Avoiding Azure Blob Storage Pitfalls in Java Applications
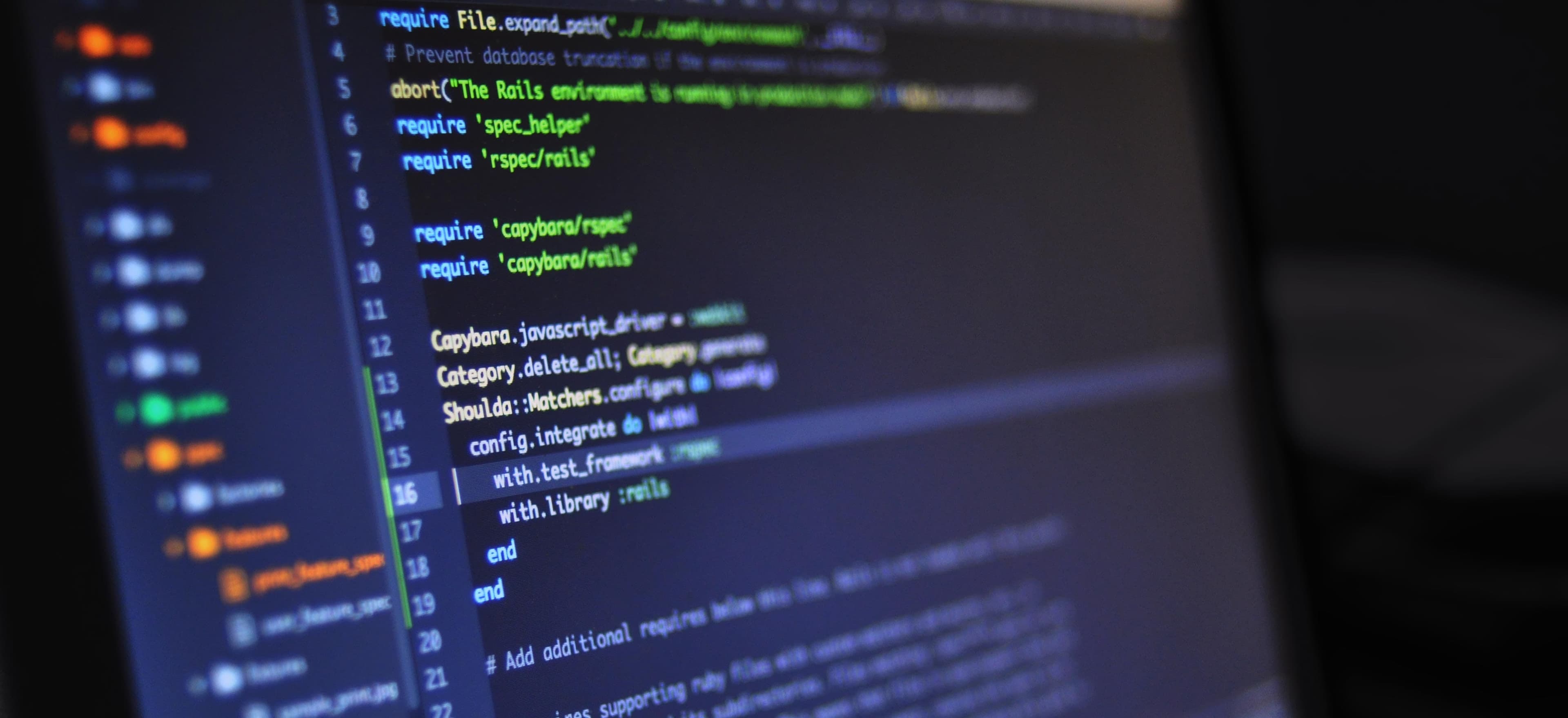
- Published on
Avoiding Azure Blob Storage Pitfalls in Java Applications
As a Java developer, integrating with cloud storage solutions like Azure Blob Storage can be an exciting venture. Cloud storage not only allows for scalable data storage but also streamlines application development and deployment. However, transitioning to a cloud environment comes with its own unique challenges and pitfalls that developers need to be aware of. In this post, we will explore some common pitfalls you should avoid while working with Azure Blob Storage in your Java applications, along with best practices and code examples.
