Troubleshooting Common AWS SQS Issues in Java Applications
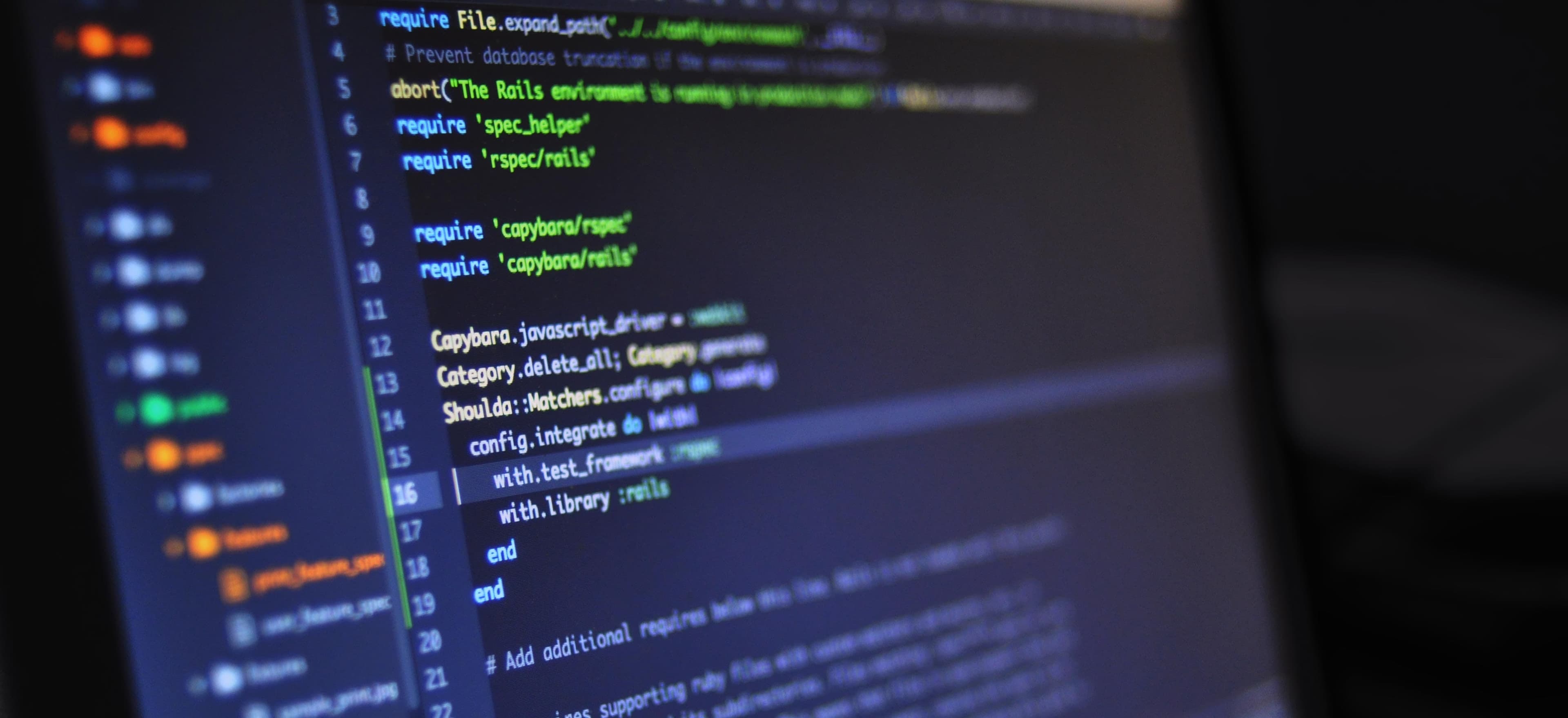
- Published on
Troubleshooting Common AWS SQS Issues in Java Applications
Amazon Simple Queue Service (SQS) is a fully managed message queuing service that enables you to decouple and scale microservices, distributed systems, and serverless applications. While using SQS in your Java applications can significantly improve your architecture, there are common issues you might face. This blog post will discuss several of these issues and their resolutions, thereby helping you maintain a smooth operation of your Java applications that rely on AWS SQS.
Why Use AWS SQS in Your Java Applications?
Before we dive deep into troubleshooting, let's understand the advantages of using SQS with Java.
- Decoupling Components: SQS enables you to decouple components of your applications which can lead to better scalability and maintenance.
- Reliability: SQS offers a reliable way to send and receive messages through its multiple redundancy and high availability features.
- Scalability: As your application grows, SQS can manage and handle any traffic fluctuations seamlessly.
Common Issues with AWS SQS in Java
Here, we will explore the most prevalent issues with Amazon SQS when using it within Java applications, followed by solutions and best practices.
1. Message Visibility Timeout Errors
One common issue is when messages are not being processed properly due to Visibility Timeout errors. Visibility Timeout is a period of time during which a message is invisible to other consumers.
Issue: When a consumer retrieves a message but fails to process it within the visibility timeout period, the message becomes visible again, potentially causing duplicates.
