Fixing the GC Overhead Limit Exceeded Error in Java
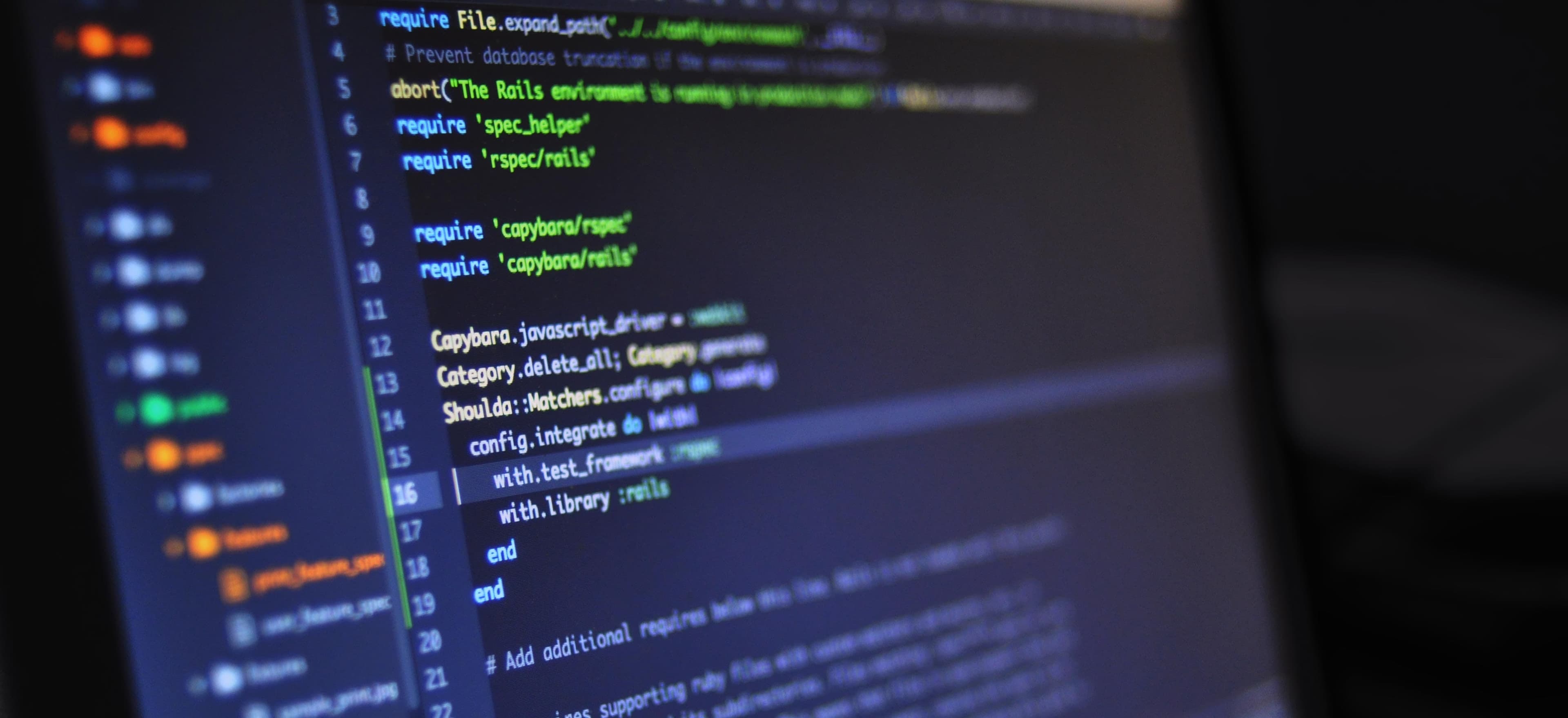
- Published on
Fixing the GC Overhead Limit Exceeded Error in Java
Java developers often encounter a variety of challenges in their coding journeys, one of which is the dreaded "GC overhead limit exceeded" error. This can be particularly frustrating when it happens during application runtime, causing unexpected application crashes. In this blog post, we will dive deep into what the error is, why it occurs, and how to effectively fix it.
Understanding the GC Overhead Limit Exceeded Error
The "GC overhead limit exceeded" error is a runtime exception that occurs when the Java Virtual Machine (JVM) spends too much time performing garbage collection (GC) and recovers very little memory as a result. Here are some key points to understand:
-
Garbage Collection in Java: Java uses automated garbage collection to manage memory. The garbage collector is responsible for clearing objects that are no longer in use, thus freeing up memory.
