Harnessing Java to Create Mindful Applications for Stress Relief
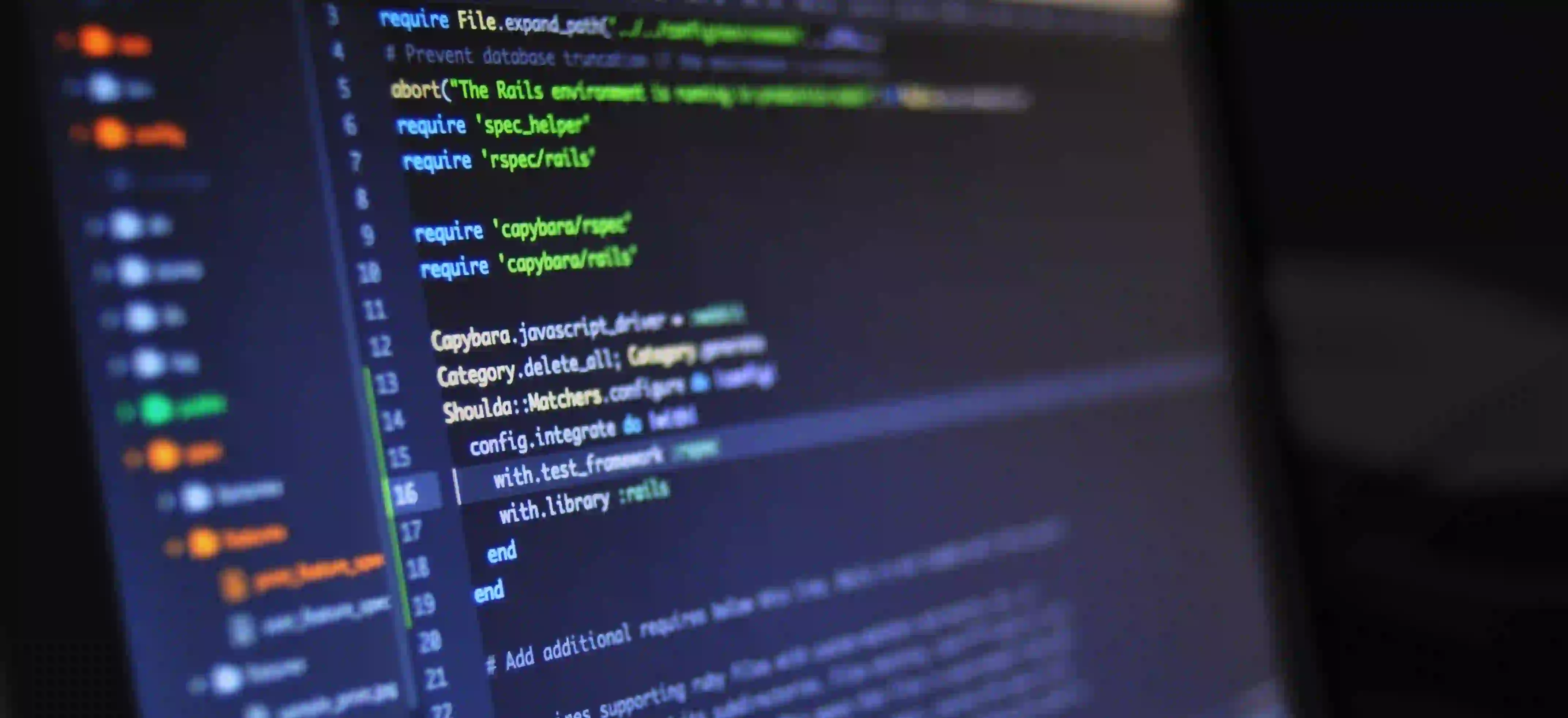
Harnessing Java to Create Mindful Applications for Stress Relief
In our fast-paced world, where stress and anxiety seem to be omnipresent, the appeal of mindfulness and relaxation techniques is growing stronger. The benefits of meditation have been widely discussed, including energy gain and mental clarity. You can explore this topic further in the article “Strom der Stille: Energiegewinn durch Meditation”. But did you ever consider how you might integrate the principles of mindfulness into your software development? This blog post will discuss how you can harness the power of Java to create applications that promote mindful practices, providing not just digital experiences but also aiding mental well-being.
Why Mindful Applications Matter
In today’s tech-centric society, applications that promote mindfulness are not just a trend—they are essential. Studies have shown that meditation can lead to a decrease in stress hormones and an increase in focus. By creating applications that encourage users to meditate and practice mindfulness, you can contribute to their mental health. Imagine developing tools that guide users through meditation sessions, provide reminders for mindful breaks, or track their progress in mindfulness practices.