Understanding Azure Blob Storage
Before we dive deep into the pitfalls, let's briefly understand what Azure Blob Storage is. Azure Blob Storage is a service for storing large amounts of unstructured data, including text or binary data. The data is stored as blobs in various tiers based on access frequency, which allows for cost-efficient storage management.
Key Features of Azure Blob Storage:
- Scalability: Supports massive volumes of data.
- Accessibility: Can be accessed through REST APIs.
- Durability: Data is stored across multiple locations ensuring high availability.
- Access Control: Azure provides various mechanisms, including shared access signatures (SAS) for securing data access.
Common Pitfalls When Using Azure Blob Storage
When integrating Azure Blob Storage into Java applications, developers often encounter a range of pitfalls. Let’s look at a few of them along with their solutions.
1. Incorrect Configuration of Azure SDK
One common mistake is misconfiguring the Azure SDK for Java. Using the wrong version or not setting the necessary credentials can lead to authentication errors.
Solution:
Make sure you are using the latest version of the Azure Storage SDK for Java and properly configure your credentials. Here’s how you can initialize the BlobServiceClient with authentication.
import com.azure.storage.blob.BlobServiceClientBuilder;
BlobServiceClient blobServiceClient = new BlobServiceClientBuilder()
.connectionString("<YourConnectionString>")
.buildClient();
Why this matters: Using a proper connection string ensures that your application securely connects to Azure Blob Storage, preventing potential access issues.
2. Not Implementing Proper Exception Handling
Errors can occur at any point during your interaction with Azure Blob Storage, whether during uploads, downloads, or deletions. Failing to implement a robust exception handling mechanism can lead to obscure bugs and application crashes.
Solution:
Always use try-catch blocks around your Azure Blob Storage operations. Here is an example:
try {
BlobClient blobClient = blobServiceClient.getBlobContainerClient("mycontainer").getBlobClient("myblob.txt");
blobClient.uploadFromFile("path/to/local/file.txt", true);
} catch (BlobStorageException e) {
System.err.println("Blob storage exception: " + e.getMessage());
} catch (Exception e) {
System.err.println("General exception: " + e.getMessage());
}
Why this matters: Exception handling allows developers to gracefully respond to errors, providing a better user experience and easier debugging.
3. Ignoring Performance Optimization
Another pitfall is overlooking performance optimization, especially when handling large files or a significant number of transactions. Azure offers features like concurrency and parallel uploads to improve performance.
Solution:
Implement parallel uploads to enhance the performance of large file uploads. Here’s an example of how to do that with the Azure Blob SDK:
import com.azure.storage.blob.models.BlobHttpHeaders;
BlobClient blobClient = blobServiceClient.getBlobContainerClient("mycontainer").getBlobClient("mylargefile.txt");
blobClient.uploadFromFile("path/to/largefile.txt", true, new BlobHttpHeaders().setContentType("text/plain"), null, null, null);
Why this matters: Parallel uploads can drastically reduce the time needed to upload large files, improving the efficiency of your Java applications.
4. Forgetting to Set the Right Access Policies
Failing to set the appropriate access policies can result in unauthorized access, or alternatively, overly permissive access that could compromise data security.
Solution:
Make sure to define and manage your Azure Blob Storage access policies appropriately. A sample code to create a Shared Access Signature (SAS) might look like this:
LocalDateTime expiryTime = LocalDateTime.now().plusHours(1);
String sasToken = blobClient.generateSasUrl(BlobSasPermission.parse("r"), expiryTime);
System.out.println("SAS Token: " + sasToken);
Why this matters: Properly configured access policies enhance the security of your application, allowing only the intended users or services to access specific resources.
The Bottom Line
While Azure Blob Storage offers immense capabilities for managing unstructured data in Java applications, developers need to be vigilant about common pitfalls. By understanding the importance of configuration, exception handling, performance optimization, and access policies, you will not only ensure a smoother integration process but also create a robust and efficient application.
For more detailed insights into Azure Blob Storage and its common pitfalls, check out this insightful article on "Common pitfalls to avoid when hosting on Azure Blob Storage".
By avoiding these pitfalls, you can harness the full power of Azure Blob Storage in your Java applications, setting the stage for scalable and efficient architectures in the cloud. Happy coding!
Checkout our other articles