Resolving SLF4J Compatibility Issues with Logger Implementations
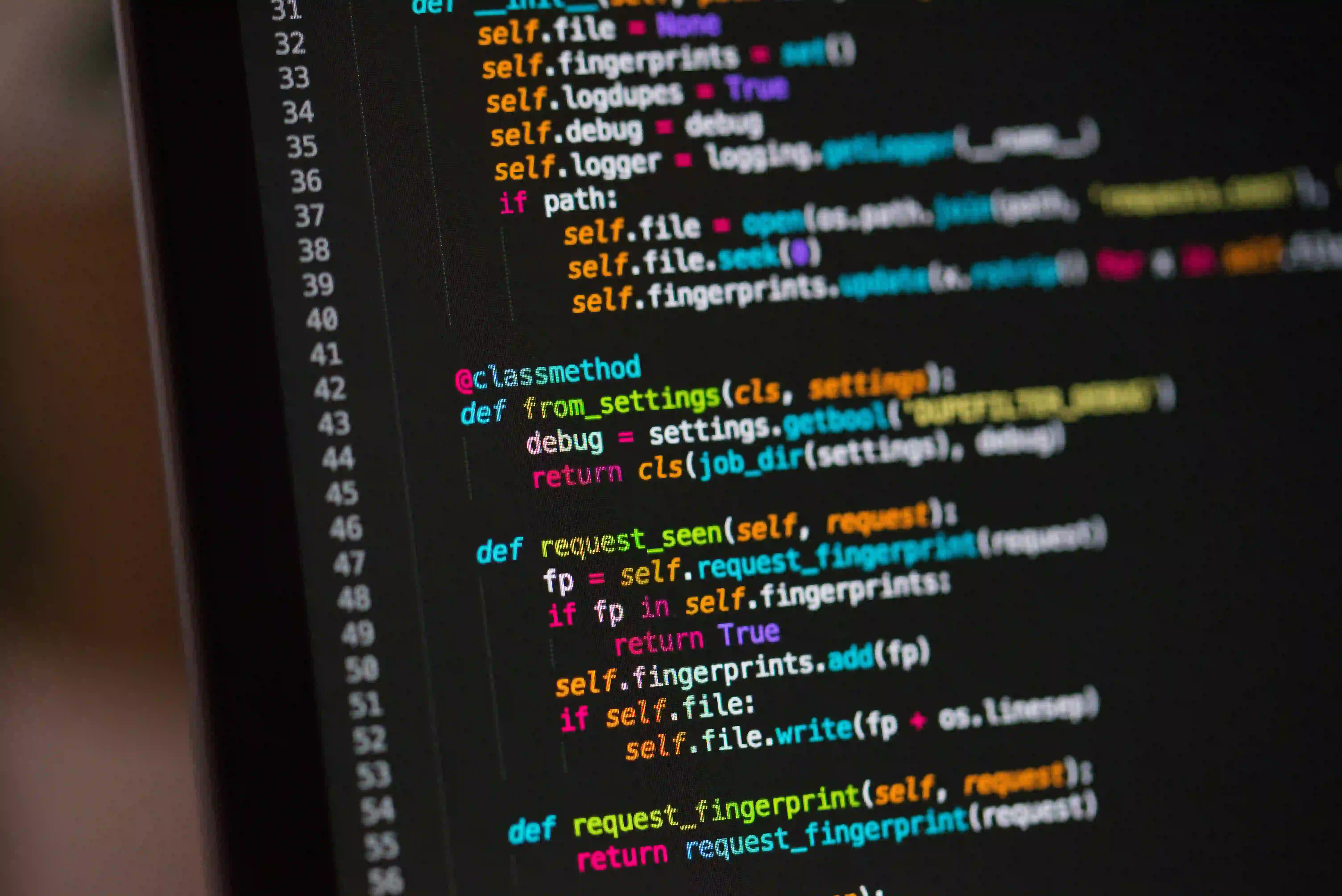
Resolving SLF4J Compatibility Issues with Logger Implementations
When developing Java applications, logging is an essential part of maintaining and debugging your code. One of the most popular logging frameworks used in conjunction with Java is SLF4J (Simple Logging Facade for Java). SLF4J allows developers to plug in different logging frameworks, such as Logback, Log4j, or Java Util Logging, while keeping a consistent interface. However, working with SLF4J can sometimes lead to compatibility issues with the underlying logging implementations. This blog post will explore common SLF4J compatibility problems and how to resolve them, making your logging experience seamless.
Table of Contents
- What is SLF4J?
