Solving Dependency Conflicts in Gradle Multi-Project Builds
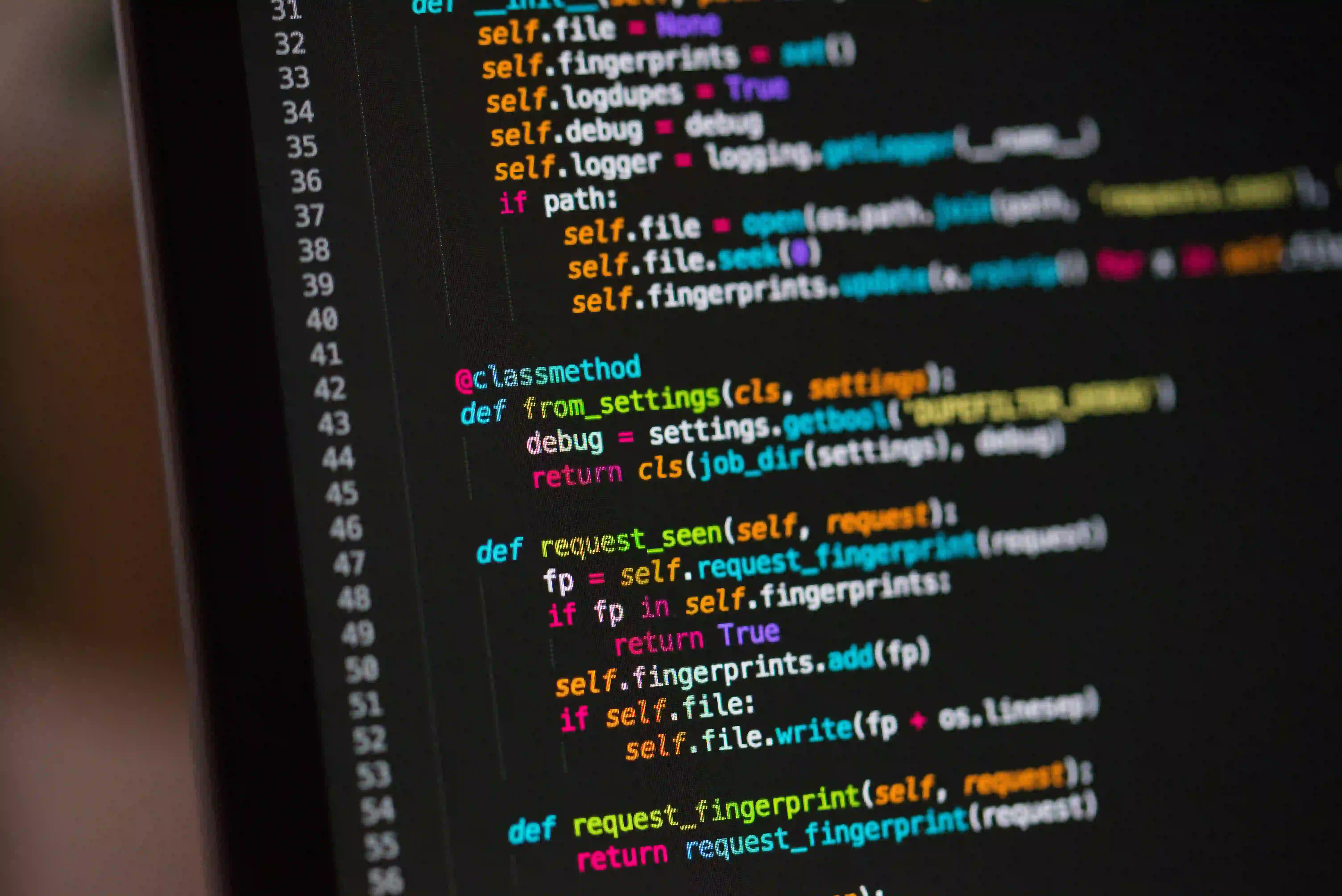
Solving Dependency Conflicts in Gradle Multi-Project Builds
When working with Gradle multi-project builds, developers often encounter dependency conflicts. These conflicts arise when multiple projects depend on different versions of the same library or module. Resolving these conflicts efficiently is crucial for maintaining project stability and ensuring smooth development. In this blog post, we’ll explore how to identify and resolve these conflicts using Gradle, along with practical code snippets and explanations.
