Top 7 Java Games with Common Performance Issues
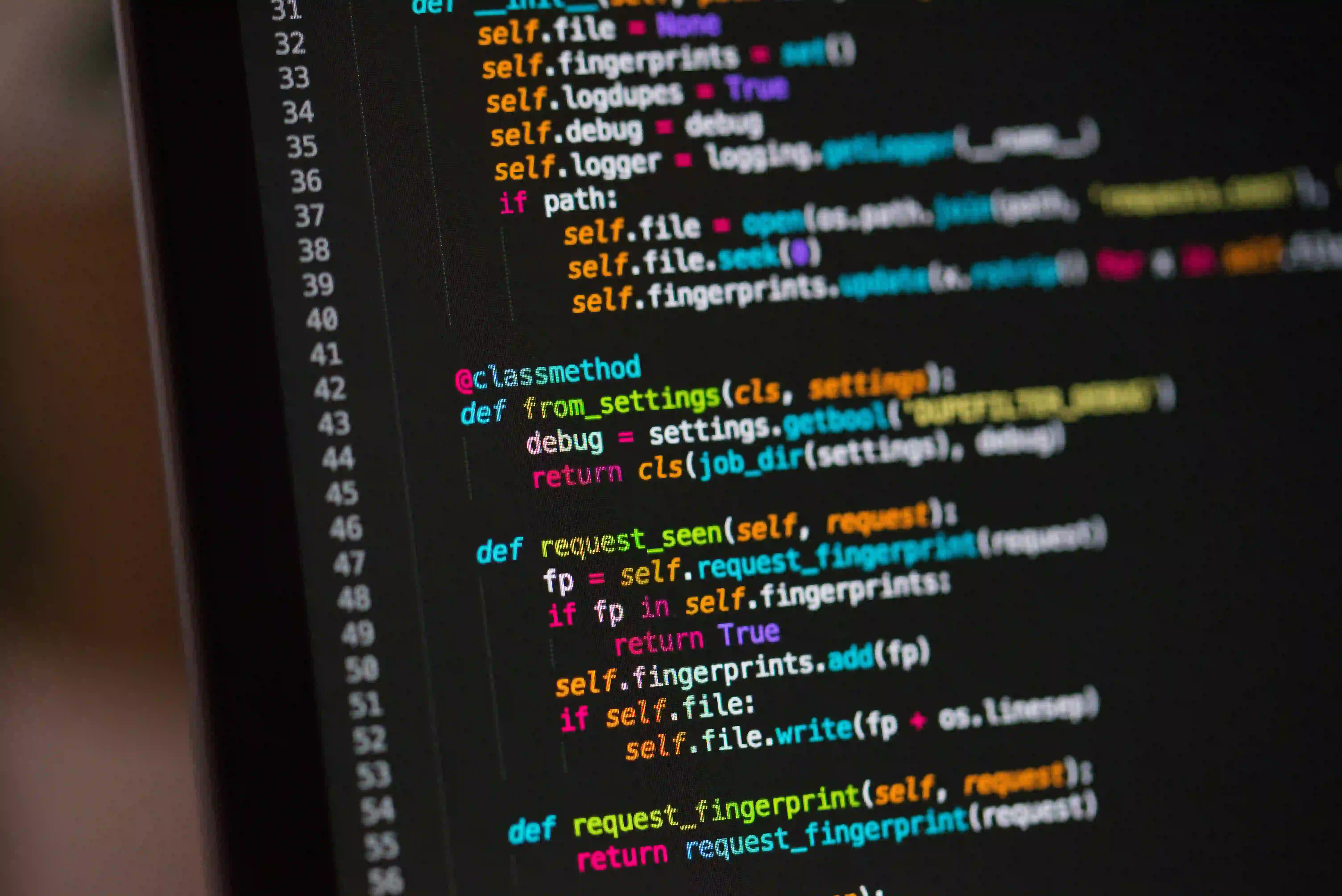
Top 7 Java Games with Common Performance Issues
Java has a longstanding reputation for being a versatile language, especially for game development. With libraries like JavaFX and frameworks such as LibGDX, many developers are able to create engaging and dynamic games. However, even seasoned developers can encounter performance issues that detract from gameplay. In this blog post, we will explore the top seven Java games known for performance problems and provide insights into potential solutions.
1. Minecraft
Overview
Minecraft is arguably the most popular Java game, boasting millions of active players. While its blocky aesthetic allows it to run on various machines, the game can suffer from significant performance issues, especially on lower-end systems.
Performance Issues
- Lag spikes: Often caused by rendering too many chunks at once.
- Memory leaks: Inefficient memory management can lead to crashes.
Quick Fixes
- Optimize settings: Reducing graphics and control settings can help improve performance.
- Use performance-enhancing mods: Mods like OptiFine can enhance performance by adjusting how the game handles graphics.
Example: Chunk Loading Optimization
When creating a game like Minecraft, it’s critical to load chunks efficiently. Here's a simplified code snippet for loading chunks:
public void loadChunk(int x, int z) {
if (!isChunkLoaded(x, z)) {
Chunk chunk = new Chunk(x, z);
chunk.load();
loadedChunks.add(chunk);
}
}
private boolean isChunkLoaded(int x, int z) {
return loadedChunks.stream().anyMatch(c -> c.x == x && c.z == z);
}
In this snippet, we check if a chunk is already loaded before attempting to load it again. This prevents unnecessary loading processes and helps maintain smoother gameplay.
2. Runescape
Overview
This MMORPG has evolved into a complex and visually appealing game. However, the Java-based client occasionally struggles with performance.
