Overcoming Common Java Microservices Challenges in Development
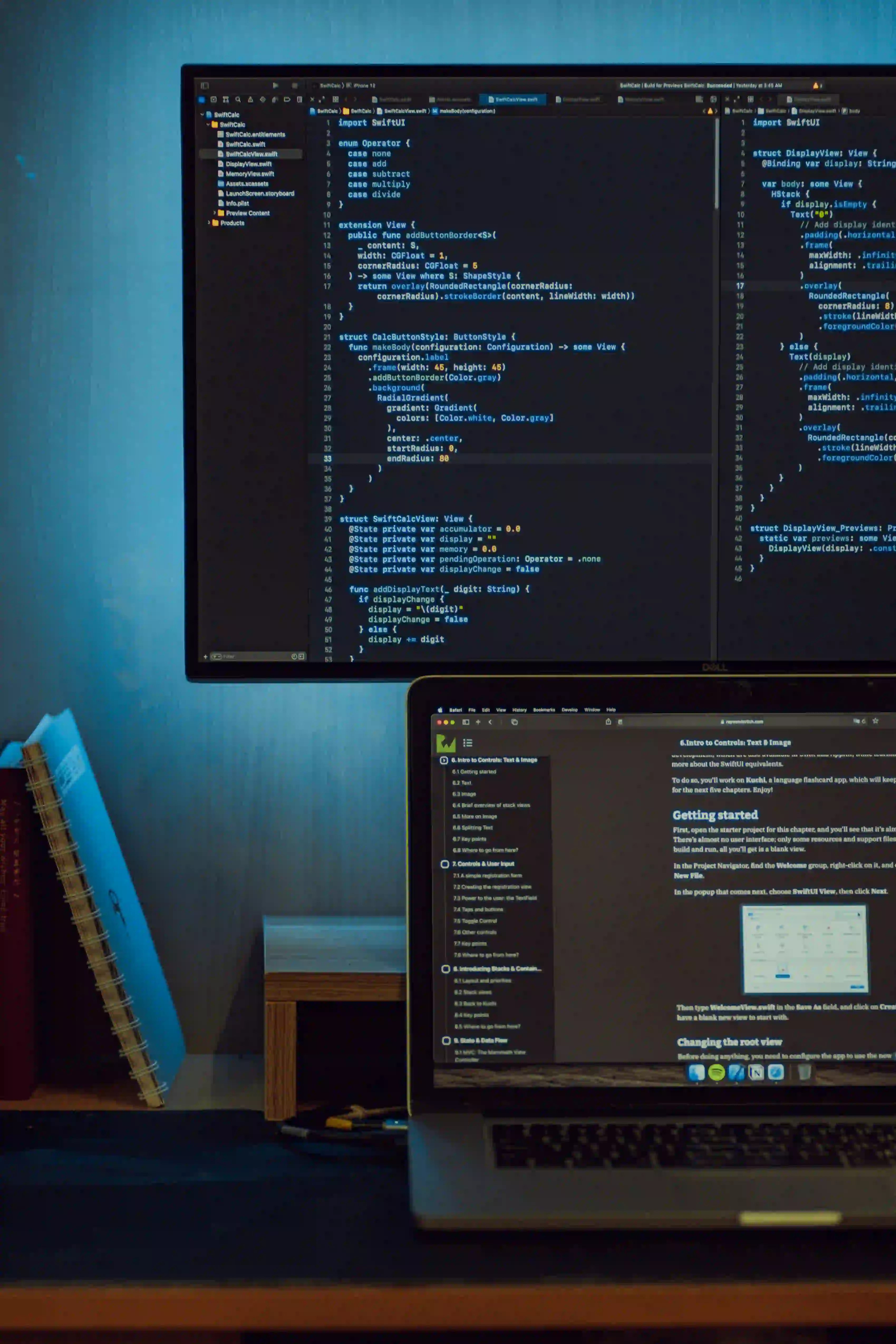
Overcoming Common Java Microservices Challenges in Development
Java microservices architecture has gained popularity among developers for its ability to create scalable and maintainable applications. However, despite its advantages, developers often face several challenges in its implementation. This blog post explores these common challenges and how to overcome them effectively.
Table of Contents
- Understanding Microservices
- Challenge 1: Service Communication
- Challenge 2: Data Management
- Challenge 3: Configuration Management
- Challenge 4: Monitoring and Debugging
- Challenge 5: Deployment Complexity
- Conclusion
Understanding Microservices
Microservices architecture involves building applications as a collection of loosely coupled services. Each service focuses on a specific business capability and communicates over a network. This approach contrasts with traditional monolithic architectures, where applications are built as a single, tightly coupled unit.
Java is an excellent choice for implementing microservices due to its scalability, robustness, and rich set of frameworks. However, leveraging these benefits comes with its own set of challenges.
