Harnessing Java: Building Apps for Mindful Energy Management
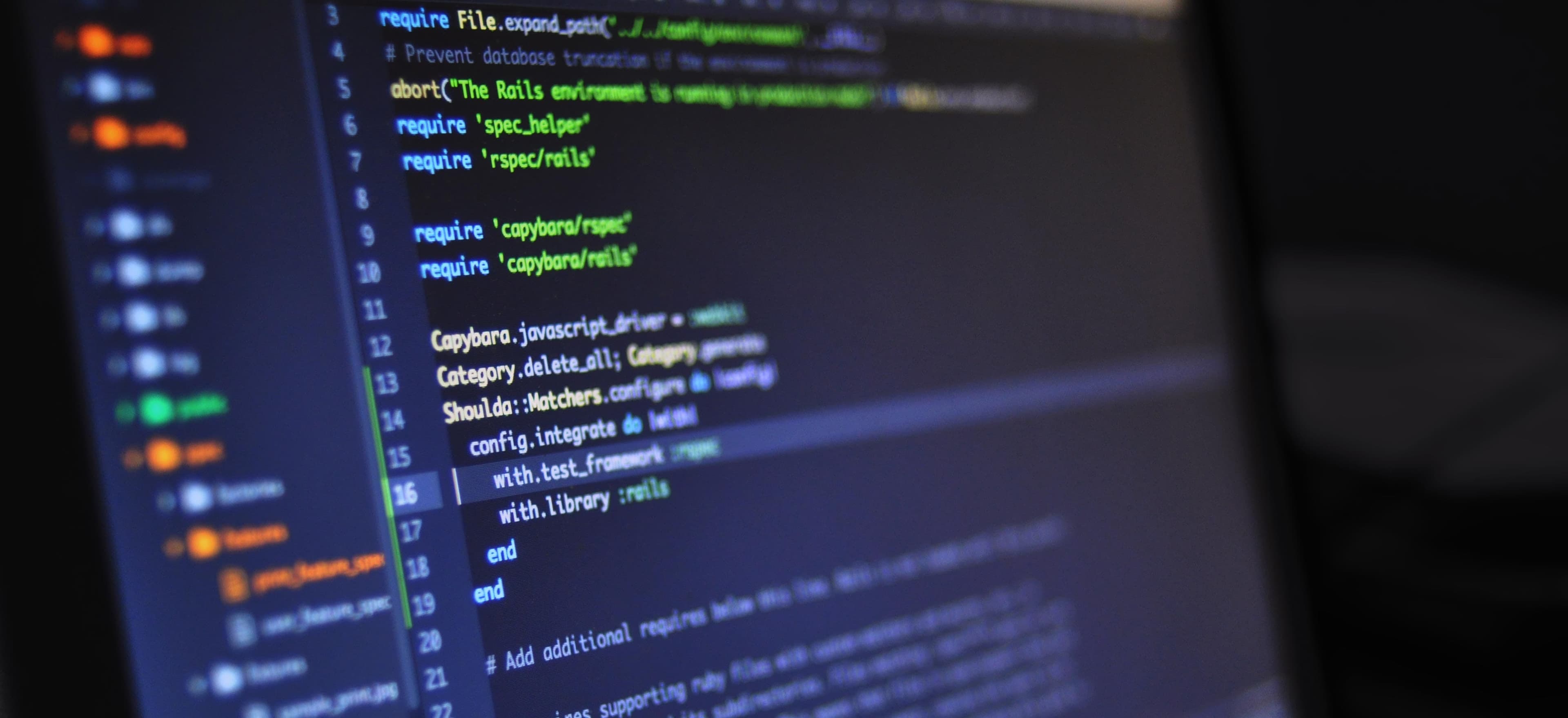
- Published on
Harnessing Java: Building Apps for Mindful Energy Management
In today's fast-paced world, maintaining balance and productivity requires not only physical energy management but also mental clarity. One innovative way to balance these realms is through technology. In this blog post, we will explore how to build a Java application that aids in mindful energy management. Specifically, we will develop an app that encourages meditation practices—an idea resonating with insights from the article, "Strom der Stille: Energiegewinn durch Meditation."
Why Mindfulness and Energy Management Matter
Mindfulness is the practice of being present and fully engaging with the current moment. It has been shown to improve focus, reduce stress, and enhance overall productivity. By integrating mindfulness into our daily routines, we can harness our energy more effectively.
Meditation, as highlighted in the aforementioned article, serves as a powerful tool for energy regeneration. According to studies, just a few minutes of meditation can result in increased focus and decreased feelings of fatigue. In this tech-oriented approach, we'll utilize Java as our development language to create an application that helps users frame their meditation schedules and track their mindful practices.
Setting Up Your Java Environment
Before diving into the code, ensure you have the following installed:
- Java Development Kit (JDK): You can download it from the Oracle website.
- Integrated Development Environment (IDE): Recommended options include IntelliJ IDEA, Eclipse, or NetBeans. Choose one based on your preference.
Creating a New Project
Once you have your environment set up, create a new Java project named MindfulEnergyApp
.
