Mastering Generics: Avoiding Common Pitfalls in Coding
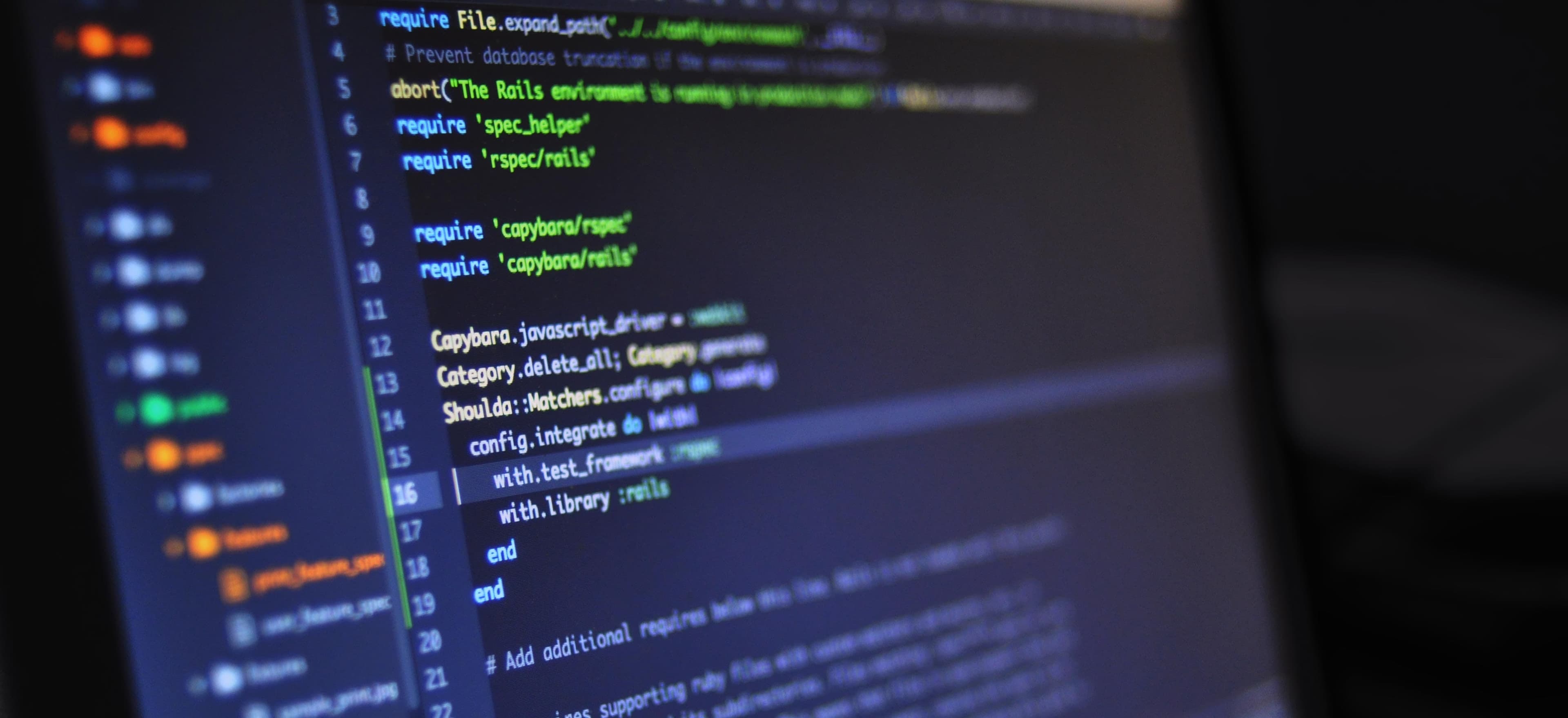
- Published on
Mastering Generics: Avoiding Common Pitfalls in Java Coding
Java generics are a powerful feature that enhances code reusability and type safety. However, they can be a double-edged sword if not used correctly. In this blog post, we're going to explore how to master generics in Java, while also highlighting some common pitfalls you should avoid.
