Avoiding Java Misconfigurations with Azure Blob Storage
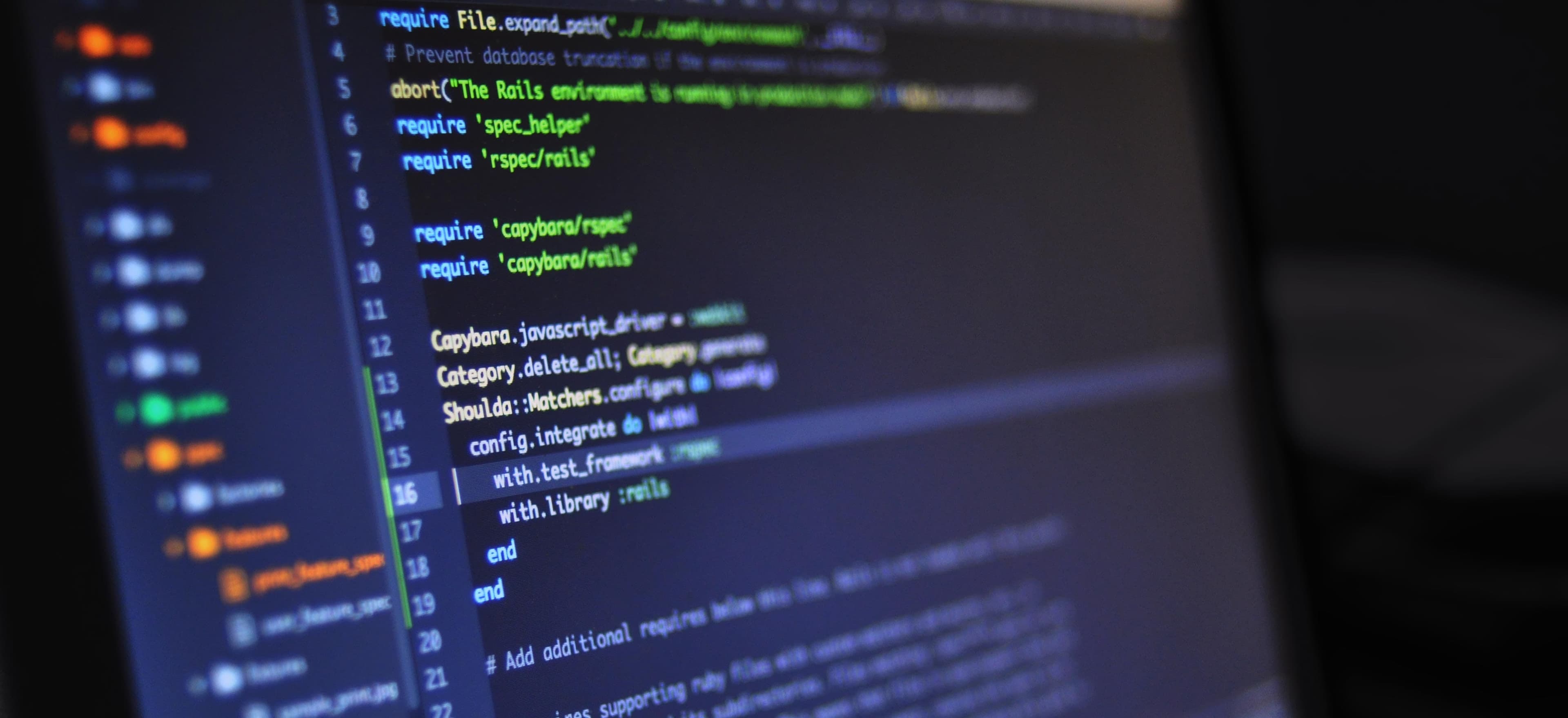
- Published on
Avoiding Java Misconfigurations with Azure Blob Storage
Azure Blob Storage is one of the most widely used services for storing unstructured data in the cloud. However, developers using Java to interact with this powerful service often encounter misconfigurations that can lead to disruptive errors and inefficiencies. In this blog post, we will discuss common misconfigurations and how to avoid them when working with Azure Blob Storage using Java, ensuring a smoother development process and a more resilient application.
Essentials at a Glance
Java developers frequently face challenges when integrating with cloud services like Azure. Azure Blob Storage, which allows for the storage of massive amounts of unstructured data, is a robust solution—but improper configuration can lead to application downtime or data loss. Let's delve into techniques and best practices to prevent these pitfalls.
