Common Pitfalls in Pub/Sub Apps with MongoDB
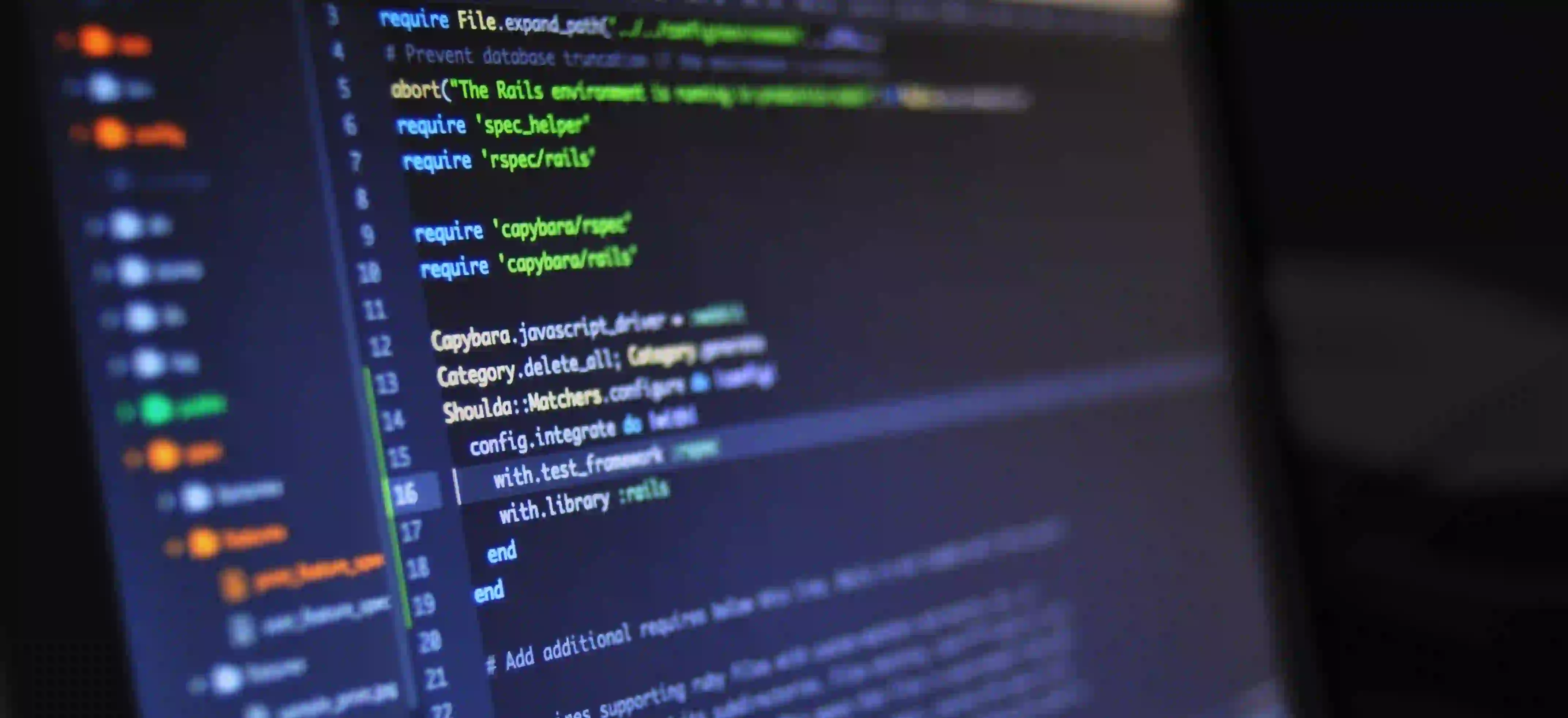
Common Pitfalls in Pub/Sub Apps with MongoDB
Creating a pub/sub (publish/subscribe) application using MongoDB can be an exhilarating challenge, but it is also fraught with pitfalls. In this post, we will discuss common issues that developers face in pub/sub architectures when using MongoDB. We will cover how to avoid these pitfalls and enhance your application’s scalability, performance, and reliability.
Understanding Pub/Sub Architecture
Before diving into potential pitfalls, let's first clarify what pub/sub architecture is. At its core, a pub/sub system consists of two main components: publishers and subscribers.
- Publishers send messages or events to a message broker.
- Subscribers receive messages based on their interests.
Using MongoDB as the backend for your pub/sub application can offer advantages such as flexibility and scalability, but the following pitfalls often arise.