-
When the Error Occurs: The error occurs when the JVM has spent more than 98% of its time performing GC but has reclaimed less than 2% of the heap memory. This typically signifies that the application is running out of memory and that the GC is doing its job, but is unable to free up enough resources for the application to continue smoothly.
-
How to Identify It: You will usually see an error message similar to the following when the issue arises:
Exception in thread "main" java.lang.OutOfMemoryError: GC overhead limit exceeded
Common Causes
Identifying the root cause of the error is crucial for effective resolution. Some common causes include:
- Memory Leaks: Unintentionally retaining references to objects that should be collected can lead to memory leaks.
- Insufficient Heap Size: If the allocated heap size is too low for the application’s workload, it may quickly run out of memory.
- Inefficient Algorithms: Some algorithms consume excessive memory as they may be holding onto more objects in memory than necessary.
Solutions to Fix the Error
Let’s explore a variety of methods to fix the GC overhead limit exceeded error.
1. Increase the Heap Size
One of the quickest fixes for this issue is to increase the Java heap size allocated to the JVM. Java has default settings, but these can be adjusted based on your application requirements.
You can change the heap size using the following JVM options:
java -Xms512m -Xmx4g -jar yourapp.jar
-Xms
sets the initial heap size (512 MB in this case).-Xmx
sets the maximum heap size (4 GB).
By increasing these values, you allow your application more memory to work with, potentially alleviating the issue.
2. Optimize Your Code
Always strive to write efficient code. Let’s examine a common case where we could encounter memory issues.
Consider the following example where a list continually grows due to improper use:
import java.util.ArrayList;
import java.util.List;
public class MemoryLeakExample {
public static void main(String[] args) {
List<Object> objects = new ArrayList<>();
while (true) {
objects.add(new Object()); // Memory leak: continuously grows in size
}
}
}
Fixing the Memory Leak
One of the best practices is to ensure that data structures are properly cleared or reused:
import java.util.ArrayList;
import java.util.List;
public class FixedExample {
public static void main(String[] args) {
List<Object> objects = new ArrayList<>();
while (true) {
if (objects.size() < 1000) {
objects.add(new Object()); // Use a cap on the size
} else {
objects.clear(); // Clear the list to prevent memory leak
}
}
}
}
By using objects.clear()
, we can ensure that we do not exceed our memory limits, thus avoiding the error.
3. Analyze Memory Usage
Tools like Java VisualVM or Eclipse Memory Analyzer (MAT) can help you analyze memory usage and detect memory leaks.
Using Java VisualVM for Memory Analysis
- Run your application with Java VisualVM.
- Click on your application in the list.
- Go to the Monitor tab to observe real-time memory usage.
- Use the Sampler tab to observe which objects consume the most memory.
By analyzing these metrics, you can identify objects that are not being de-referenced appropriately and fix memory leaks accordingly.
4. Review Third-Party Libraries
Sometimes, memory issues arise from libraries that do not manage memory effectively. Be sure to:
- Stay Updated: Use the latest stable versions of libraries, as they often contain fixes for memory-related liabilities.
- Read Documentation: Understand any potential memory management issues that could affect your application.
5. Adjust the GC Settings
You can adjust the frequency and behavior of the garbage collector in the JVM. For example, switching the GC algorithm can yield better performance:
java -XX:+UseG1GC -jar yourapp.jar
The G1 (Garbage First) garbage collector is often better for applications with large heaps and can help mitigate GC overhead issues by collecting smaller regions of the heap and reducing long pauses.
My Closing Thoughts on the Matter
Dealing with the "GC overhead limit exceeded" error may seem intimidating at first glance, but thorough understanding and systematic troubleshooting can lead to effective solutions. By increasing heap sizes, optimizing code, analyzing memory usage, reviewing libraries, and tuning GC settings, you can significantly reduce the chances of encountering this error.
Understanding how Java's garbage collection works will empower you to write better, more efficient code and manage memory more effectively. For more information, you can refer to the official Java documentation on Garbage Collection.
By being proactive and vigilant about memory management in your applications, you can ensure smoother, more reliable performance while enhancing your overall Java programming proficiency. Happy coding!
Checkout our other articles