Common Mistakes When Adding RGB Values in Java's SetColor
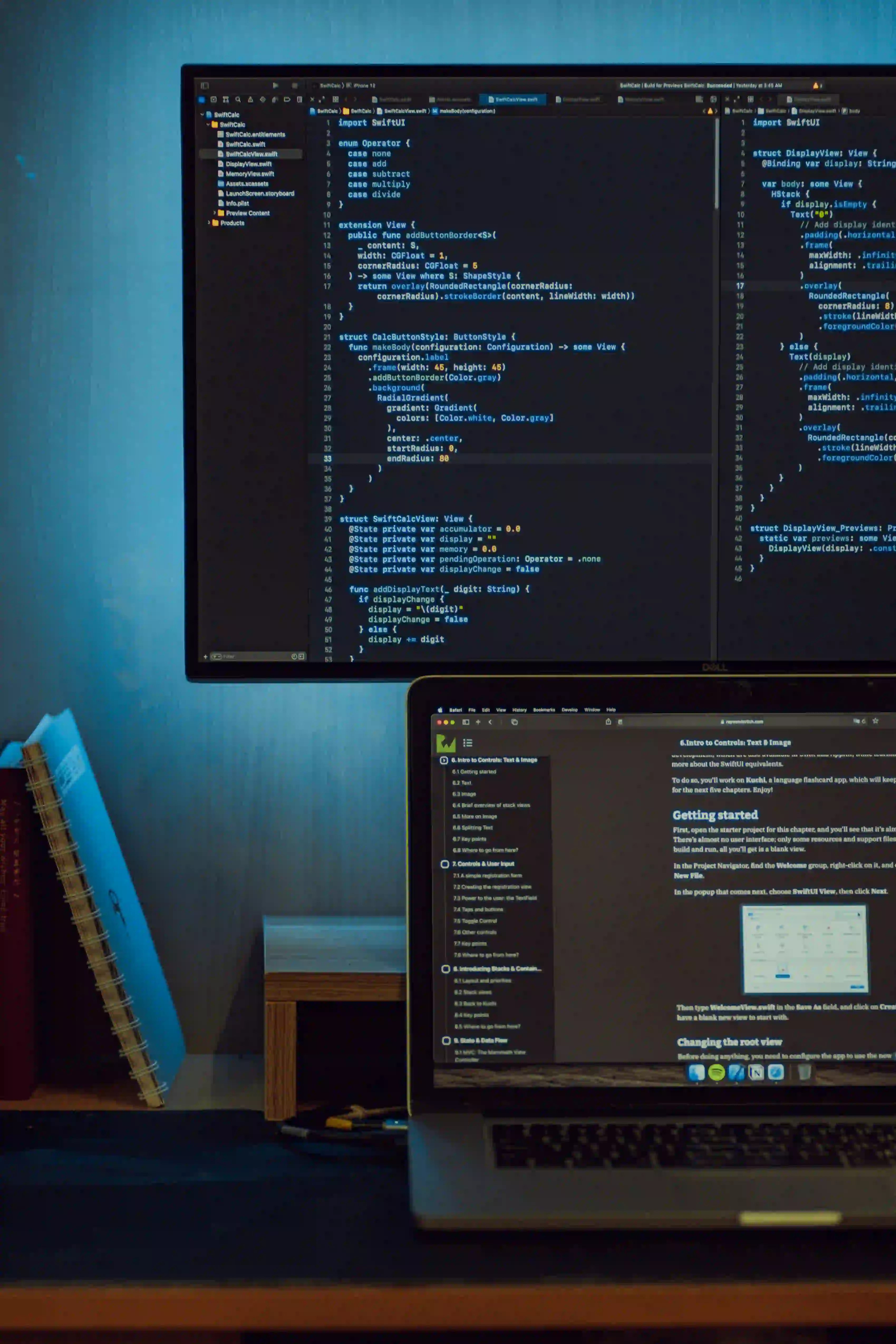
Common Mistakes When Adding RGB Values in Java's SetColor
When it comes to working with color in Java, particularly through the Java AWT (Abstract Window Toolkit) and Swing framework, using RGB values can be both powerful and tricky. The setColor
method is a commonly used approach to define colors in graphical components. However, many developers encounter frustrating pitfalls while trying to manipulate RGB values. This blog post will explore some common mistakes when adding RGB values and provide clarifications and solutions through practical examples.