What Are Generics?
Generics allow developers to define classes, interfaces, and methods with a placeholder for types. Instead of specifying a concrete type (like int, String, etc.), you use a type parameter (like T, E, K, V). This means you can create more flexible and reusable components.
public class GenericBox<T> {
private T item;
public void setItem(T item) {
this.item = item;
}
public T getItem() {
return item;
}
}
In this example, GenericBox
can hold any type of object, providing type safety at compile time.
Common Pitfall #1: Type Erasure
One of the primary complexity points with generics in Java is type erasure. Generics are mainly a compile-time feature. The generic type information is erased during runtime to maintain backward compatibility with earlier versions of Java.
Example of Type Erasure
Consider the following code snippet:
List<String> stringList = new ArrayList<>();
stringList.add("Hello");
List<Integer> integerList = new ArrayList<>();
integerList.add(123);
if (stringList.getClass() == integerList.getClass()) {
System.out.println("Both lists are of the same class.");
}
Output: "Both lists are of the same class."
Although stringList
and integerList
contain different types, due to type erasure, both are treated as ArrayList
.
Avoiding the Pitfall
To avoid confusion, remember that you cannot check a generic type during runtime:
public void checkType(List<?> list) {
if (list instanceof List<String>) { // This will not compile
// Do something with String list
}
}
Common Pitfall #2: Raw Types
Raw types refer to using a generic class or interface without a type parameter. While it was widely accepted in earlier Java versions, using raw types can lead to runtime exceptions.
Example of Raw Types
Suppose you define a raw type:
List rawList = new ArrayList();
rawList.add("Test");
rawList.add(123); // No compile-time error, but could lead to ClassCastException later
Avoiding the Pitfall
Always specify the type parameter to ensure type safety:
List<String> safeList = new ArrayList<>();
safeList.add("Test");
// safeList.add(123); // Uncommenting this line will cause a compile-time error
Common Pitfall #3: Wildcards
Wildcards can be tricky. They enable us to define a generics type without specifying an exact type parameter. The wildcard is represented by a question mark (?).
Wildcard Types: Bounded and Unbounded
- Unbounded Wildcards: Denoted by
?
, can represent any type. - Bounded Wildcards:
- Upper-bounded wildcards, represented as
<? extends T>
, are used when you need to read data from a structure. - Lower-bounded wildcards, represented as
<? super T>
, allow you to write data to a structure.
- Upper-bounded wildcards, represented as
Example of Bounded Wildcards
public void printList(List<? extends Number> list) {
for (Number number : list) {
System.out.println(number);
}
}
Here, we can print numbers from any list of types that are subclasses of Number (like Integer, Double, etc.).
Avoiding the Pitfall
When using wildcards, ensure you're clear about whether you're reading from or writing to a collection. Misuse might lead to compile-time errors or missed functionality.
Common Pitfall #4: The Inheritance of Generic Types
One common misconception is that generic types are inherited. If a class is generic, its subclasses do not automatically inherit that property.
Example of Generic Classes
class GenericClass<T> {
T value;
}
class SubClass extends GenericClass<String> {
}
The subclass SubClass
is not generic; it has a concrete type String
, not T.
Avoiding the Pitfall
When creating a class hierarchy using generics, ensure that subclasses are properly defined to maintain type safety if they need to be generic.
Diagnosing Issues in Code using Generics
When errors arise, knowing how to interpret compiler warnings and errors can save significant time. If you receive a type compatibility error, check the following:
- Are raw types being used?
- Is type erasure affecting your implementation?
- Are wildcards correctly bounded?
The Bottom Line
By mastering generics in Java, you can write code that is type-safe, reusable, and maintainable. Understanding common pitfalls associated with generics will save you from runtime headaches and increase your coding efficiency.
For more insights into Java programming practices, consider reading Effective Java by Joshua Bloch. This book provides a wealth of information and practical examples for improving your Java skills.
If you want further exploration into the theoretical underpinnings of generics in Java, check out Java Language Specification.
Happy coding, and may your generics always be type-safe!
Checkout our other articles