- Common Compatibility Issues
- Resolving Compatibility Issues
- Best Practices for Loggers
- Conclusion
What is SLF4J?
SLF4J serves as a simple facade or abstraction for various logging frameworks. It allows developers to decouple their application logging from a specific logging implementation, providing flexibility to swap in different libraries as needed. For a deeper understanding of SLF4J, you can refer to the official SLF4J documentation.
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
public class MyApplication {
private static final Logger logger = LoggerFactory.getLogger(MyApplication.class);
public static void main(String[] args) {
logger.info("Hello from SLF4J!");
}
}
In the code above, we use LoggerFactory
to create a logger instance. This enables logging messages without being tied to a specific logging framework.
Common Compatibility Issues
While SLF4J simplifies logging, it can also introduce challenges, particularly when integrating with various logging implementations. Here are some common issues you may encounter:
1. Classpath Conflicts
Class conflicts arise when multiple libraries include different versions of the same SLF4J API or logging implementations. This can lead to NoClassDefFoundError
or NoSuchMethodError
.
2. Bridge Implementation Conflicts
When you use SLF4J with implementations like Log4j or Logback, you may inadvertently include incompatible bridge implementations. This can lead to unexpected logging behavior.
3. Multiple SLF4J Bindings
Having multiple SLF4J bindings on your classpath can result in runtime errors. SLF4J generally only allows one binding; having more than one can lead to unpredictable logging outputs.
4. Missing Dependencies
Sometimes, a logging implementation may require additional dependencies that are not automatically included, resulting in runtime issues.
Resolving Compatibility Issues
Now that we have identified common compatibility issues, let's explore methods to resolve these problems.
1. Use Dependency Management Tools
One effective way to manage your dependencies and avoid conflicts is by using build tools like Maven or Gradle. These tools allow you to specify the logging framework and its version, ensuring that you are using compatible versions.
Maven Example
Hereβs an example of how to configure SLF4J with Logback in your pom.xml
:
<dependencies>
<!-- SLF4J API -->
<dependency>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-api</artifactId>
<version>1.7.32</version>
</dependency>
<!-- Logback Classic -->
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-classic</artifactId>
<version>1.2.6</version>
</dependency>
</dependencies>
2. Exclude Unwanted Dependencies
In cases where your projects or their dependencies pull in conflicting versions of SLF4J, you might want to exclude the unwanted versions. This can also be done easily with Maven.
Maven Exclusion Example
<dependency>
<groupId>com.example</groupId>
<artifactId>some-library</artifactId>
<version>1.0</version>
<exclusions>
<exclusion>
<groupId>org.slf4j</groupId>
<artifactId>slf4j-log4j12</artifactId>
</exclusion>
</exclusions>
</dependency>
3. Verify Single Binding
Make sure that your project has exactly one SLF4J binding. You can check this in your pom.xml
or build.gradle
. Conflicting bindings can cause runtime errors or inconsistent logging output.
4. Additional Dependencies
Make sure to include all necessary dependencies required by your chosen logging framework. For instance, if using Logback, it requires at least SLF4J and the Logback core. Check the Logback documentation for a comprehensive list.
<dependency>
<groupId>ch.qos.logback</groupId>
<artifactId>logback-core</artifactId>
<version>1.2.6</version>
</dependency>
Best Practices for Loggers
Following best practices can significantly reduce compatibility issues. Here are a few recommendations:
1. Maintain Dependency Consistency
Ensure that all your dependencies are compatible regarding versions. Use tools like Maven Enforcer Plugin to enforce rules on dependency versions.
2. Use a Consistent Logging Framework
Choose a logging framework and stick with it across your entire project. While SLF4J allows for flexibility, mixing multiple logging frameworks can lead to confusion and maintenance overhead.
3. Regularly Update Dependencies
Libraries and frameworks evolve. Regularly check for updates to your dependencies and ensure they are compatible. Use tools like Dependabot to help manage versioning.
4. Monitor Application Logs
Monitor your logs to identify any unusual behaviors or errors related to logging. This can help you catch issues that stem from compatibility problems early on.
A Final Look
SLF4J is a powerful logging facade that simplifies the way we handle logging in Java applications. However, as we've explored, it can introduce complexity, especially concerning compatibility with various logging implementations. By understanding common issues and implementing best practices for dependency management, you can mitigate the risks associated with SLF4J.
Staying proactive with logging not only aids in debugging but also maintains the overall health of your application. For more information on SLF4J and its usage, check out the SLF4J FAQ.
Happy logging!