Choosing the Right JSON Parser: Jackson vs Gson vs Json-B
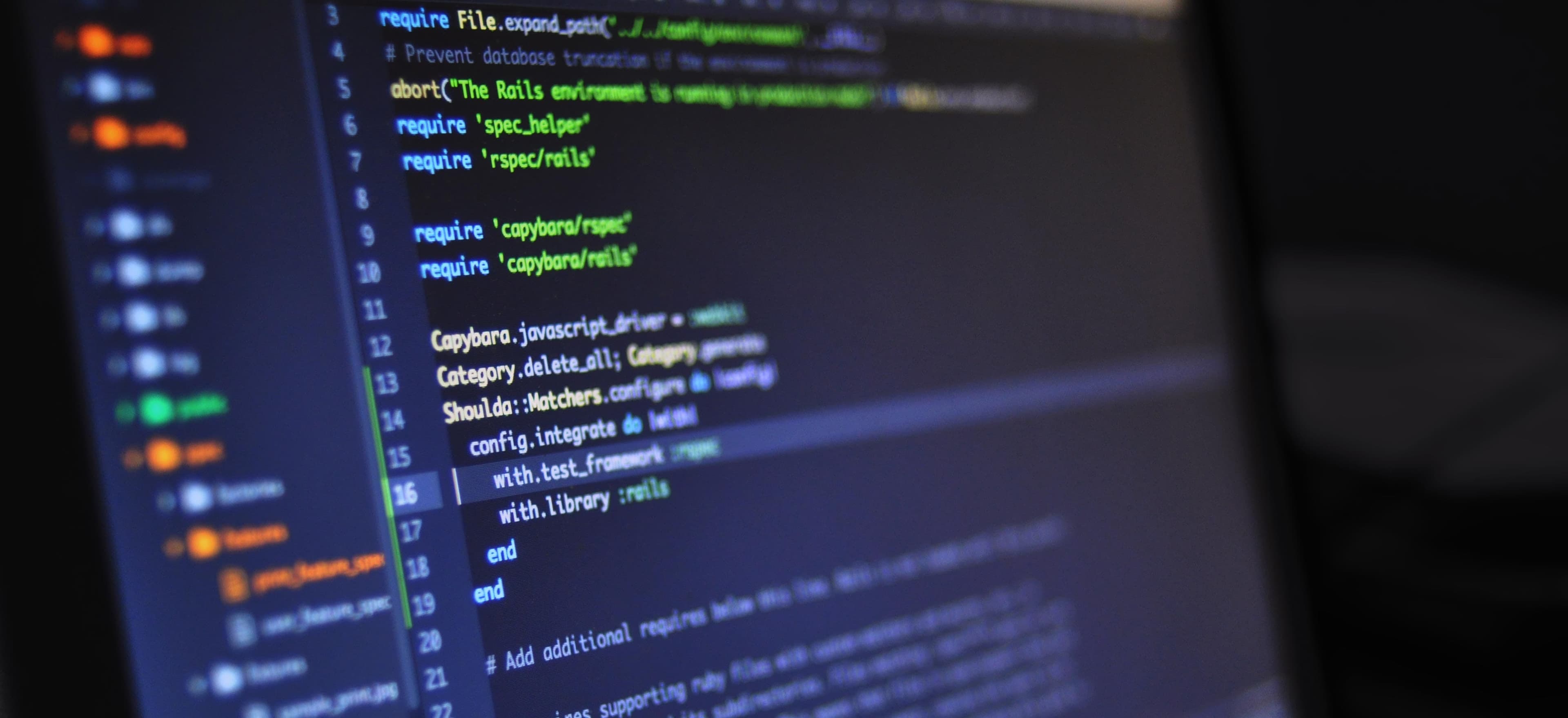
- Published on
Choosing the Right JSON Parser: Jackson vs Gson vs Json-B
When it comes to working with JSON in Java, developers often find themselves at a crossroads: which JSON parser should they choose? Three of the most prominent options are Jackson, Gson, and Json-B. Each library has its own strengths, weaknesses, and use cases. In this blog post, we will extensively explore these libraries, providing examples and discussing why you might choose one over the others.
Understanding JSON Parsing in Java
JSON (JavaScript Object Notation) is a lightweight data interchange format that is easy for humans to read and write and easy for machines to parse and generate. Java, being a robust programming language, provides several libraries to work efficiently with JSON.
Selecting the appropriate JSON parser can greatly influence not only the development process but also application performance and maintainability.
Overview of the JSON Libraries
Jackson
Jackson is a high-performance JSON processor for Java. Known for its speed and efficiency, it supports a wide range of data formats, making it versatile. Jackson is particularly powerful when dealing with large-scale applications.
Key Features:
- Performance: Jackson is often considered the fastest JSON library available for Java.
- Data Binding: It supports both XML and JSON data binding.
- Streaming: Jackson offers a streaming API for reading and writing JSON.
