Mastering Kafka Producer Retries: Avoiding Message Loss
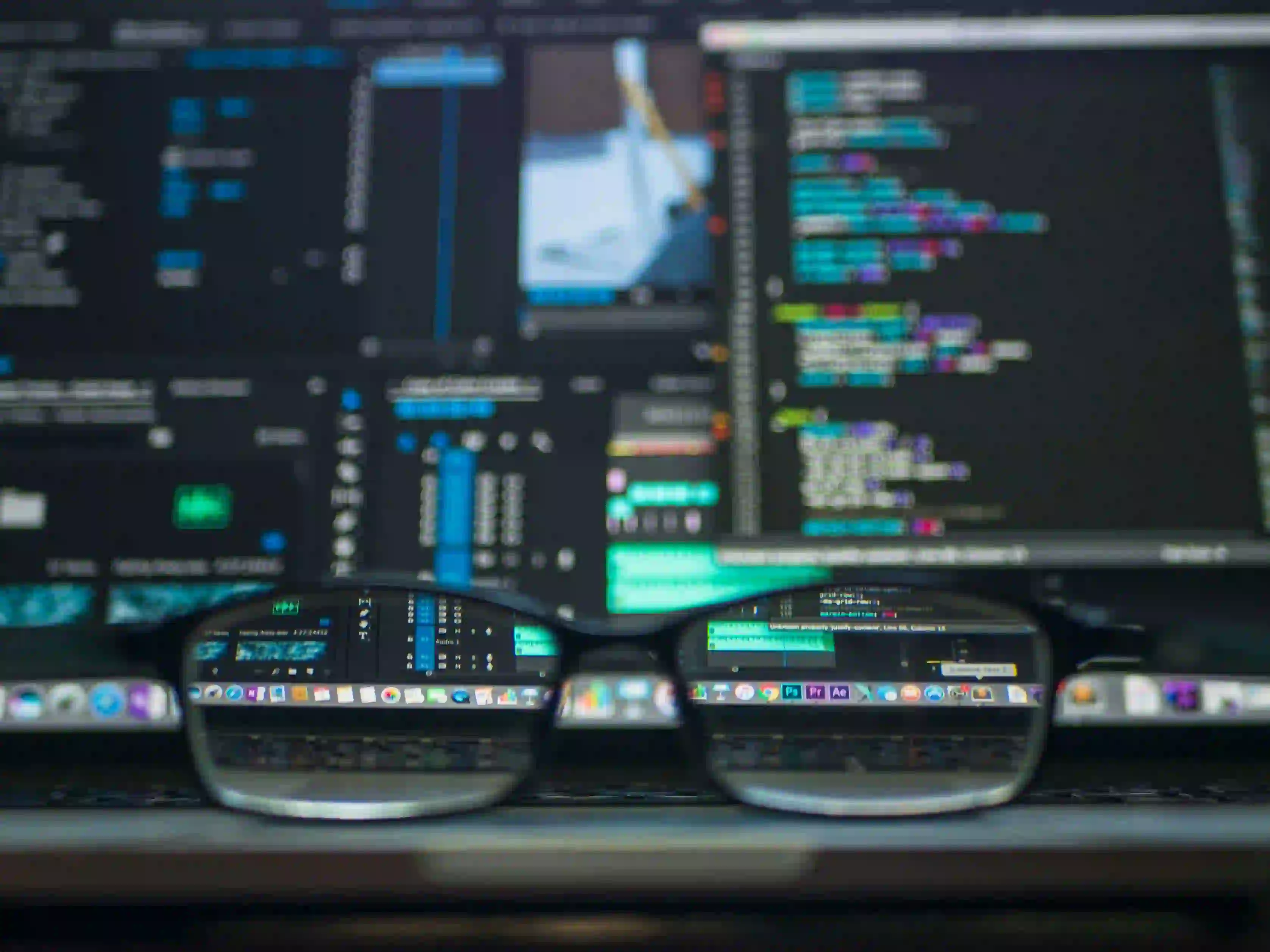
Mastering Kafka Producer Retries: Avoiding Message Loss
Apache Kafka is a powerful distributed streaming platform that excels in high throughput and fault tolerance. One of the critical aspects of ensuring data integrity and reliability within Kafka is mastering producer retries. In this blog post, we will delve into producer retries, the importance of avoiding message loss, and how best to implement retry strategies in Java applications.
Understanding Kafka Producer Retries
When a producer sends a message to a Kafka topic, several things can go wrong—network issues, broker downtime, or even partition reassignment. When such failures occur, it is crucial to ensure that your messages are not lost. This is where retries come into play.
Why Implement Retries?
Kafka producers can attempt to resend messages in the face of transient errors. By implementing retries, you help secure message delivery in the following scenarios:
- Temporary Network Issues: If a network hiccup occurs, retries allow the producer to reattempt sending the message.