What Does Java Bring to the Table?
Java is an exceptional language for building such applications due to its platform independence, robust security features, and extensive library ecosystem.
- Platform Independence: Java applications operate seamlessly across different platforms, whether on desktop, web, or mobile.
- Object-Oriented: The principles of object-oriented programming (OOP) in Java allow for modularity and easy maintenance.
- Rich Libraries: Java supports a variety of libraries that can enhance your application with features like audio playback, data visualization, and user interface designs.
Getting Started: Setting Up Your Java Environment
Before we get into the development process, let’s ensure you have everything set up properly.
-
Download Java Development Kit (JDK): Make sure you have the latest version of the JDK installed on your machine. You can find it here.
-
Choose an Integrated Development Environment (IDE): Some popular IDEs include IntelliJ IDEA, Eclipse, and NetBeans. For this post, we will use IntelliJ IDEA for its intuitive interface.
-
Create a New Project: Open your IDE, select "Create New Project," and choose Java.
Building a Simple Meditation Timer
Let’s create a simple meditation timer application. This app will allow users to set a duration for their meditation, and at the end of the timer, it will play a sound to indicate that the session is complete.
Step 1: Define the User Interface
In Java Swing, the GUI components can be designed using JFrame, JPanel, and JButton. Here’s a basic example:
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class MeditationTimer {
private JFrame frame;
private JTextField timeInput;
private JButton startButton;
public MeditationTimer() {
frame = new JFrame("Meditation Timer");
timeInput = new JTextField(10);
startButton = new JButton("Start Meditation");
frame.setLayout(new FlowLayout());
frame.add(new JLabel("Enter time in seconds: "));
frame.add(timeInput);
frame.add(startButton);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setSize(300, 150);
frame.setVisible(true);
}
public static void main(String[] args) {
new MeditationTimer();
}
}
Commentary
- JFrame: Acts as the main window for our application.
- JTextField: Allows the user to enter the number of seconds for their meditation session.
- JButton: When clicked, this will start the timer.
Step 2: Implement the Meditation Timer Logic
Next, we need to add functionality to the start button to initialize the countdown.
startButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
int duration = Integer.parseInt(timeInput.getText());
startMeditation(duration);
}
});
private void startMeditation(int duration) {
Timer timer = new Timer(duration * 1000, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
JOptionPane.showMessageDialog(frame, "Meditation session is over!");
((Timer) e.getSource()).stop();
playSound();
}
});
timer.setRepeats(false);
timer.start();
}
private void playSound() {
// This method is where you can implement sound after meditation ends
}
Commentary
- Timer: We use a Swing Timer to handle the countdown. At the end of the duration, it triggers an action to show a dialog.
- JOptionPane: This is helpful for displaying pop-up messages, alerting the user that their session has ended.
Step 3: Adding Sound for Completion
Finally, to enrich the user experience, let's implement a sound notification to indicate that the meditation session has ended.
private void playSound() {
try {
java.applet.AudioClip clip = java.applet.Applet.newAudioClip(new java.net.URL("file:/path/to/your/sound/file.wav"));
clip.play();
} catch (MalformedURLException e) {
e.printStackTrace();
}
}
Commentary
- AudioClip: This built-in Java class plays sound files. Ensure that you have a valid audio file to notify the end of the session.
Expanding Your Application
Feature Enhancements
- User Profiles: Let users create profiles to track their meditation history.
- Guided Meditations: Integrate audio guidance during meditation sessions.
- Reminders: Implement reminders to encourage consistent practice.
Natural Integration of Mindfulness Features
Your app should seamlessly integrate mindfulness principles. Consider including breathing exercises, calming visuals, or nature sounds to enhance the user's experience.
Lessons Learned
Creating mindful applications using Java offers an opportunity to contribute positively to mental health. By developing features that promote meditation and mindfulness practices, you engage users in an activity that can vastly improve their quality of life. The simple meditation timer we discussed serves as a foundation, and from here, your creativity can take flight. As you innovate, remember to focus on user experience, as it plays a significant role in the success of mindfulness applications.
To explore further about how meditation impacts energy gain, consider reading Strom der Stille: Energiegewinn durch Meditation. Embrace the fusion of technology and well-being, and let the benefits of mindfulness permeate through your software development journey!
Further Reading
The journey into mindful programming is just beginning—embrace it!