Breaking Down HATEOAS: The Challenge of Linkless APIs
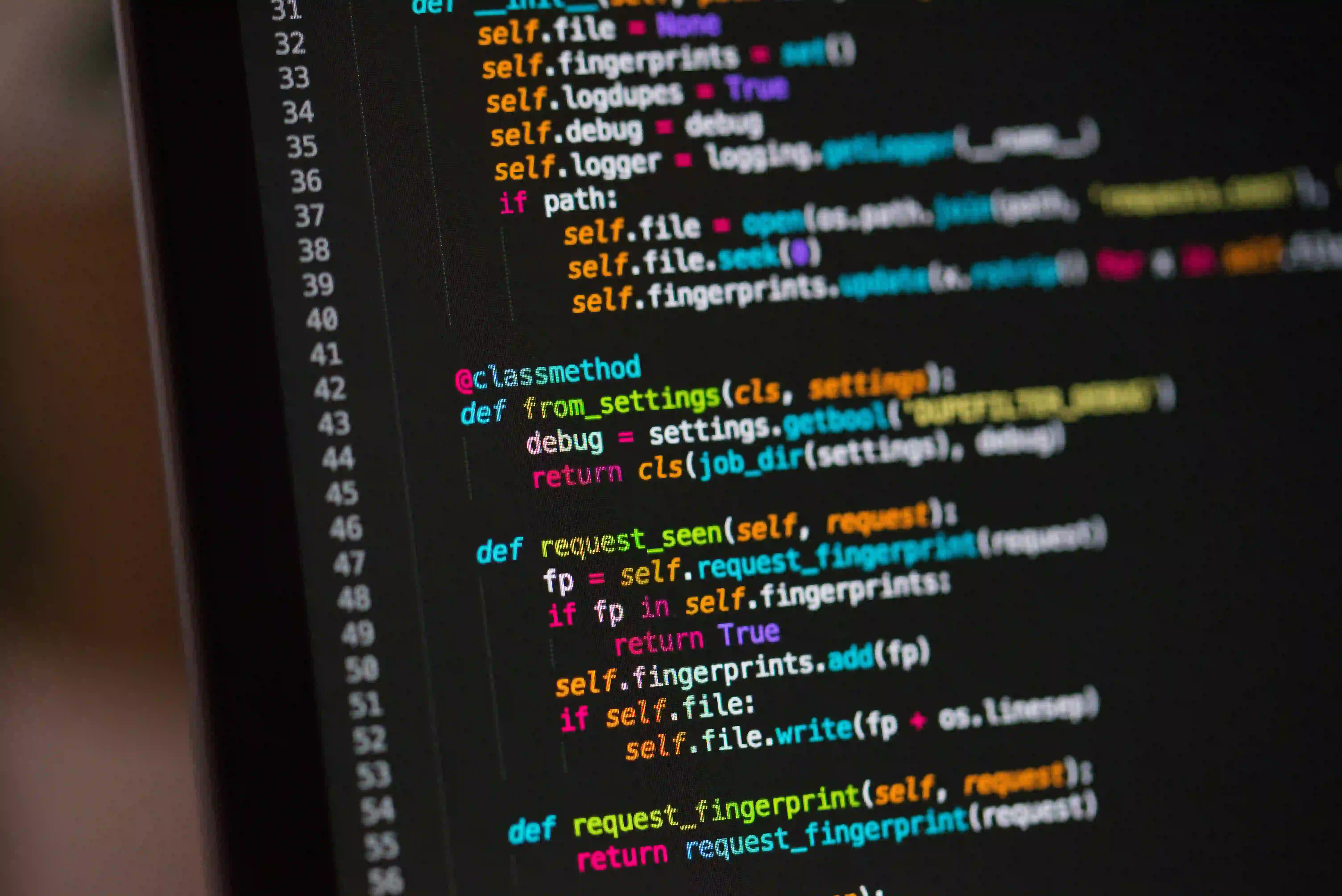
Breaking Down HATEOAS: The Challenge of Linkless APIs
In the realm of web APIs, the architecture you choose can make or break your application's efficiency. One critical aspect of API design is hypermedia. In this context, HATEOAS, or Hypermedia as the Engine of Application State, stands out as a powerful concept. In this blog post, we will delve into HATEOAS, its relevance, and the implications of using linkless APIs.
Understanding HATEOAS
HATEOAS is a constraint of the REST application architecture. It allows clients to dynamically navigate the API by following hyperlinks contained in the responses from the server. In simpler terms, it means that the client should use the links provided by the server to transition to different states in the application.
Why HATEOAS?