Understanding Dependency Management in Gradle
Gradle employs a powerful dependency management system, which allows developers to specify the libraries and versions their projects depend on. In a multi-project build setup, each subproject can have its own build.gradle
file and define its dependencies.
Why Dependency Conflicts Arise
Dependency conflicts typically arise due to:
- Transitive Dependencies: When libraries themselves have dependencies on other libraries, it can lead to multiple versions of the same library being included in your project.
- Outdated Dependencies: If different subprojects rely on outdated versions, Gradle needs to resolve which version to include.
- Manual Version Specifications: Occasionally, developers might manually specify different versions of the same library in separate projects.
The Impact of Conflicts
At best, dependency conflicts can lead to warnings or errors during compilation. At worst, they can cause runtime issues, unexpected behaviors, or application crashes.
Identifying Dependency Conflicts
Before resolving conflicts, it's essential to identify them. Gradle provides a command to display the dependency tree of a project.
./gradlew dependencies
This command will output a detailed structure of your project's dependencies. In multi-project builds, you can run it per subproject by navigating to each subproject directory.
Example Dependency Output
You might see output like this:
+--- com.google.guava:guava:28.1-jre
| +--- org.checkerframework:checker-qual:2.5.0
| \--- com.google.guava:guava-parent:28.1-jre
+--- org.apache.httpcomponents:httpclient:4.5.13
| +--- org.apache.httpcomponents:httpcore:4.4.13
| +--- commons-logging:commons-logging:1.2
| \--- com.google.guava:guava:27.0.1-jre
\--- some.other:library:1.0
In this example, guava
appears in two different versions (27.0.1 and 28.1). This is a classic case of a dependency conflict that needs resolution.
Resolving Dependency Conflicts
Using Dependency Constraints
One effective way to manage conflicts is by leveraging dependency constraints. This allows you to enforce a specific version across your subprojects.
Example
In your root build.gradle
, you can define a dependency constraint as follows:
subprojects {
dependencies {
constraints {
implementation("com.google.guava:guava:28.1-jre") {
because 'We need a unified version of Guava across all projects'
}
}
}
}
This approach ensures that all subprojects will rely on Guava 28.1
, resolving the version conflict.
Excluding Transitive Dependencies
Another way to resolve conflicts is by excluding transitive dependencies where they are not needed.
Example
If one of your projects depends on a library that brings in an undesired version, you can exclude it:
dependencies {
implementation("com.example:some-library:1.0") {
exclude group: 'com.google.guava', module: 'guava'
}
}
Adding this exclusion allows you to control which version of the dependency is included, helping you avoid conflicts.
Using ResolutionStrategy
Gradle's ResolutionStrategy
offers a powerful mechanism for resolving conflicts by specifying how to handle dependency version conflicts globally.
Example
In your root build.gradle
, you could define:
configurations.all {
resolutionStrategy {
failOnVersionConflict()
force 'com.google.guava:guava:28.1-jre'
}
}
In this case, Gradle will throw an exception if two different versions are detected and will force usage of Guava 28.1
.
Why Use ResolutionStrategy?
Using a resolution strategy allows you to define conflict resolution logic in one central place, making it easier to manage dependencies across a multi-project build.
Additional Best Practices
- Regularly Update Dependencies: Keeping your libraries up to date minimizes the risk of legacy conflicts.
- Use BOM (Bill of Materials): For projects that have many dependencies, injecting a BOM can simplify version management by centralizing dependency versions.
- Run Dependency Reports: Make it a habit to run
./gradlew dependencies
regularly during the development phase, especially after adding new libraries.
Final Thoughts
Dependency conflicts in Gradle multi-project builds can pose significant challenges, but they are manageable through careful planning and strategic use of Gradle's features. Whether through dependency constraints, exclusions, or resolution strategies, understanding the intricacies of dependency management is key to maintaining project health.
For additional assistance with Gradle and dependency management, consider exploring the Gradle Documentation and the Gradle User Guide.
By employing these practices, developers can enjoy the advantages of Gradle's powerful dependency management without falling victim to conflicts. Happy coding!