Challenge 1: Service Communication
The Problem
Services in a microservices architecture need to communicate effectively. This can happen synchronously or asynchronously, and it introduces complexities in terms of latency, fault tolerance, and protocol handling.
Solution
Utilizing API gateways and service meshes can solve this issue. An API Gateway acts as a single entry point for all client requests, serving as a proxy to route requests to the appropriate microservices.
Here is a basic implementation using Spring Cloud Gateway:
import org.springframework.cloud.gateway.route.RouteLocator;
import org.springframework.cloud.gateway.route.builder.RouteLocatorBuilder;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
@Configuration
public class GatewayConfig {
@Bean
public RouteLocator customRouteLocator(RouteLocatorBuilder builder) {
return builder.routes()
.route("service1", r -> r.path("/service1/**")
.uri("http://localhost:8081"))
.route("service2", r -> r.path("/service2/**")
.uri("http://localhost:8082"))
.build();
}
}
Why This Works
The Spring Cloud Gateway simplifies routing and provides a central point to handle cross-cutting concerns like authentication and monitoring. This setup allows easy scalability as services can evolve independently.
For more information on API Gateway patterns, you can refer to Microservices.io.
Challenge 2: Data Management
The Problem
Each microservice typically manages its own data source. This can lead to data consistency challenges, especially in distributed systems where transactions span multiple services.
Solution
One approach to manage data across microservices is the Saga pattern, which breaks a large transaction into smaller, manageable ones, handled by individual services.
Here's an outline of how to implement a simple saga using Spring Boot:
@Service
public class OrderService {
@Transactional
public Order createOrder(Order order) {
// Step 1: Create Order
Order savedOrder = orderRepository.save(order);
// Step 2: Make Payment
paymentService.processPayment(savedOrder);
// Step 3: Ship Order
shipmentService.scheduleShipment(savedOrder);
return savedOrder;
}
}
Why This Works
Implementing transactions asynchronously allows services to remain decoupled while still ensuring eventual consistency. If any step in the process fails, compensating actions can be triggered to maintain data integrity.
To dive deeper into Saga patterns, check out Martin Fowler's website.
Challenge 3: Configuration Management
The Problem
Managing configurations across multiple microservices can become cumbersome, particularly when dealing with different environments (development, testing, production).
Solution
Utilizing a centralized configuration server helps manage configurations effectively. Spring Cloud Config provides a server and client for this purpose.
Here’s how you can set it up in your Spring Boot application:
@SpringBootApplication
@EnableConfigServer
public class ConfigServerApplication {
public static void main(String[] args) {
SpringApplication.run(ConfigServerApplication.class, args);
}
}
Why This Works
By externalizing configurations, services can be updated without the need for redeployment. Dynamic configurations lead to more flexible service management.
You can find more about configuration management in microservices here.
Challenge 4: Monitoring and Debugging
The Problem
In a distributed system, monitoring and debugging become critical. Lack of visibility can cause significant downtime and impact user experience.
Solution
Incorporating tools like Spring Boot Actuator, coupled with distributed tracing mechanisms such as Zipkin or Jaeger, allows developers to collect metrics, monitor service health, and trace requests across microservices.
A minimal setup to enable Spring Boot Actuator is straightforward:
@SpringBootApplication
@EnableActuator
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
Why This Works
Actuator provides a range of built-in endpoints to check the status and metrics of your application. Paired with distributed tracing tools, it can illuminate the interaction paths between services, making debugging much more manageable.
Challenge 5: Deployment Complexity
The Problem
As the number of microservices grows, so does the complexity of managing deployments. Continuous integration and deployment are critical but can be challenging.
Solution
Using container orchestration platforms like Kubernetes can streamline this process. Kubernetes manages the deployment, scaling, and operation of application containers across clusters of hosts.
Here’s a simple deployment YAML for a Java microservice:
apiVersion: apps/v1
kind: Deployment
metadata:
name: my-java-app
spec:
replicas: 3
selector:
matchLabels:
app: my-java-app
template:
metadata:
labels:
app: my-java-app
spec:
containers:
- name: my-java-app
image: my-java-app:latest
ports:
- containerPort: 8080
Why This Works
Kubernetes automates the deployment and scaling of services, allowing developers to focus on coding instead of worrying about the underlying infrastructure. In a microservices environment, it simplifies service discovery, load balancing, and resource management.
Wrapping Up
Microservices architecture offers numerous benefits but also presents distinct challenges during development. Understanding and addressing these issues can significantly enhance the efficiency of your Java microservices applications. By implementing solutions such as API Gateways, the Saga pattern, centralized configuration management, robust monitoring, and Kubernetes orchestration, developers can streamline their microservices' architecture and improve overall application performance.
If you are a developer stepping into the world of microservices or looking to improve your existing setup, embracing these concepts will accompany you on your journey to mastering Java microservices.
Further Reading:
By overcoming these challenges, you can enjoy the full potential of microservices while leveraging the power of Java. Happy coding!