-
Decoupling: HATEOAS decouples clients from the server's implementation. If the backend changes (e.g., adding a new endpoint), the client does not need to be explicitly updated—just follow the new links.
-
Discoverability: It enhances the discoverability of API functionality. When consumers have access to relevant links, they can easily navigate to associated resources without needing a separate specification.
-
Reduced Documentation Burden: As you rely on hypermedia, the need for extensive documentation diminishes. The links serve as a map of the API's capabilities.
Example of HATEOAS
Let’s break down how HATEOAS works in a simplified example. Consider a user resource that provides information about users and their related links.
JSON Response Example
{
"user": {
"id": 1,
"name": "John Doe",
"email": "john@example.com"
},
"links": [
{
"rel": "self",
"href": "/users/1"
},
{
"rel": "friends",
"href": "/users/1/friends"
},
{
"rel": "posts",
"href": "/users/1/posts"
}
]
}
Explanation of the JSON
In the above JSON response:
- User Object: Contains user details (id, name, email).
- Links Array: Provides actionable links related to the user. The
rel
attribute describes the relationship, whilehref
presents the URL.
By using this response structure, clients can effectively navigate to a user’s friends or posts simply by following the links.
The Challenge of Linkless APIs
In stark contrast to APIs adhering to HATEOAS principles, linkless APIs provide no self-navigation capabilities. These APIs define what resources are available and how to interact with them through separate documentation rather than built-in navigation.
Characteristics of Linkless APIs
-
Rigid Structure: Clients are often tightly coupled to the resources and actions defined in the API documentation. If any endpoint changes, the client must also change accordingly.
-
Complexity in Usage: Developers need to be more attentive to documentation updates. This can lead to confusion and errors as clients may mistakenly call outdated or incorrect endpoints.
Comparison: HATEOAS vs. Linkless APIs
| Feature | HATEOAS | Linkless APIs | |--------------------|--------------------------------------|---------------------------------------| | Coupling | Loosely coupled | Tightly coupled | | Discoverability | High | Low | | Documentation | Minimal (self-descriptive) | Extensive requirement | | Flexibility | High (dynamic navigation) | Low (static paths) |
Why Moving Toward HATEOAS is Important
As software evolves towards microservices and decentralized architecture, adopting HATEOAS becomes essential. This kind of flexibility proves invaluable in reducing integration overhead as well as continuous maintenance of software systems.
Implementing HATEOAS in Java
Now that we've established the significance of HATEOAS, it's crucial to understand how to implement it using Java, specifically with Spring Boot, which is one of the most popular frameworks for building RESTful APIs.
Step-by-Step Implementation
1. Setting Up Your Spring Boot Project
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-hateoas</artifactId>
</dependency>
In your pom.xml
, include the HATEOAS starter dependency. This dependency provides you with everything you need to get started.
2. Creating the Model
@Entity
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
private String name;
private String email;
// Getters and Setters
}
This simple User entity represents our data structure.
3. Creating the Controller
@RestController
@RequestMapping("/users")
public class UserController {
@Autowired
private UserRepository userRepository;
@GetMapping("/{id}")
public EntityModel<User> getUserById(@PathVariable Long id) {
User user = userRepository.findById(id)
.orElseThrow(() -> new UserNotFoundException(id));
EntityModel<User> resource = EntityModel.of(user);
resource.add(linkTo(methodOn(UserController.class).getUserById(user.getId())).withSelfRel());
resource.add(linkTo(methodOn(UserController.class).getUserFriends(user.getId())).withRel("friends"));
resource.add(linkTo(methodOn(UserController.class).getUserPosts(user.getId())).withRel("posts"));
return resource;
}
// other methods (like getUserFriends and getUserPosts)
}
Explanation of the Controller
In this controller:
EntityModel<T>
wraps theUser
object and allows adding hypermedia links.linkTo
andmethodOn
create URIs dynamically based on existing endpoints, enhancing flexibility.
The Future of APIs: Embracing HATEOAS
The transition to HATEOAS not only introduces flexibility and discoverability, but it also positions applications to evolve in a more manageable and scalable manner. As microservices and decoupled architectures grow in popularity, APIs adhering to HATEOAS principles will dominate the market.
Closing Remarks
In conclusion, embracing HATEOAS is a forward-thinking approach to API design that promotes agility, reduces maintenance burdens, and enhances discoverability. By implementing these principles, developers are better equipped to handle the complexities of modern application architectures.
For further reading on HATEOAS, consider checking out these resources:
- Spring HATEOAS Documentation
- RESTful Web Services with Spring Boot
Adopting HATEOAS might seem like an extra layer of complexity, but the long-term benefits of flexibility and robustness are well worth it. Happy coding!