.
Solution
Choosing the right garbage collector is paramount. There are several types of garbage collectors available:
- Serial GC: Good for smaller applications.
- Parallel GC: Better suited for multi-core processors.
- G1 GC: Suitable for applications with large heap spaces and lower pause times.
For example, to enable G1 GC, you can launch your application with the following command:
java -XX:+UseG1GC -jar YourApp.jar
2. Memory Leaks
Memory leaks occur when an application retains references to objects that are no longer needed. This can lead to increased memory consumption over time, eventually resulting in OutOfMemoryError
.
How to Identify Memory Leaks
You might notice the following signs of memory leaks:
- Increasing memory consumption in long-running applications.
OutOfMemoryError
exceptions in logs.
Using profiling tools like VisualVM or JProfiler can help track down memory leaks and understand object retention.
Solution
Most memory leak issues come from maintaining unnecessary references in Java. Ensure that:
- You nullify references to objects when they are no longer necessary.
- Utilize weak references for caches or temporary storage.
3. Inefficient Code
Underlying code inefficiencies can significantly affect performance. Some common problems include:
- Unoptimized algorithms: Choosing the wrong algorithm can lead to excessive complexity.
- Excessive object creation: Creating unnecessary objects, especially within loops.
Solution
To optimize your code, consider the following practices:
- Profile your application using Java Profiling Tools to identify slow sections of the code.
- Utilize
StringBuilder
instead ofString
concatenation in loops:
StringBuilder sb = new StringBuilder();
for (String s : stringArray) {
sb.append(s).append(" ");
}
Why? This approach is preferred because String
concatenation creates multiple immutable objects, which increases memory overhead. StringBuilder
allows reusing the same memory space, enhancing performance.
4. Thread Contention
In multi-threaded applications, when multiple threads compete for the same resource (like synchronized blocks), contention can occur, slowing down performance.
How to Identify Thread Contention
You can detect thread contention through:
- Thread dumps, which provide insights on thread states.
- Profiling tools that indicate blocked or waiting threads.
Solution
- Reduce synchronization: Refactor code to minimize locked resources or use concurrent data structures like
ConcurrentHashMap
. - Increase granularity: Instead of using one lock, consider using multiple locks.
Example:
class Counter {
private final Object lock = new Object();
private int count;
public void increment() {
synchronized (lock) {
count++;
}
}
}
Why? By synchronizing only the critical section, we minimize contention.
5. JVM Settings and Configurations
Improper JVM settings can lead to suboptimal performance. Key configurations include:
- Heap Size: Setting inappropriate heap sizes may lead to frequent garbage collections or memory-runout situations.
- Thread Stack Size: Excessive stack sizes can waste memory.
Solution
Adjust the heap size during JVM startup:
java -Xms512m -Xmx2048m -jar YourApp.jar
Why? Setting an initial heap size (-Xms
) and a maximum heap size (-Xmx
) helps in stabilizing the memory footprint of your application.
Monitoring JVM Performance
Apart from identifying performance issues, it’s essential to monitor the JVM's health regularly. Various tools can assist with this:
- Java Mission Control: A powerful profiling and diagnostics tool.
- VisualVM: It provides visualization of the JMX data.
- Prometheus and Grafana: Excellent for collecting metrics and visualizing performance metrics over time.
A Final Look
Understanding JVM performance is crucial for developers aiming to create efficient Java applications. By addressing issues such as garbage collection inefficiencies, memory leaks, inefficient coding practices, thread contention, and poor JVM configurations, you can significantly optimize your application's performance.
To learn more about managing Java application's performance, take a look at the following resources:
- Java Garbage Collection Basics
- Java Profiling Tools
Effective JVM management not only enhances your application’s performance but also leads to a better user experience. Stay vigilant, monitor performance, and adapt your strategies as needed. By doing so, you'll ensure that any slowdowns are swiftly addressed, allowing your Java applications to thrive.