Troubleshooting Common SMPP Client Connection Issues
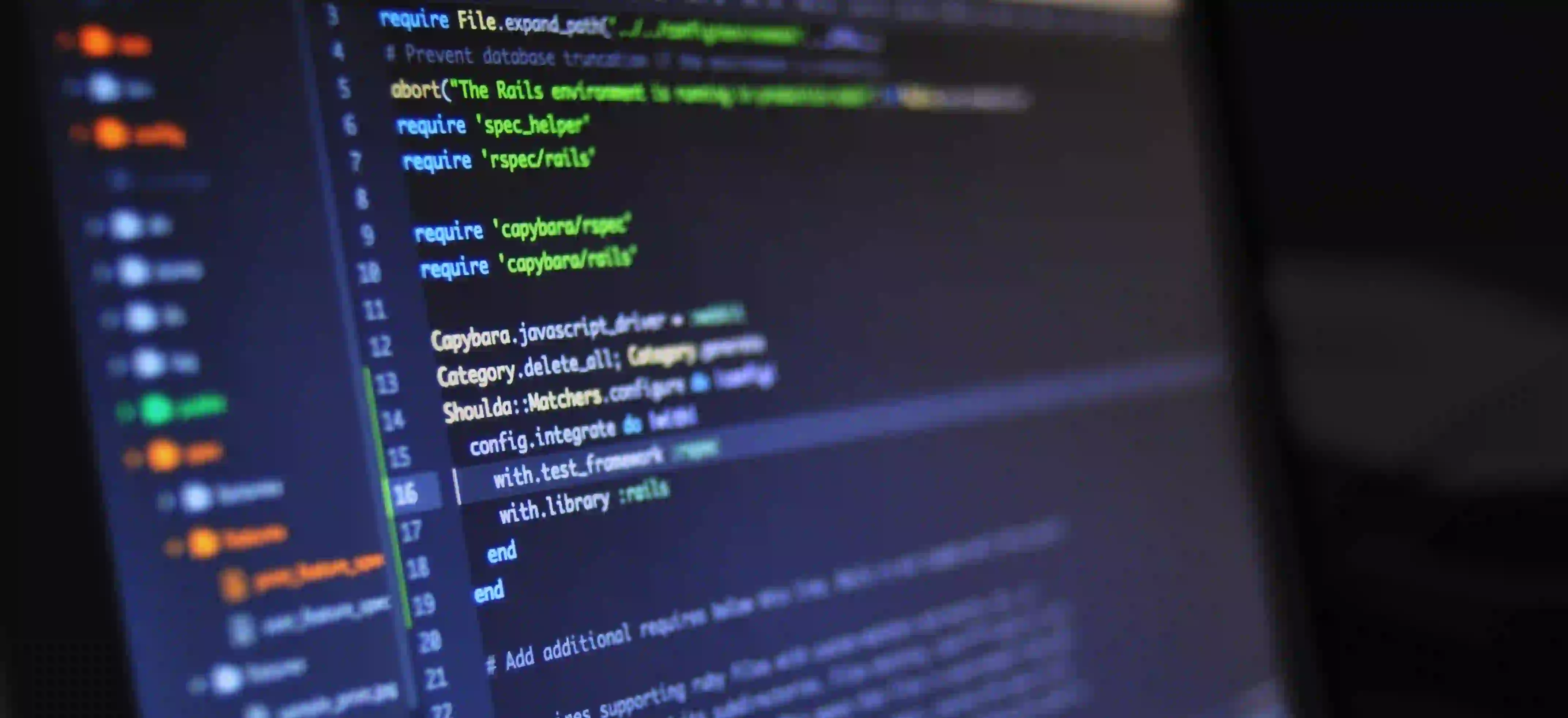
Troubleshooting Common SMPP Client Connection Issues
SMPP (Short Message Peer-to-Peer) is a protocol used by the telecom industry, particularly for sending SMS messages between entities like Short Message Service Centers (SMSC) and external client applications. While it serves as a robust solution for SMS messaging, various connection issues can arise that can impede your messaging service. In this blog post, we will explore common SMPP client connection issues and provide troubleshooting tips, accompanied by code snippets and explanations.