- Broker Failures: In instances where a broker is temporarily down, retries can facilitate message delivery once the broker is back online.
- Load Balancing: During high-load scenarios, partitions might be under pressure; retries can alleviate dropped messages due to such spikes.
Configuring Producer Retries
Kafka allows for configurations at the producer level to dictate how many times a message should be retried before it is considered truly undeliverable. The configuration options are primarily controlled through the retries
setting.
Here’s how you can configure a Kafka producer in Java:
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerConfig;
import org.apache.kafka.common.serialization.StringSerializer;
import java.util.Properties;
public class CustomKafkaProducer {
public static KafkaProducer<String, String> createProducer() {
Properties props = new Properties();
props.put(ProducerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
props.put(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
props.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
props.put(ProducerConfig.RETRIES_CONFIG, 5); // Set the number of retries
// Configure the acknowledgments for message delivery
props.put(ProducerConfig.ACKS_CONFIG, "all"); // Wait for full acknowledgment from all replicas
return new KafkaProducer<>(props);
}
}
Explanation of Code Snippet
BOOTSTRAP_SERVERS_CONFIG
: Specifies the Kafka broker addresses that your producer can target.KEY_SERIALIZER_CLASS_CONFIG
andVALUE_SERIALIZER_CLASS_CONFIG
: These specify how the keys and messages will be serialized before being sent to the Kafka topic.RETRIES_CONFIG
: Here, we set the retry count to 5. The producer will attempt to resend the message up to five times before giving up.ACKS_CONFIG
: Setting this to "all" ensures that the producer does not receive acknowledgment until all in-sync replicas have received the message. This helps enhance data durability.
Handling Message Loss
While retries can significantly help mitigate message loss, they do not guarantee it entirely. Under certain circumstances, such as when employing at-most-once semantics, you might inadvertently drop messages. It is essential to evaluate your use case and decide on the appropriate processing guarantee you need:
- At Most Once: Messages might get lost but will not be processed more than once.
- At Least Once: Messages are guaranteed to be delivered but may be processed multiple times.
- Exactly Once: Messages will be delivered once and only once—a more complex model to implement.
Implementing Exactly Once Delivery Semantics
Using Kafka’s transactional capabilities, you can ensure exactly-once semantics. Here’s an enhanced version of the producer that utilizes transactions:
import org.apache.kafka.clients.producer.KafkaProducer;
import org.apache.kafka.clients.producer.ProducerConfig;
import org.apache.kafka.common.serialization.StringSerializer;
import java.util.Properties;
public class TransactionalKafkaProducer {
public static KafkaProducer<String, String> createTransactionalProducer() {
Properties props = new Properties();
props.put(ProducerConfig.BOOTSTRAP_SERVERS_CONFIG, "localhost:9092");
props.put(ProducerConfig.KEY_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
props.put(ProducerConfig.VALUE_SERIALIZER_CLASS_CONFIG, StringSerializer.class.getName());
props.put(ProducerConfig.TRANSACTIONAL_ID_CONFIG, "transactional-id-1"); // Unique transactional ID
KafkaProducer<String, String> producer = new KafkaProducer<>(props);
producer.initTransactions(); // Initialize the transaction
return producer;
}
public static void sendTransactionalMessage(KafkaProducer<String, String> producer, String topic, String key, String value) {
try {
producer.beginTransaction();
producer.send(new ProducerRecord<>(topic, key, value));
producer.commitTransaction(); // Commit the transaction if all messages are sent successfully
} catch (Exception e) {
producer.abortTransaction(); // Abort the transaction in case of an error
e.printStackTrace();
}
}
}
Explanation of the Code Snippet
TRANSACTIONAL_ID_CONFIG
: A unique identifier for the transactional producer. Each producer should have a unique ID to effectively manage transactions.initTransactions
: This method initializes the transaction state of the producer. It must be called before thebeginTransaction
method.beginTransaction
: Starts a new transaction.commitTransaction
: If everything goes well, this commits the transaction.abortTransaction
: In case of failure during sending messages, it aborts the transaction to prevent partial data.
Wrapping Up
Mastering Kafka producer retries is key to maintaining robustness in your applications. The strategies discussed can significantly reduce the chances of message loss while ensuring data is processed reliably.
Investing time in understanding these configurations allows developers to create resilient applications capable of handling Kafka's complexities. As you build and optimize your Kafka producers, consider conducting performance testing to find the optimal retry count and acknowledgment strategies tailored to your needs.
For further learning, check out the official Kafka documentation and the Confluent Developer resources. Kafka’s ecosystem offers a wealth of tools and libraries that can facilitate advanced use cases, including stream processing and event sourcing models.
Stay tuned for our upcoming posts as we explore more Kafka topics and best practices!
Feel free to share your thoughts or experiences with Kafka producers in the comments below!