Solution:
To ensure that messages are processed within the visibility timeout, you can increase the timeout value. For example, you might set the visibility timeout to 30 seconds if your processing time usually takes about 15 seconds:
import com.amazonaws.services.sqs.AmazonSQS;
import com.amazonaws.services.sqs.AmazonSQSClientBuilder;
import com.amazonaws.services.sqs.model.ChangeMessageVisibilityRequest;
public void changeVisibility(String queueUrl, String receiptHandle) {
AmazonSQS sqs = AmazonSQSClientBuilder.defaultClient();
ChangeMessageVisibilityRequest changeMessageVisibilityRequest =
new ChangeMessageVisibilityRequest(queueUrl, receiptHandle, 30); // Time is in seconds
sqs.changeMessageVisibility(changeMessageVisibilityRequest);
}
By adjusting the visibility timeout, you can ensure that your messages are adequately processed without becoming available to other consumers prematurely.
2. Message Delivery Delays
Another issue frequently encountered is the unpredictability of message delivery times.
Issue: Sometimes, applications might experience message delay resulting in slower processing.
Solution:
When messages are sent to the SQS Queue, it’s important to understand that SQS does not guarantee immediate delivery, especially in high traffic situations. You can implement exponential backoff strategies to handle these delays, allowing your application to retry message retrieval at increasing intervals until successful.
Here is a simple example of exponential backoff in Java:
public void receiveMessagesWithBackoff(String queueUrl) {
AmazonSQS sqs = AmazonSQSClientBuilder.defaultClient();
for (int attempt = 1; attempt <= MAX_ATTEMPTS; attempt++) {
try {
List<Message> messages = sqs.receiveMessage(queueUrl).getMessages();
// process messages
break; // exit loop if successful
} catch (Exception e) {
int waitTime = (int) Math.pow(2, attempt); // exponentially increasing wait time
try {
Thread.sleep(waitTime * 1000); // wait before next attempt
} catch (InterruptedException ie) {
Thread.currentThread().interrupt();
break;
}
}
}
}
By implementing an exponential backoff, your application can handle heavy load scenarios better with less risk of overwhelming the service.
3. Out of Order Messages
While SQS provides a reliable message delivery infrastructure, it does not guarantee the order of message delivery in standard queues.
Issue: If maintaining order is crucial, you may encounter out-of-order processing challenges.
Solution:
Use FIFO (First-In-First-Out) queues in SQS, which are designed to ensure that messages are processed exactly once in the order they are sent. To use FIFO queues in Java, prefix the queue name with .fifo
and ensure to provide a MessageGroupId
for ordered messages.
A simple producer example using FIFO queues:
import com.amazonaws.services.sqs.AmazonSQS;
import com.amazonaws.services.sqs.AmazonSQSClientBuilder;
import com.amazonaws.services.sqs.model.SendMessageRequest;
public void sendFifoMessage(String queueUrl, String messageBody, String messageGroupId) {
AmazonSQS sqs = AmazonSQSClientBuilder.defaultClient();
SendMessageRequest sendRequest = new SendMessageRequest()
.withQueueUrl(queueUrl)
.withMessageBody(messageBody)
.withMessageGroupId(messageGroupId) // Ensures ordering
.withMessageDeduplicationId(UUID.randomUUID().toString()); // Unique id for deduplication
sqs.sendMessage(sendRequest);
}
By leveraging FIFO queues, you can ensure the right order of message processing.
4. Insufficient Permissions
Issues related to AWS permissions are also a common source of errors in Java applications using SQS.
Issue: Your application may fail to send or receive messages due to insufficient IAM permissions.
Solution:
Ensure that your IAM user or role has the proper permissions. You can attach a policy like the following:
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"sqs:SendMessage",
"sqs:ReceiveMessage",
"sqs:DeleteMessage",
"sqs:ChangeMessageVisibility"
],
"Resource": "arn:aws:sqs:us-east-1:123456789012:YourQueueName"
}
]
}
By granting the required permissions, your application will be able to interact with the SQS queue as expected.
5. Handling Large Messages
SQS has a message size limit of 256 KB. Exceeding this limit causes issues.
Issue: Attempting to send messages larger than the limit will result in failures.
Solution:
Consider using Amazon S3 for larger messages. Store the message in an S3 bucket and send the S3 URL as a message body to SQS.
Here’s a simplified example:
import com.amazonaws.services.sqs.AmazonSQS;
import com.amazonaws.services.sqs.AmazonSQSClientBuilder;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3ClientBuilder;
public void sendLargeMessage(String s3Bucket, String s3Key, String queueUrl) {
AmazonS3 s3 = AmazonS3ClientBuilder.defaultClient();
String messageUrl = s3.getUrl(s3Bucket, s3Key).toString();
AmazonSQS sqs = AmazonSQSClientBuilder.defaultClient();
sqs.sendMessage(queueUrl, messageUrl);
}
This way, your messages remain within the SQS limits while still allowing you to process larger data.
Wrapping Up
In this blog post, we have discussed several common AWS SQS issues encountered in Java applications, including message visibility timeouts, message delivery delays, out-of-order messages, insufficient permissions, and handling large messages. Each section provided actionable solutions to help you troubleshoot effectively.
By applying these practices and solutions, you can enhance your application's reliability and robustness in AWS environments. For more information on AWS SQS, consider checking the AWS SQS Developer Guide.
Harnessing the power of SQS can lead to more efficient and scalable applications, ultimately contributing to a better user experience and operational effectiveness. Happy coding!
Checkout our other articles