Understanding the Application's Structure
Before coding, let's understand what our application requires:
- User Input: Users need to provide their preferred meditation timings.
- Tracking: We will keep a record of these sessions.
- Notifications: The app will remind users to meditate.
Basic Class Structure
The structure of our application can be represented as follows:
public class MindfulEnergyApp {
public static void main(String[] args) {
// Entry point of the application
UserInterface ui = new UserInterface();
ui.displayMenu();
}
}
This code snippet marks the starting point of our application, where we instantiate the UserInterface
class to display a graphical or console-based menu.
Developing the User Interface
First, let's create a simple console-based user interface. Java's Scanner
class will help us capture user input.
The User Interface Class
import java.util.Scanner;
public class UserInterface {
private Scanner scanner;
public UserInterface() {
scanner = new Scanner(System.in);
}
public void displayMenu() {
System.out.println("Welcome to the Mindful Energy App!");
System.out.println("1. Set Meditation Time");
System.out.println("2. View Meditation History");
System.out.println("3. Exit");
int choice = scanner.nextInt();
handleUserChoice(choice);
}
private void handleUserChoice(int choice) {
switch (choice) {
case 1:
setMeditationTime();
break;
case 2:
viewMeditationHistory();
break;
case 3:
System.out.println("Exiting the application.");
break;
default:
System.out.println("Invalid choice. Please try again.");
displayMenu();
}
}
// Further method implementations will go here
}
Code Commentary
- User Interaction: The
UserInterface
class usesScanner
, allowing users to interact with the application via console inputs. - Switch Case: The
handleUserChoice
method is designed to manage menu navigation efficiently, leading to different functionalities as per user choice.
Setting Meditation Time
Now that we have our main structure, let’s implement the method to set meditation times.
Implementing the setMeditationTime Method
import java.time.LocalTime;
import java.util.ArrayList;
import java.util.List;
public class UserInterface {
private List<LocalTime> meditationTimes;
public UserInterface() {
scanner = new Scanner(System.in);
meditationTimes = new ArrayList<>();
}
private void setMeditationTime() {
System.out.print("Enter meditation time (HH:mm): ");
String inputTime = scanner.next();
LocalTime meditationTime = LocalTime.parse(inputTime);
meditationTimes.add(meditationTime);
System.out.println("Meditation time set for: " + meditationTime);
displayMenu(); // Return to menu after setting time
}
}
Code Explanation
- LocalTime: The
LocalTime
class, part of Java 8's Date and Time API, is used for time manipulation. It adds clarity and ease to handle times. - List Management: We use an
ArrayList
to store multiple meditation times, allowing users to set more than one.
Viewing Meditation History
The next step is allowing users to view their meditation history. This provides a sense of accomplishment and encourages continued practice.
Implementing the viewMeditationHistory Method
private void viewMeditationHistory() {
if (meditationTimes.isEmpty()) {
System.out.println("No meditation times set yet.");
} else {
System.out.println("Your Meditation Times:");
for (LocalTime time : meditationTimes) {
System.out.println(time);
}
}
displayMenu(); // Return to menu after displaying history
}
Code Breakdown
- Conditional Check: The application checks whether the meditation history is empty and informs the user accordingly.
- Looping Through Times: If meditation times exist, the application iterates through and displays each time to the user.
Adding Notification Feature
To enhance our app, we can implement a simple reminder system. This can be done using Java's ScheduledExecutorService
.
Implementing Notifications
import java.util.concurrent.Executors;
import java.util.concurrent.ScheduledExecutorService;
import java.util.concurrent.TimeUnit;
public class Reminder {
private ScheduledExecutorService executorService;
public Reminder() {
executorService = Executors.newScheduledThreadPool(1);
}
public void scheduleMeditationReminder(LocalTime time) {
long delay = calculateDelay(time); // Method to calculate delay until next reminder
executorService.schedule(() -> System.out.println("Time to meditate!"), delay, TimeUnit.SECONDS);
}
private long calculateDelay(LocalTime time) {
// Calculate how many seconds until next meditation time
}
}
Code Commentary
- ExecutorService: This provides an elegant way to manage threads in Java. We create a single-threaded executor for scheduling tasks.
- Reminders: When a user sets a meditation time, it can automatically trigger the reminder.
Lessons Learned: The Road Ahead
Creating a meditation app in Java not only promotes mindfulness and mental clarity but also showcases Java's capabilities in developing user-friendly applications. Each feature—setting times, viewing history, and notifications—is interlinked to enhance user experience and promote energy management.
Equipping oneself with mindfulness techniques is essential in today's world. Drawing on the insights from "Strom der Stille: Energiegewinn durch Meditation," it's clear that digital tools can effectively support these practices.
As we conclude, consider expanding your app by integrating additional features, such as meditation guides or personalized reminders. The road ahead is bright, filled with opportunities to delve deeper into energy management through mindfulness, powered by Java.
In this blog post, we learned how to create a Java-based application dedicated to mindful energy management. We crafted a simple user interface, implemented core functionalities, and explored advanced features like reminders. With the right tools and mindset, you can turn this foundation into a comprehensive app supporting well-being and productivity.
Checkout our other articles