Understanding RGB Color Model
Before diving into code, it is important to understand the RGB color model. RGB stands for Red, Green, and Blue. It's an additive color model where colors are created by combining these three primary colors in various intensities. In Java, RGB values range from 0 to 255, where:
- 0 means no color,
- 255 represents full intensity.
For example, pure red is defined as (255, 0, 0), while pure blue is (0, 0, 255).
Common Mistakes
1. Incorrect RGB Range
One of the most frequent mistakes when using RGB values in Java is not adhering to the correct range. Although the values must stay within 0 to 255, it's common to see calculations that inadvertently push these values beyond limits.
Example
int red = 260; // Incorrect
int green = 100;
int blue = 50;
Color color = new Color(red, green, blue); // Will cause less-than-ideal behavior
Solution
Always validate RGB inputs before creating a Color object. A good way to do this is by clamping the values.
private int clamp(int value) {
return Math.max(0, Math.min(value, 255));
}
int red = clamp(260); // Now evaluates to 255
Color color = new Color(red, green, blue);
2. Misunderstanding Transparency
Java's Color class does allow for transparency, but newcomers often ignore this feature. This leads to unintended results when rendering graphics, especially when dealing with semi-transparent colors.
Example
Color color = new Color(255, 0, 0, 150); // Semi-transparent red
Transparency Code Explanation
The fourth parameter in the Color
constructor defines alpha (transparency). A value of 255 is fully opaque, while 0 is completely transparent. Semi-transparency can be useful when layering components.
Solution
Consider the alpha channel in your design. To visualize how transparency impacts your graphics, systematically tweak the alpha value.
for (int alpha = 0; alpha <= 255; alpha += 51) {
g.setColor(new Color(255, 0, 0, alpha)); // Varying opacity
g.fillRect(10, 10, 100, 100); // Draw squares with different opacities
}
3. Mixing Colors Incorrectly
Another common mistake is inadequately combining colors, especially by adding RGB values directly. Directly adding colors can yield unexpected results, leading to values that fall outside the 0-255 range.
Example
int red1 = 200;
int green1 = 100;
int blue1 = 50;
int red2 = 80;
int green2 = 200;
int blue2 = 100;
int mixedRed = red1 + red2; // This will be 280 which is invalid
Mixing Code Explanation
In this instance, you should employ an averaging technique or another logical method to mix colors.
Refined Mixing Code
int mixedRed = clamp((red1 + red2) / 2);
int mixedGreen = clamp((green1 + green2) / 2);
int mixedBlue = clamp((blue1 + blue2) / 2);
Color mixedColor = new Color(mixedRed, mixedGreen, mixedBlue);
4. Forgetting to Set the Color
In Java graphics programming, you often need to set the color before using it. Forgetting to do so can lead to the default color being applied.
Example
public void paintComponent(Graphics g) {
g.drawRect(10, 10, 100, 100); // Default color will be used
}
Solution
Always ensure the color is set prior to drawing operations.
public void paintComponent(Graphics g) {
g.setColor(new Color(255, 0, 0)); // Set to red before drawing
g.drawRect(10, 10, 100, 100);
}
Additional Resources
For those looking to dive deeper into Java graphics, consider checking out the official Java AWT Graphics documentation. Similarly, for practical applications and projects, sites like Java2D provide comprehensive tutorials on 2D graphics in Java.
Bringing It All Together
Color handling in Java may seem straightforward at first glance, but it conceals a range of complexities. The key takeaways from this post include:
- Always validate RGB values and maintain the correct range.
- Understand and incorporate the alpha channel for transparency.
- Utilize proper mixing techniques to combine colors effectively.
- Remember to set the color before performing any graphic operations.
By avoiding these common mistakes, you can create visually appealing graphics in your Java applications with greater ease and necessity. Happy coding!