Understanding SMPP
Before diving into troubleshooting, let’s revisit what SMPP is and how it works. SMPP is a telecommunication protocol that allows for the exchange of SMS messages. It supports multiple message formats which can be plain text, binary data, and even Unicode characters.
For developers and businesses looking to implement SMS services through SMPP, connectivity is crucial. Reliable connection management ensures seamless messaging.
Common SMPP Client Connection Issues
While using SMPP, you might face several connection-related challenges. Here are some of the most common issues and how to troubleshoot them.
1. Incorrect Connection Settings
One of the primary reasons for connection failure is incorrect SMPP settings. Variables like host, port, system_id, and password must be correct.
Solution:
Double-check your SMPP configuration. The settings might include:
String host = "your-smpp-server.com";
int port = 2775; // Typically 2775 for SMPP
String systemId = "your_system_id";
String password = "your_password";
Each of these variables should match the credentials provided by your SMS service provider.
2. Firewall or Network Issues
Network issues, particularly firewalls, can block connectivity. Your client application should be able to communicate over the designated ports.
Solution:
Perform the following:
- Ensure that the ports you are using (default is usually 2775) are open.
- Check if your ISP allows for outbound connections on that port.
You can check port accessibility using telnet:
telnet your-smpp-server.com 2775
If the connection fails, the issue resides in your network settings.
3. SMPP Version Mismatch
Different implementations of SMPP can lead to compatibility errors. Ensure that both the client and the server are using the same version of SMPP.
Solution:
Check the SMPP version your client is using. You can typically specify this in your code:
SmppConnectionSettings settings = new SmppConnectionSettings();
settings.setVersion(SmppVersion.V34); // Make sure this matches the server's configuration
Refer to your service provider’s documentation to ascertain the SMPP version they support.
4. Exceeding Connections Limit
Many SMSC providers impose limits on the number of simultaneous connections. Exceeding this number can cause your connection attempts to be rejected.
Solution:
Monitor your connection attempts and ensure that you do not exceed the allowance. You can check your connection count with this snippet:
if (currentConnections >= maxAllowedConnections) {
System.out.println("Max connections limit reached");
// Handle connection limits here
}
Adjust your application to gracefully handle connection limits.
5. Session Timeout
SMPP sessions could time out due to inactivity. Longer delays between sent and received messages can lead to session closure.
Solution:
Consider implementing periodic "keep-alive" messages to maintain your session:
ScheduledExecutorService scheduler = Executors.newScheduledThreadPool(1);
scheduler.scheduleAtFixedRate(() -> {
// Send a no-op or keep-alive message
smppClient.sendNoOp();
}, 0, 30, TimeUnit.SECONDS); // Adjust the interval as necessary
By sending periodic messages, you can prevent sessions from timing out.
6. Incorrect Binding
Binding is the initiation of the connection from the client to the SMSC and is critical for establishing session management. If the binding credentials are wrong, your application will fail to connect.
Solution:
Ensure you bind with the correct parameters:
SmppClient smppClient = new SmppClient();
smppClient.bind(systemId, password, SmppBindType.TRANSCEIVER);
Keep track of the success or failure of the binding operation, and implement retries with exponential back-off if necessary.
7. Protocol Errors
Handling protocol errors requires careful inspection of messages returned by the server. If your application receives a negative acknowledgment (NAK), you'll need to diagnose why.
Solution:
Implement error handling for your operations:
try {
smppClient.sendMessage(message);
} catch (SmppException e) {
// Log the error
System.err.println("Failed to send message: " + e.getMessage());
// Handle retries, etc.
}
Review logs for specific error codes that the SMPP server returns. Each code provides insight into what went wrong.
Bringing It All Together
SMPP connection issues can be challenging but are manageable with a proactive approach. By understanding common issues such as incorrect settings, network problems, version mismatches, exceeding connections, session timeouts, and binding errors, you can effectively troubleshoot and maintain a successful SMS messaging service.
Further Reading
For additional resources and deeper insights into SMPP and message handling:
- SMPP Protocol Specifications
- Java SMPP Client Libraries
With patience and strategic troubleshooting, you can ensure robust and reliable SMS messaging for your applications. Happy coding!