Prerequisites
Before starting, ensure that you have the following:
- An Azure Subscription
- The Azure SDK for Java
- A basic understanding of Java and cloud storage concepts
Setting Up Your Environment
To interact with Azure Blob Storage, you'll need the Azure Storage SDK. At the moment of writing, the latest version can be included in your Maven project as follows:
<dependency>
<groupId>com.azure</groupId>
<artifactId>azure-storage-blob</artifactId>
<version>12.13.0</version>
</dependency>
To install it, add the above snippet to your pom.xml
file. Make sure you're using the latest version of the library, as it comes with performance enhancements and important bug fixes.
Common Misconfigurations
When working with Azure Blob Storage in Java, you might experience issues stemming from:
- Connection Strings
- Blob Permissions
- Error Handling
- Data Redundancy Options
1. Connection Strings
Using incorrect connection strings can lead to authentication failures.
Code Example
Here’s how to properly initialize a BlobServiceClient with a connection string:
import com.azure.storage.blob.BlobServiceClient;
import com.azure.storage.blob.BlobServiceClientBuilder;
public class AzureBlobExample {
private final static String CONNECTION_STRING = "your_connection_string_here";
public BlobServiceClient createBlobServiceClient() {
return new BlobServiceClientBuilder()
.connectionString(CONNECTION_STRING)
.buildClient();
}
}
Why: Always use a secure method to store and retrieve your connection string, such as application settings or managed identities, to avoid hardcoding it in your source code. This ensures that your credentials are protected from unauthorized access.
2. Blob Permissions
Default blob permissions can lead to unauthorized access or, conversely, can cause applications to fail if permissions are too restrictive.
Code Example
To set appropriate permissions when uploading a blob, you can use:
import com.azure.storage.blob.models.BlobHttpHeaders;
public void uploadBlobWithPermissions(String containerName, String blobName, byte[] data) {
BlobContainerClient containerClient = createBlobServiceClient().getBlobContainerClient(containerName);
containerClient.createIfNotExists();
BlobClient blobClient = containerClient.getBlobClient(blobName);
blobClient.upload(BinaryData.fromBytes(data), true);
// Set blob metadata or HTTP headers here if needed
blobClient.setHttpHeaders(new BlobHttpHeaders().setContentType("application/octet-stream"));
}
Why: Define permissions adequately and review them regularly, especially for public access settings. Always prefer finer-grained permissions over blanket permissions to enhance security.
3. Error Handling
Inadequate error handling can lead to vague exceptions and application crashes.
Code Example
A robust try-catch block helps manage errors gracefully.
public void downloadBlob(String containerName, String blobName) {
try {
BlobClient blobClient = createBlobServiceClient().getBlobContainerClient(containerName).getBlobClient(blobName);
BlobProperties properties = blobClient.getProperties();
System.out.println("Blob size: " + properties.getBlobSize());
// Perform download operation
} catch (BlobStorageException e) {
System.err.println("Blob not found: " + e.getMessage());
// Further actions based on specific error codes
}
}
Why: Identifying and handling specific errors allows for better recovery strategies and user notifications, minimizing the impact of failures on the user experience.
4. Data Redundancy Options
Choosing the wrong data redundancy option can affect both cost and data integrity.
Azure Blob Storage offers several redundancy options:
- Locally Redundant Storage (LRS)
- Geo-Redundant Storage (GRS)
- Read-Access Geo-Redundant Storage (RA-GRS)
Code Example
Setting redundancy options can be done during the creation of a storage account:
import com.azure.storage.blob.models.BlobServiceProperties;
public void configureBlobStorageRedundancy(String accountName) {
BlobServiceClient blobServiceClient = createBlobServiceClient();
BlobServiceProperties properties = blobServiceClient.getProperties();
// Example of setting LRS
properties.setDataLakeAnalyticsAccountRedundancyOptions("LRS");
blobServiceClient.setProperties(properties);
}
Why: Always evaluate your application's needs before deciding on a redundancy option. LRS is cheaper but offers less protection against regional failures, while GRS can safeguard against such scenarios at a higher cost.
The Bottom Line
Avoiding misconfigurations when utilizing Azure Blob Storage in your Java applications is essential for performance, security, and reliability. Always review your connection strings, permissions, error handling, and redundancy settings to ensure a robust integration.
For more insights into Azure Blob Storage and the common pitfalls developers face, you can check out the article titled "Common pitfalls to avoid when hosting on Azure Blob Storage" at infinitejs.com/posts/common-pitfalls-azure-blob-storage.
By adhering to the best practices and guidelines shared in this post, you'll significantly improve your Java applications' interaction with Azure Blob Storage and minimize unwarranted issues. Happy coding!
Checkout our other articles