- Annotations: Jackson provides a rich variety of annotations to customize serialization/deserialization.
Example Code Snippet
import com.fasterxml.jackson.databind.ObjectMapper;
public class JacksonExample {
public static void main(String[] args) throws Exception {
ObjectMapper objectMapper = new ObjectMapper();
// Sample Java Object
User user = new User("John", "Doe");
// Serialize Java object to JSON
String jsonString = objectMapper.writeValueAsString(user);
System.out.println("Serialized JSON: " + jsonString);
// Deserialize JSON back to Java object
User deserializedUser = objectMapper.readValue(jsonString, User.class);
System.out.println("Deserialized User: " + deserializedUser);
}
}
class User {
private String firstName;
private String lastName;
// Constructors, Getters, and Setters
public User() { }
public User(String firstName, String lastName) {
this.firstName = firstName;
this.lastName = lastName;
}
@Override
public String toString() {
return firstName + " " + lastName;
}
}
In this Jackson example:
- We define a
User
class. - We utilize the
ObjectMapper
to serialize aUser
instance into JSON and then deserialize it back into aUser
object. - The use of Jackson annotations can further enhance this process by customizing the mapping, but the example gives a clear demonstration of basic usage.
Gson
Gson, developed by Google, is another powerful library used for converting Java objects into JSON and vice versa. It is known for its simplicity and ease of use, making it a good choice for many developers.
Key Features:
- Simplicity: Gson's API is straightforward, making it easy for beginners to grasp.
- Type Conversion: It automatically handles complex types and allows for user-defined types.
- Null Object Handling: Gson has great support for null objects.
Example Code Snippet
import com.google.gson.Gson;
public class GsonExample {
public static void main(String[] args) {
Gson gson = new Gson();
User user = new User("Jane", "Doe");
// Serialize Java object to JSON
String jsonString = gson.toJson(user);
System.out.println("Serialized JSON: " + jsonString);
// Deserialize JSON back to Java object
User deserializedUser = gson.fromJson(jsonString, User.class);
System.out.println("Deserialized User: " + deserializedUser);
}
}
In this Gson example:
- Similar to the previous code, we define the
User
class. - We use the
Gson
object to convert a Java object to JSON and vice versa. - Its simplicity allows developers to quickly implement code without much overhead.
Json-B
Json-B (Java API for JSON Binding) is a newer standard for binding Java objects to JSON and vice versa. It offers a more Java-centric approach and integrates seamlessly with Jakarta EE.
Key Features:
- Standardization: Being a Java EE specification, Json-B provides consistent serialization across different implementations.
- Annotations: Similar to Jackson, Json-B supports annotations for customization.
- Stream-based API: It has a streaming API for efficient processing.
Example Code Snippet
import javax.json.bind.Jsonb;
import javax.json.bind.JsonbBuilder;
public class JsonBExample {
public static void main(String[] args) {
Jsonb jsonb = JsonbBuilder.create();
User user = new User("Alice", "Smith");
// Serialize Java object to JSON
String jsonString = jsonb.toJson(user);
System.out.println("Serialized JSON: " + jsonString);
// Deserialize JSON back to Java object
User deserializedUser = jsonb.fromJson(jsonString, User.class);
System.out.println("Deserialized User: " + deserializedUser);
}
}
In this Json-B example:
- We create an instance of
Jsonb
to handle our object conversion. - The
toJson
andfromJson
methods are straightforward, demonstrating Json-B's compatibility with the Java programming model.
Performance Overview
When it comes to performance, Jackson generally outperforms both Gson and Json-B in terms of speed and memory consumption, making it ideal for large-scale applications. However, both Gson and Json-B are more than adequate for standard use cases.
To accurately choose a library, consider the size of your JSON data and the need for speed. If performance is critical, lean towards Jackson. You can evaluate performance using profiling tools, or run benchmarks to compare speed and memory usage based on your specific use cases.
Complexity vs. Simplicity
- Complexity: If your project requires handling complex JSON objects, Jackson offers extensive annotations and features that allow for advanced mapping. However, it does carry a steeper learning curve.
- Simplicity: For straightforward applications, Gson is often preferred due to its simple API and ease of use. Json-B offers a balance, being user-friendly while adhering to Java EE standards.
Final Thoughts
Choosing between Jackson, Gson, and Json-B ultimately depends on the specific requirements of your project. Jackson offers top-tier performance and flexibility but with complexity, while Gson presents a straightforward solution for simpler tasks, and Json-B is a great choice for standardization in Java EE environments.
Make sure to evaluate your project needs, including performance, complexity, and standardization, before making a decision. For further context on these libraries, you might want to visit the official Jackson documentation and the Gson documentation.
By understanding the capabilities and limitations of each JSON parser, you can make a more informed choice, ensuring that your Java applications are efficient and maintainable. The right tool can make all the difference in your development experience. Happy coding!
Checkout our other articles