Pitfall 1: Lack of Indexing
Why Indexing Matters
One of the significant challenges in a pub/sub setup is handling message throughput. If you're storing messages in a collection, querying those messages without proper indexing can create performance bottlenecks, especially as your application scales.
Solution
Ensure that you index relevant fields such as message timestamps, categories, or subject IDs. For example, assuming you have a messages
collection:
db.messages.createIndex({ "timestamp": 1 })
This index allows quick retrieval of messages based on their timestamps, improving performance in read-heavy scenarios.
Pitfall 2: Inefficient Message Retrieval
The Issue
After deploying your pub/sub application, you might find that retrieving messages for subscribers becomes increasingly slow, especially with a growing message history.
Solution
Consider using aggregation pipelines to optimize how messages are retrieved. Aggregation provides a powerful way to transform and curate messages before they reach the subscriber. For example, if you want to retrieve messages within a certain date range, you could leverage an aggregation query:
List<Document> results = messagesCollection.aggregate(Arrays.asList(
new Document("$match",
new Document("timestamp",
new Document("$gte", startDate)
.append("$lte", endDate))),
new Document("$sort",
new Document("timestamp", 1))
)).into(new ArrayList<>());
This snippet will efficiently filter messages between two dates, improving retrieval performance.
Pitfall 3: High Latency in Message Delivery
Understanding Latency
In a pub/sub system, latency—that is, the time it takes for a message to travel from the publisher to the subscriber—can have a significant impact on user experience.
Solution
To minimize latency, consider batching messages. Instead of sending individual messages immediately, aggregate them and send a batch periodically. For example:
public void sendMessages(List<Message> messages) {
if(messages.size() >= BATCH_SIZE) {
// Code to publish messages
}
}
By batching messages, overall network latency can be reduced, leading to a smoother experience for subscribers.
Pitfall 4: Unmanaged Consumer Scale
The Problem
As your application grows, you may have more consumers subscribing to the same topic. Each additional consumer can lead to increased load on the database and can amplify any efficiency issues present in your current setup.
Solution
Implement a strategy to manage the scale of consumers effectively. Consider using a message queue system in front of your MongoDB database to offload real-time message passing. Tools like RabbitMQ or Apache Kafka can serve as excellent intermediaries. This separation allows you to manage consumer scale without overwhelming your MongoDB instance.
Pitfall 5: Message Duplication
Recognizing Duplication
In distributed systems, message duplication can often occur. Once a message is published, there’s a risk that it gets sent multiple times to a subscriber.
Solution
To avoid message duplication, implement idempotency within your message processing logic. Each message should have a unique identifier. This way, subscribers can filter out duplicate messages if needed.
For example:
public void processMessage(Message message) {
if (processedMessages.contains(message.getId())) {
return; // Message has already been processed
}
// Process the message
processedMessages.add(message.getId());
}
This simple check helps ensure that no message will be processed more than once.
Pitfall 6: Failure to Scale Reads and Writes
The Challenge
A common misconception is that if a database scales, it automatically handles both reads and writes efficiently. This is not always the case with MongoDB.
Solution
To manage this effectively, consider using a sharding strategy. MongoDB supports sharding, allowing you to partition your data across multiple servers. This way, both read and write loads can be distributed effectively.
To set up sharding in MongoDB, you can use the following command:
sh.enableSharding("myDatabase")
sh.shardCollection("myDatabase.messages", { "userId": 1 })
This command enables sharding on the messages collection, indicating that it should be partitioned based on the user ID.
Pitfall 7: Ignoring Security Measures
Security Risks
Whenever you are handling messages and user data, security should be a priority. A lack of proper access controls can lead to data breaches.
Solution
Ensure that your MongoDB instance is secured with proper authentication and role-based access controls. For instance:
use admin
db.createUser(
{
user: "appUser",
pwd: "password",
roles: [ { role: "readWrite", db: "myDatabase" } ]
}
)
This command creates a user with read/write access only to the specific database, minimizing security risks.
The Last Word
In summary, while building pub/sub applications with MongoDB presents unique opportunities, it also involves navigating several potential pitfalls. Proper indexing, efficient message retrieval, managing consumer scale, avoiding duplication, scaling effectively, and implementing robust security measures are all critical areas to focus on.
By addressing these common issues, you set the stage for a more efficient, reliable, and scalable pub/sub application.
For further reading on optimizing MongoDB queries, check out the MongoDB Documentation. If you’re interested in learning more about the pub/sub architecture, visit Confluent's Introduction to Pub/Sub. Happy coding!