Performance Issues
- High CPU usage: The game’s graphics rendering can demand excessive processing power.
- Slow loading times: A large client can take time to update and load new areas.
Quick Fixes
- Clear your cache: This can significantly speed up loading times.
- Adjust graphic settings: Lower settings can ease CPU load.
Example: Resource Management
Efficient resource loading is crucial in an MMORPG. Here’s an approach to manage resources effectively:
public class ResourceManager {
private Map<String, Texture> textures = new HashMap<>();
public Texture getTexture(String filename) {
if (!textures.containsKey(filename)) {
textures.put(filename, new Texture(filename));
}
return textures.get(filename);
}
}
Here, we use a Map
to cache Texture
objects. This prevents the need to reload resources multiple times, effectively reducing CPU usage.
3. Terraria
Overview
While Terraria provides a vivid 2D experience, it is also built using a Java prototype. Players often report performance hiccups, especially in densely populated areas.
Performance Issues
- Frame rate drops: Particularly in large biomes filled with data-heavy elements.
- Garbage collection issues: Frequent allocations can lead to noticeable stutter.
Quick Fixes
- Limit NPCs and items: Reducing the number of entities on-screen can alleviate frame rate drops.
- Tune garbage collection settings: Java's garbage collector settings can significantly impact performance.
Example: Object Pooling
One way to minimize garbage collection issues is by implementing object pooling. The following shows a basic object pool setup:
public class GameObjectPool {
private List<GameObject> availableObjects = new ArrayList<>();
public GameObject acquireObject() {
if (availableObjects.isEmpty()) {
return new GameObject();
} else {
return availableObjects.remove(availableObjects.size() - 1);
}
}
public void releaseObject(GameObject obj) {
availableObjects.add(obj);
}
}
By reusing GameObject
instances, we mitigate the constant creation and destruction of objects, leading to a more stable frame rate.
4. STALKER: Anomaly
Overview
A fan-made standalone mod for the STALKER series, STALKER: Anomaly is built on Java and has garnered attention for both its depth and its performance bottlenecks.
Performance Issues
- Frame rate drops: Especially in expansive zones with numerous interactive objects.
- High memory consumption: Can lead to crashes on lower-end hardware.
Quick Fixes
- Adjust rendering distance: Lowering this can conserve memory.
- Use lower resolution textures: Simplifying visual fidelity can yield performance gains.
Example: Rendering Adjustments
To dynamically adjust rendering distance based on system performance, the following could be implemented:
public void adjustRenderDistance(int availableMemory) {
if (availableMemory < 512) {
renderDistance = 5; // Low
} else if (availableMemory < 1024) {
renderDistance = 10; // Medium
} else {
renderDistance = 20; // High
}
}
This approach allows the game to react according to the available resources, ensuring smoother gameplay.
5. Slay the Spire
Overview
This indie card game has gained recognition for its unique gameplay mechanics. Despite its simplicity, it can struggle with performance issues on more complex runs.
Performance Issues
- Framerate drops when many cards are used: This leads to lag during crucial gameplay moments.
- Memory spikes: Especially during long game sessions.
Quick Fixes
- Limit card animations: Disabling some animations can improve gameplay speed.
- Slot GPU acceleration: Ensuring your Java runtime uses GPU can enhance the game's visuals without taking a performance hit.
Example: Card Animation Control
You can optimize card animations with a simple boolean flag:
public void playCard(Card card) {
if (useAnimations) {
card.animate();
}
// Process card effects without animation
}
By controlling whether animations are enabled, you can reduce the framerate impact during complex turns.
6. Project Zomboid
Overview
This survival horror game has attracted a strong following, but it can be demanding on system resources. Players have shown frustration over frame rate inconsistencies.
Performance Issues
- High CPU and RAM usage: Particularly due to complex simulation mechanics.
- Network lag in multiplayer: Delay in communications can affect the game’s competitiveness.
Quick Fixes
- Limit player connections: Reduce the number of players in a session to maintain performance.
- Optimize networking code: Regular profiling and updates can rectify bottlenecks.
Example: Networking Optimization
Consider implementing a simple packet batching mechanism:
public void sendPackets(List<Packet> packets) {
PacketBatch batch = new PacketBatch();
for (Packet packet : packets) {
batch.add(packet);
}
network.send(batch);
}
Batching packets reduces the number of calls to the network, helping maintain a stable connection.
7. OpenRA
Overview
This open-source reimplementation of classic real-time strategy games often invites players to reminisce. However, performance inconsistencies can negatively affect game-play.
Performance Issues
- High resource usage during large battles: CPU demands can spike dramatically.
- Stuttering animations: Which can affect the overall experience during battles.
Quick Fixes
- Optimize unit count: Keeping battles manageable in size can help.
- Enhance AI efficiency: Improve the AI algorithm to reduce resource contention.
Example: AI Optimization
To enhance AI efficiency, consider tracking unit statuses:
public void updateUnits(List<Unit> units) {
for (Unit unit : units) {
if (!unit.isIdle() && unit.isAlive()) {
unit.performAction();
}
}
}
Minimizing actions to only "active" units reduces the load significantly.
In Conclusion, Here is What Matters
Java games can provide rich experiences, but without diligent attention to performance, players may encounter significant issues. By implementing the strategies outlined in this post, developers can not only optimize existing games but also pave the way for smoother and more engaging future developments. Performance is not merely a feature; it’s essential for player satisfaction.
For more resources on Java game development, check out the Official Java Documentation and LibGDX Wiki for additional insights and community support. Happy coding and gaming!